Sending text messages from your iOS app in Swift using MFMessageComposeViewController
Time to read: 2 minutes
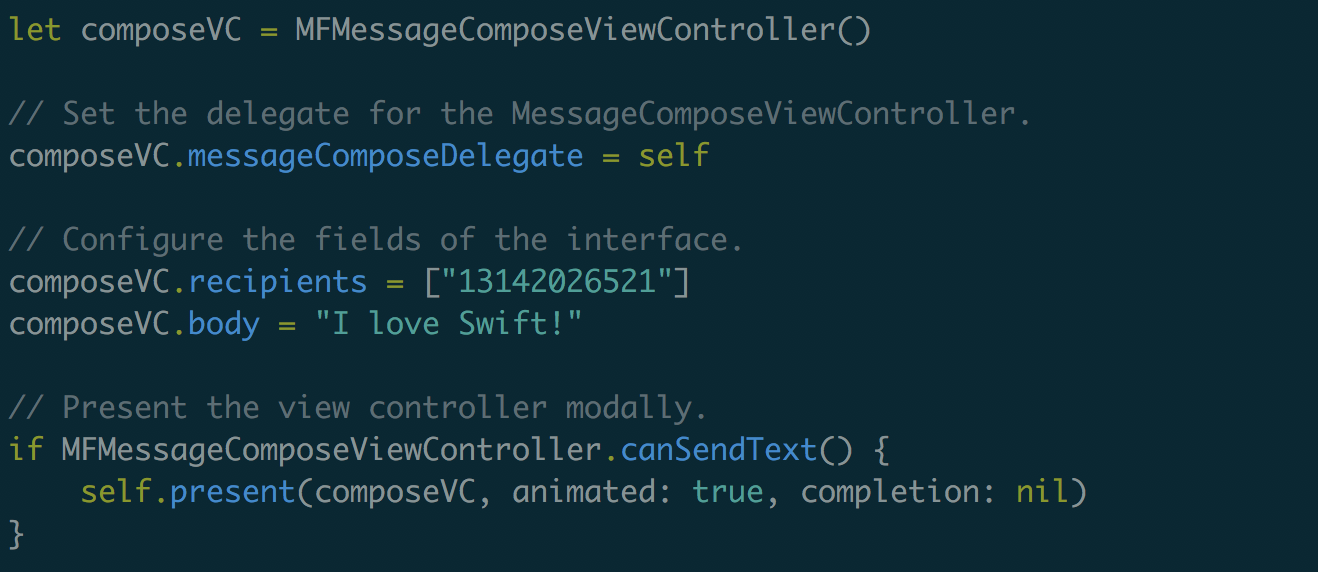
Do you ever want to send text messages in your iOS app from your user’s phone number? While you can’t do this directly without your user’s consent, you can display a pre-composed message for your user to send using MFMessageComposeViewController.
If you want to programmatically send an SMS from a different phone number, you can do this with Swift using Twilio.
That’s cool. Let’s send a message!
If you already have an app and want to get straight to the point, here’s the code you need to display the message composition interface:
This code will send a text message to a Twilio number that is set up to give a programmatic response. To get it working, the class containing the method needs to conform to MFMessageComposeViewControllerDelegate
.
How can I do this from scratch?
First make sure you have Xcode 9 and Swift 4 . Open Xcode and create a new project, selecting the “Single View App” option.
Navigate to ViewController.swift
and replace the code in it with the following:
This adds the MFMessageComposeViewControllerDelegate
protocol to your ViewController
class, and adds a stub method to ensure it conforms to that protocol.
Now create a new function called displayMessageInterface
in your ViewController
class:
This is the code that will display the message display interface with a pre-populated body and recipient number.
Let’s wire this function up to a button so we have a place to execute this code.
Buttons are fun. Let’s do that!
Navigate to Main.storyboard
and drag a button onto your ViewController. Change the text to say “Send Text Message” as seen in this GIF:
https://twilioinc.wpengine.com/wp-content/uploads/2018/07/messagebutton.mp4
Let’s make it so that when the button is pressed, the displayMessageInterface
is called.
Open the Assistant Editor (⌥ ⌘ enter) to have both Main.storyboard
and ViewController.swift
open.
Next ctrl click the button and drag it over to your code to create an @IBAction
named sendMessageButtonAction
to call your function as seen in the following GIF:
https://twilioinc.wpengine.com/wp-content/uploads/2018/07/sendmessageaction.mp4
Finally, add a call to the displayMessageInterface
function in the new code that is added to your ViewController:
You should now be able to send text messages from your app!
Note that you may need to run this on an actual phone as text messaging is typically not available in the simulator.
What next?
You can now add this code to the other apps you’re building. You could also check out Twilio SMS if you want to programmatically send text messages in Swift from a third party number.
If you have any other cool ideas, feel free to reach out and let me know or ask any questions:
- Email: Sagnew@twilio.com
- Twitter: @Sagnewshreds
- GitHub: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.