How to Send 1000 (or more) Text Messages in PHP Without Timing Out
Time to read: 5 minutes
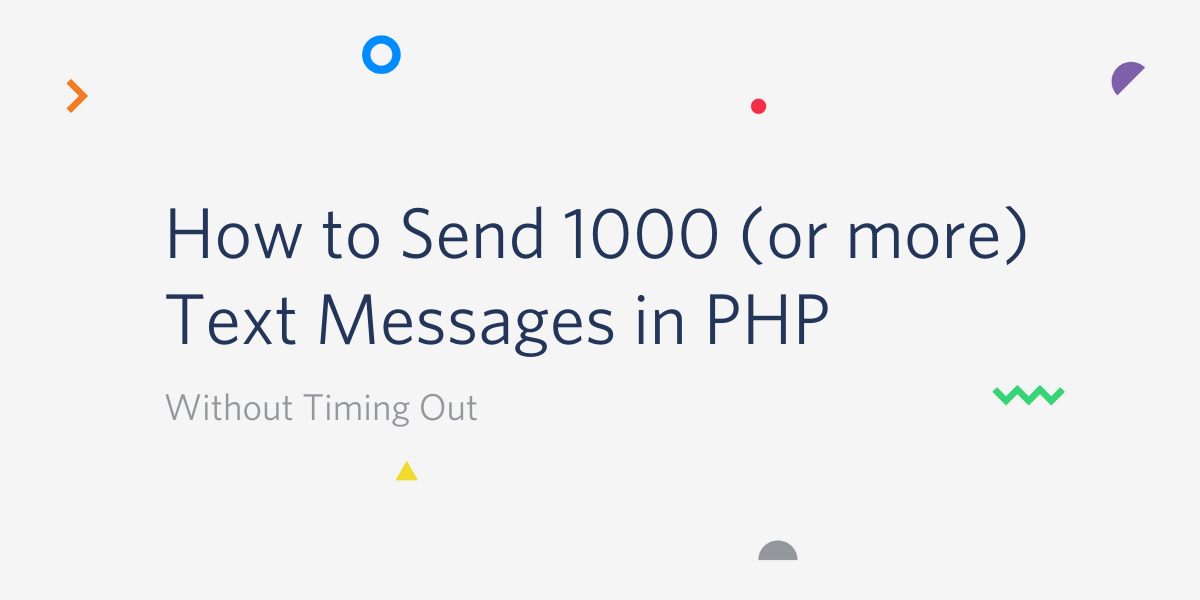
PHP developers of all experiences are painfully aware that your script may not execute if it takes longer than 30 seconds to process. There’s nothing worse than failing to do proper testing and finding that your code doesn’t scale in production.
In a previous tutorial, I shared how you can calculate the script execution time of sending SMS messages. At the conclusion we discovered that each message takes about .33 seconds to send, meaning that PHP’s 30 second timeout will allow you to send 90 messages before failure.
So what do you do when you want to send more than 90 messages? What about 1000?
Unfortunately, if you want to send bulk SMS (or WhatsApp) messages using the standard Programmable Messaging API, it’s not going to scale in PHP. Great engineering practices would suggest that you break apart your requests and run batches asynchronously. However, Twilio engineering has provided another service specifically for bulk sending called Twilio Notify.
In this quick tutorial, we will learn how to send bulk SMS in PHP using Twilio Notify.
Set Up a New Project Folder
Even though this tutorial only requires one script to execute, we will need a folder to store the dependencies required to securely connect to the Twilio Notify API. We will be using Composer to include the Twilio SDK along with .env files to securely store the Twilio credentials.
In your command line create a new project folder where you normally keep your development files.
Now that the project folder is created, a composer.json file will need to be created in order to store our dependencies.
When prompted to “define your dev dependencies (require-dev) interactively” type n
for “No.”
Add the Twilio SDK
Now that Composer is installed, we’re ready to install the Twilio PHP SDK. Run the following in your command line:
To send the SMS, we will need a PHP script to contain the code that communicates with the Twilio Notify service. In the sms-bulk-send folder, create a new file called send-bulk-sms.php.
Once the file has been created, require the vendor library and include the Twilio Rest Client to access the Twilio API.
The Twilio API requires that all requests are authenticated using your Account SID and Auth Token. Both of these credentials are available within your console. Copy and paste those credentials to a safe place on your computer.
In order to use them within the code, we will install the dotenv package so that the credentials aren’t actually visible within the code or accidentally committed to a public repository.
Run the following command to install the package:
Now that the PHP dotenv package is installed, we can create two new variables ($account_sid
and $auth_token
) to include your Twilio credentials.
Create a new .env file in the project folder and define the following credentials with your values from the console:
Add the following code below the Twilio Rest Client requirement in the previous step:
This code loads the .env variables into the file and assigns your Twilio credentials to the $account_sid
and $auth_token
variables.
Create a New Notify Service
If you’ve sent an SMS with Twilio before, then you know that you normally include the Twilio phone number as the sender directly in the API request. Using Twilio Notify differs by attaching a Twilio phone number to a Messaging Service, which Notify uses to automatically select based on the notification channel.
We’ll walk through how to set that up.
Purchase a phone number
You will need a Twilio phone number to deliver the message. If you haven’t purchased one, log into your console and navigate to Phone Numbers > Manage > Buy a number.
Attach the phone number to Messaging Service
After your number has been purchased, it will need to be connected to a Messaging Service. Messaging Services are a way to bundle senders (phone numbers) with a predefined set of features and responses.
They are perfect if you have one endpoint that needs to provide the same response, but maybe different regions. With a message service, one API response could respond to all of your subscribers in different countries, with different numbers.
In the console, navigate to Messaging > Services. If you don't have a messaging service to use, create one by clicking on the blue Create Messaging Service button. When the prompt loads, input "Send Bulk SMS" as the Messaging Service Name. Click on the blue Create button once you're done.
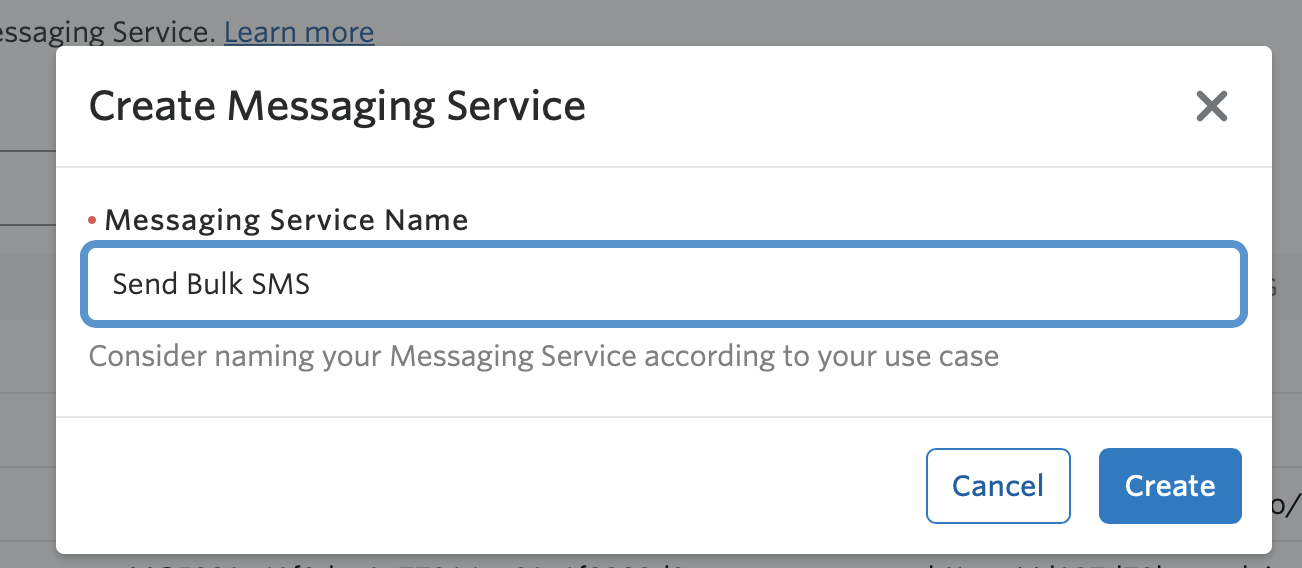
Once the service is created, you will be redirected to the Sender Pool. This option allows you to add your phone number as a sender. Follow the prompts by clicking on the Add Senders button.
Assign the Messaging Service to a Notify Service
Next, the Messaging service will need to be assigned to a Notify Service.
Twilio Notify is a service that makes it possible to reach one customer or a million on their preferred channel with just one API call. The key for our use case is that because it’s one API call, the likelihood of it completing within 30 seconds is drastically higher.
Notify Services also allow you to segment your data based on use-case. You could essentially segment your Messaging Services based on environment (dev, staging, prod) by assigning them to different Notify Services.
Navigate to Notify > Services from the Twilio Console and click the red and white plus sign to create a new Notify Service. Let's name it "Bulk Notification." Click on the red Create button to move on.
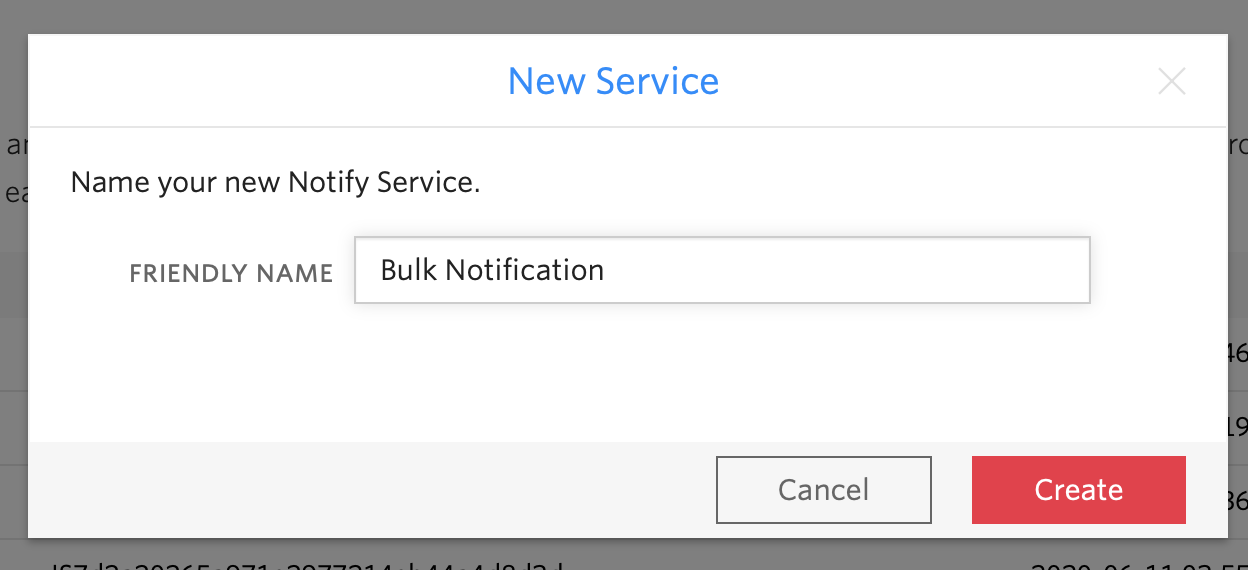
Once redirected to the configuration page, assign the Messaging Service SID via the dropdown and click Save.
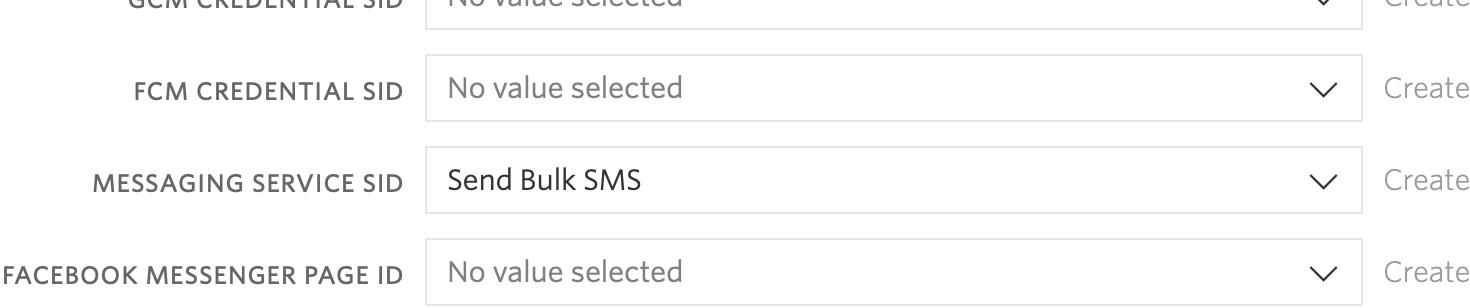
After you have defined the Notify Service an SID will be generated for you. Create a new variable in your .env file called TWILIO_NOTIFY_SID
and assign the value provided on the dashboard.
Return to the send-bulk-sms.php file and create a new variable called $notify_sid
. We will dynamically assign the variable through dotenv.
Now that the dotenv variables have been defined, we will initialize a new Twilio Rest Client and define a variable to record the current time. Copy and paste the following code below the Twilio credentials previously defined.
NOTE: We are also tracking the start time as in the previous post to prove the speed of the Notify Service.
Now declare an array containing key-value pairs of toBinding
and body
.
toBinding
accepts a JSON object containing the binding_type
which in our case is sms
and the address
which is the subscriber's phone number in E.164 format. The following code will show you how to assign multiple recipients.
Lastly, insert the array into the Notify API request to prepare your message to be sent.
At the end of the script we will record the execution time for the Notify request using the following variable:
Measure the Script Execution Time
It’s time to test your script by running the script in your command line:
As with the previous tutorial, I would recommend running this and recording the results at least three times.
It's important to note that even though the request is complete, the delivery time will still run according to the type of sender used. For instance, if you're using a 10-digit or long code phone number, the delivery time is 1 message per second.
How long did it take to send? For me it was an average of .97 seconds!
In the case of this tutorial, sending 1,000 SMS with a long code number will take 16 minutes, but the request itself has completed in under 1 second! This means that your request will almost be seamless in the user experience.
Conclusion
With this knowledge in hand, you can now make more informed decisions about building your communications-based app. It's even possible to increase the execution time by off loading this script via an AJAX request.
I would recommend taking a look at the docs to see how you can extend this with Facebook Messenger or Push with saved subscribers in a database.
Marcus Battle is a Senior Manager of Developer Voices at Twilio where he prompts and rallies developers to build the future of communications. He can be reached via:
- Email: mbattle@twilio.com
- Twitter: @themarcusbattle
- Github: https://github.com/themarcusbattle
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.