How to Schedule Automated SMS Messages Using Twilio and GitHub Actions
Time to read:
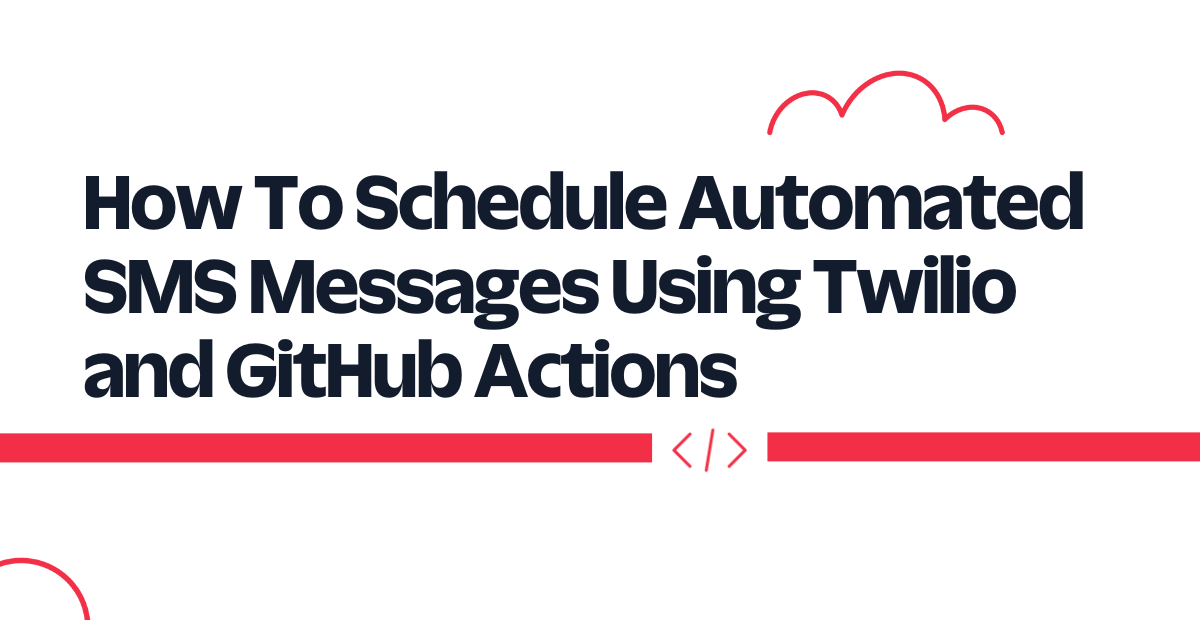
This tutorial will teach you how to run a scheduled task using GitHub Actions. We’ll build a Python app that sends you random motivational quotes, deploy this app to GitHub, and then configure GitHub Actions to run the app on a daily basis.
Technical requirements
To follow along, you’ll need the following:
- A free Twilio account
- A Python Development Environment running Python 3.9+
- A GitHub account
Creating a Python environment
Let’s create a directory where our project will reside. From the terminal, run the following command:
Next, cd
into the project directory and run the following command to create a virtual environment.
To activate the virtual environment on a Mac or Linux machine, run the following command:
If you are using a Windows computer, then the activation command is different:
Next, we’ll install all the dependencies our project will be needing:
- twilio: A Twilio helper library for interacting with Twilio’s REST API.
- python-dotenv: A library for importing environment variables from a .env file.
Next, run the following command to install all of the dependencies at once:
Building the App
For the purpose of this tutorial, you’ll use a JSON file to store all the motivational quotes you’ll be sending to yourself. At the root of your project directory, create a quotes.json file and paste the following into the file:
All the quotes here were obtained from ZenQuotes.
Next, create a main.py at the root of your project directory and add the following code to the file:
At the top of this file, all the major dependencies the project will be needing have been imported:
- The
load_dotenv()
function is used to load environment variables.
- The
twilio_client
object will be used for interacting with the Twilio API to send the SMS notification. TheTWILIO_ACCOUNT_SID
andTWILIO_AUTH_TOKEN
environment variables loaded by theload_dotenv()
function will be automatically used to authenticate against the Twilio service. - A random quote is then fetched from the quotes.json file you created earlier and is then sent via SMS to a preconfigured phone number.
Create a GitHub Actions workflow file
GitHub Workflows usually reside in a /.github/workflows directory within a repository. Within your project directory, run the following command:
This will create a .github/workflows directory. Next, run the following command to create a sms.yml file within that directory:
Add the following code to the file you just created:
The on
value of the workflow includes the schedule
value which is used to trigger a workflow to run at a scheduled time. You can schedule a workflow to run using the POSIX Cron Syntax. In this case, the workflow will be triggered every day at 8:00 UTC. You can learn more about the schedule
event here.
The daily_sms
job installs Python version 3.9 and then subsequently runs the main.py
script.
Setting up Twilio
After you sign up for an account on Twilio, head over to your Twilio Console and click on Phone Numbers. If you already have one or more phone numbers assigned to your account, select the number you would like to use for this project. If this is a brand new account, buy a new phone number to use on this project.
On your Twilio Console, take note of your Account SID and Auth Token. You are going to need these values to authenticate with the Twilio service.
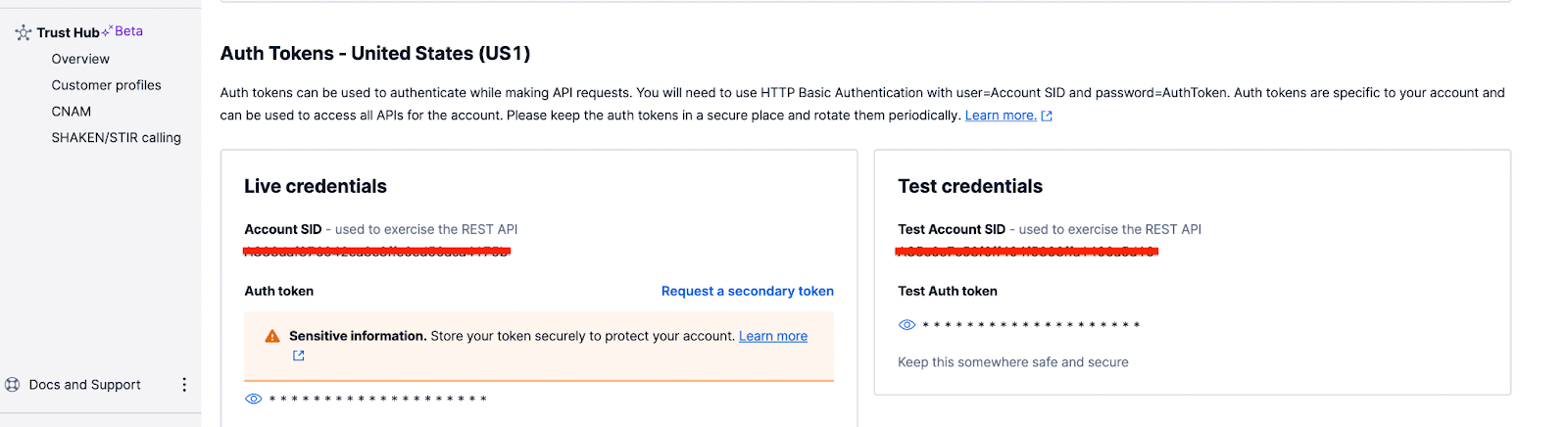
Next, head back to the project’s directory and create a .env file. Edit the file with all the credentials and settings we’ve noted thus far:
The TO_PHONE_NUMBER
here refers to the actual number that will be receiving the SMS and should be in the canonical E.164 format.
Since you'll be pushing this codebase to GitHub shortly, it’s important to make sure you don’t mistakenly commit sensitive credentials to GitHub. Create a .gitignore file and add the following to the file:
Testing
Before committing all your changes and pushing to GitHub, let’s make sure everything works as expected locally. To start the application, open a terminal window, activate the virtual environment and run the following command:
The phone number you configured earlier in the .env file should have received a random motivational quote via SMS.
Once you confirm everything works as expected, you can create a repository on GitHub and then push the code changes to the repository.
After pushing your changes to GitHub, you need to configure certain environment variables the workflow needs to run. Head over to the main page of your repository and select “Settings”. Under the “Security” section of the sidebar, select “Secrets and variables” and then click “Actions”. Select the “Secrets” tab and then click “New repository secret”. You can now add each of the environment variables you defined earlier and their corresponding values.
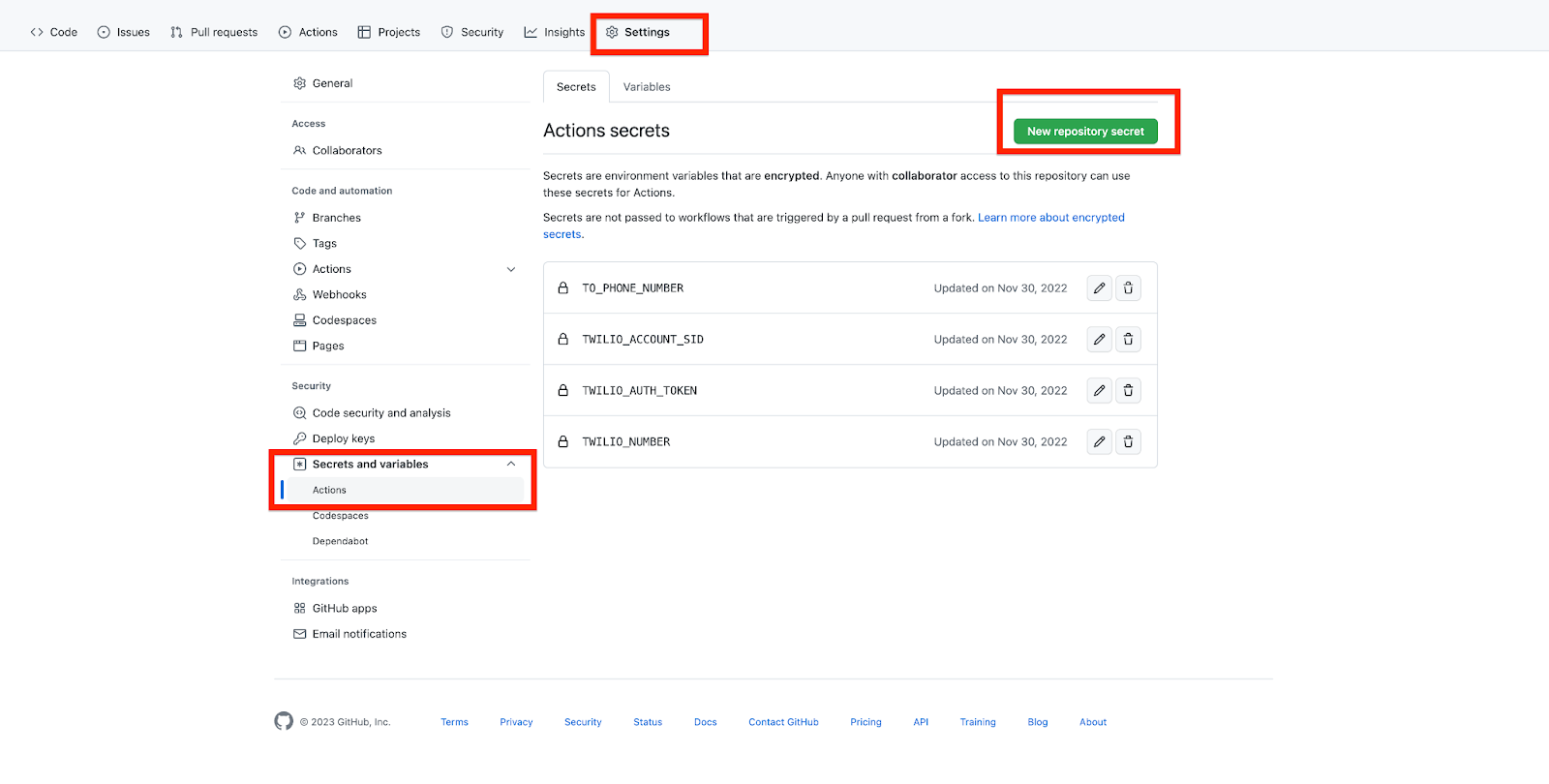
Once you have added all 4 secrets, your workflow will have all it needs to automatically send you a new motivational quote via SMS every day at 8:00 UTC. If you like, feel free to change this to a different time of your choice.
Conclusion
In this tutorial, we’ve seen how, by using Twilio SMS and GitHub Actions, we can run a scheduled task on a predefined interval. This can serve as a low cost way of running a scheduled task without deploying your code on an actual server.
Dotun is a backend software engineer who enjoys building awesome tools and products. He also enjoys technical writing in his spare time. Some of his favorite programming languages and frameworks include Go, PHP, Laravel, NestJS, and Node.
Website: https://dotunj.dev/
GitHub: https://github.com/Dotunj
Twitter: https://twitter.com/Dotunj_
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.