Receive and Respond to Text Messages with Server-Side Swift, Perfect, and Twilio
Time to read: 2 minutes
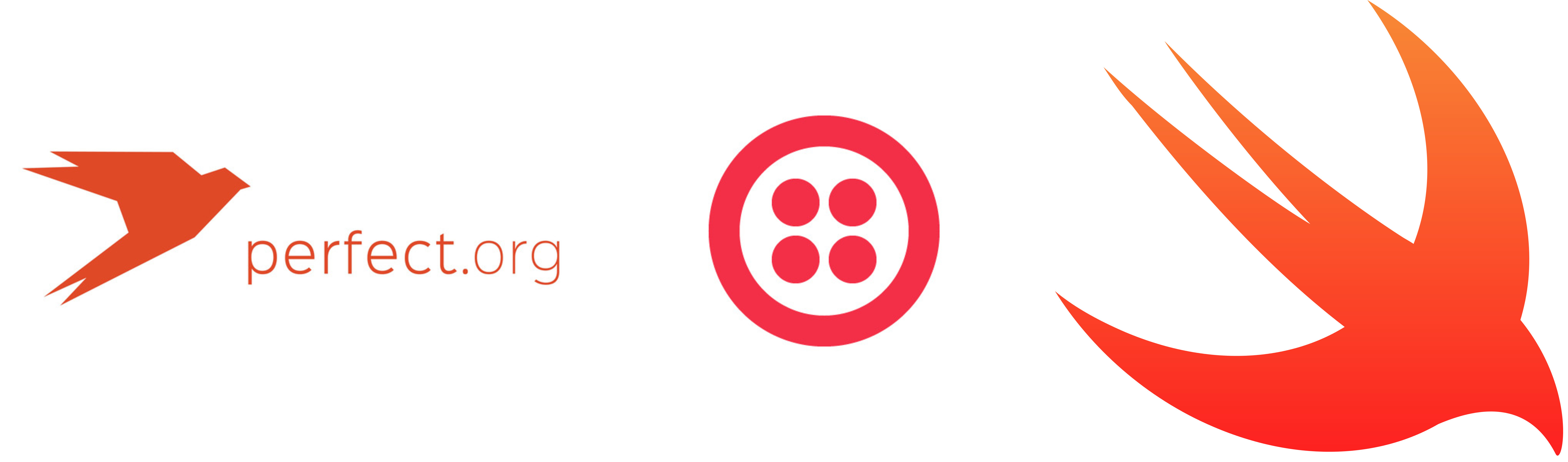
Perfect is a versatile open source server-side Swift framework and toolset that makes it easy for developers to quickly create server- and client-side apps. Let's see how easy it is to send SMS with Twilio and Perfect.
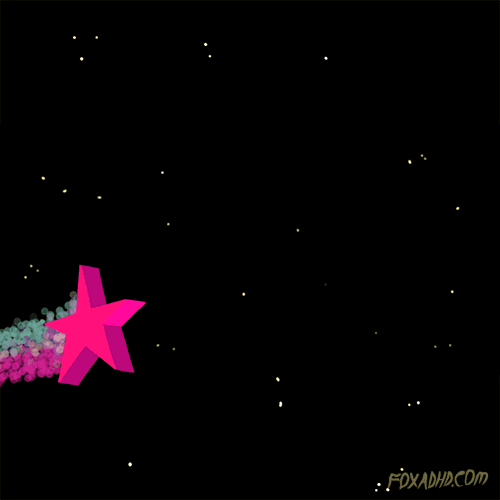
Setup
To follow along with this post we'll need
Install Perfect using the Swift Package Manager. Create a new project directory called PerfectSMS
and then on the command line in your project directory, run
This generates a package with the same name as your current directory.
- Package.swift at the top-level of your project contains your package description and your package’s dependencies.
- Sources/ is home to all your Swift source files, including main.swift, which will be the entry point for your project. It currently prints hello, world to the Terminal.
- Tests/ will contain unit tests you can write using XCTest. We'll be ignoring these for the simplicity of this post.
In Package.swift, add this line to your Dependencies:
And then this line to your Target Dependencies array:
The complete file should look like this:
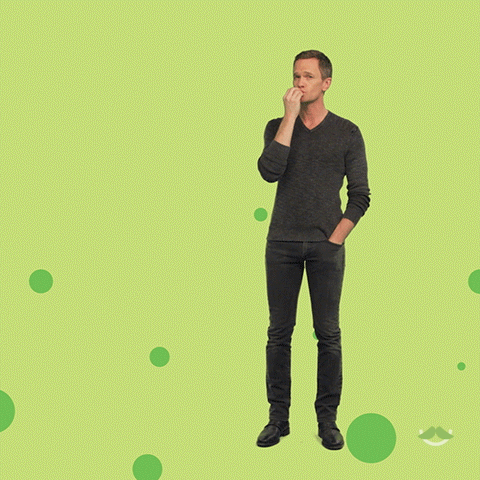
Setting up your Twilio Account
If you haven't bought a Twilio phone number yet, buy one now. In your current directory, run the command
on the command line. If you just installed ngrok and that command gives you an error, you may have to instead run
from the directory that the ngrok executable is in.
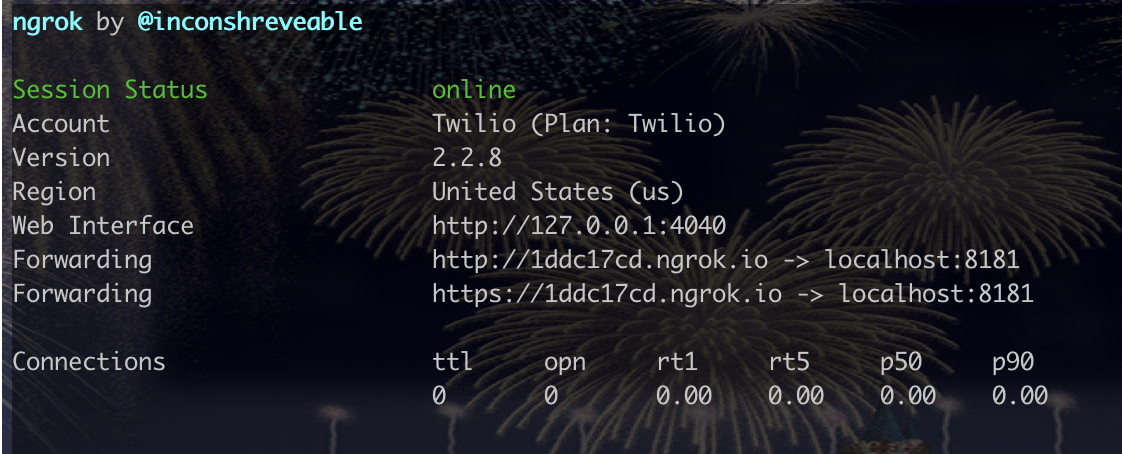
This gives us a publicly-accessible URL to your application. Configure your Twilio phone number as shown below by adding your ngrok URL with /sms appended to it to the “Messaging” section below:
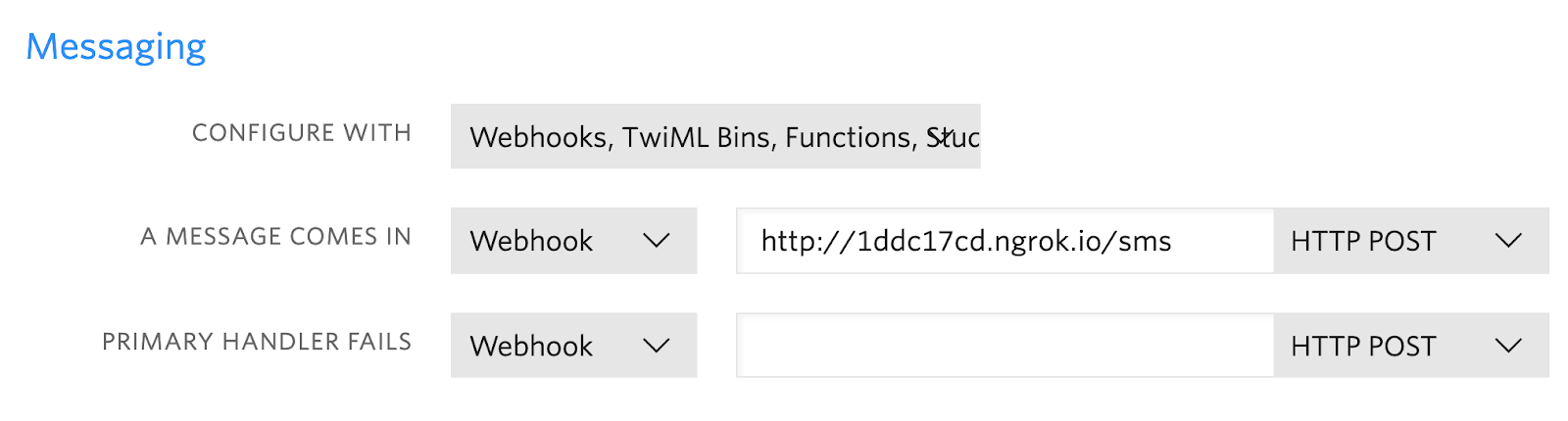
Send an SMS with Perfect and Twilio
In Sources/PerfectSMS/main.swift, import the following libraries at the top.
Then create a route to handle different requests based on the HTTP request method and request path. The POST /sms
route below will respond to incoming SMS messages.
This code creates a respStr containing TwiML that unwraps the inbound text that is an optional and responds from our Twilio number with the same message that was sent. Now let's launch a HTTP Server, passing it our route we just made.
On the command line in a new tab, run
When your terminal prompts you with a question to "allow incoming connections" click "allow." Now try sending a text message to your Twilio number and you should get a response back containing the same message you sent.
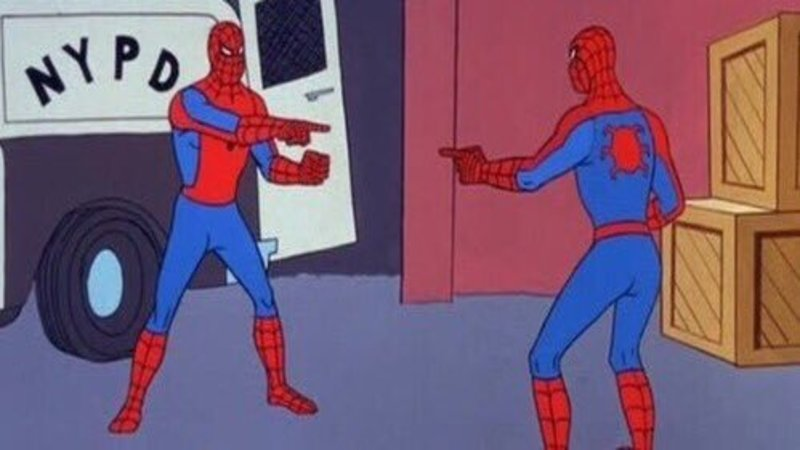
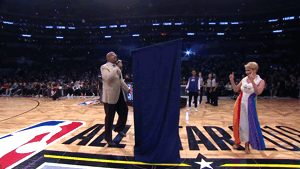
You can also use Perfect in other environments like Heroku, Amazon Web Services, Docker, Microsoft Azure, Google Cloud, and IBM Bluemix CloudFoundry.
Other neat Twilio and Swift posts include Receiving and Responding to Text Messages with Server Side Swift, Vapor and Twilio, Handling Incoming Phone Calls with Server Side Swift, Vapor and Twilio, and How to receive a POST request with server side Swift using Vapor.
I'd love to hear about what you're building with Twilio in Swift. Feel free to leave a message in the comments below or find me on Twitter @lizziepika, GitHub @elizabethsiegle, or via email lsiegle@twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.