How to Send Bulk Emails in PHP using Twilio SendGrid
Time to read:
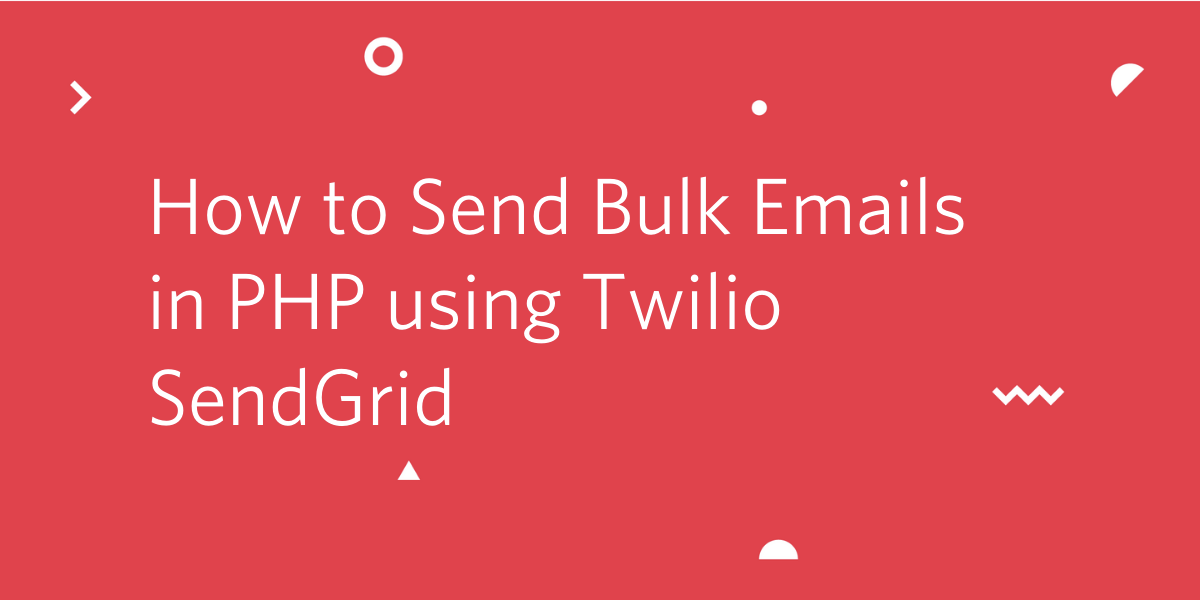
Frequent and relevant communication with attendees is necessary when planning an event. There are too many opportunities for confusion if timely and detailed messages are not sent. Even when everything goes according to plan guests still need reminders as the event date draws closer.
Bulk emails are a great method to keep everyone in the loop.
In this tutorial, we will learn how to send bulk emails to an email list in PHP using Twilio SendGrid.
We’ll use a foreach() loop to parse through an array of email addresses and build a single array of recipients using SendGrid’s personalization class. SendGrid allows us to personalize each email that every recipient receives. We can even modify content based on the individual email address.
Tools Needed to Complete This Tutorial
To complete this tutorial you’ll need the following dependencies installed:
- A Twilio SendGrid Account
- Composer globally installed
Get Started with Twilio SendGrid
Take a look at the Send Transactional Emails in PHP with Twilio SendGrid tutorial to get started. You’ll pick up from where that tutorial ends. You can also begin this tutorial by cloning the relative repository as follows:
Create the Code to Send a Bulk Email
Now that you’ve completed the first part of this tutorial, you’re ready to create the code to send bulk emails. Open up email.php
in the root directory and replace the contents with the following code:
The Personalization class works by appending an array of personalizations to an existing Mail
object. You’ll notice the first recipient is assigned to the newly created $email
object. Each additional recipient is defined in the $email_addresses
array.
The SendGrid Mail object only accepts one primary recipient, so we will add each additional recipient by using the Personalization class. The $email_addresses
are iterated over using a foreach
loop. Each pass instantiates a new Personalization object and assigns the recipient using the addTo
method. Once each Personalization is added to the parent Mail object $email
they are all sent using the same logic as the first tutorial.
Testing
Run the following command in your console window from the root directory: php email.php
A successful execution will display emails sent!
in your console window, preceded by the status code and headers from our email.
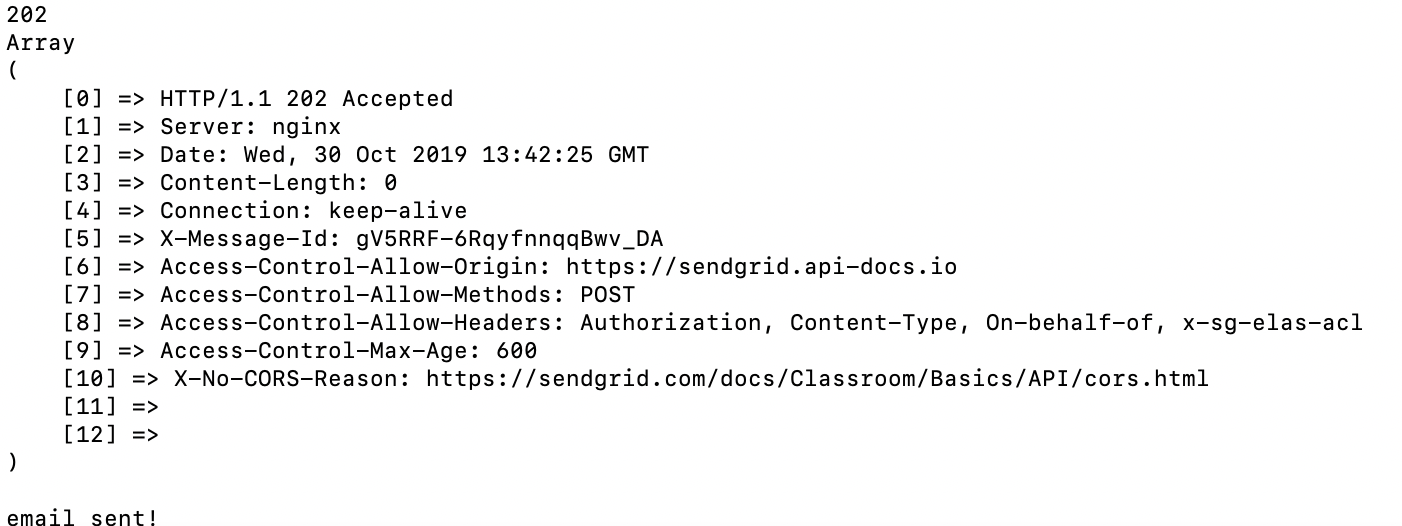
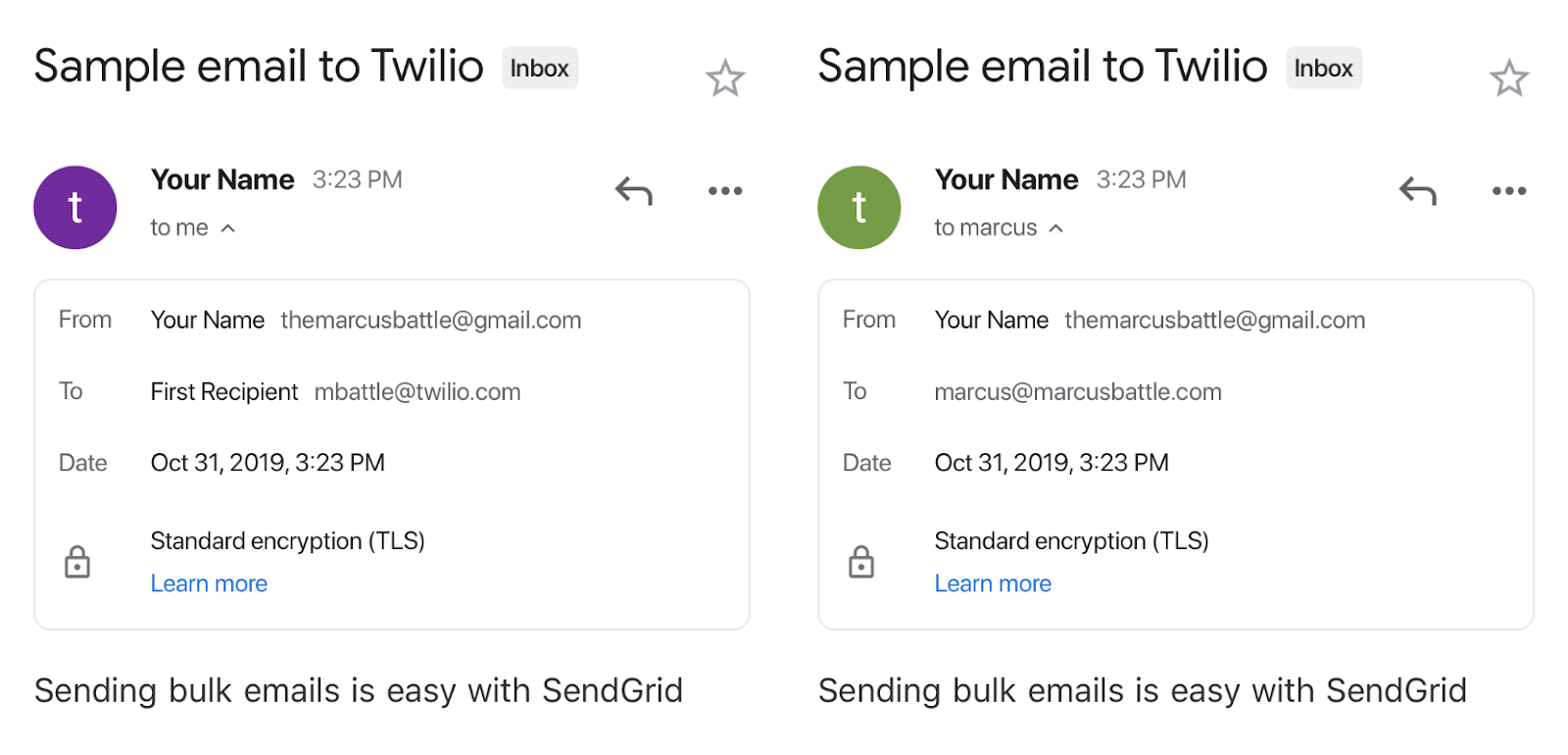
Conclusion
SendGrid really makes sending bulk emails easy, coincidentally increasing confidence that all your recipients stay up to date with mass notifications.
Take a look at the personalization class to see how you can fine-tune each email sent to your recipients.
Marcus Battle is the PHP Developer of Technical Content at Twilio. He is committed to prompting and rallying PHP developers across the world to build the future of communications. He can be reached on Twitter at @themarcusbattle and via email.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.