Send a WhatsApp Message in 30 Seconds with Ruby
Time to read: 3 minutes
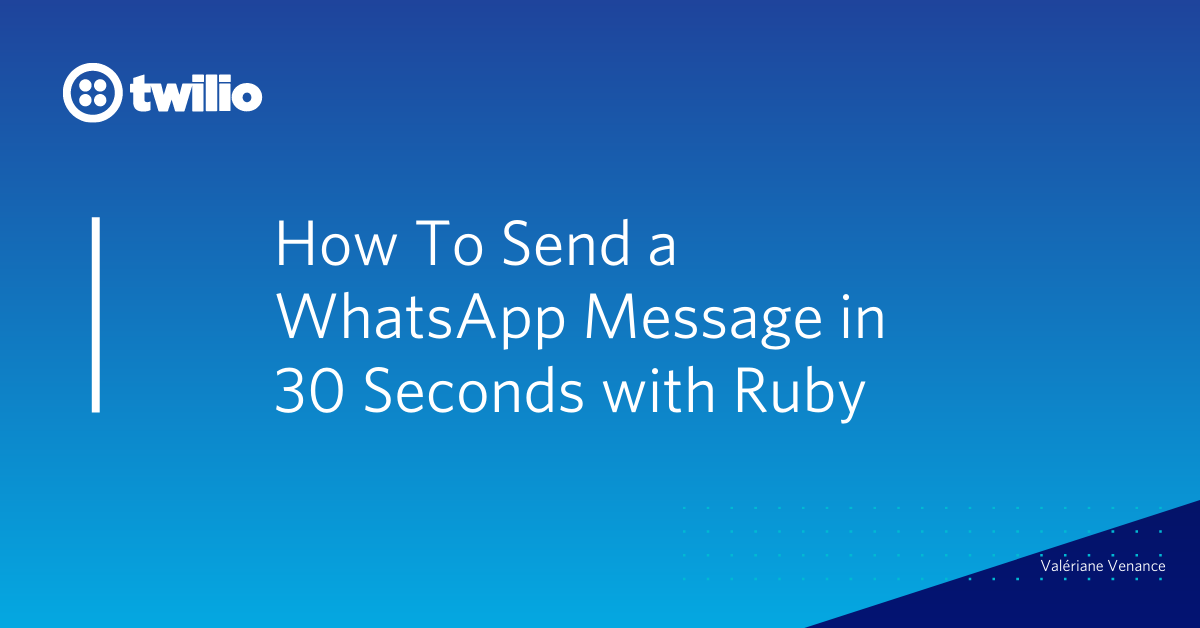
Do I need to introduce WhatsApp? If you are reading this article, you are probably familiar with it, but in case you don't, WhatsApp is a cross-platform centralized messaging and voice-over-IP service that allows people from all over the world to communicate via text and voice calls.
In this tutorial, you will learn how to send your first WhatsApp message using the Ruby programming language and Twilio.
A bit of configuration, 30 seconds of code and you’ll be done!
Requirements
To follow along with me, you will need:
- A Twilio account
- Ruby’s latest version installed on your machine (I use rvm and recommend that you use a manager, but this is not mandatory)
Getting started
Create a new folder called ruby-whatsapp
on your computer, this is where we are going to create our code files.
Install dependencies
We will need the following gems:
bundler
for avoiding permissions error
twilio-ruby
First, let’s install bundler. In a terminal window, (or in a command prompt with administrative rights if you are using Windows) make sure you are in the ruby-whatsapp
directory and run
Let’s create a Gemfile
in our ruby-whatsapp
folder and seed it with:
Install the gem by running this command in the ruby-whatsapp
directory in the CLI:
Join the Whatsapp Sandbox
Go to the WhatsApp page in the Twilio Console and activate the sandbox.
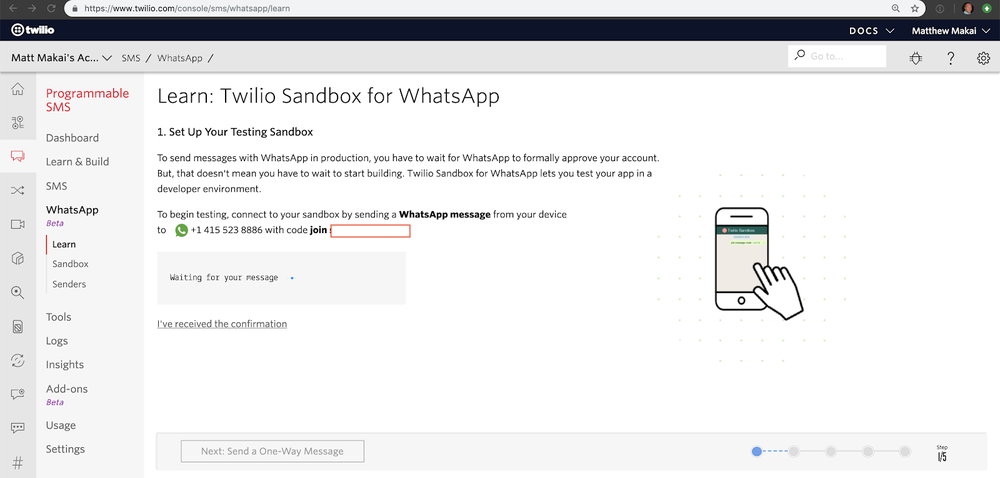
You will be redirected to the page above which shows you how to connect to your sandbox by sending a WhatsApp message through your device. In my case, I’m required to send something similar to join name-of-sandbox
to +14155238886.
Get your Twilio Credentials
From your Twilio Console Dashboard, find your Account SID and Auth Token. Set these as environment variables in your console.To keep things simple, use TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
as environment variable names so we will use the same you and I.
Write the Ruby code to send WhatsApp messages
We are ready to write our Ruby code and send our first WhatsApp message.
In our ruby-whatsapp
folder, let’s create a new file named whatsapp-sender.rb
.
This file should contain the following code:
Save the whatsapp-sender.rb
file and run it with:
Now check your phone and see the incoming WhatsApp message you just received 🎉
Awesome! Let’s have a closer look at the code:
- We create a new
Twilio::REST::Client
with our credentials - We send a message using this client
client.messages.create
- We set our
from
parameter to our WhatsApp sandbox number and specify that it’s a WhatsApp number - We set our
to
parameter to our own WhatsApp number and also specify that it’s a WhatsApp number - We define the message body so the message says
Ahoy WhatsApp user!
And that’s it, really.
Bonus : Send images via WhatsApp with Ruby
Our code will mostly be the same as before, we just need to add a media URL to the message parameters:
Save the whatsapp-sender.rb
file and run it with:
This will send a new WhatsApp message, containing the same text but this time with a doggo picture because everyone needs to see a cute dog from time to time. I found this photo by Dominika Roseclay on Pexels. The cool thing about the WhatsApp media URL parameter is that it also works for other types of files, likes PDFs and audio.
And what if you want to share something that’s not available on the World Wide Web? Twilio’s got you covered! Just upload your files to your Twilio account as assets and and send them to WhatsApp users by using their URL as media-url
in the Ruby code.
Wrapping up
Woo, you just learned how to send WhatsApp messages from your Ruby code! And how to add cute puppies to your messages, too.
It’s a very good start. You can continue your journey by learning how to receive and reply to WhatsApp messages in Ruby or you could go deeper and build a location-aware WhatsApp weather bot with Ruby, Sinatra and Twilio. The choice is yours!
I can’t wait to see what you build!
Valériane Venance is a Developer Evangelist at Twilio. Leave her a message at vvenance@twilio.com or on Twitter if you’ve built something cool with Ruby. She’d love to hear about it!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.