How to Send WhatsApp Messages from Java Applications with Twilio
Time to read: 2 minutes
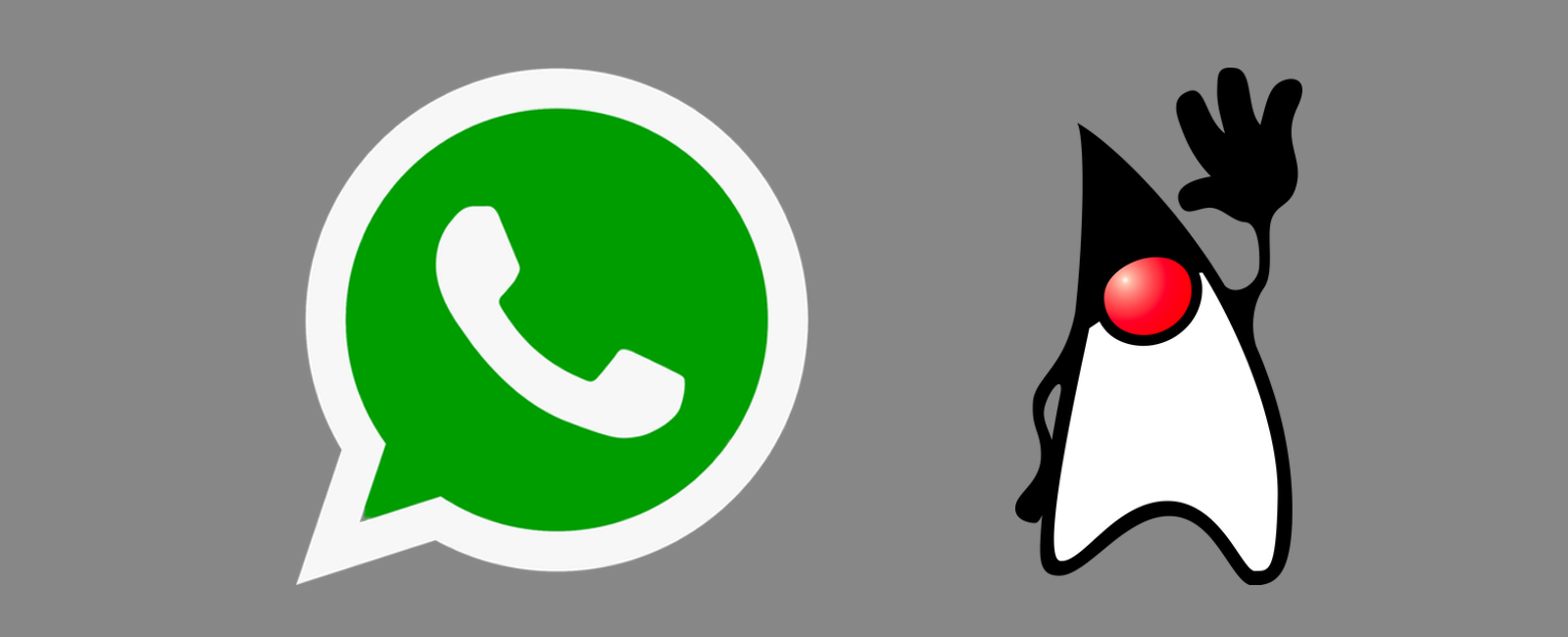
WhatsApp is a global messaging service that helps billions of people communicate with each other. Applications can now also programmatically interact with people on the service using the Twilio Messaging API and Twilio's Java Helper Library. Let's learn how to quickly send messages to people from a new or existing Java application.
Installing Dependencies
Our local development environment needs the following dependencies to properly send WhatsApp messages from Java.
- Java SE version 7 or higher
- A free Twilio account with an activated WhatsApp Sandbox
- The Twilio Java Helper Library
First, install Java on your development machine if you do not already have it. You can also read this detailed tutorial on setting up your Java development environment if you are having trouble.
Next, log into your existing Twilio account or sign up for a new free Twilio account.
Take note of your Account SID and Auth Token when you log into the Twilio Console, as shown in the following screenshot.
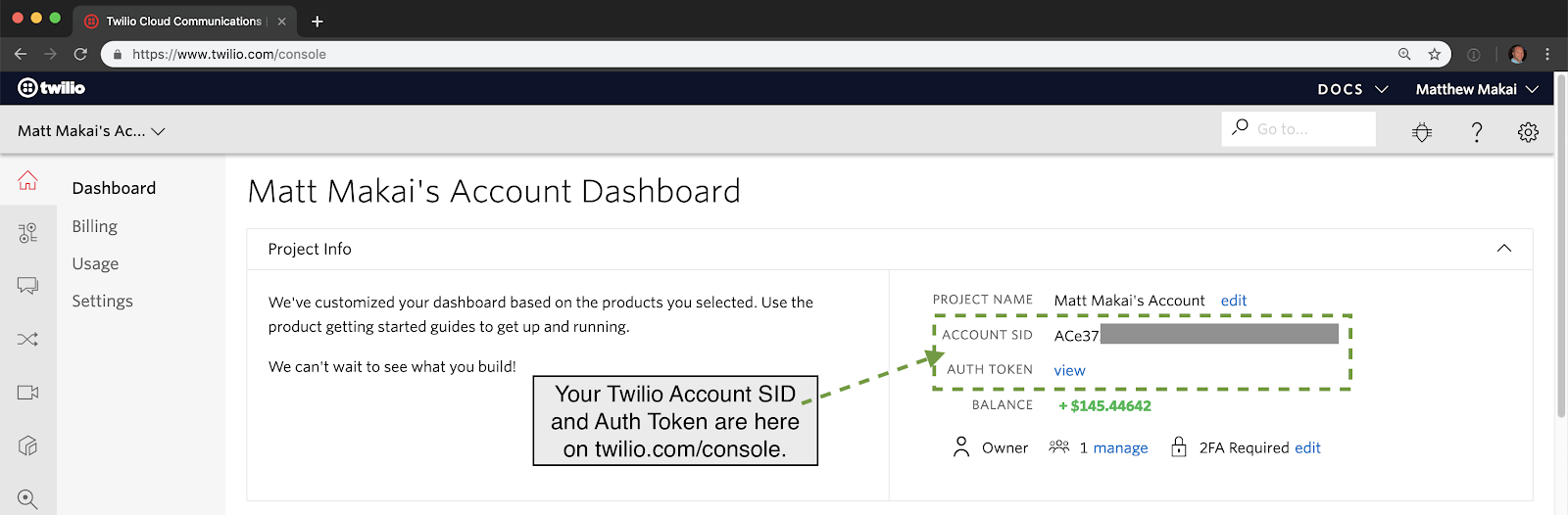
The Account SID uniquely identifies your Twilio account. The Auth Token is a secret key that should never be shared because anyone with both the Account SID and Auth Token will have full access to your Twilio account.
We will use the Account SID and Auth Token in our Java application in a moment. For now, start your Java application by creating a new file named SendWhatsAppMessage.java
in the editor of your choice.
Write or paste in the following code:
The above code imports the Twilio Java helper library, declares two constants for the Account SID and Auth Token, instantiates the Java helper library and then sends a WhatsApp message via the create
method.
Our Java is ready to go, so save the file. Compile the source file using your IDE or the command line as shown in the following command. Remember to ensure the Twilio Java helper library is on your CLASSPATH
.
In this case I am using version 7.36.1 of the Twilio Java helper library that includes all of the dependencies. Now that our application is compiled we just need to activate the Twilio WhatsApp sandbox to test it out.
Sending Our First WhatsApp Message
Go to the WhatsApp page in the Twilio Console and activate the sandbox.
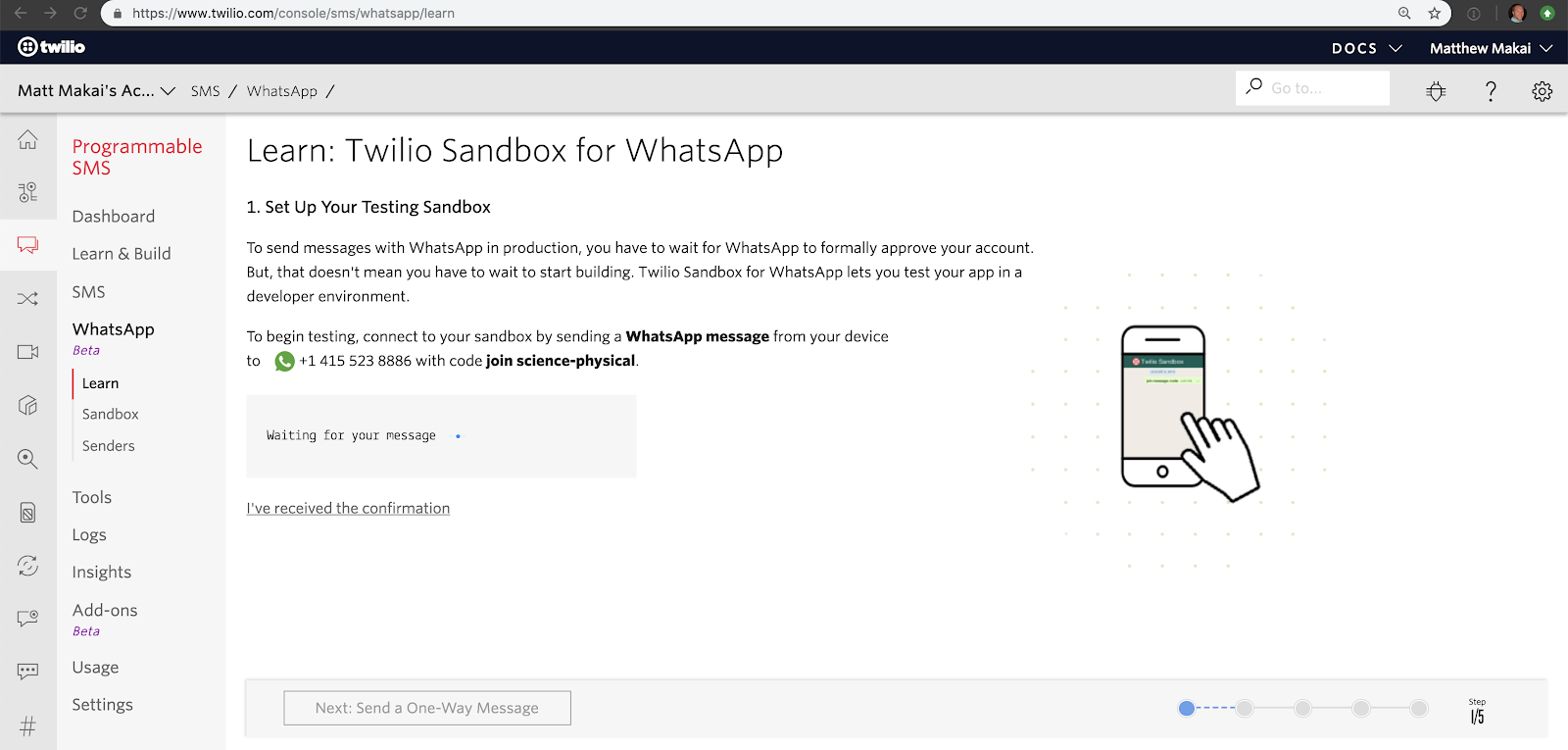
You will be redirected to the page above which instructs you how to connect to your sandbox by sending a WhatsApp message through your device. In my case, I’m required to send join science-physical
to +14155238886.
Run the compiled SendWhatsAppMessage
class through your IDE or if you are using the terminal here is the command I used to execute my Java application:
Check out your WhatsApp Messaging app and you should see your first message sent through the Twilio API from your Java application.
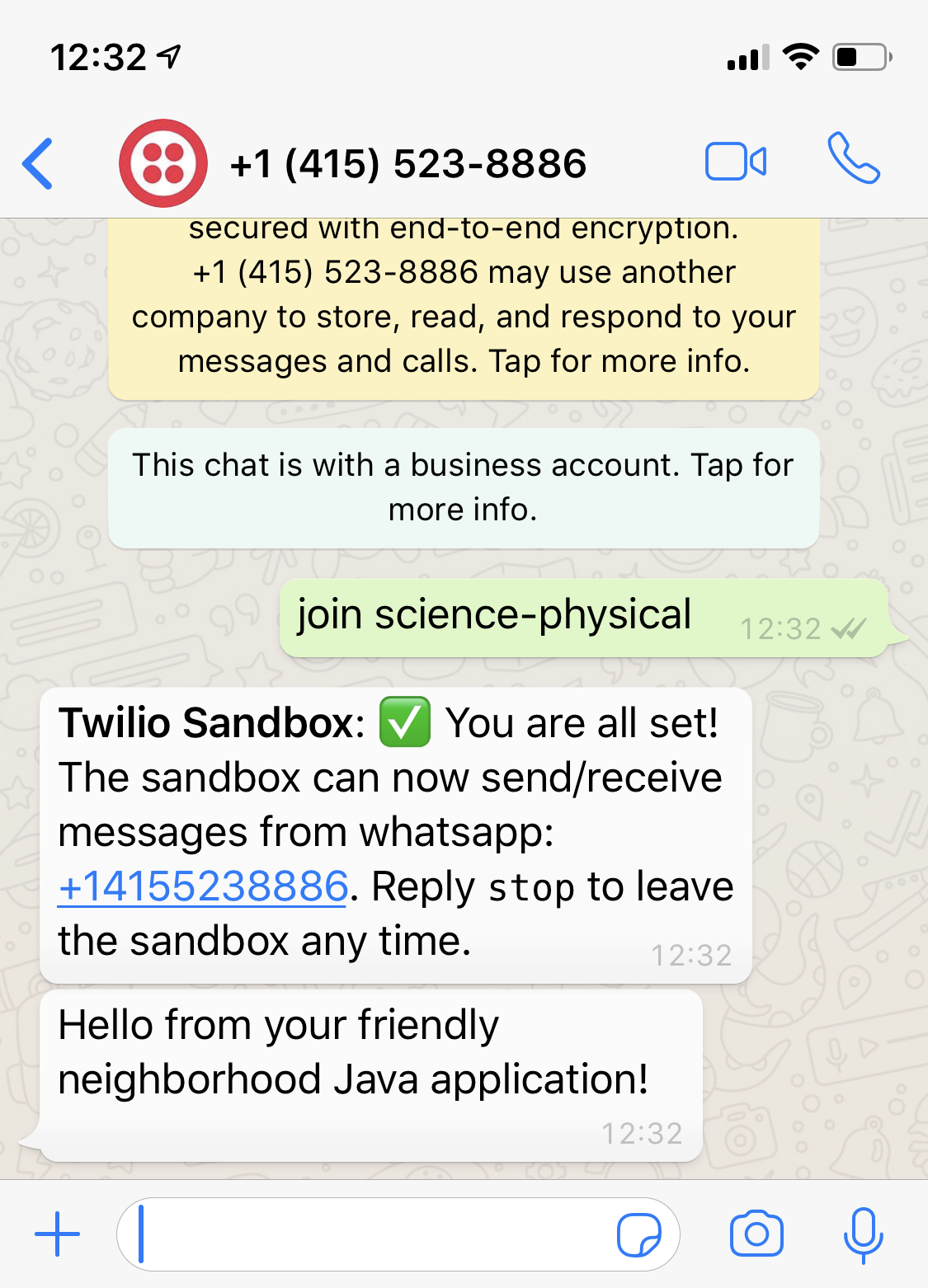
What's Next?
We just learned how to send WhatsApp messages using the Twilio API for WhatsApp Messaging. Next you can try the following tutorials to do even more with the Twilio API and many other forms of communication:
- Learn how to programmatically receive WhatsApp messages
- Use the WhatsApp Messaging Templates
- Read the Twilio WhatsApp Quickstart Docs to learn more
Questions about this tutorial? Ping me on Twitter @mattmakai.
Twilio.org helps social impact builders use digital technology and financial resources to scale their reach and impact. Get started today at no cost. Sign up here for your Impact Access Program product credits. Eligibility criteria applies.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.