How to use JsonSerializerOptions with source generated JSON deserialization
Time to read: 2 minutes
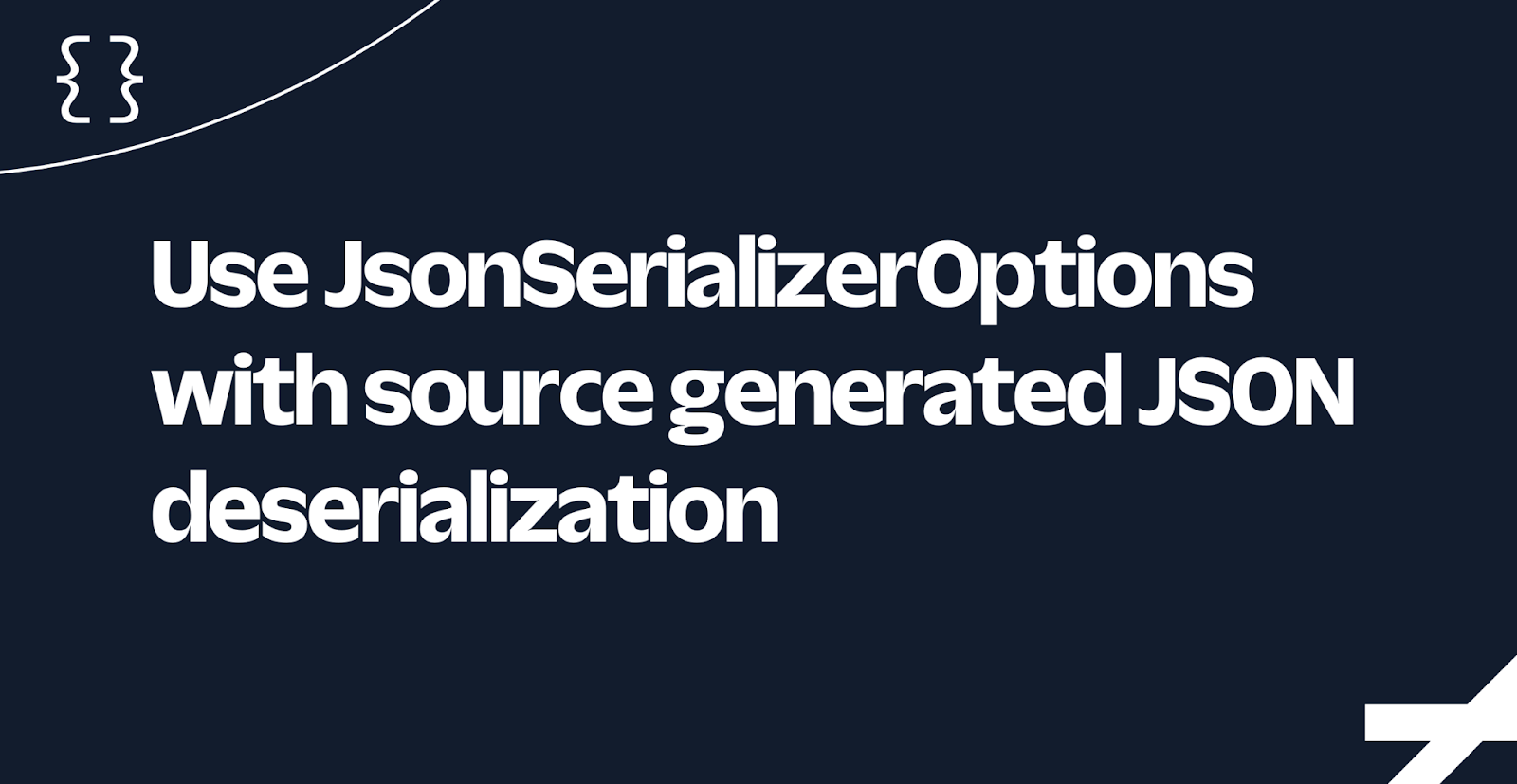
In 2021, Microsoft released a source generator that improves the performance of serialization with the System.Text.Json APIs.
It is generally easy to use, you add an annotation to the class you want to serialize/deserialize, and then use the generated type when performing the serialization/deserialization.
But it is not immediately obvious how to use JsonSerializerOptions
when deserializing.
There are a number of reasons to use JsonSerializerOptions
when deserializing. For example, if the C# properties don't match the case of the JSON keys, or you need to map an enum to a string. In these cases, you could use attributes on the class you are deserializing, but I find these annotations distracting when reading the code.
Prerequisites
You will need the following things in this tutorial:
- A Windows, Linux, or Mac machine
- .NET 6/7 SDK (or newer)
- A .NET code editor or IDE (e.g. VS Code with the C# extension, Visual Studio, JetBrains Rider, or Fleet)
The problem
If you have the following JSON -
person1.json:
And use the following code to deserialize it:
The output will be -
Not what you want.
The JSON key names are not exact matches for the property names, and the role is a string, rather than a number (the backing type of an enum).
The fix is to use JsonSerializerOptions
to control the deserialization process.
The solution
Create new console application:
Open the .csproj file and add the following ItemGroup
-
This will copy the JSON files to the output directory so that they can be read by the console app.
The JSON files
Create four JSON files:
Note that the key names don't match the name of the properties in the Person
class, Role
is a string, and that person4.json has a string for the age. This is deliberate, to illustrate how options work with the source generated deserialization code.
The type to deserialize
Create a new file called Person.cs. It has strings for first and last names, an int for age, and an enum for role. The age and role are deliberately not strings, to illustrate features/deficiencies of the source generated deserialization code.
Lines 3-4 are the annotations that tell the source generator to generate the code to serialize/deserialize the Person
class.
Performing the deserialization
In the Program.cs file add -
At this point, you can run the application.
The output will be -
Not what you want!
The fix is to use JsonSerializerOptions
to control the deserialization process.
Add the following code to Program.cs:
Deserialization approach 1
You can try again to deserialize the json1
string. Pass the options
object to the Deserialize
method:
The output will be:
Deserialization approach 2
Create an instance of MyJsonContext
and pass in the options you created above:
Now you can deserialize the JSON using the Deserialize
overload that takes JsonTypeInfo<TValue>
.
The output will be:
Deserialization approach 3
Another way to deserialize is to use the Deserialize
overload that takes a Type
and JsonSerializerOptions
.
The output will be:
The problem with JsonNumberHandling.AllowReadingFromString
When using source generated code for deserialization, the JsonNumberHandling.AllowReadingFromString
option is not supported.
The file person4.json has a string for the age - "age": "44"
. This will throw an exception when deserialized.
The output from this will be:
If you need support for handling numbers read from strings, source generated deserialization will not work for you.
Conclusion
In this post, you learned how to use JsonSerializerOptions
with source generated deserialization code. But you should keep in mind that not all the familiar options are not available when using source generated code.
Bryan Hogan is a blogger, podcaster, Microsoft MVP, and Pluralsight author. He has been working on .NET for almost 20 years. You can reach him on Twitter @bryanjhogan.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.