SMS and MMS Notifications with Ruby and Sinatra
Time to read: 2 minutes
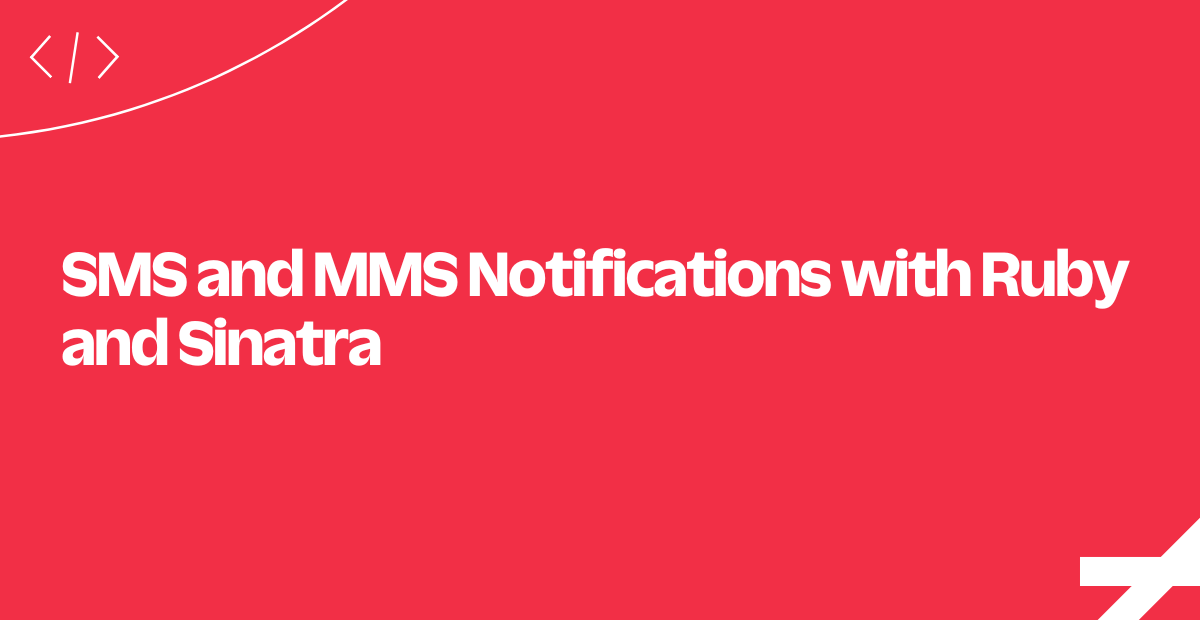
Today we'll use Ruby and the Sinatra framework to demonstrate how to send SMS alerts to a list of system administrators when something goes wrong with your server. We'll cover all the key details in depth so you can easily incorporate this important feature into your own application.
Clone our sample application from Github, then head to the application's README.md to see how to run the application locally.
Let's get started!
Configuring the Twilio REST Client
To send messages we'll need to create a Twilio REST client which requires reading a TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
from environment variables.
The values for your account SID and Auth Token will come from the Twilio console:
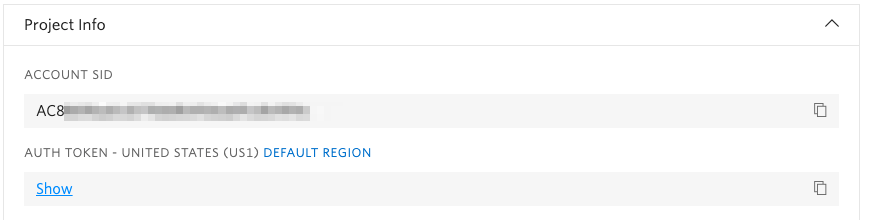
Click the eyeball icon to expose your Auth Token in a form you can copy and paste.
Our Twilio REST Client is now ready. Let's take a closer look at that administrator list, shall we?
A List of Server Admins - And Anyone Else Who Should be Hailed
Here we create a yaml list of people who should be notified if a server error occurs.
The only essential piece of data we need is a phone_number
for each administrator.
Next, let's look at how we're going to piggyback on application exceptions.
Handling Application Exceptions
We use Sinatra Errors Handlers and send out the notifications when an exception occurs.
Next up: sending a message to each administrator.
Trigger Notifications for Each Entry in the Administrator List
In our Notifier
module, we read the administrators from our YAML file and send alert messages to each one of them with the method send_sms
.
Next up, we will see how to send a text message.
Sending a Text Message
There are three parameters needed to send an SMS using the Twilio REST API: from
, to
, and body
.
US and Canadian phone numbers can also send an image with the message. Other countries will have an automatically shortened URL added to the message body.
It's as simple as that!
We've just implemented an automated server notification system that can push out alerts if anything goes wrong.
Next, let's look at what other features the Twilio Ruby SDK makes simple to integrate.
Where to Next?
We've got a lot of other excellent Ruby code we'd love to share with you... but we'll keep our links to just two:
Increase the security of your login system by verifying a user's mobile phone in addition to their password.
SMS and MMS marketing notifications
SMS and MMS messages are a personal way to engage with users, offering a much higher open rate than e-mail and more impact than a tweet.
Did this Help?
Thanks for checking out this tutorial! Tweet @twilio with what you thought, what you're thinking, and most importantly... what you're going to build.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.