Sending Bulk Emails With Twilio SendGrid and Python
Time to read: 3 minutes
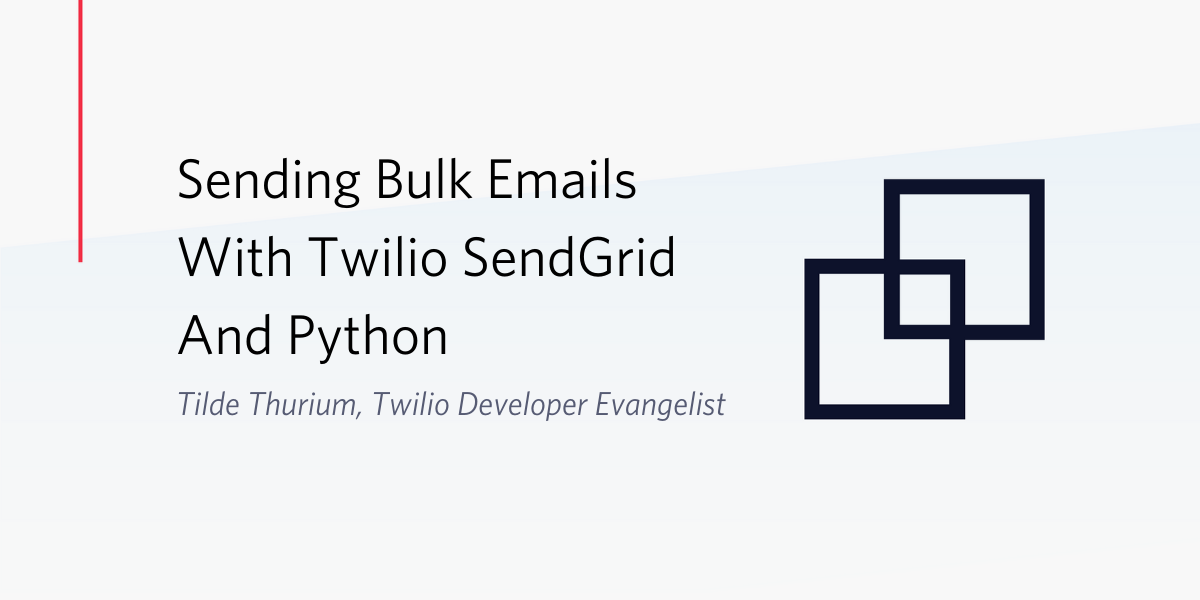
Sending a single email is great, but one of the big advantages of email is the ability to quickly reach a wide audience. Today I’ll show you how to send mass emails with Python and SendGrid. Just for fun, let’s say you’re a Python developer who works at a donut shop. You need a way of letting the customers who have signed up for your email list know when fresh donuts have come straight out of the oven. 🍩
Prerequisites
- Python 2 or 3 installed. Since it’s almost 2020 I’d strongly recommend Python 3.
- A free SendGrid account - sign up here
- At least two email addresses, to test things out and make sure they’re working. You can sign up for (multiple) free Gmail addresses here. Or you can try the old hack of adding a + to your existing Gmail address. Caveat: we ran into some deliverability issues there.
Setting Up Your Python Development Environment
First, create a new Python project. If you need to brush up on general Python environment setup, follow this useful guide.
Create your API key from the SendGrid dashboard. Let’s call it “bulk email.”
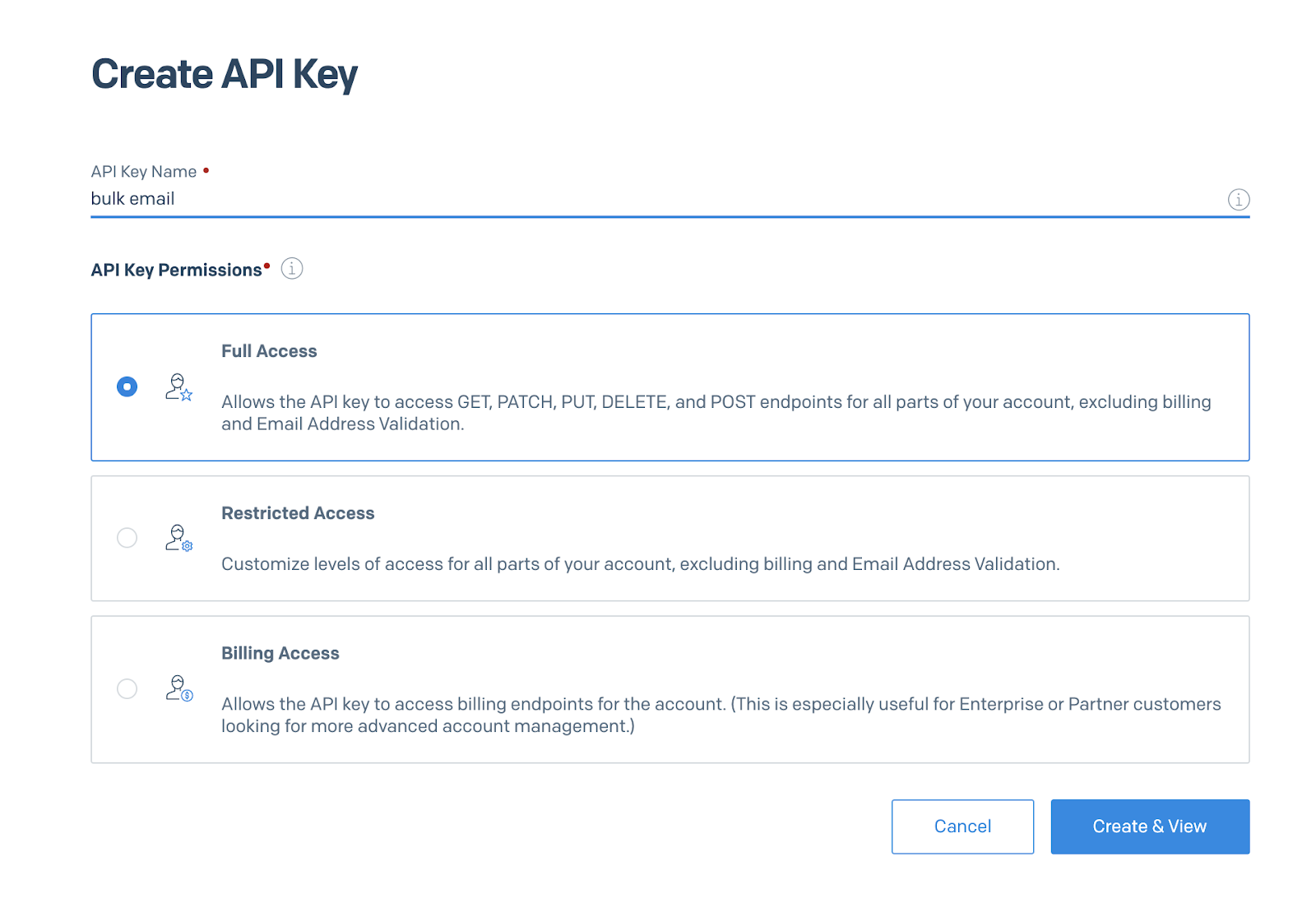
After clicking “Create & View”, you’ll see your key. Before you close this dialog box, save the key in an environment variable SENDGRID_API_KEY
since you won’t be able to get that same key from the SendGrid dashboard again for security reasons.
Install the Sendgrid Python helper library with pip install sendgrid
. Create a file, send_mail.py
, and open it in your editor of choice.
Sending a single email to multiple recipients
Copy the following code into send_mail.py
:
On your command line you’ll see some output resembling the following:
Also, you should receive some emails in your inboxes. If you don’t see it check your spam or promotions folder.
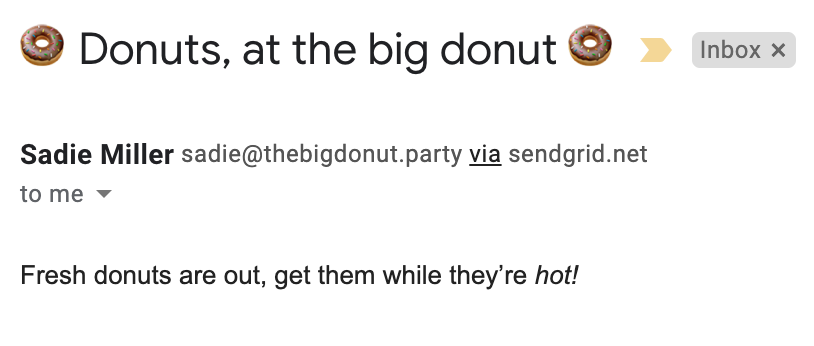
The current API rate limit is 1000 emails per request. Does your high-throughput, scalable, fault-tolerant donut shop have more customers than that? If yes you’ll need to split the email addresses into lists of less than 1000 and make multiple calls to the send
method.
Recipients of this email won’t be able to see one another’s email addresses. If you want to send multiple email addresses but keep the recipients addresses visible to one another, use the same code as above but remove the is_multiple
parameter from line 18. That said, be careful and don’t expose your customers’ email addresses unless you have a good use case for doing so.
Personalizing mass emails
If you’re a good donut shop owner, you’ve been paying attention to your customers. You noticed that Steven really loves bacon donuts.
With personalizations, you can change the emails you send to your customers to make them more, well, personal. Like mentioning their favorite kind of donut in the subject line to entice them to come in.
Replace the code you’ve got in send_mail.py
with the following:
Run python send_mail.py
to test it out. If a personalized subject line isn’t supplied for a particular recipient, we fall back to the default. You should have received one email with a bacon subject line and one with a generic subject line.
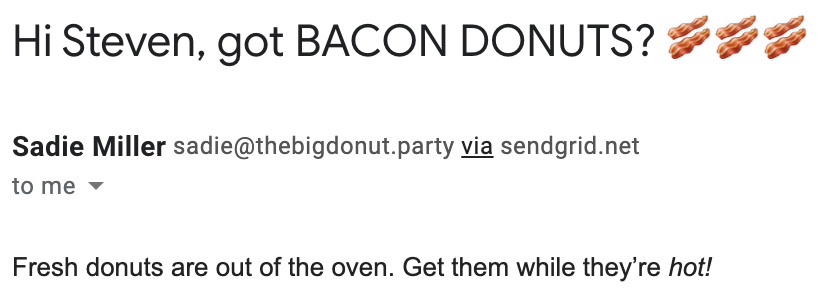
The personalizations API can do more than change the subject line. Here’s the list of attributes that can currently be personalized:
subject
- the email subject line.headers
- any headers you want to send with the email.substitutions
- key/value pairs representing strings that you would like to substitute into an email subject line or body.custom_args
- any custom arguments you would like to include in your email that will override substitutions.send_at
- specifies a particular time you’d like the email to be sent, in Unix timestamp format.
Wrapping up our Python SendGrid bulk email adventure
Let’s review what we’ve learned today:
- How to send a single SendGrid email to multiple recipients, without ccing
- How to send a single SendGrid email and cc multiple recipients
- How to use personalizations to customize emails to multiple recipients
Give yourself a reward, you’ve earned it. 🎉 If you’re craving donuts now, sorry not sorry.
The SendGrid API is so full-featured and flexible that it’s impossible to fit everything it can do into one blog post. For the most up to date information, check out the docs. You can also look at the open source code for the Python helper library which is available on GitHub. Thanks for reading, and happy emailing.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.