How to Send an SMS in 30 Seconds with Ruby
Time to read: 4 minutes
Twilio is all about powering communication, regardless of the language—or language framework—that you're using for a given project.
In this tutorial, you'll learn how to send an SMS directly from a Ruby application. What's more, you're going to use Twilio's Ruby Helper Library to make sending an SMS even faster than it would otherwise be. Ready to go? Let's dive in!
Tutorial requirements
To complete the tutorial, you will need the following things:
- A Twilio account (free or paid). If you are new to Twilio, click here to create a free account
- Ruby, version 2.7 or later. If you don't already have Ruby installed, you can install it using your operating system's package manager (if you're using Linux or macOS) or download it.
- Bundler
- A smartphone with active service, to test the project.
- The Twilio CLI (optional)
Get your Twilio phone number
As we're sending an SMS, you're also going to need a Twilio phone number, one that is SMS capable.
If you already have a Twilio phone number, you can retrieve it through the Twilio Console. To do so, go to "Phone Numbers > Active Numbers" and copy a phone number that is SMS capable from the "Number" column.
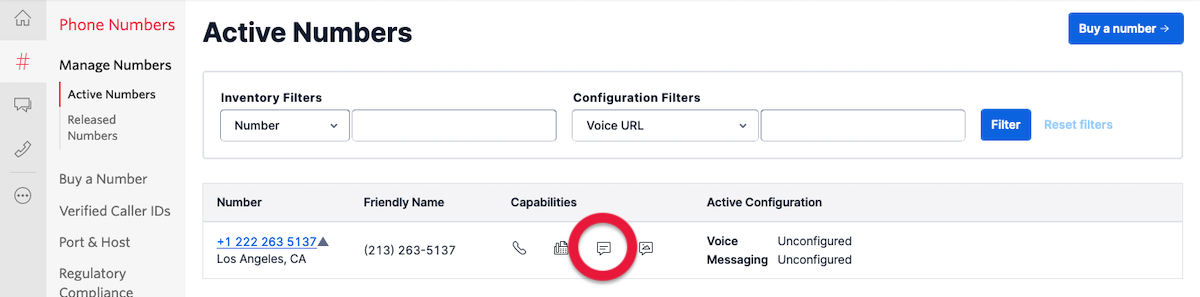
If you don't have a Twilio phone number, purchase one to send the SMS from. To do so, log in to the Twilio Console, select Phone Numbers, and then click on the red plus sign to buy a Twilio number.
NOTE: If you are using a free account you will be using your trial credit for this purchase.
On the “Buy a Number” page, select your country and check “SMS” in the capabilities field. If you’d like to request a number from your region, enter your area code in the “Number” field.
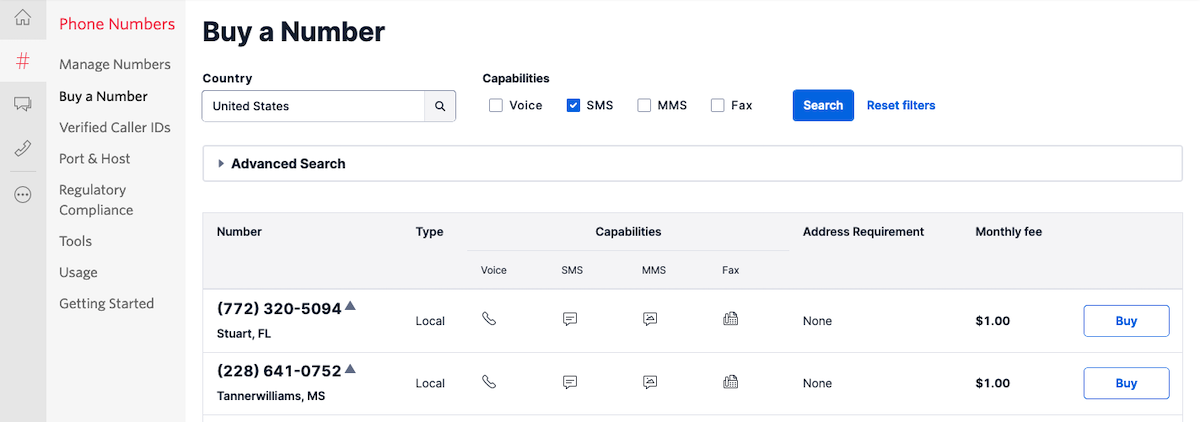
Click the “Search” button to see what numbers are available. Then click “Buy” for the number that you want from the results. After you confirm your purchase, click the “Close” button.
Configure your Twilio credentials and phone number
To be able to send an SMS via Twilio, the Ruby application needs four things:
- Your Twilio “Account SID”.
- Your Twilio “Auth Token”.
- A phone number for the sender.
- A phone number for the recipient.
NOTE: Your Twilio Account SID and Auth Token are required to authenticate with Twilio.
The sender's phone number is your existing (or newly purchased) Twilio phone number. The recipient's phone number will likely be your mobile phone number (or a friend's, perhaps).
The most convenient and secure way to store this information is to set environment variables for each of them.
You can find your Twilio “Account SID” and “Auth Token” on the dashboard of the Twilio Console:
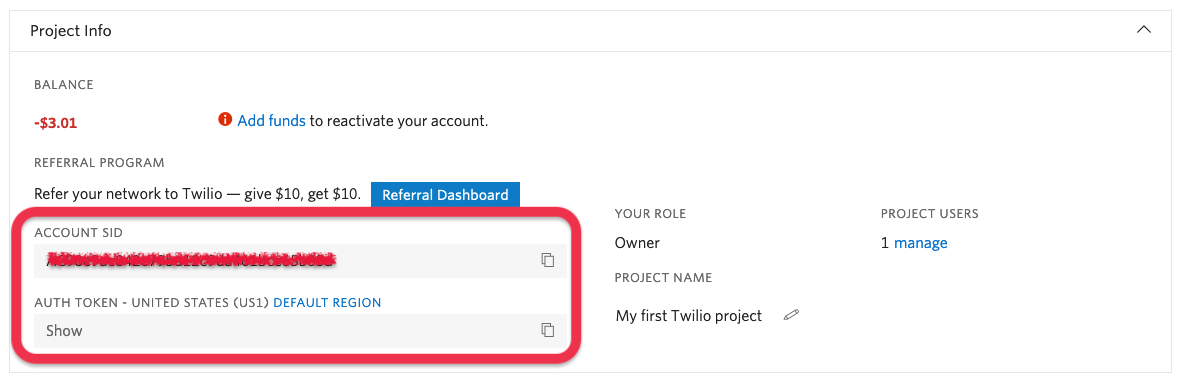
With the Twilio credentials and two phone numbers available, in your terminal, set them as environment variables by running the following commands—after replacing the placeholders with the relevant information.
NOTE: If you are following this tutorial on a Windows computer, use set
instead of export
to define your environment variables in the command prompt. For the phone numbers, use theE.164 format, which includes a plus sign and the country code. If you want to learn more about environment variables, check out our how to set environment variables tutorial.
Send an SMS with Ruby
Create the project directory
To keep the project files nice and tidy, create a new project directory, named twilio-sms-ruby, then cd into the new project directory using the commands below.
Install the required Gem
Before we write the Ruby code, we need to install the twilio-ruby Gem. To do so, create a Gemfile and in it add the configuration below.
Then, run the command below to install the Gem.
Write the Ruby code
With the environment variables and Twilio Gem ready, it's time to write the Ruby code. There's not all that much of it, to be honest, which you can see below. Create a new file, named send-sms.rb, and copy the code below into it.
The code starts off by importing the twilio-ruby Gem. It then initializes a new variable, client
, using the Twilio Account SID and Auth Token retrieved from the respective environment variables. This object handles authenticating and interacting with Twilio's API.
After that, it calls the client.messages.create
method, passing in three things:
- The message to send. Feel free to change this to whatever you like, so long as it is not longer than 1600 characters.
- The recipient's phone number.
- The sender's phone number.
Both phone numbers are retrieved from the respective environment variables, which we exported earlier.
The create
method sends the SMS and stores the JSON response in message
. If the SMS was sent successfully, the response will include a lot of helpful information, including the SMS' sid, which is printed to the terminal. If it was not sent successfully, then the response will include relevant error details.
Test the code
Now that the code's ready, it's time to test it. To do that, run the command below.
Assuming that the SMS was sent successfully, in a short while you (or your friend) will receive the SMS on your mobile phone!
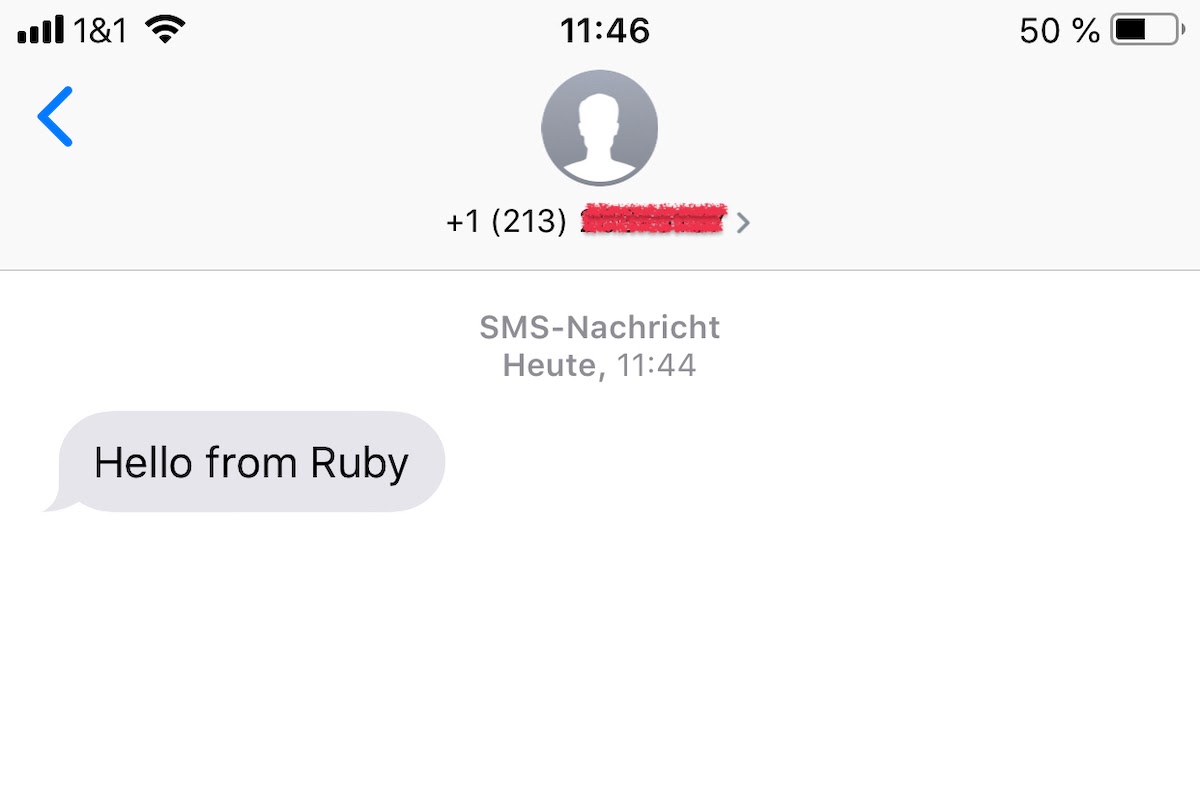
NOTE: that if you are using a free Twilio account, Twilio requires that the number that you use as an SMS recipient is verified in your account. This requirement does not apply if you are using a paid account.
That's how to send an SMS in 30 seconds with Ruby
You've now learned the essentials. I'm sure you can think of so many different applications for sending SMS' in your applications. Be sure to check out the documentation, as well as the Message Resource Reference to learn about the complete functionality available.
I can’t wait to see what you build with Twilio and Ruby!
Matthew Setter is a PHP and Go editor in the Twilio Voices team, and a PHP and Go developer. He’s also the author of Mezzio Essentials and Deploy With Docker Compose. You can find him at msetter@twilio.com. He's also on LinkedIn and GitHub.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.