Manage your Zoom Meetings on WhatsApp with Python and Twilio
Time to read: 10 minutes
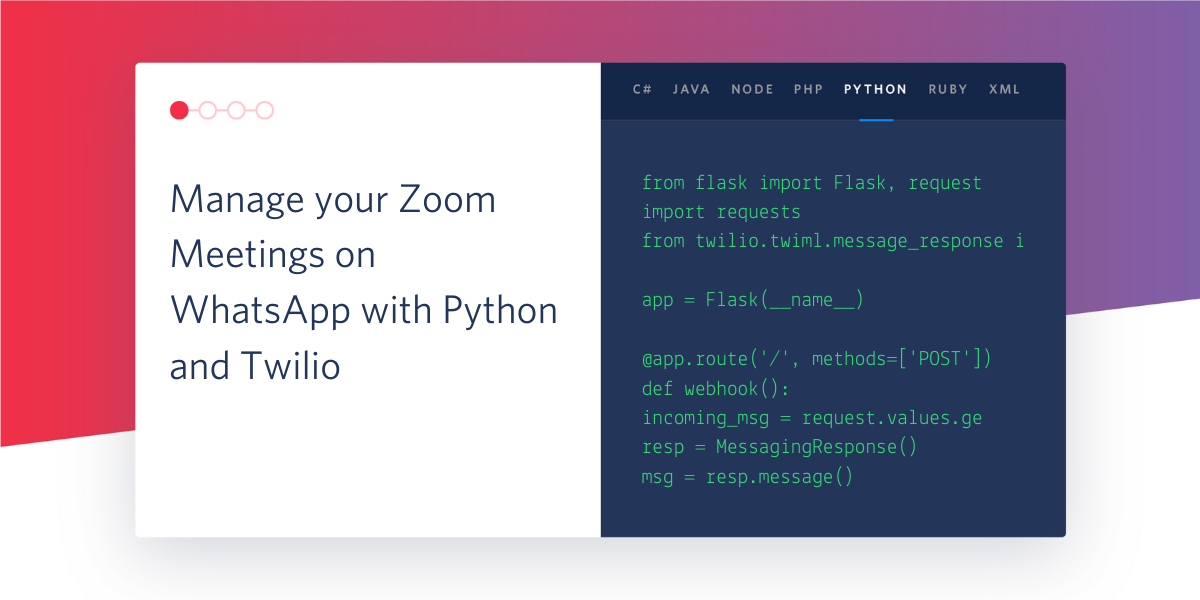
In this tutorial, we are going to build a WhatsApp bot that allows you to manage your Zoom meetings. Some of the functions include creating or deleting a meeting and listing all upcoming meetings. The bot will be built using Twilio API for WhatsApp, Zoom API and the Flask framework for Python.
Tutorial requirements
You need the following to follow along in this tutorial;
- A Twilio Account: You need to create a free Twilio account to gain access to the WhatsApp sandbox environment. This will allow you to interact securely with the Twilio number assigned to you on your WhatsApp application. A free twilio account comes with certain features and limitations.
- A Zoom Account: You need to create a free Zoom account if you do not already have one. With a zoom account, you can create a zoom app which comes with access to their API.
- Python 3.6 or newer. Python installers for different versions and OS are available for download here.
- A smartphone with an active phone number and WhatsApp account.
- Ngrok: ngrok allows you to securely expose your local server to the public internet. you can find instructions to download and install ngrok here.
Setting up the Development Environment
Now that we have set up the Twilio WhatsApp sandbox environment and we have active Zoom API credentials, we will start creating the application. First, we have to create a directory for this project and create a python virtual environment in that directory.
We will use python’s inbuilt venv
module to create the environment and install the following packages.
- The Twilio Python Helper Library to interact with the Twilio API
- The Flask framework module for creating the web application
- The Zoomus package which is the Python client library for the Zoom REST API
Enter the following commands in a bash terminal to complete the tasks described above.
If you are following this tutorial on a Windows computer, enter the following commands in the command prompt window to setup your development environment:
For Unix or Mac OS users, enter the following commands instead:
Configure the Zoom API
In order to gain access to all of Zoom’s collection of resources, we need to create a Zoom app which will grant us access to their APIs. The Zoom App will grant you access to mirror some of its popular features such as creating a meeting, deleting meetings, listing meetings e.t.c. If you don’t have a zoom account you have to sign up for one.
Once you have created an account, you need to navigate to Zoom App Marketplace to create an App. On the navigation bar, click on (1) “Develop” and then on (2) “Build App”. Finally, click on (3) “Create” in the “JWT” box. Give your application a name such as Meeting Bot and click “Create”.
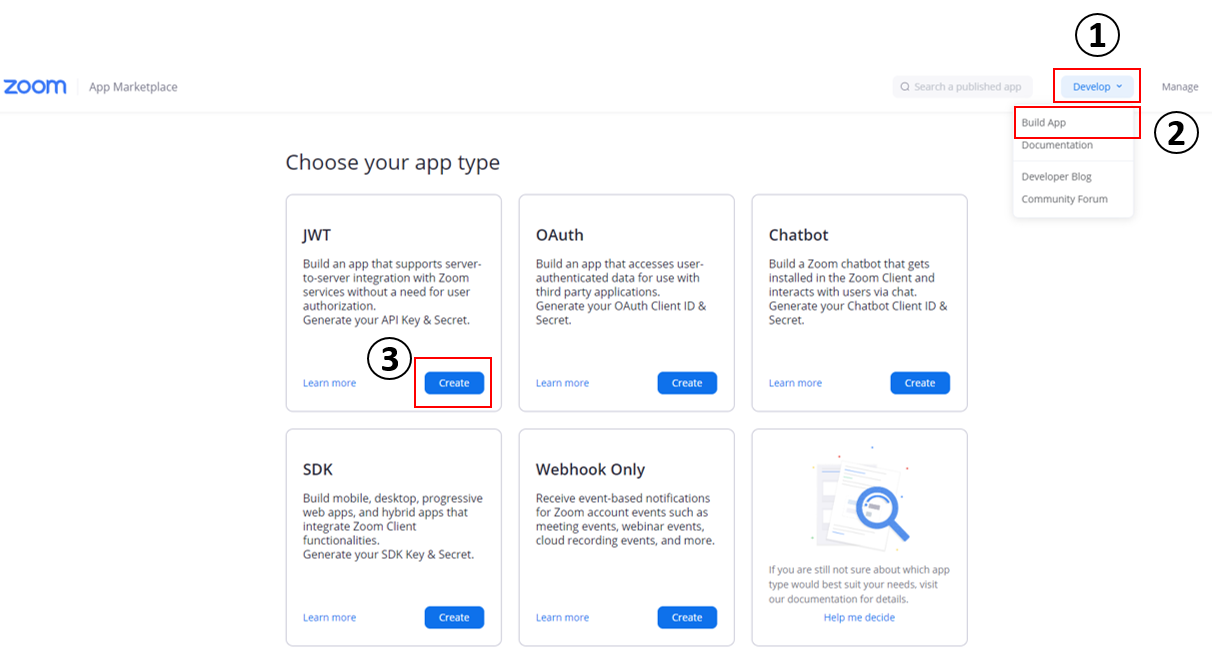
To activate the application and gain access to the API credentials you need to fill in the basic information listed below:
- “Company name”
- “Developer Name”
- “Developer Email Address”
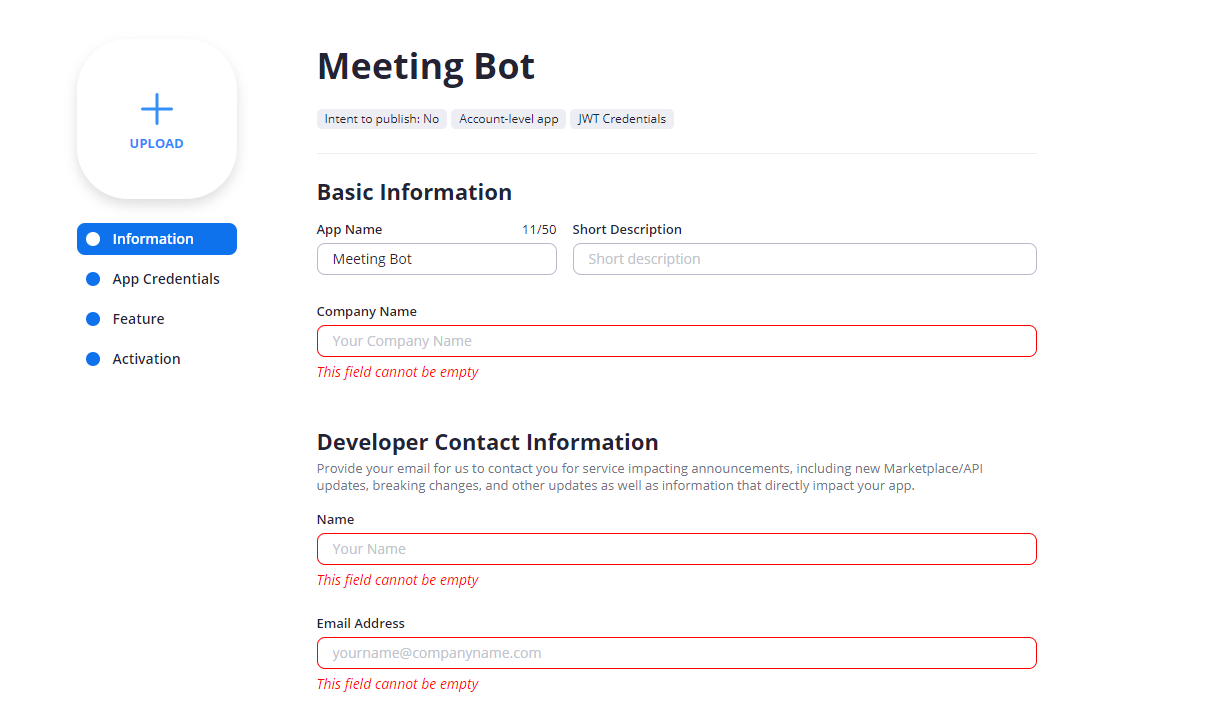
Once that is done, you click “Continue” and then click on “App Credentials” on the sidebar to view the API Credentials (API Key, API Secret & JWT Token).
Now we are going to store our Zoom API key, API secret, and email as environment variables. Create a .env
file in the root directory and enter the following variables in it:
Remember to replace the placeholders with the actual values.
In the next section we will test the API.
Testing the API
To test the API credentials that were provided, we are going to retrieve our user information by using the zoom client to call the zoom endpoint with our email address stored in the 'USER_EMAIL' environmental variable.
We will create a test.py
file and add the code block below to import the necessary packages and environmental variables needed to test the API.
Then we will add the code block that makes the get request to pull our user information from Zoom.
Now, execute the ‘test.py’ file to verify that the API credentials were correctly set up.
Setting up the Twilio WhatsApp Sandbox
One of the dependencies of this project is a free Twilio Account that provides you with a WhatsApp sandbox to create and test your application. The free tier account comes with a trial balance that allows you to exchange messages for a period of time, after which you are billed for what you use. Once your WhatsApp application is production ready, you need to get approval from WhatsApp before it can be deployed on your own phone number.
Click here to set up your sandbox environment. From the Twilio Console, click on the icon with the 3 dots on the left navigation bar and click on “Programmable Messaging”, then click on “Try it Out”, in the ensuing drop down, select “Try Whatsapp”. On the screen, you will see a message asking you to send a custom code from your device to the Sandbox number via WhatsApp.
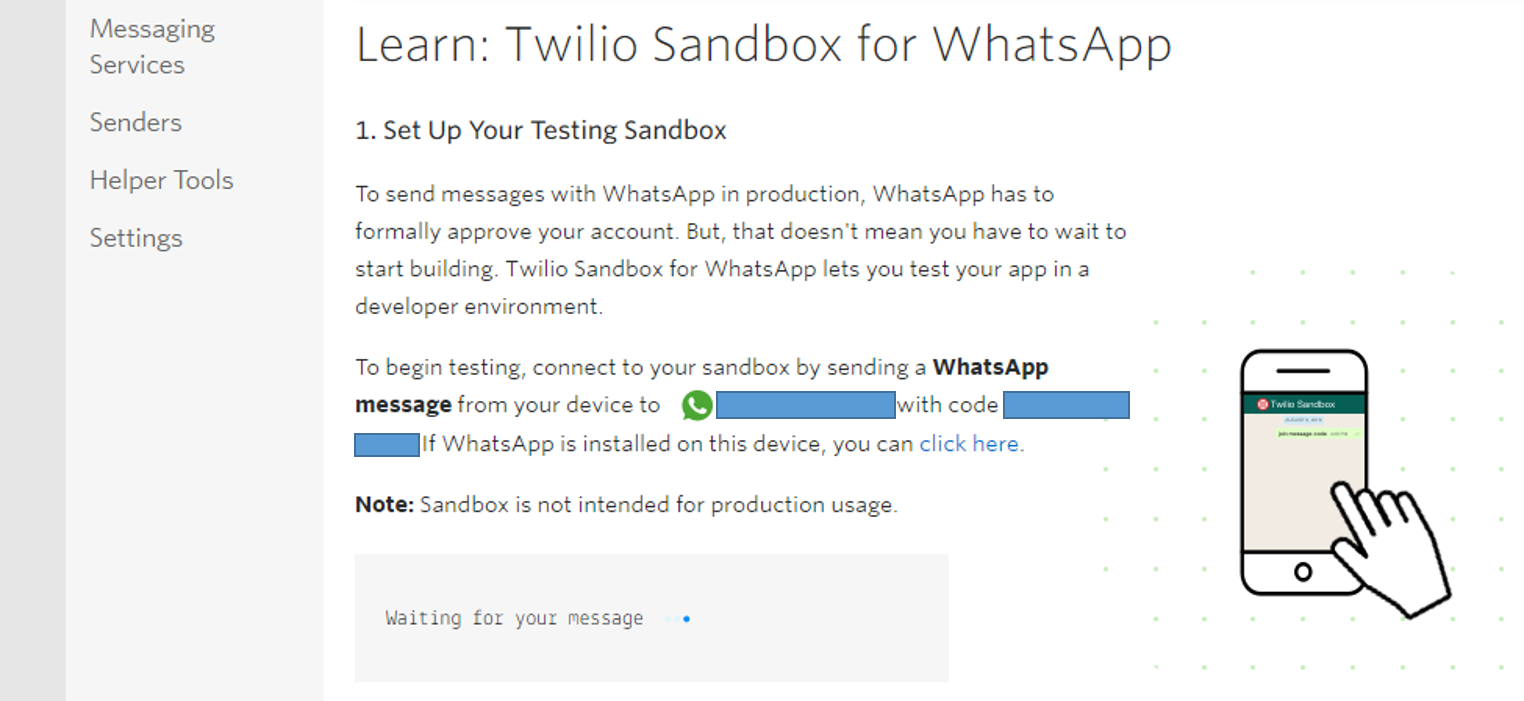
Once that is done, you will get a notification on your twilio console and on your device indicating that your phone number is now linked to your Sandbox.
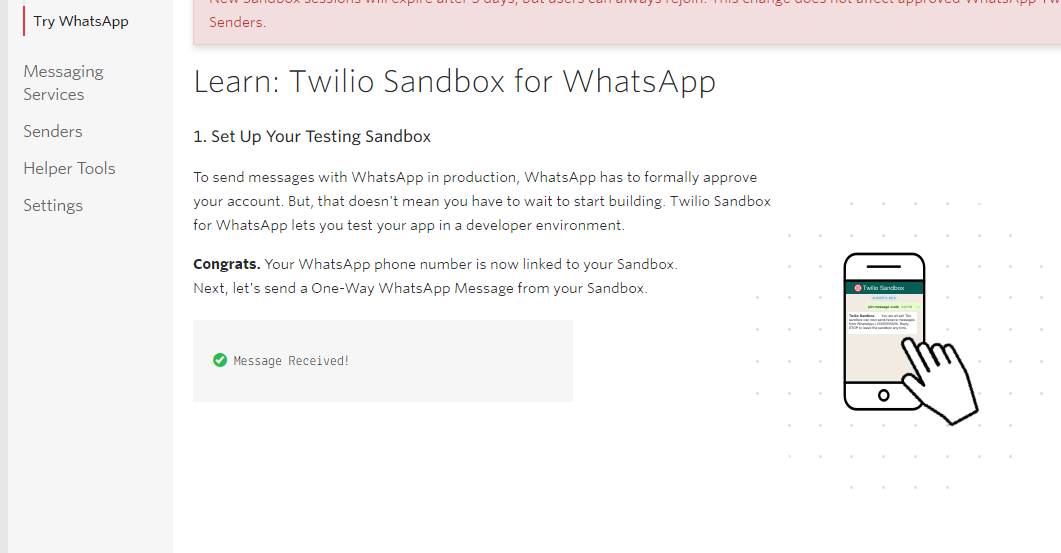
You can link more phone numbers with your sandbox environment by repeating the same steps above.
Creating the Flask Chatbot Service
Now that our development environment is set, we can begin creating the chatbot service. The meeting management bot we are going to create will be a simple one, it will communicate and interact with users by looking out for certain keywords in the users response in some cases and in others the bot will provide the users with options to select from and extract their responses.
The Twilio WhatsApp API allows us to interact with users using webhooks.
Outgoing Messages
Now, I’m going to introduce you to the Twilio Markup Language, also known as TwiML. TwiML is an XML document with special tags defined by Twilio to help you build your SMS and voice applications. To process outgoing responses to the user, Twilio expects a TwiML response from our webhook.
Here’s a simple TwiML message to respond to an incoming message
Twilio provides a Python package that abstracts the creation of XML responses. Instead of writing an XML code, you can send a response message to the user with the code below:
Incoming Messages
Incoming messages from the user are included in the Body
of Twilio POST
requests. We will retrieve the messages sent by the user to the chatbot using the request
object provided by Flask. We will use the code below to access incoming messages from the user.
Flask Sessions
A session object is a dictionary object that allows us to store user information in key value pairs. We are going to use sessions to store the information collected from the user.
Secret keys are needed to secure client-side sessions and you can generate one with the code below from a Python terminal
Now, copy the code generated and add it to your .env
file, assigning it to a variable as shown below
Chatbot
The application that we are building will configure an endpoint that Twilio will communicate with using a webhook. The Flask framework is a simple, lightweight and extensible framework that allows us to easily define webhooks. Now, we are going to create a file called app.py
in the top-level directory of our project. This file is going to house most of our application code.
To begin, we will import all the necessary libraries and environment variables into our application by adding the following lines of code to the ‘app.py’ file
Next, we will create a regex pattern to validate the meeting time format provided by the user in a later part of the application, and also create the Zoom Client that will be used to manage our meetings.
So, we will add the following code immediately after the last line of code in our file to perform the actions described above
Now it is time to instantiate the Flask application. We will do this by defining a route at the /meeting
endpoint which supports POST
requests. Add the code below to the app.py
file.
If you are using source control, remember to add the .env file to your .gitignore file so you don’t commit your API credentials to a public repository by mistake.
Helper Functions
Next, we will create some helper functions to make our work easier. These functions will be added directly beneath the route function.
We will define functions to:
- Convert time from one timezone to another
- List out all the scheduled meetings for a particular user
- Create meetings
- Get the details of a meeting
- Delete meetings
- Create an initial reply to the user
Convert Timezone
We will create a function to convert time from one timezone to another. The zoomus
Python package does not account for different timezones which is why we need to create a function to offset the GMT time difference. Copy the code that follows at the end of app.py
:
List Meetings
Next we will create a function that returns a list of meetings scheduled for a particular user. The function sends a GET
request to the Zoom meetings endpoint with the user's email as a parameter.
Create Meeting
Next we are going to create a function that schedules a zoom meeting. This function is going to take in arguments such topic, agenda, duration, start time) collected from the user.
The function will return a status code and dictionary containing the created meeting information.
Get Meeting
The next function checks if a meeting exists using its meeting ID. For this we use the get
method of the zoom client meeting component.
Delete Meeting
This function is going to delete a scheduled meeting.
Initial Response
The last helper function returns a custom TwiML response to the user to kickstart the conversation.
Conversational Flow
The bot will interact with the user by presenting them with a list of options in some cases and in others, the bot directs questions, provides them with the format to respond in, and validates their responses using regular expressions. The bot will process every response that comes in from the user. The responses will be stored in the Flask user session for further processing.
Now, let’s get into building the conversational flow. First we will configure our chatbot to send a custom greeting to the user using the initial_response()
helper function that was defined above. We will add this code in the meeting
route function to check if the user has sent “Hello” or “Hi”. We will also add another block to send a default response to the user when they send a message that is not recognized to the bot.
At this point, we expect the user to have selected one of the three options. We are going to create conditional blocks to process each of the user’s requests.
If their response is 1
, this means that the user wants to list out their scheduled meetings. Since we do not need additional information from the user to perform this action, we would simply use the EMAIL_ADDRESS
as an argument to call the list_meetings()
function to return all upcoming meetings.
We will add the code snippet that does this after the ‘msg = resp.message()’ assignment inside the ‘meeting()’ function.
If their response is 2
, this means that the user wants to schedule a meeting. To create a meeting we need to collect some information from the user such as duration of the meeting, meeting topic, meeting agenda and start time, and include it in the body of the API request. You can view the list of all the other parameters here.
Next, we will ask the user for each of the variables above one by one. We will add the block of code directly below the previous one to respond to the user’s request by asking them to provide the meeting start time.
To complete the part of the application that deals with creating a meeting; we will validate the format of the meeting start time that was provided using the regex pattern regex_start_time
that was created earlier.
The latter part of this code snippet takes care of storing the meeting topic in the session variable and asking the user for the meeting duration. This block is an extension of the previous conditional block.
Up next, we will store the provided meeting duration in the session dictionary and proceed to create the meeting using the Zoom Client.
To ensure the meeting was successfully created, we will check that the response code of the API call is ‘201’. If the meeting was created successfully, we will respond to the user with the meeting information contained in the returning JSON variable.
This is also an extension of the previous code block and should be placed directly below it.
If the user’s initial response is 3
, this means that the user wants to delete an already scheduled meeting. To delete a meeting, we only need the meeting id.
Thus, we need to introduce a new conditional block to handle the delete request but first, we will add few lines of code to request the meeting id from the user:
Next, we will validate the meeting id provided by the user using the ‘get_meeting()’ function. If the meeting id is valid, we will delete the meeting using the ‘delete_meeting()’ function that we created earlier.
We will then check that the delete action was successful using the response code, and then respond to the user with a message showing that the meeting was successfully deleted or not.
Everything Together
Your app.py file should look like this:
Testing the Chatbot
To test the application, first we will ensure that the virtual environment is activated and then run the command below.

Now that the application is running on port 5000 on our local machine, we are going to run the ngrok
command to make our application temporarily available on a public server that Twilio can reach. We will execute the command below on a second terminal window.
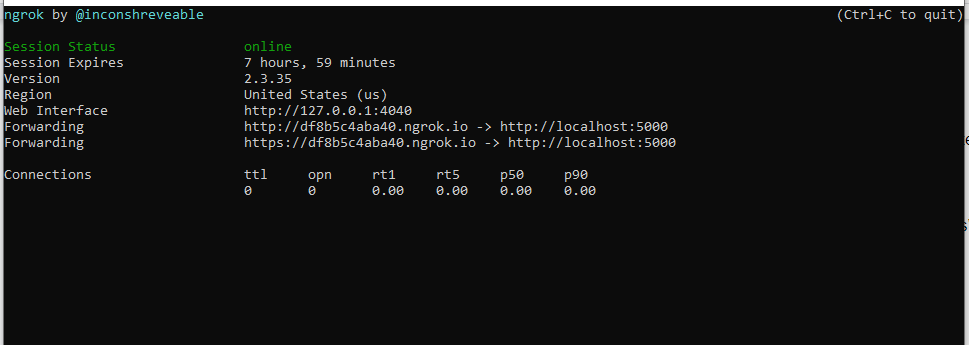
Now copy the secure URL shown in the “Forwarding” lines. Ngrok uses this URL to redirect requests to our application.
Navigate to the Twilio Console, click on Programmable Messaging > Settings > WhatsApp Sandbox Settings. Paste the URL on the “When a message comes in” field, with /meeting
appended at the end. The /meeting
path is where we installed the endpoint of our application.
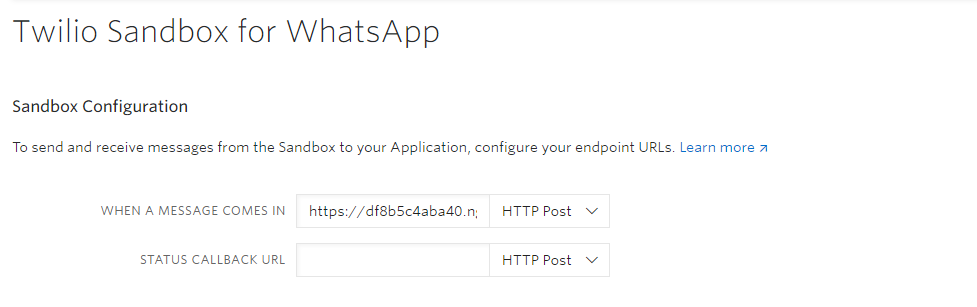
Now, send “Hello” to the WhatsApp sandbox number to begin the conversation with the bot!
Conclusion
With the recent surge in remote work and virtual workspaces, it has become imperative that we find ways to keep track of the myriad of meetings and calls required to go about our daily tasks. In this tutorial, we have successfully created a bot to effectively manage our Zoom meetings on WhatsApp, one of the popular messaging applications.
The repository for the code can be found here. The application can be extended to do much more than it currently does. An extended list of other capabilities that can be built into this application can be found on the Zoom API reference page.
Happy coding!
My name is Odunayo and I’m passionate about technology and literature. I also enjoy writing to share experiences and knowledge. Feel free to connect on LinkedIn.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.