Building a Twilio + Google Chrome Extension
Time to read:
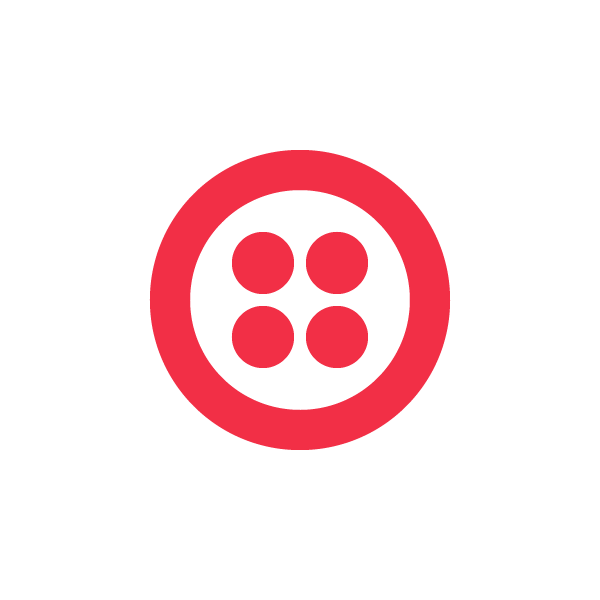
Editor’s Note: This app is no longer live but the code is still available on GitHub here
We are currently running a contest with our friends on the Google Chrome Extension team at Google challenging developers to build extensions that include Twilio. Developers have until 11:59pm this Sunday, May 9th, to submit their extensions for a chance to win netbooks and other awesome prizes from Google and Twilio. Check out the contest page for more info.
—–
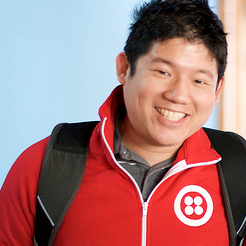
Getting Familiarized with Google Chrome Extensions
After building this extension, I cannot believe how easy it and how slick it was to put this together. Extensions are packed with Google libraries that allows cross-origin requests, manipulation of brower content, and control of browser events. Using extensions you can add more functionality to your browser to help you minimize your mundane tasks.
Google Chrome also comes with some very helpful WebKit debugger tools that I have never used before. I am glad that Google designs their products around developers and continues to stay up to date. The only complaint I have is the missing color syntax of the code in the Google samples.
Building Twilio Chrome
Twilio Chrome will be a simple extension that makes calls and sends SMS from a browser. Check back after the contest for new features added to this extension. This project is open source for your viewing pleasure and I am expecting some great submissions from you all. It is so easy to snag a free Netbook that I wish I am allowed to enter the contest.
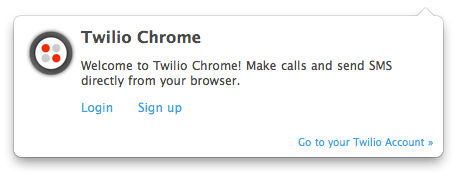
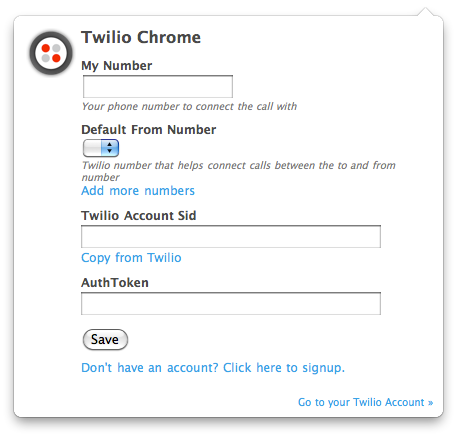
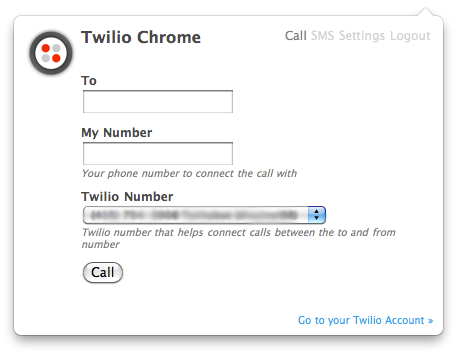
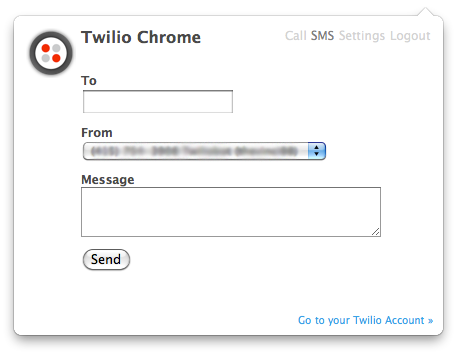
Give it a try! Add the Twilio Chrome Extension or download the Twilio Chrome source code
Anatomy of an Extension
Building a Google Chrome extension is pretty straightforward. I highly recommend getting started with the tutorial. An extension is made up of a manifest, a popup, and a background.
- Manifest: contains all the configuration or permissions, files, and icon
- Popup:covers the view when the extension icon in the browser toolbar is clicked
- Background: continuously runs behind the scene when your browser turns on
Communication Between Extension and Browser Page
The most difficult hurdle was communicating between the background or popup of the extension with the content in the browser. I am sure because of security concerns, you cannot access the DOM of the content directly from your extension. But have no fear, it is possible. Google explains it here.
So, in a nutshell, we have to inject scripts into content to send messages or listen for messages from the extension. On the extension we would have to do the same as well. I had to use this method to grab information from the Twilio account page and store it in your browser.
Debugging
Make sure you take full use of the debugger tools available in Chrome. Read more from Google here. To activate the debugger for your extension, make sure your browser developer mode is turned on at chrome://extensions/ and right click on the extension icon for “Inspect popup”. This will turn on the developer console for only your extension.
If you want to see the console for the background portion, go back to chrome://extensions/ and hit “Inspect background”. The background has a separate console. When dealing with XHR request, make sure you turn on the resource tracker in the console, which can make life much easier. To send messages to your console, just console.log(‘This will show up in your console’) in your javascript.
HTML 5
Adding more to the feud between HTML 5 and Flash, just recently Adobe CTO announced that they will strive to make the best tools for HTML 5. Extensions can use Web API localStorage to store information to your browser. This makes live easier to not write an API to store information to sessions and track that. You can see the stored data in your WebKit.
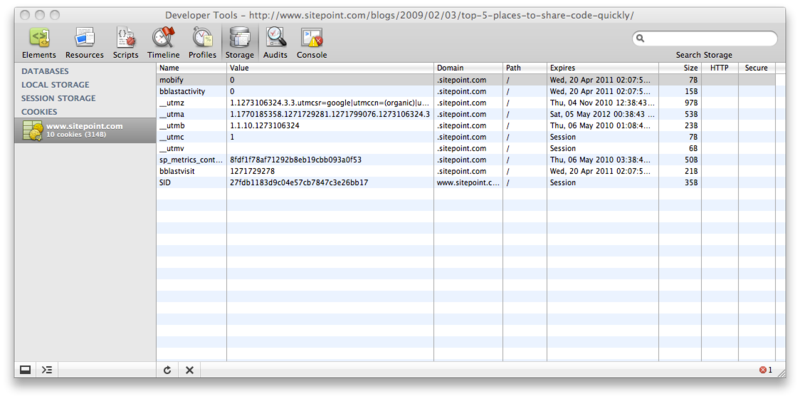
AppEngine
Finally, this is the very last part. I used AppEngine to host the TwiML to make all of these calls and SMS possible. Its free and you can create a max of 10 scalable applications each with about 6 GB of daily bandwidth and 6.50 CPU processing time. Very awesome. You can get started here at Google AppEngine references. At this time, they support Python and Java but I see a huge demand for PHP in their forum.
So the process for this pretty much starts by downloading the AppEngine SDK onto your computer. Make sure you have Python installed (this come installed on Macs). There is a graphic GUI to deploy to your server, but I have been using the command line appcfg.py. The server code for this is also available online with the extension here.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.