New C++ Twilio API Wrapper by Laurent Luce
Time to read: 3 minutes
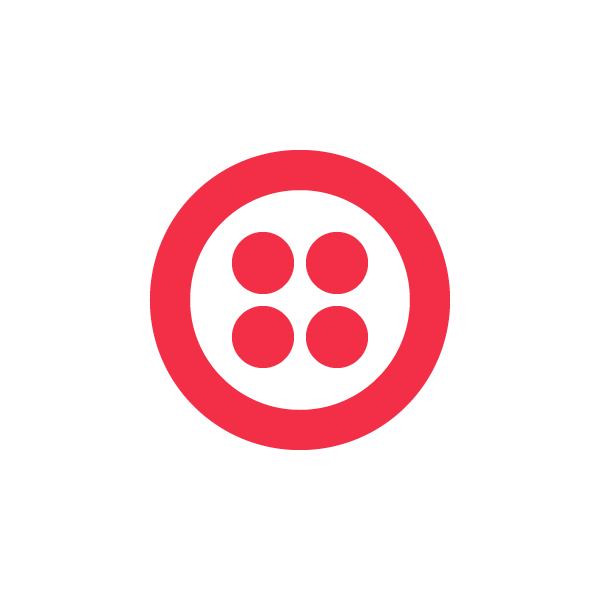
The C++ Twilio REST SDK simplifies the process of making requests to the Twilio REST API, generating TwiML and validating HTTP request signatures using C++. The project is hosted on GitHub.
The library consists of the following files:
- Rest.cpp: Twilio REST helper
- TwiML.cpp: TwiML generation helper
- Utils.cpp: Utils to validate a HTTP request signature: X-Twilio-Signature
- Examples.cpp: Examples of usage
- UnitTests.h: Unit tests
- Makefile: makefile to build the library (requires libcurl and openssl), examples code and unit tests suite.
To build the library just run ‘make’. Run ‘make examples’ to build the examples and run ‘make unittests’ to build the unit tests suite and run it. When using the library, don’t forget to include Utils.h, Rest.h and TwiML.h at the top of your source code and to use the twilio namespace: “using namespace twilio;”.
Laurent put together the following code examples based on a restaurant dish recommendation service using SMS for communication. For all of the examples, we are going to use the C++ std namespace: “using namespace std;” and define the following constants:
Receiving and replying to a SMS asking for a restaurant dish recommendation.
When someone asks for a dish recommendation, he sends a SMS with a text such as “Burger in San Francisco”. Twilio issues a HTTP POST request to your server with the text in the POST parameter “Body”. The URL called is SMS_URL.
First, we need to validate the HTTP POST request to make sure it is coming from a Twilio server. We use the Utils class to help us with that.
Then we need to reply with our dish recommendation. We use the classes TwiMLResponse and Sms to help us generate the XML.
response.toXML() returns the following:
Sending a daily recommendation to a list of subscribers using SMS.
We need to issue POST requests using the following URL: /2010-04-01/Accounts/{AccountSid}/SMS/Messages. In the following code, “subscribers” is a vector containing the phone numbers that we need to send to. We use the class Rest to help us send the SMS messages.
Retrieving the list of SMS messages we sent and their status.
We need to issue a GET request using the following URL: /2010-04-01/Accounts/{AccountSid}/SMS/Messages. We use the class Rest to help us with the GET request.
“res” should contain the list of SMS sent:
Note that pagination might need to be handled if the number of SMS messages is too large for one HTTP response. See the paging documentation for more details.
There is a lot more you can do using the Twilio C++ library so go ahead and have fun with it. Laurent would love to get your feedback and ideas over on the GitHub project page as well.
Do you have a Twilio API library or other code the community may find useful? We want to hear from you! Let us know in the comments or email help@twilio.com so we can write about it.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.