Employee Directory with Python and Flask
Time to read:
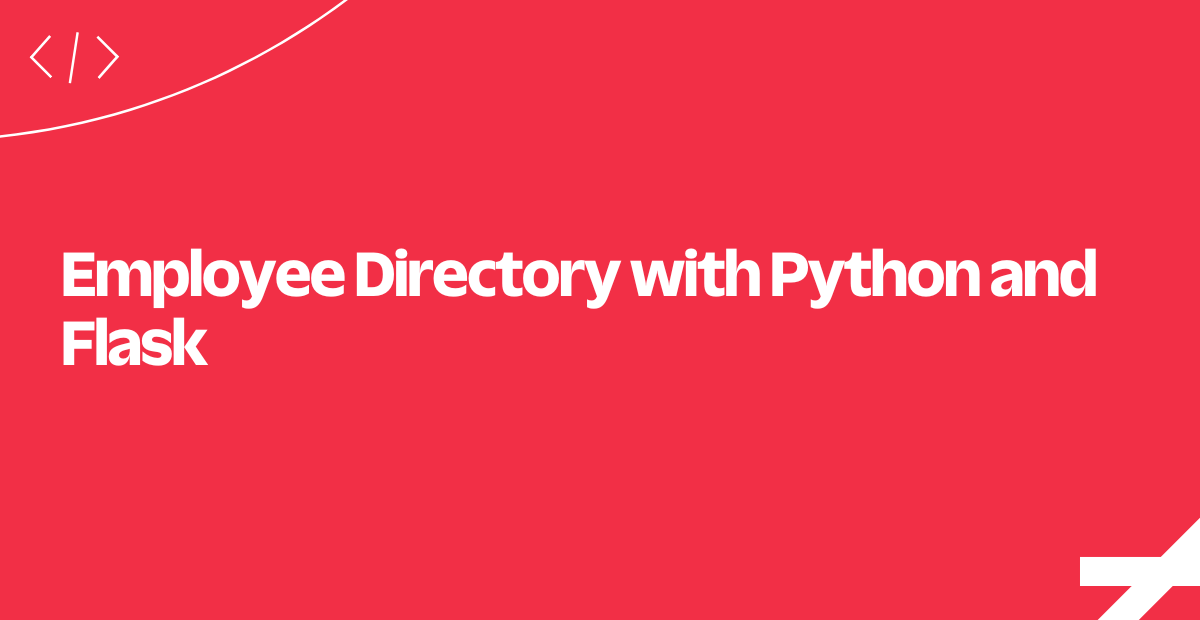
Learn how to implement an employee directory that you can query using SMS. Request information from anyone at your company just by sending a text message to a Twilio Number
Here is how it works at a high level:
- The user sends a SMS with an Employee's name to the Twilio number.
- The user receives information for the requested Employee.
Handle Twilio's SMS Request
When your Twilio Number receives an SMS, Twilio will make a POST request to /directory/search
asking for TwiML instructions.
Once the application identifies one of the 3 possible scenarios (single partial match, multiple partial match or no match), it will send a TwiML response to Twilio. This response will instruct Twilio to send an SMS Message back to the user.
Let's take a closer look at each one of the scenarios.
Single Employee Match
This is the simplest scenario. We'll verify that only 1 match is obtained. If a single match is found, a message containing this employee's information is built and sent to Twilio as TwiML instructions.
If multiple matches are found we'll try to do a multiple partial match. That is our next possible scenario.
Multiple Employee Matches
At this point we have already tried to use the user's query as a single partial match. Now we'll try to get a partial match that returns more than one result. We'll use Twilio Cookies to store suggestions. The only difference here is that we use a list to store suggestions. This way the user can reply with a number that references one of the suggestions in order to get the employee's information. The way this information is stored will be explained on the next step.
The last scenario is simple. If none of the previous scenarios occur, it means that there is no employee in the database that matches the user's query. In that case, a reply will be sent to the user explaining that their query doesn't match any of the employees found on the database.
Next, we'll see how we use cookies in Flask to cache search suggestions.
Store Suggestions With Cookies
When a user gets a partial match by searching the employee directory, we reply with one or more suggestions. We need to store these suggestions, so the next time the user sends an SMS we know this is not a query for a new employee, but a selection of one of the suggestions.
We'll use Twilio Cookies and a Flask Session to store suggestions. They will allow you to keep track of an SMS conversation between multiple numbers and your Twilio powered application.
That's it! We have just implemented employee directory using Flask. Now you can get your employee's information by texting a Twilio number.
Where to next?
If you're a Python developer working with Twilio, you might also enjoy these tutorials:
Instantly collect structured data from your users with a survey conducted over a call or SMS text messages. Let's get started!
Learn how to implement ETA Notifications using Flask and Twilio.
Did this help?
Thanks for checking out this tutorial! If you have any feedback to share with us, we'd love to hear it. Tweet @twilio to let us know what you think!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.