Using the Latest Twilio NuGet Package with Legacy Versions of the .NET Framework
Time to read: 5 minutes
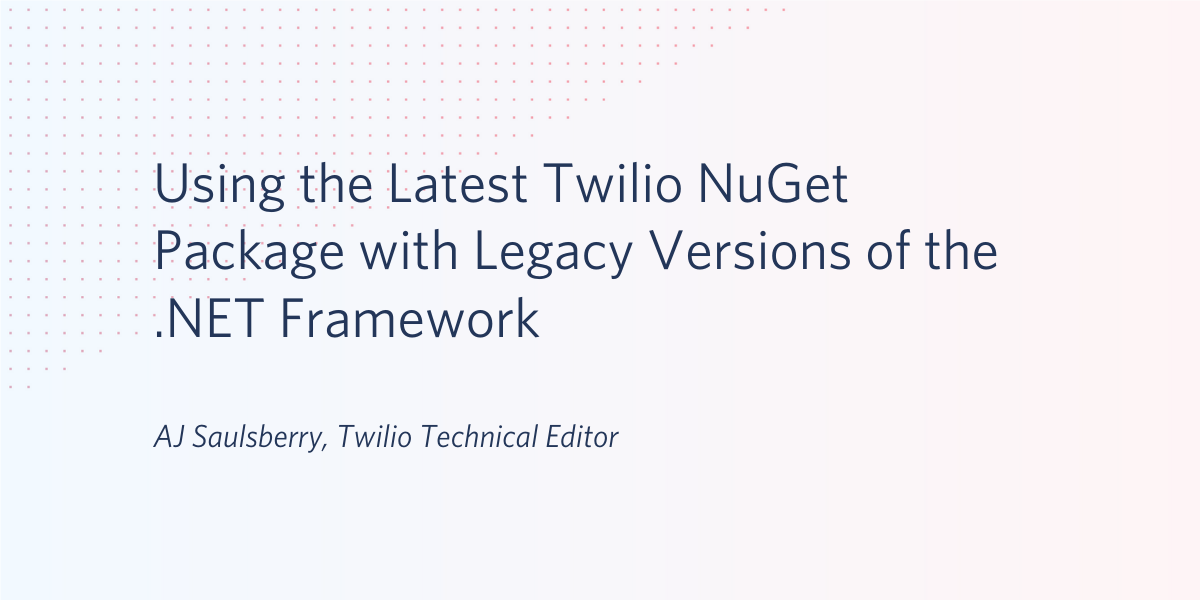
Developers have a natural inclination to work with the latest and greatest technologies; new libraries, language features, and tools are some of the things that keep the profession interesting. Production environments, on the other hand, often lag behind because of stability, compatibility, and cost considerations.
Twilio understands both perspectives, so .NET developers who build software for Windows machines get the best of both worlds when they work with Twilio components. You can develop with the latest tools and use the most recent Twilio libraries and API capabilities while maintaining compatibility with legacy operating systems and .NET frameworks.
This post will walk you through a quick case study project demonstrating how to code an application that can run on older Windows systems and legacy versions of the .NET Framework while using the latest version of Visual Studio 2019 and the newest Twilio NuGet package. You can use the code to test platform compatibility and deployment with the software you run in your production environment.
Prerequisites
You’ll need the following resources to complete the tutorial in this post:
Visual Studio 2019 – The Community edition is free.
.NET Framework 4.5.2 – Download and install the Developer Pack.
Twilio account – Sign up for a free trial account with this link and get an additional $10 credit.
There is a companion repository containing the complete source code for this project available on GitHub.
Obtaining your Twilio credentials
If you don’t already have a Twilio account, use the link above to create one. During the registration process be sure to register a phone number that can receive SMS messages.
Go to the Twilio console dashboard and get the Account SID and Auth Token for the ExplorationOne project (or the project of your choice, if you’ve created additional projects). You can find these credentials on the upper right-hand side of the dashboard. These are user secrets, so copy them someplace safe and handle them securely.
Obtaining an SMS-enabled Twilio phone number
Go to the Phone Numbers page on your Twilio console and add a number with SMS capabilities. You won’t be charged for the number you register in your trial account. Copy the phone number someplace handy.
For more information on getting started with your Twilio trial account, see How to Work with your Free Twilio Trial Account.
Creating the Visual Studio project
It may be a challenge remembering which features are included in all the various versions of the .NET Framework and .NET Core, but working with old and new framework versions is easy with Visual Studio 2019. Start by creating a new project and narrow down the list of templates by using the filters to select C#, Windows, and Console for the language, operating system, and project type, respectively.
Choose the Console App (.NET Framework) template.
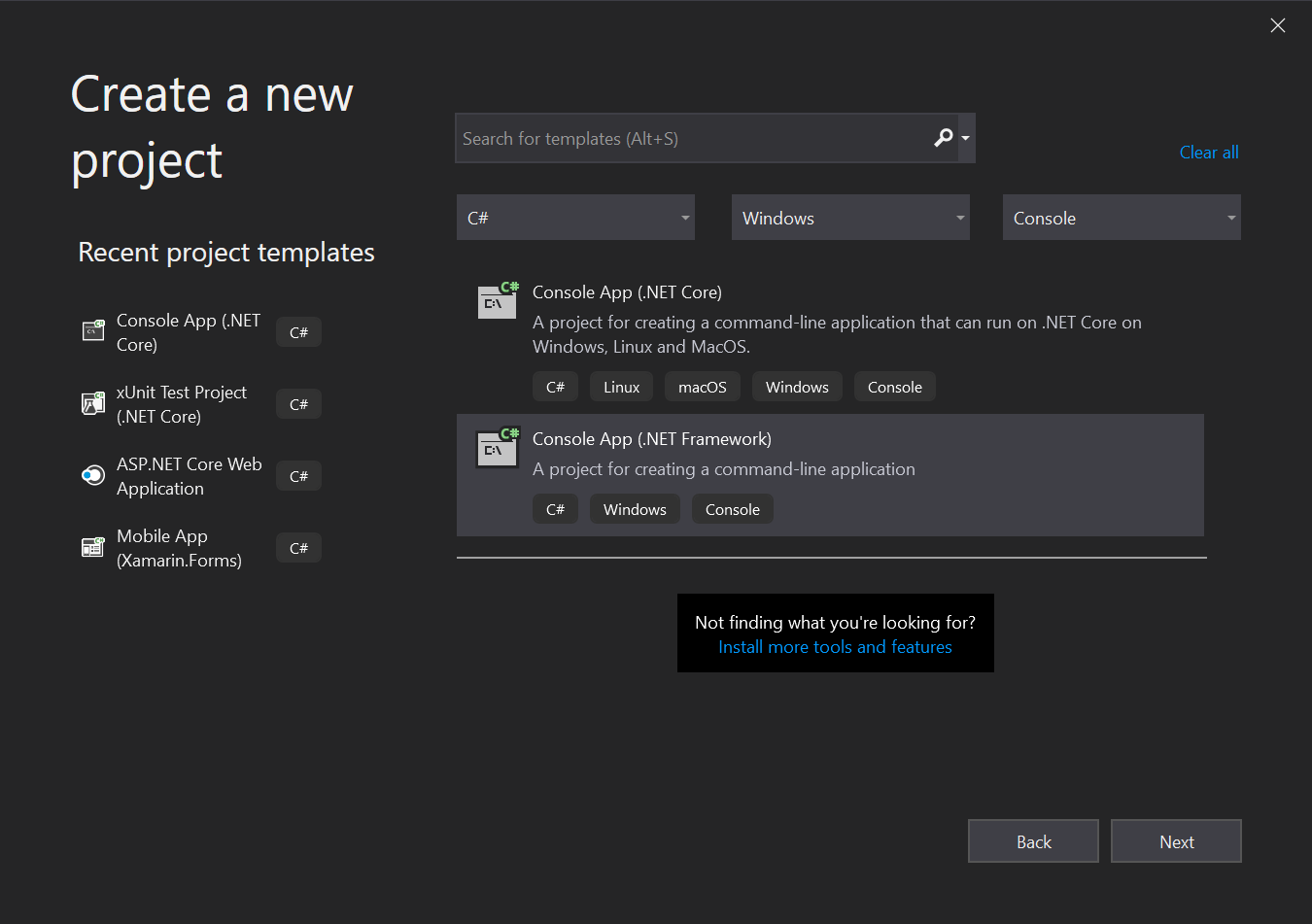
In the Configure your new project window, name the project FrameworkReporter and select an appropriate location for the files.
In the Framework box, select .NET Framework 4.5.2.
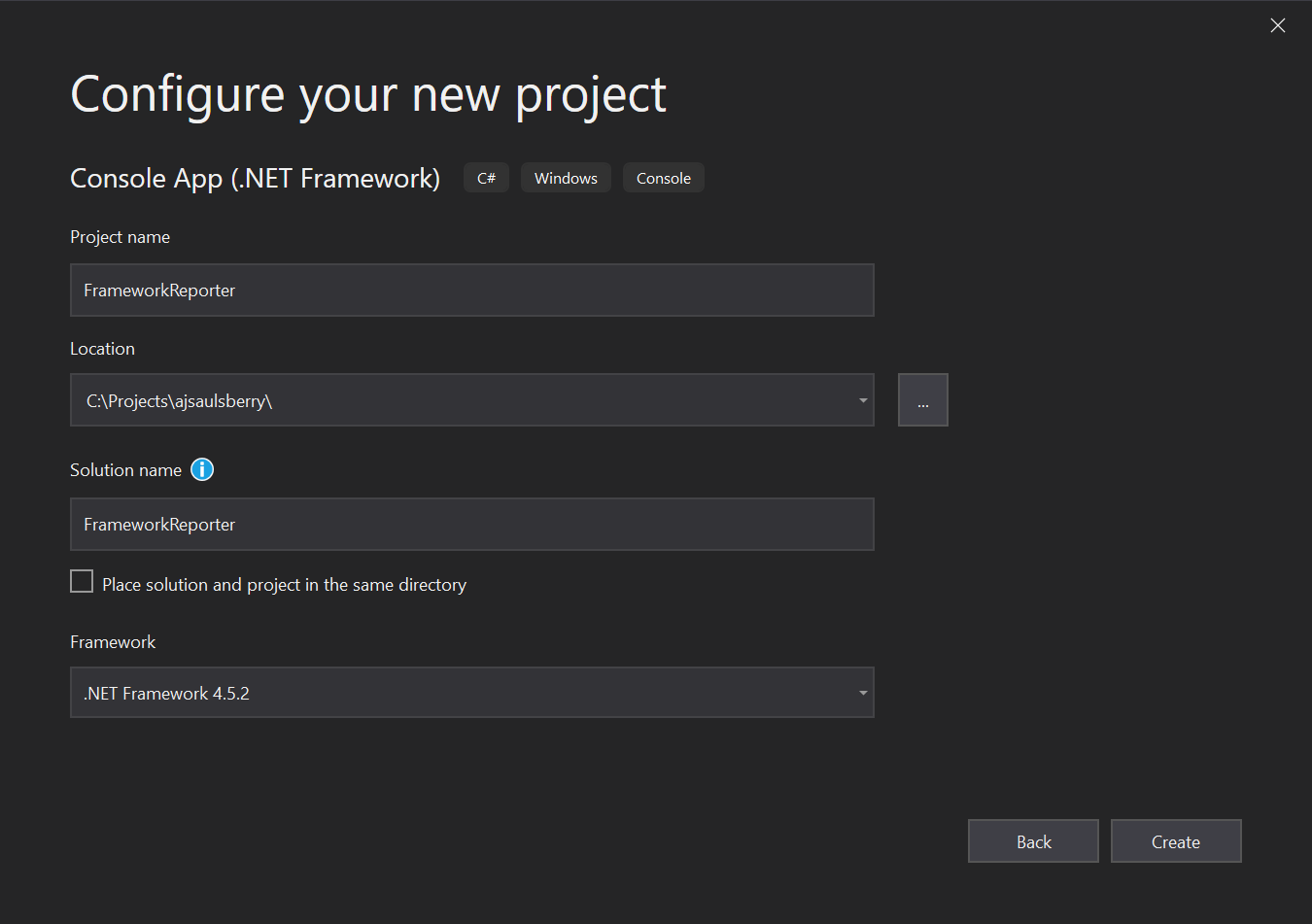
Create the project. After the tooling is finished chugging you should see the trusty Main
method of the Program
class.
Note that the tooling also creates an App.config file.
The FrameworkReporter project will use the System.Configuration.ConfigurationManager class to load your Twilio credentials. In .NET Framework 4.5.2 it’s included in the System.Configuration.dll assembly, so you’ll need to add a reference.
In the Solution Explorer panel, expand the References node under the FrameworkReporter.
Right-click on References and click Add reference.
In the Reference Manager, scroll through the long list of available assemblies and add a checkmark next to System.Configuration. Click OK.
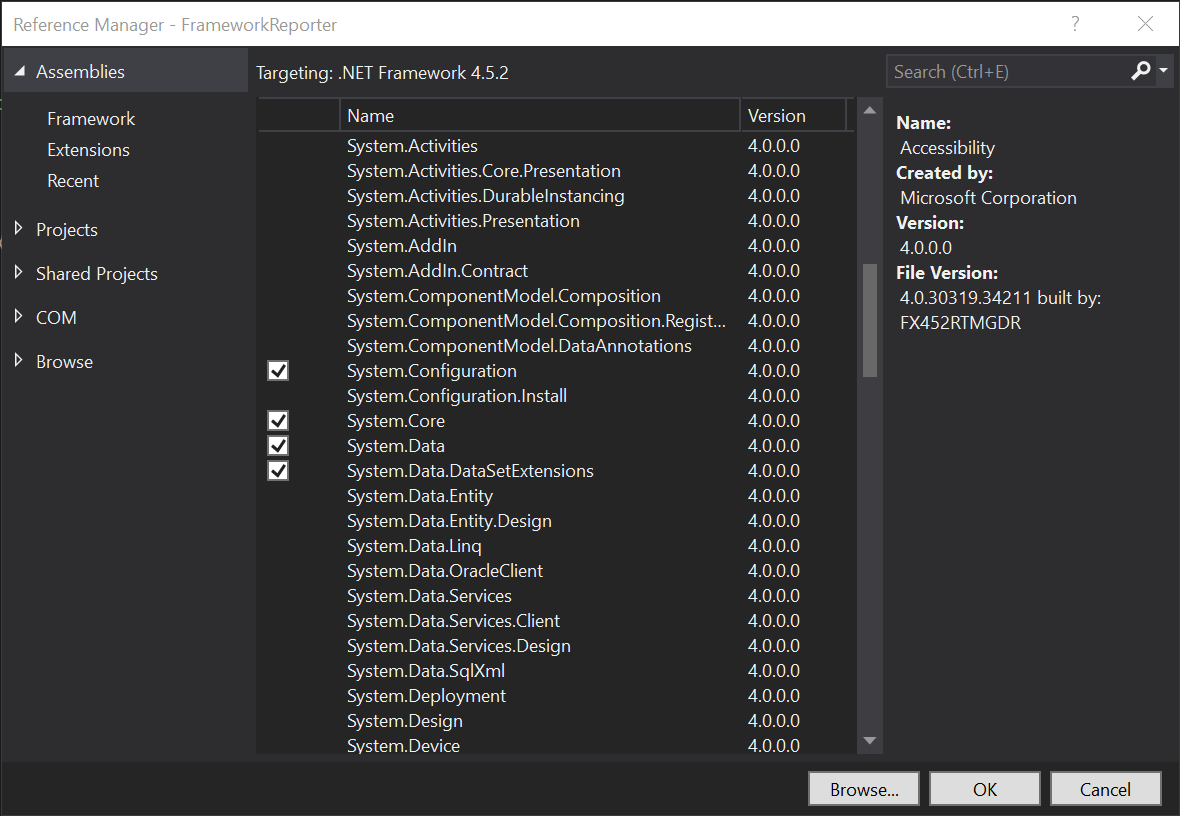
You should see System.configuration under the Reference node. Note that the lowercase “c” in “configuration” seems to be normal; it shouldn’t affect the operation of the program.
This would be a good time to check the solution into source code control.
Adding Twilio credentials to the project
In .NET Framework console projects of this vintage it’s conventional to put configuration information in the App.config file, so that’s where your Twilio credentials will go. Since these values are user secrets connected to your account, you’ll want to exclude this file from your source code control repository if you’re planning on making it public or widely accessible.
For development purposes, open the App.config file and add the following <appSettings>
node to the <configuration>
node:
Replace the placeholder values with your actual Twilio Account SID, Auth Token, and SMS-enabled phone number. The phone number should be entered in E.164 format.
Adding the Twilio NuGet package
This is a simple demo, so you’ll only need one essential NuGet package. Open the Package Manager Console window and execute the following Package Manager CLI instruction:
This will install the latest version of the Twilio helper library for .NET. As of this writing it’s 5.41.0. A number of dependencies will also be installed and will appear under the References node.
Great! Now it’s time to code all 16 lines of the program (not including comments).
Sending SMS messages from a .NET Framework 4.5.2 console app
Open the Program.cs file and replace the existing using
directives with the following:
Replace the contents of the Main
method with the following:
As of June, 2019, Twilio’s REST APIs require Transport Layer Security (TLS) version 1.2. This is pretty standard for apps built with the current versions of .NET Core and the .NET Framework, but it’s not turned on by default in .NET Framework 4.5.2. The first statement in the Main
method forces TLS 1.2 to be used.
Note for Windows 7, Windows Server 2008, and Windows Server 2012 users: TLS 1.2 might not be enabled if you haven’t been keeping up with service packs and Windows Update. See this Microsoft Support article for more information: Update to enable TLS 1.1 and TLS 1.2 as default secure protocols in WinHTTP in Windows. As always, make a backup, create a restore point, and devise a rollback plan before making manual modifications to the Windows Registry.
Testing the application
Press F5 and pick up your phone. If everything is working correctly you should receive an SMS message from the application momentarily.
If you don’t get a message and your application isn’t throwing errors, you can check the message log for the number to see if Twilio is receiving the message. Go to the Phone Number section of the Twilio console, click on the phone number you’re using in the app, and select the Messages Log tab.
If you’re having problems with TLS, verify the machine has TLS 1.2 enabled by going to Internet Options in Windows Settings or the Control Panel. Click on the Advanced tab and scroll down. Use TLS 1.2 should be listed and checked.
Potential enhancements
Although this is a simple demo app, there are a number of things you could do to make it more useful. Here are some ideas:
- Add functionality to determine which .NET Framework versions are installed on a machine.
- Have the messages the app sends identify which .NET Framework versions are installed.
- Provision the app with a JSON file identifying a number of phone numbers to receive messages.
- Turn the app into a Windows service so it can run continuously on a server, checking for installed (or uninstalled) .NET Framework versions periodically.
Additional resources
Programmable SMS – Twilio documentation, including quickstarts for multiple languages, tutorials, and the API reference.
Default SecurityProtocol in .NET 4.5 – One of the more exhaustive Stack Overflow topics on TLS support in .NET Framework 4.5.
Lifecycle FAQ - .NET Framework – This is the place to start for understanding how long you have before your operating system or .NET Framework edition is no longer supported.
TwilioQuest – Level up your programming skills by completing missions in Twilio’s adventure game. It’s fun for the whole quarantined family!
Encrypting the Web – The EFF explains why encryption is so important to security and what you can do to help
AJ Saulsberry is a Technical Editor at Twilio. Reach out to him if you’ve been “there and back again” with a .NET development topic and want to get paid to write about it for the Twilio blog.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.