Sending One-time Passwords in WhatsApp using PHP, Laravel, and the Twilio API for WhatsApp
Time to read: 5 minutes
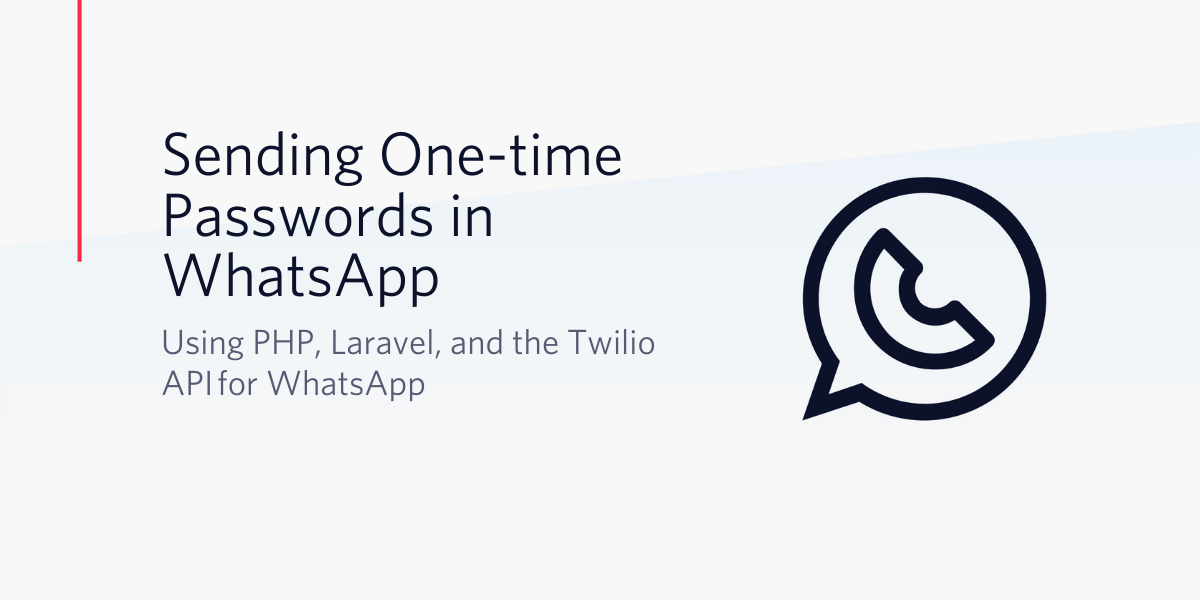
WhatsApp is often contested as the world’s most popular messaging app, allowing its users to communicate securely and in real-time. As a business owner, you can build upon the speed and security provided by WhatsApp to engage with your customers, send alerts and notifications, provide customer support, or even send One-Time Passwords (OTPs) to your customers.
In this tutorial, you will learn how to send WhatsApp notifications to your users by sending out one-time passwords (OTP) via WhatsApp using the Twilio API for WhatsApp during registration.
Prerequisites
To follow through with this tutorial, you will need the following:
- Basic knowledge of Laravel
- Laravel Installed on your local machine
- Composer globally installed
- Twilio Account
- WhatsApp Enabled Twilio Number
Project Setup
This tutorial will make use of Laravel, so the first step is to generate a new Laravel application. Using the Laravel Installer, generate a new Laravel project by running the following in your terminal:
Next, you will need to set up a database for the application. For this tutorial, we will make use of a MySQL database. If you don't have MySQL installed on your local machine, head over to the official site to see how to. After successful installation, fire up your terminal and run this command to login to MySQL:
NOTE: Add the -p flag if you have a password for your MySQL instance.
Once you are logged in, run the following command to create a new database:
Next, update your .env
file with your database credentials. Open up .env
and make the following adjustments:
Setting up the Twilio API for WhatsApp
To make use of the Twilio API for Whatsapp, you will need to get a WhatsApp approved Twilio number which will be used for sending and receiving WhatsApp messages. Although, this is only a prerequisite if you are going to make use of the API in production. For testing and development purposes, Twilio provides a sandbox area that can be used for sending and receiving WhatsApp messages in a developer environment.
Joining a WhatsApp Sandbox
Before you can start testing with the Twilio Sandbox, you have to first join-in. To do this, head over to the WhatsApp section in your Twilio dashboard and send the provided text (which is in the format join-{unique text}
) to the WhatsApp number provided. It’s usually +1 (415) 523-8886
.
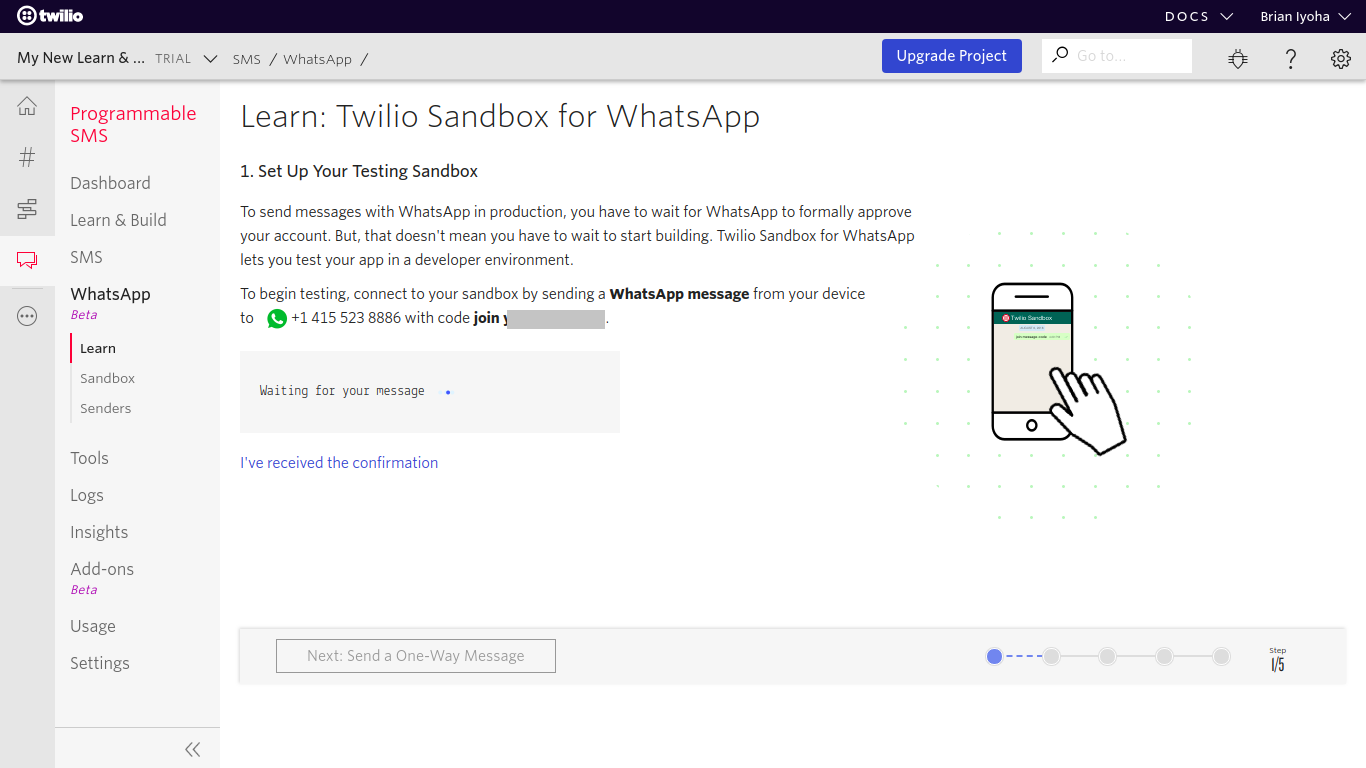
If your message goes through successfully, you should get a response similar to this:
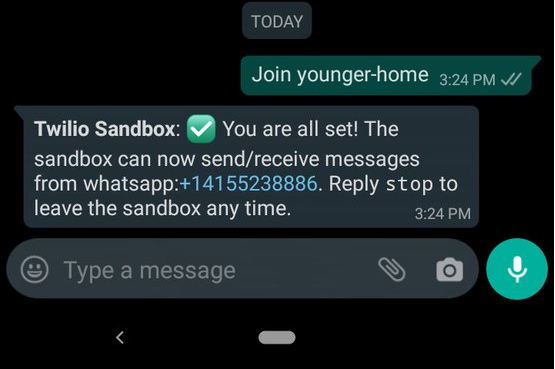
Now that you have connected to your WhatsApp sandbox, your Twilio credentials will need to be added to your .env
file as follows:
NOTE: Your Twilio WhatsApp number can be found in your sandbox under Sandbox Participants and your Account SID and Auth Token are available in your dashboard.
Sending out OTPs via WhatsApp
To expedite this tutorial the Laravel authentication scaffold will be used to authenticate users into the application. Fire up a terminal in the project directory and run the following command to install the laravel/ui
package and also scaffold a basic authentication system:
After successful execution of the commands above, you should have the login, registration, and home views, as well as routes for authentication.
NOTE: To make use of the npm
command, you must have Node.js globally installed in your machine.
Next, you need to create a column in your users
table for storing the generated OTP. Open up the user migration file (database/migrations/2014_10_12_000000_create_users_table.php
) and make the following changes to the up()
method:
This adds the otp
and phone_number
columns in the users table which will be used for storing user's OTPs and phone numbers respectively.
Next, open up the User model (app/User.php
) file to add the otp
and phone_number
fields to the fillable array:
Now to make this schema reflect in your database, run the following command to execute the available migrations:
Modify registration logic
The basic Laravel authentication scaffold needs to be modified to support the generation of an OTP at the time of registration and delivering it via the Twilio API for WhatsApp. Before proceeding to make adjustments to the registration logic, you first need to install the Twilio SDK which will be used for sending out WhatsApp Messages. Open up a terminal in the project directory and run the following command to get it installed via Composer:
Next, open the registration controller (app/Http/Controllers/Auth/RegisterController.php
) and make the following changes:
The validator()
method has been modified to include the phone_number
field while the create()
method has also been modified to generate a 4-digit numeric OTP using the built-in rand() method of PHP:
Next, the user is stored in the database using the Eloquent create()
method which returns the saved User model. Afterwhich, the generated OTP is sent to the user by calling the sendWhatsappNotification()
method and passing in the user's otp
and phone_number
as arguments.
The Twilio SDK is initialized in the sendWhatsappNotification()
method using your Twilio credentials (available in your Twilio dashboard), by instantiating a new class of the Client
class:
Next, the message template for sending out OTP is prepared:
NOTE: To send out a one-way message using the Twilio API for WhatsApp, you must make use of an approved template. Click here to learn more about WhatsApp Templates. The Whatsapp Sandbox comes with predefined templates, one of which is: Your {{1}} code is {{2}}
This is the template currently in use where {{1}} and {{2}} are placeholders that are to be replaced dynamically; in this case they are replaced with registration
and $otp
respectively.
Next, using the instance of the Twilio Client, the templated message is sent to the recipient:
The messages→create()
method takes in two arguments of a receiver of the message and an array with the properties of from
and body
where from
is your WhatsApp Approved Twilio phone number and body
is the message that’s to be sent to the recipient.
NOTE: To tell the Twilio SDK to send this message via WhatsApp, you have to prepend whatsapp: to the recipient phone number and also to your Twilio Whatsapp number. If this is not prepended the message will be sent as a regular SMS to the recipient.
Updating The View
At this point, you should have successfully modified the scaffolded registration logic to also send out OTP via WhatsApp to your users. Next, you have to update the view to include the phone_number
field to the registration form. Open up resources/views/auth/register.blade.php
and replace the code with the following changes:
Testing
To test your application, open a terminal and run the following command:
After successful execution, you should see your localhost URL and port which your Laravel application is accessible from, usually 127.0.0.1:8000. Next, navigate to the registration page and proceed to fill out the form. Afterwhich, click on the Send OTP button and you should receive a WhatsApp message shortly with your OTP.
Conclusion
Now that you have finished this tutorial, you have successfully learned how to send out WhatsApp notifications from your Laravel application while also learning how to make modifications to the scaffolded Laravel auth registration logic. If you would like to take a look at the complete source code for this tutorial, you can find it on Github.
You can take this further by modifying the authentication process to verify the OTP sent to your user before granting them access to your application. Also, you can read more about securing your Laravel application using Twilio Authy here.
I’d love to answer any question(s) you might have concerning this tutorial. You can reach me via:
- Email: brian.iyoha@gmail.com
- Twitter: thecodearcher
- GitHub: thecodearcher
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.