Sending text messages in Swift with Twilio
Time to read: 3 minutes
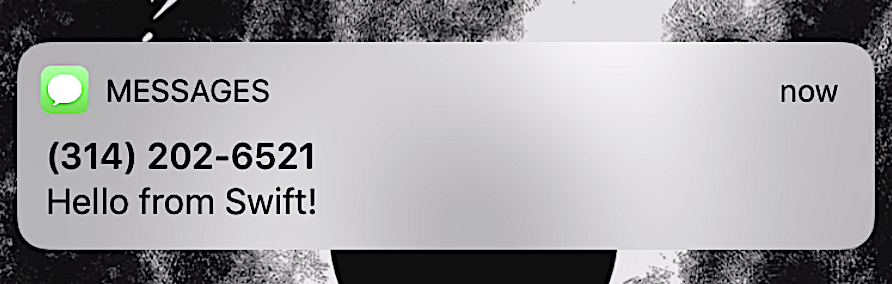
Programmatically sending text messages is awesome, but doing so from an iOS app can be dangerous because it requires you to store your Twilio credentials in the app. With Swift’s ability to run on the server, you can avoid the risks of using Twilio client-side!
Let’s learn how to send an SMS with Twilio from our server using Swift.
If you just want to skip right to the point, here is all the code you need:
Read ahead to learn how to get this code working. Before moving on you’ll need to create a Twilio account if you haven’t already, and make sure you have Swift 4 installed.
What is this Alamofire thing?
In order to send a text message with Twilio, we will need to make an HTTP request to Twilio’s REST API. We will be using a library called Alamofire to do this. Check out this other tutorial for more general info on sending HTTP requests in Swift.
Since the premise of this is to run code from the server side, we are going to use Swift Package Manager to handle dependencies. Start by initiating a Swift package in the directory you want to run the code (I named my directory “SwiftSMS”):
This will generate a Package.swift
file for you, where we can add our dependencies. Open Package.swift
and make sure it has the following code:
Keep in mind that in the targets
section, you may need to modify the name
to fit your package.
Now that we are good to go with dependencies, it’s time to add code to our executable.
Sending an SMS in Swift
Before being able to send messages, you’ll need a Twilio phone number. You can buy a phone number here (it’s free if you’re using the number to test your code during development).
To send a text message, you’ll need to send your Twilio account SID and auth token as part of the request. You can find those in your Twilio Console. Store them in environment variables with the following command:
Now open the file main.swift
in your Sources/SwiftSMS/
folder (replace “SwiftSMS” with whatever you named your project) and add the following code:
Remember to enter your Twilio phone number and personal phone number as the From
and To
values. Run it with this command, and you should receive a text message!
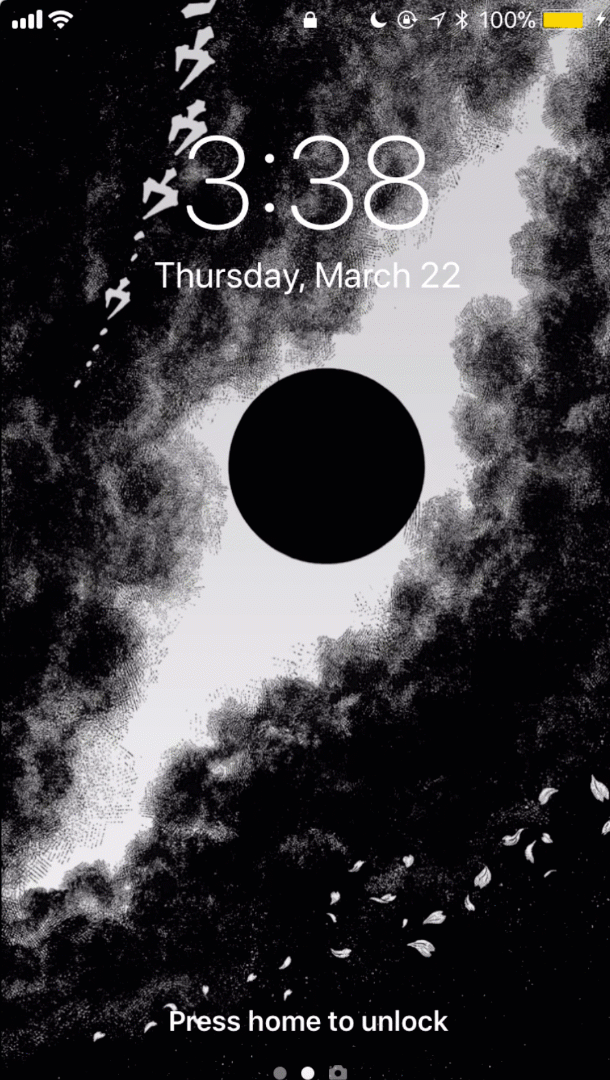
Now what?
You can send text messages using server side Swift now. Congrats! You might want to add this to an actual web application using a back end web framework for Swift such as Vapor. You also might want to try implementing this without using Alamofire if you don’t want to rely on dependencies. Check this post out to learn how to send HTTP requests in Swift 3 or 4.
If you want to do more with Swift and Twilio, you can check out these other posts:
- Making phone calls with Swift
- Handling phone calls with server side Swift
- Receiving and Responding to text messages with server side Swift
If you have any other cool ideas, feel free to reach out and let me know or ask any questions:
- Email: Sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.