How to send SMS Without a Phone Number using Alpha Sender and Ruby
Time to read: 5 minutes
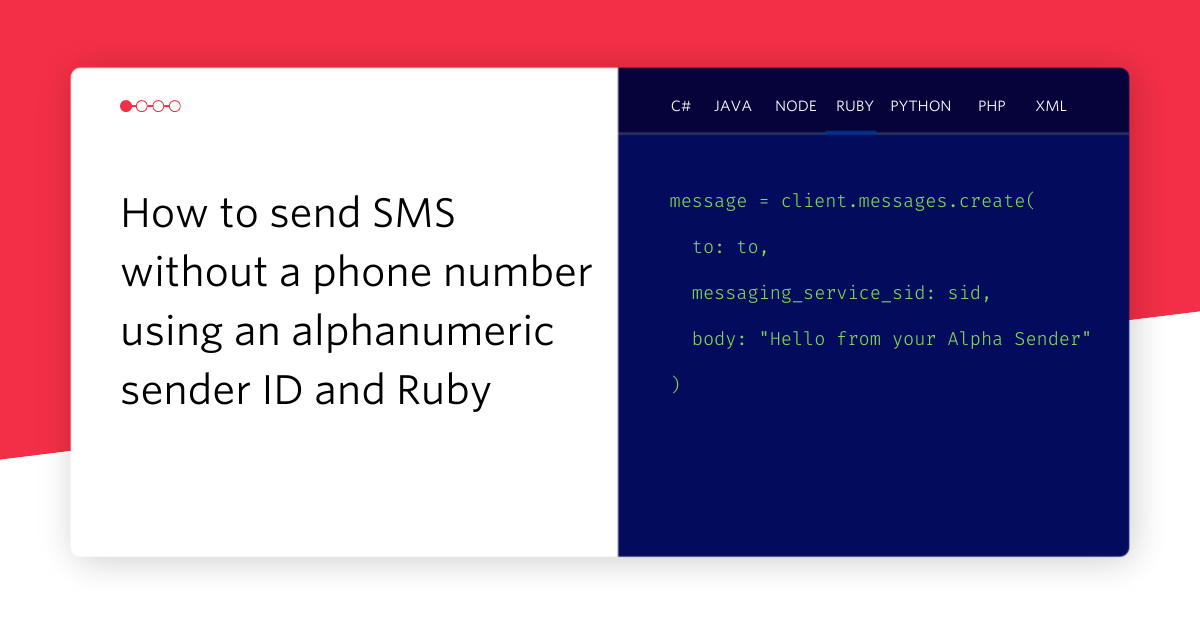
We talk a lot about conversational messaging at Twilio; being able to send to and receive messages from your customers allows you to better engage with them. Sometimes you just need to send a one-way message though, for example when you are sending alerts, notifications or verifications. For a one-way message like this you could consider using an Alphanumeric Sender ID instead of a phone number.
When a user receives a message from an alphanumeric sender ID, it looks like this.
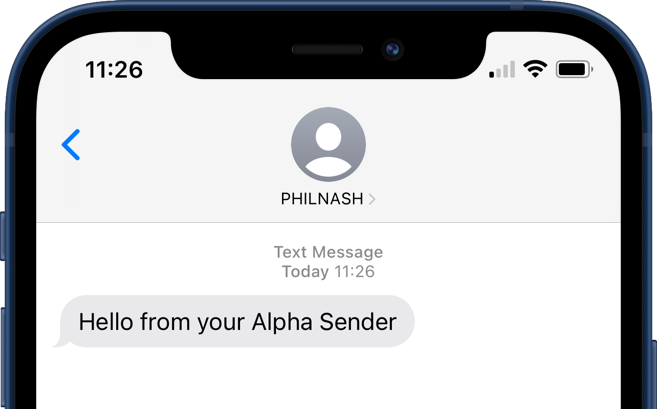
In this case, I sent the message from PHILNASH and you can see that the ID takes the place of the phone number for the contact in the iOS messages app.
Alphanumeric sender IDs behave differently to phone numbers and have some benefits under the right circumstances:
- Your business is instantly identifiable by name, the recipient doesn't have to have your phone number in their contacts to see your name, leading to better open rates
- Alphanumeric sender IDs can be used across many different countries without the need for a dedicated phone number in each country
- There is no charge to use an alphanumeric sender ID in most countries
- It is not possible for recipients to reply to messages sent from an alphanumeric sender ID, so you don't need an application to handle inbound messages
There are some drawbacks too, like:
- It is not possible for recipients to reply to messages sent from an alphanumeric sender ID, so conversational use-cases are not possible
- You need to provide alternative ways for users to opt out of messages because they cannot send "STOP"
- Alphanumeric sender IDs aren't supported in all countries and in some countries require pre-registration which can come with a fee
Overall, alphanumeric sender IDs are really useful for the right use-case. We'll spend the rest of this post setting up our Twilio account to send from an alphanumeric sender ID and showing you how to do so in Ruby.
What you'll need to send SMS messages from an alphanumeric sender ID
- You will need an upgraded Twilio account
- I'll be using Ruby to demonstrate how to send a message from your alphanumeric sender ID, so if you want to code along, make sure you have Ruby installed
How to set up your alphanumeric sender ID
We recommend that you configure sending from an alphanumeric sender ID via a Messaging Service. Messaging Services have a lot of benefits, including falling back to a long-code number if you try to send to a country that doesn't support alphanumeric sender IDs. It only takes a couple of minutes to set up your messaging service, so let's configure one now.
Create and configure a Messaging Service
To create a Messaging Service you need to navigate to the Twilio Console and, under the Messaging menu, select Services. From this page you can now click Create Messaging Service and you will see a 4 step process.
Step 1
The first step is the easiest; give your new Messaging Service a name.
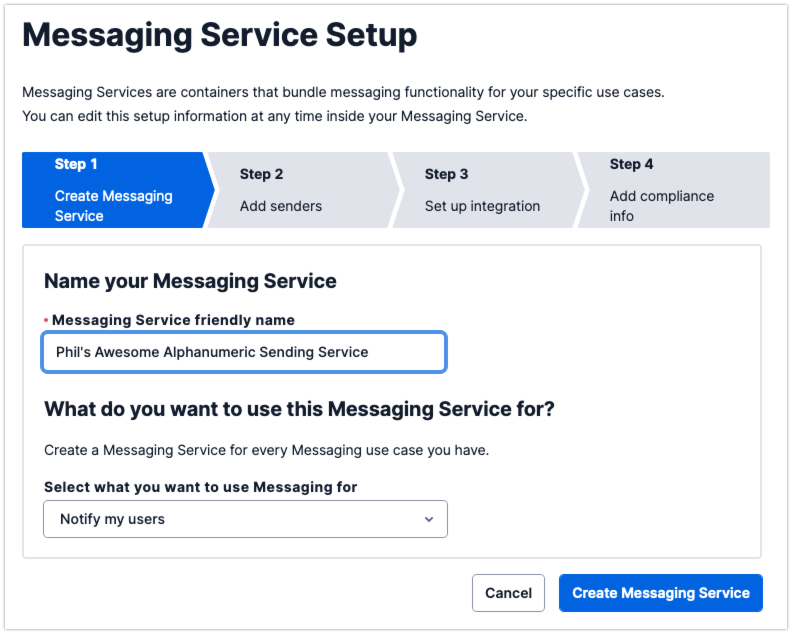
Step 2
Next, add an alphanumeric sender ID to your Messaging Service. Choose the sender type "Alpha Sender".
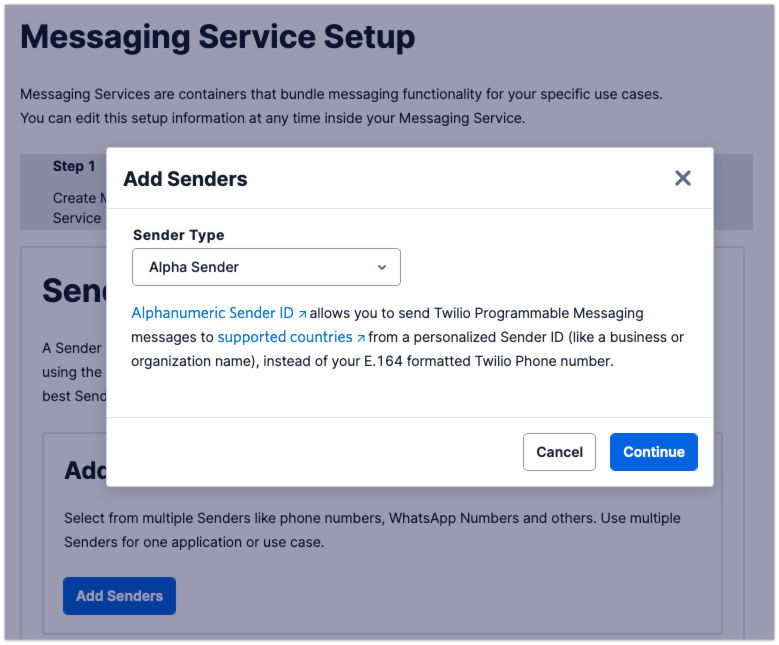
Click Continue and then enter the alphanumeric sender ID of your choosing. There are some rules here; you are limited to 11 characters and at least one of them must be a letter.
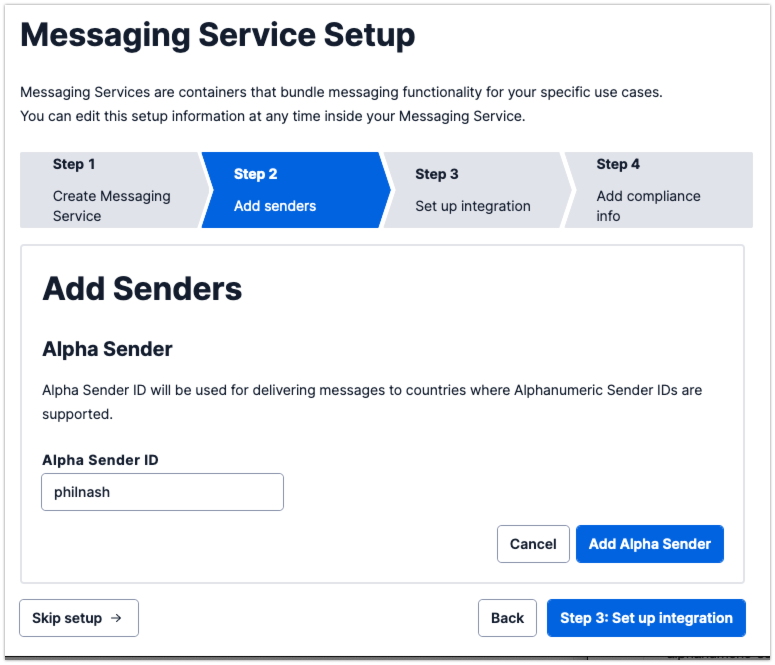
Once you click Add Alpha Sender you will see your sender pool containing your new alphanumeric sender ID. Click on Step 3: Set up integration.
Step 3
This step is to set up how your Messaging Service handles incoming messages. Since we are only dealing with alphanumeric sender IDs that can't receive incoming messages we can move straight on to the next step. If you do add a long code number to your sender pool, you will want to revisit these settings later. Click Step 4: Add compliance info.
Step 4
If you are sending Application-to-Person SMS in the USA you will be subject toA2P10DLC rules. I won't cover that here, but if that applies to you then you should learn more about and register to use A2P 10DLC messaging. Click Complete Messaging Service Setup then View my new Messaging Service.
Now you're ready to write some code and send SMS messages from your Alpha Sender powered Messaging Service.
Send an SMS message from an alphanumeric sender ID in Ruby
Now your Twilio account is set up for you to send messages from your alphanumeric sender ID, it's time to write some code to send that message. If you want to skip to the end, the code is all available on GitHub.
Create a new Ruby project
First, make sure you have Ruby installed on your machine. To manage dependencies, make sure you have Bundler installed as well.
Create a new directory for this project and then change into that directory on the command line:
Inside the directory run:
This command creates a new Gemfile to store our project's dependencies. Add the Twilio gem as a dependency:
That command added the latest version of the library to the Gemfile. We'll need to store some credentials in the project, so add the dotenv gem as well:
With those two dependencies in place, we need a file for our code and a file for our credentials. Create one new file called send-sms.rb and another called .env.
Open the .env file. In here we're going to store the credentials we need to make calls to the API and the Messaging Service SID that we just created. Copy the below and enter your details for each of the credentials. You can find your Messaging Service SID
Now let's write some code! Open up the send-sms.rb file. Start by requiring "dotenv/load"
, which loads the variables we listed in the .env file into the environment, and "twilio-ruby"
, the Twilio Ruby helper library.
Then extract the variables we need from the environment:
Using ENV.fetch
like this will throw an error if the variable is not present in the environment, which is useful for catching bugs early.
To send a message you need an address to send it from, handled by our Messaging Service, a number to send the message to and the message you want to send. For this script, let's pass the number into the script on the command line. To get that argument, we access the first member of the ARGV
list.
Now we need to create an API client from the Twilio library and use it to send the message. Finish off the code with these lines:
That code all together is:
Run the script on the command line, passing in your own phone number (in e.164 format) and you'll receive a message from your alphanumeric sender ID.
Congratulations, you've successfully sent yourself a message from your own alphanumeric sender ID!
Get to know Messaging Services
In this post you've seen how to set up a Messaging Service with an alphanumeric sender ID and send a message using that service in Ruby. You can see all the code in this repository on GitHub.
Sending a message without a phone number is just one of the things a Messaging Service can do for you though. You can also set up your Messaging Service to:
- Fallback to a long code for countries where alphanumeric sender IDs aren't supported
- Select the right number from the number pool for the country you are sending to with geomatching
- Distribute messages amongst the numbers in your pool to scale up to handle sending higher volumes of messages
- But always use the same number for a particular end-user, with Sticky Sender
- And more!
I'd love to hear what you're building with Twilio messaging. Drop me a message on Twitter at @philnash or send me an email at philnash@twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.