Leaving A Message For A Rockstar with Python and Twilio
Time to read:
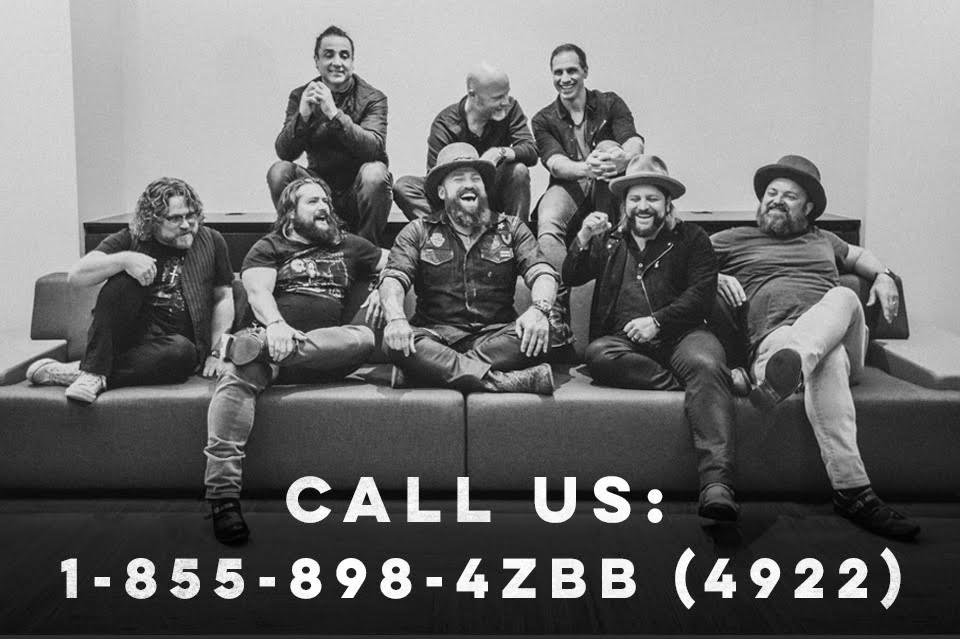
You know when you’re selling out stadiums and want to connect with your fans one-on-one but can’t exactly give out your personal phone number? Yeah, common problem — for Zac Brown & his band.
Zac Brown Band has about 1.67 million Twitter followers. The ZBB squad wanted to reach out to every fan before dropping their new record (which came out today). Dialing up 1.67 million people is tricky, so the ZBB team let the fans come to them.
Now, all of them can call up Zac Brown Band’s Twilio number and leave a message relaying their favorite memory, song, or moment from an album.
Here’s an initial prototype of the app I built for Zac’s management crew to get ideas flowing. The entire app runs on about 60 lines of Python.
The goal is to do the following things in order:
- Greet the caller with a friendly message
- Allow them to record a message
- Tell Twilio when they’re done recording a message and bid them adieu.
In this prototype, we set a max length of 30 seconds to ensure the team can get through everyone’s messages easily, and to ward off any would-be-butt-dial voicemails.
Here’s all the code you need to make it happen.
If you’ve got any ideas for what you can build in Python, keep me posted – kylekellyyahner.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.