Employee Directory with Ruby and Sinatra
Time to read: 2 minutes
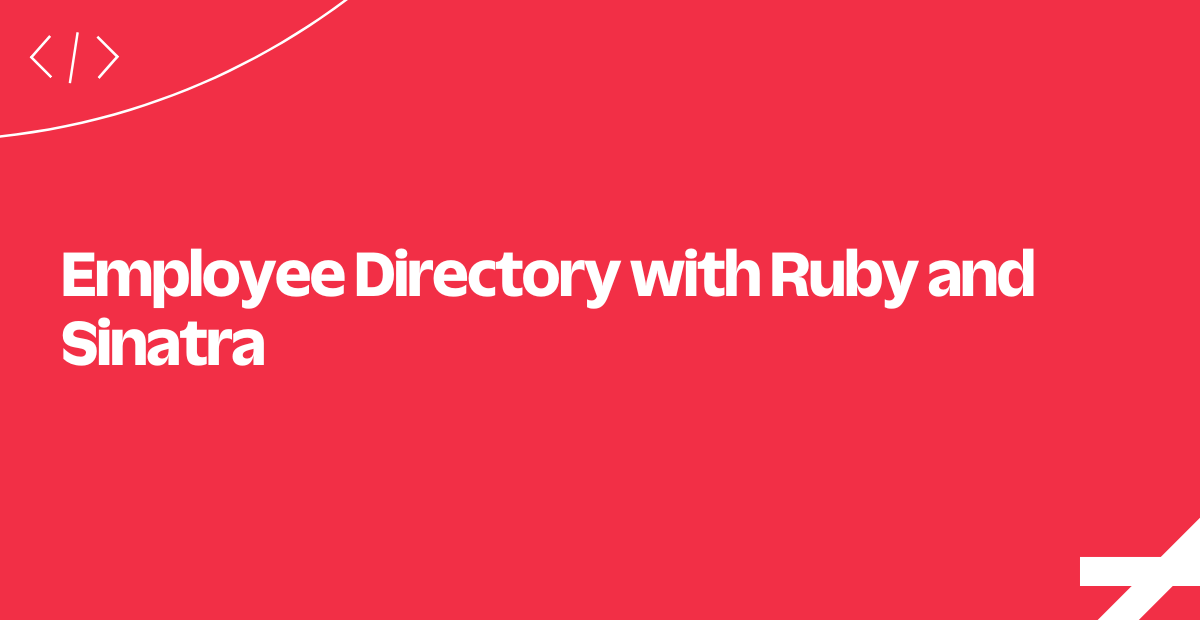
This Sinatra application is an Employee Directory that can be used from any SMS client to find an employee's contact information. To build this app, we will need to.
- Create an Employee model.
- Receive an incoming SMS.
- Search for an Employee by name.
- Respond with an outgoing MMS.
Create an Employee Model
The first thing we need is a database of employees. We will be using DataMapper for this. It will allow us to define our database structure without having to worry with database specifics.
Our employee entity has a few fields for contact information, including their name, e-mail, phone number, and a public URL containing an image of them.
Now, let's build the logic to search for employees.
Search for an Employee by Name
The Searcher
module allows us to search the database for employees either by name or by their unique database identifier.
When searching using the employee name as parameter, we'll return a list of employees whose name might match a search query. If we pass a number as parameter we'll use it to search the employee by its id.
We'll use this search functionality when responding to an SMS from a user, which we'll look at next.
Receive an incoming SMS
When your number receives an SMS message, Twilio will send an HTTP POST request to our application. This will be handled by the Lookup
action in EmployeeController
.
We check for numeric input (more on that later) or perform a lookup for the desired employee. The results are packaged up as a XML and sent back to Twilio and, in turn, the original sender of the SMS.
Let's take a closer look at building the response.
Respond with Single Match for an Employee Name
Let's say the service finds a single employee matching the text message. In this case, we simply write out a response that contains the employee's contact information, including a photo, making our response a MMS message.
A single matching employee isn't the only scenario, however. Next, we will learn how to handle multiple employee matches.
What If No Employee or Multiple Employees Match?
If we don't find any employees, we can simply return a "Not found" message.
What about multiple matches? For this case, we want to return a list of the matching employees' names along with an ID number the end user can use to make their selection. For example, if someone searched for "Peter" they might get something like:
1-Peter Parker 2-Peter Quill
Let's see how these options are stored next.
Return Employee's Contact Information by Number Choice
When searching for an employee, we check whether the body of the text input is a number. If that's the case, we use it to retrieve the employee by its ID. Otherwise, we use it to do a search by employee's name.
That's it! We've built a working Employee Directory.
Where to Next?
Take a look at the code on GitHub to run the application yourself. There you will find the complete solution and instructions for getting up and running.
If you're a ruby developer working with Twilio, you might want to check out these other tutorials.
Automated Survey with Ruby and Sinatra
Receive and Reply to SMS and MMS Messages in Ruby
Did this help?
Thanks for checking out this tutorial! If you have any feedback to share with us, we'd love to hear it. Tweet @twilio to let us know what you think!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.