Getting started with the Star Wars API in Node.js using Twilio Functions
Time to read: 3 minutes
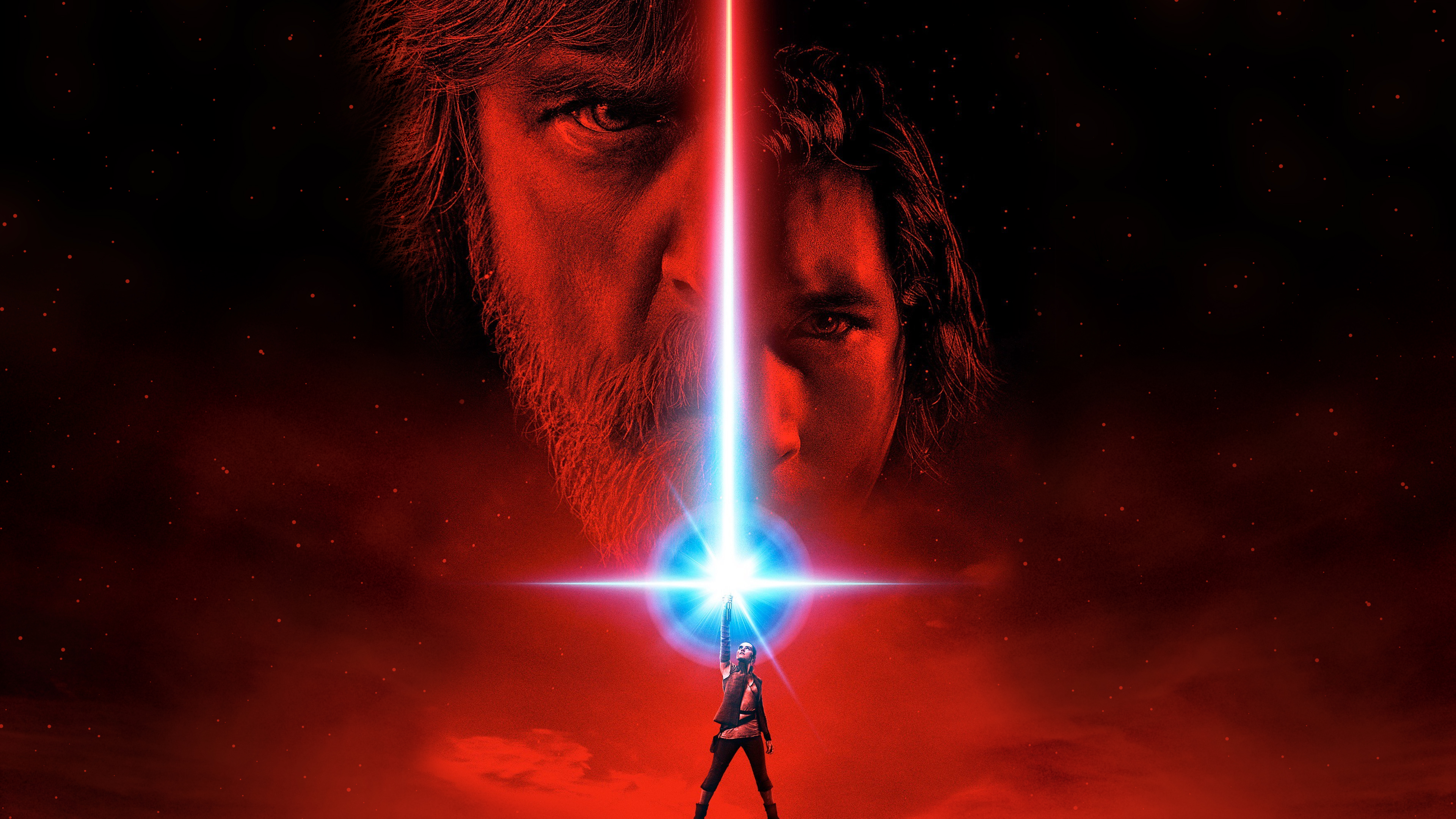
Star Wars: The Last Jedi comes out tonight and I am massively excited to see it. However, some of my other friends who are seeing the movie aren’t as familiar with the Star Wars universe as I am. Let’s use the amazing Star Wars API to build a Twilio powered SMS hack to help fix that.
We are going to use Twilio Functions to avoid having to go through the hassle of deploying a web application, the Star Wars API to gather relevant data on Star Wars characters, the Giphy API to grab GIFs of those characters, and Twilio SMS to facilitate the communications with our users.
Working with the Star Wars API
The Star Wars API is a very simple REST API that only functions using GET requests. Twilio functions gives us access to Got, which we can use for these HTTP requests. In particular, we’ll be working with the people endpoint.
Here’s some example code on how to use Got to grab the data on Luke Skywalker, if you want to run them in your own terminal to test things out, you’ll need to have node.js and npm installed, and will need to run npm install got
in the directory your code lives in:
There’s also search functionality that we can use to find characters given a query:
If you want to get access to a character’s species or homeworld, you have to make another API request:
Now that we know how to work with the Star Wars API, let’s put it in a Twilio function.
Setting up a Twilio Function
You’ll need to create a Twilio account if you don’t already have one. From the Twilio Functions page in your Twilio Console, create a new Function and select the “Blank” template as seen in this image:
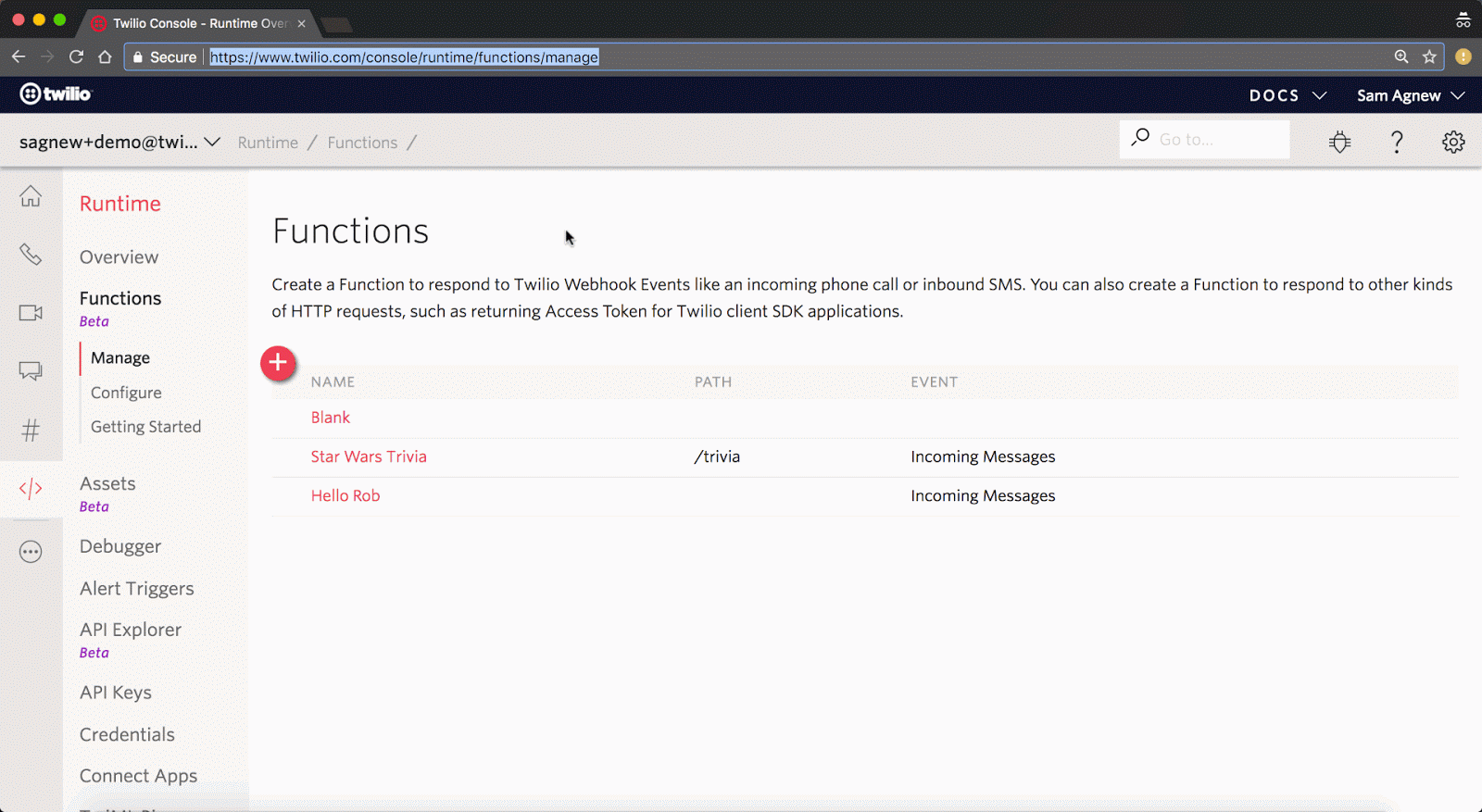
Give your function a name and a path.
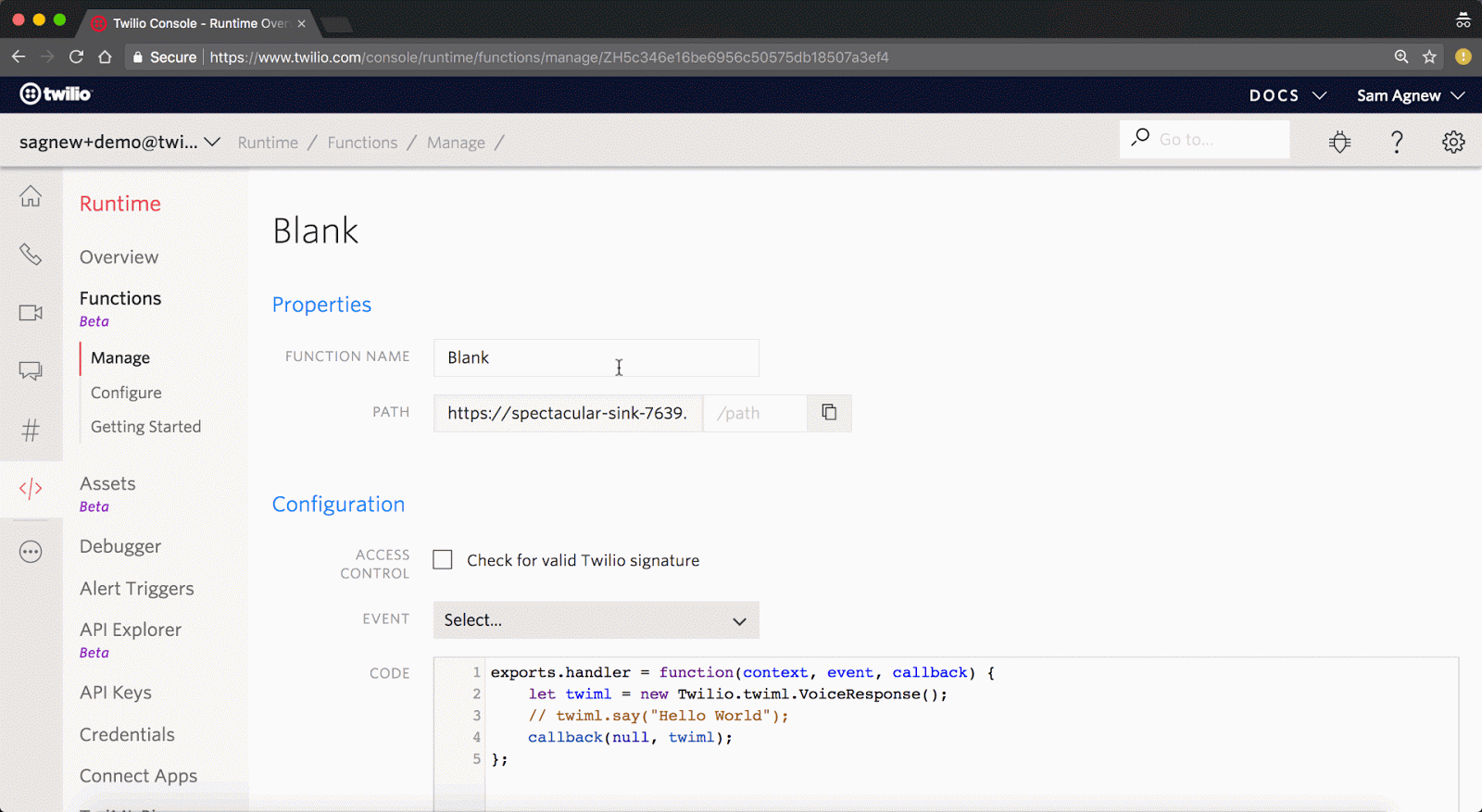
Now insert the following code for our app, and click save:
What’s happening in this code is that the user:
- Texts the Twilio phone number that you will link this function to.
- Your function executes and makes several back-to-back requests to the Star Wars API to grab all of the relevant data: the character’s name, species, and home planet.
- After the Star Wars data is gathered, a request to the Giphy API is made to grab a GIF of the character your user searched for.
- When all is said and done, some TwiML is generated to tell Twilio to respond with a text message.
Getting everything running
Now buy a Twilio number and link it up to your function. After buying your number, scroll down to the “Messaging” section and configure it to call your function whenever it receives a text message, as seen in this image:
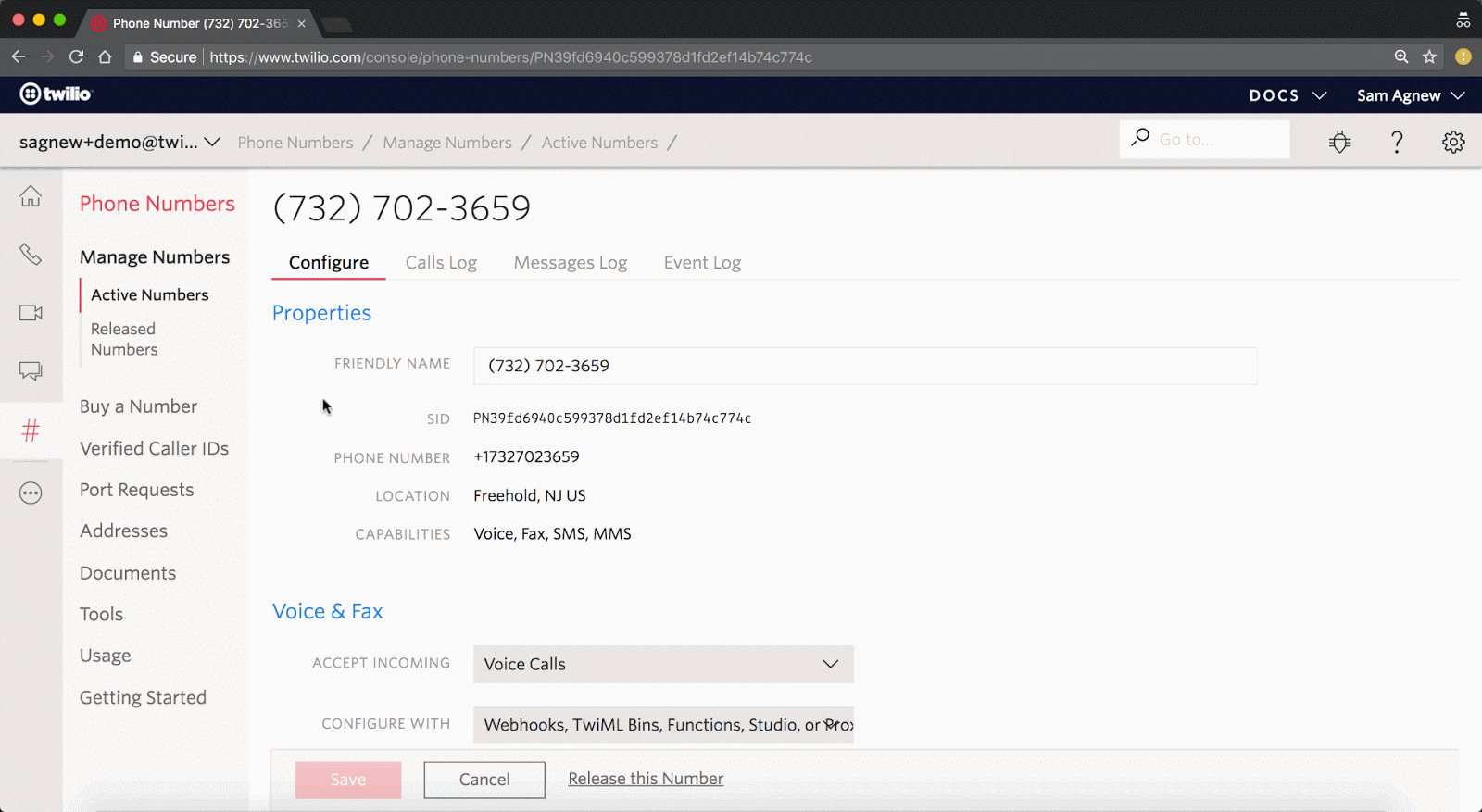
Now you should be able to text your phone number and see some Star Wars info!
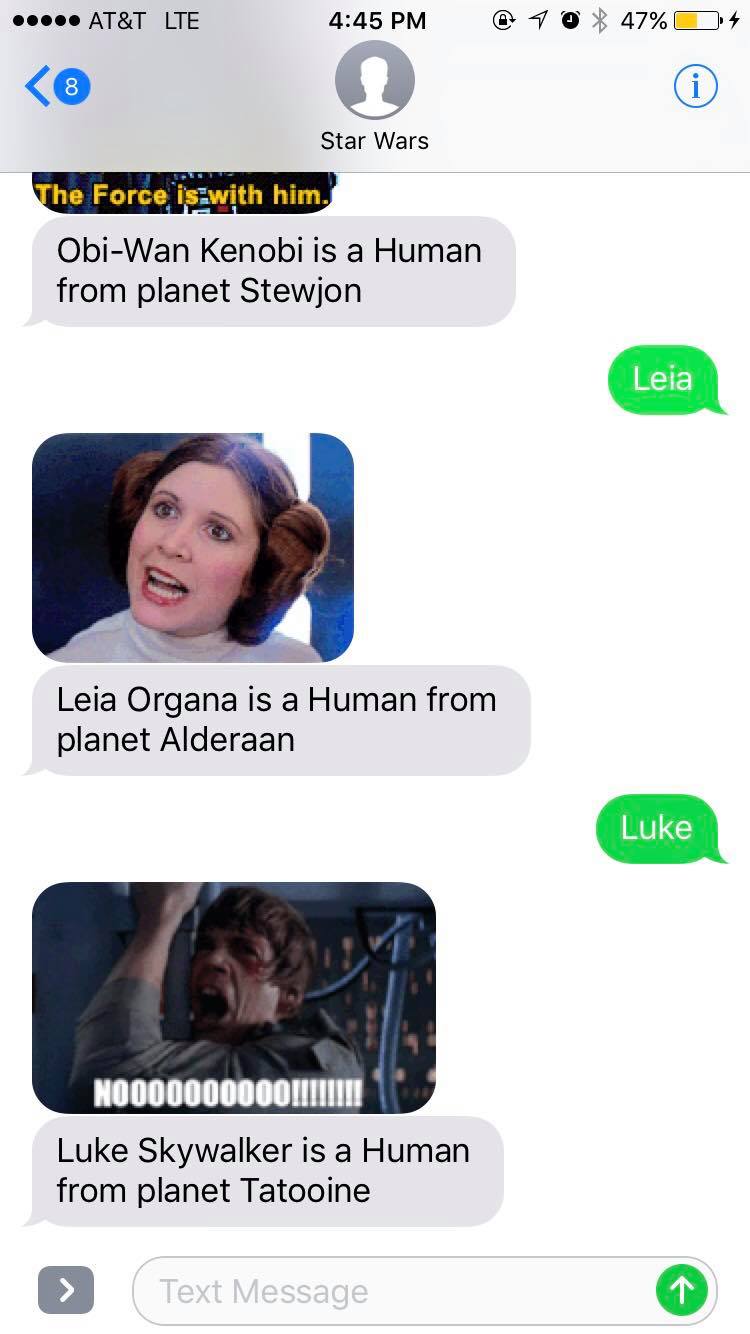
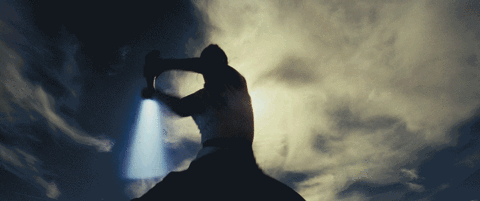
Feel free to reach out if you have any questions or comments or just want to show off the cool stuff you’ve built.
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.