Send Custom SMS Notifications for Failed Builds on CircleCI with Twilio
Time to read: 4 minutes
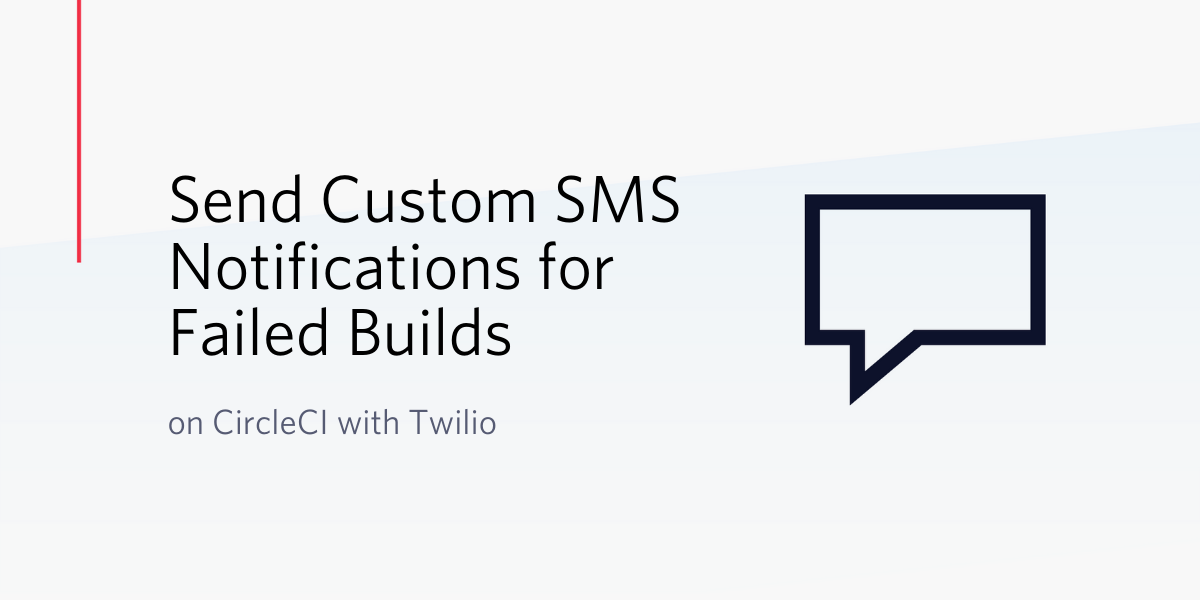
In a previous article, we built a Laravel API project using a test-driven development approach and wrote a couple of tests for all of the endpoints in that project. This approach not only ensures proper implementation of software requirements, but also reassures the developer that methods will operate as expected in production.
In this tutorial, we will extend good programming practices by learning how to set up continuous integration using CircleCI. The goal is to automatically run the tests for our application once we push an update to a code repository such as GitHub. We will take this further by setting up notifications via Twilio SMS. We will write configuration steps to send messages to a specific phone number once the build is successful or fails on the CircleCI pipeline.
At the close of this tutorial, you will have gathered enough knowledge on how to set up automated testing for a Laravel application and how to stay up-to-date with the status of your builds with the help of notifications.
Prerequisites
To get the best out of this tutorial, you will need the following:
- A Twilio Account
- A CircleCI Account
- Composer globally installed to manage dependencies
- A knowledge of building applications with Laravel
- Although not mandatory, going through the previous article will give you an insight into how tests were written for Laravel API endpoints.
Cloning the Starter Repository
To get started, clone the source code from the previously mentioned article by issuing the following command from the terminal:
This will download the project into a folder named laravel-api-testing-sms
. Once the installation process is completed, move to the project directory and use Composer to install all of the dependencies:
Next, create a .env
file and copy the contents of .env.example
file into it. You can run the command below instead of performing this task manually:
Now, generate an application key with:
Great! We are all set. We now have a Laravel API project with several endpoints and tests written for each endpoint using PHPUnit. Again, refer to this article to learn more.
Running the Test Locally
To be certain that all tests are still passing as structured, run the following command from the terminal from the root directory of the downloaded project:
You will see the following output:
Perfect! If your output is similar to the output above then we are on the right track.
Automating the Test
We will now create a configuration file and write the code required to set up continuous integration with CircleCI. To begin, create a folder called .circleci
and a new file named config.yml
within it. Open the new file and use the following content for it:
From the config file above, we pulled a Docker image with the specified PHP version required for our project. We then proceeded to install all of the dependencies, generated an environment key, and finally ran the tests.
Creating a Repository
Create a new repository for this project as it will be required to link it to CircleCI. Proceed to GitHub and create a new repository:
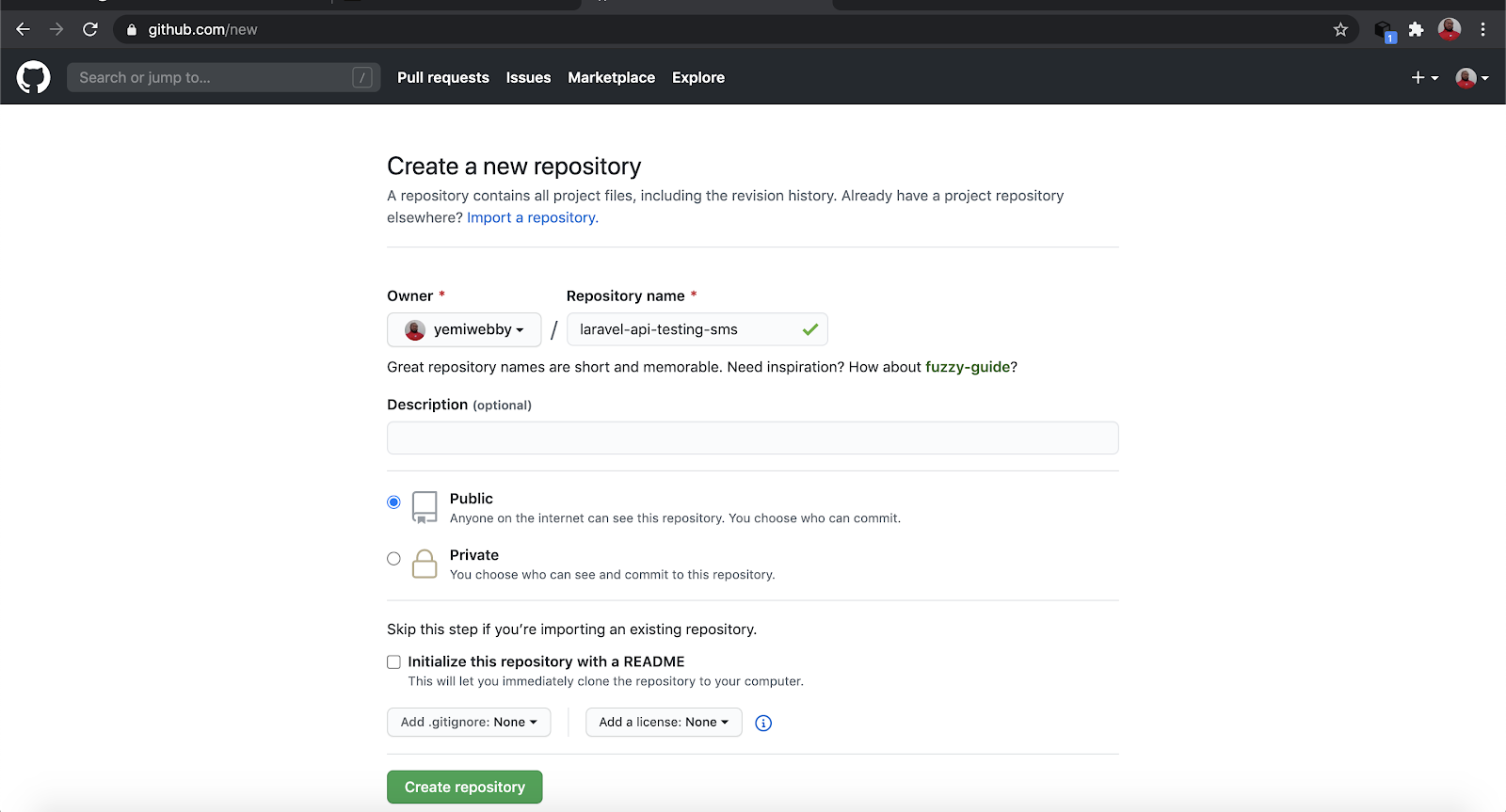
Please note that you can name your repository anything other than laravel-api-testing-sms
. Next, add your code update, set a new remote url and push all code updates to the repo. The following command will suffice:
NOTE: Make sure you replace the URL for git remote set-url orgin
with your repository URL.
Build on CircleCI
Next, we will set up our project on CircleCI. Proceed to the CircleCI website and log in to your account. Search and select your GitHub project from the CircleCI console and click on “Set Up Project”.
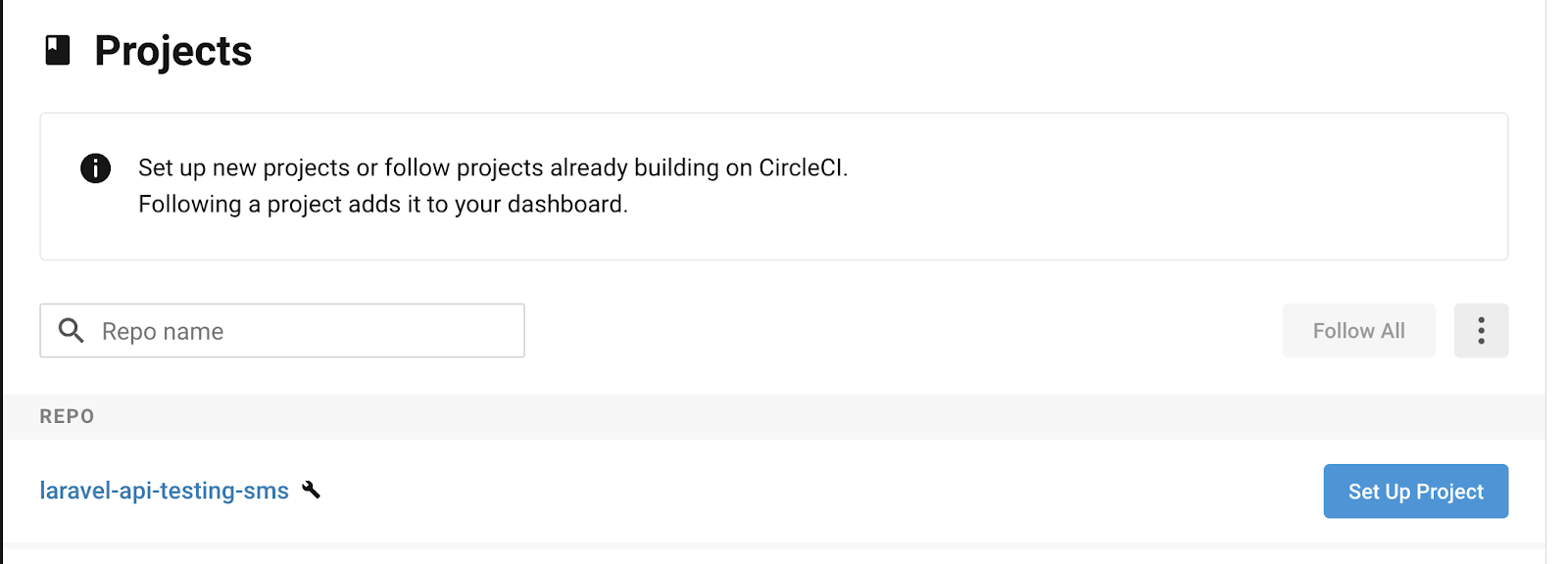
From the next page, click on “Add Config”.
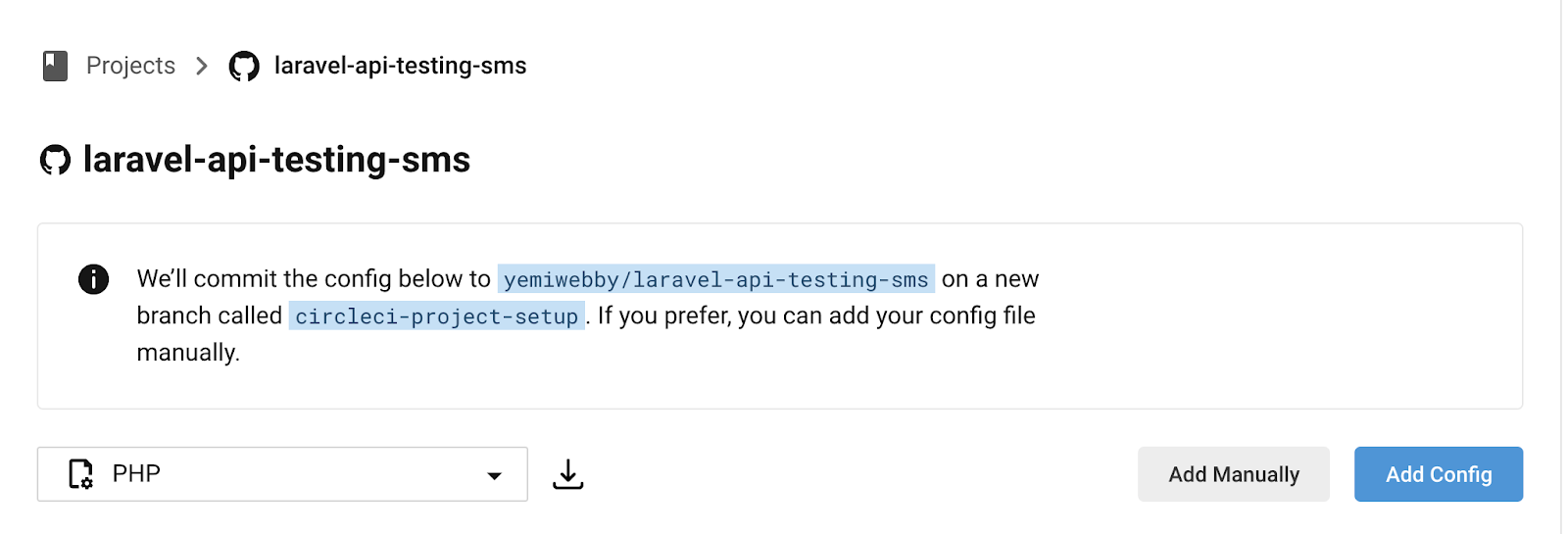
And then, click on “Start Building” from the prompt.
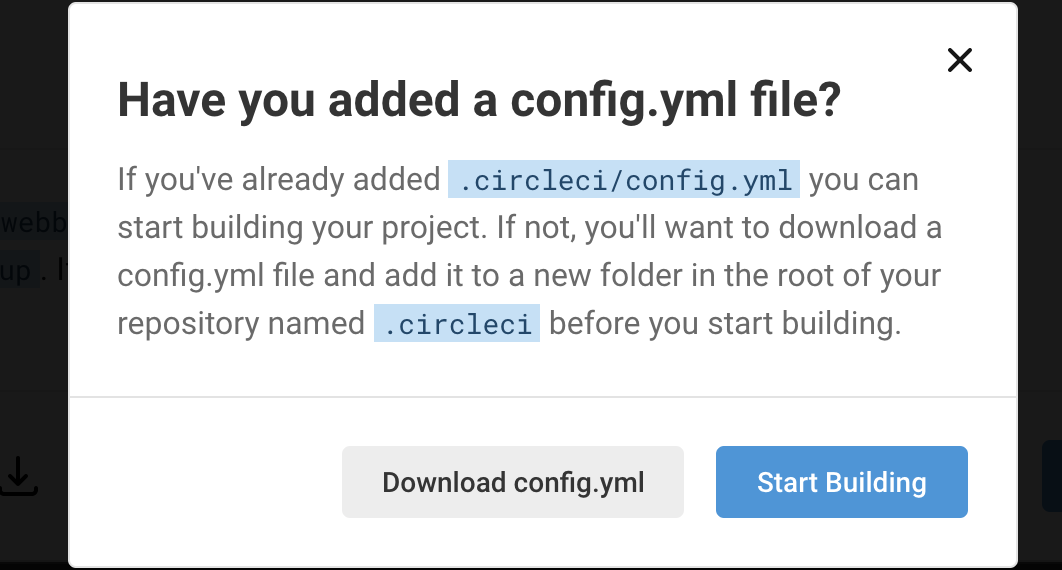
This will immediately trigger the build.

You should get a successful build similar to the image below

Adding Twilio Integration
Now that we have successfully set up our project on CircleCI with our test passing, we will begin integrating Twilio for notification purposes for our CircleCI build.
Here are the important credentials that CircleCI will require to have a proper handshake with Twilio.
TWILIO_FROM
: A Twilio phone number that you own.TWILIO_TO
: The destination phone number for your SMS. Must have a format of+
and country code, also known as E.164 format.TWILIO_SID
: This is your TwilioAccount SID
. It can be obtained from your Twilio account dashboard.TWILIO_TOKEN
: This is your Twilio accountAUTH TOKEN
.body
: A custom message that will be sent to your specified phone number by Twilio.
Back on the CircleCI console click on “Project Settings” and on the next page, click on “Environment Variables” on the side menu.

Next, click on the “Add Variable” button and input your Twilio credentials as shown here.
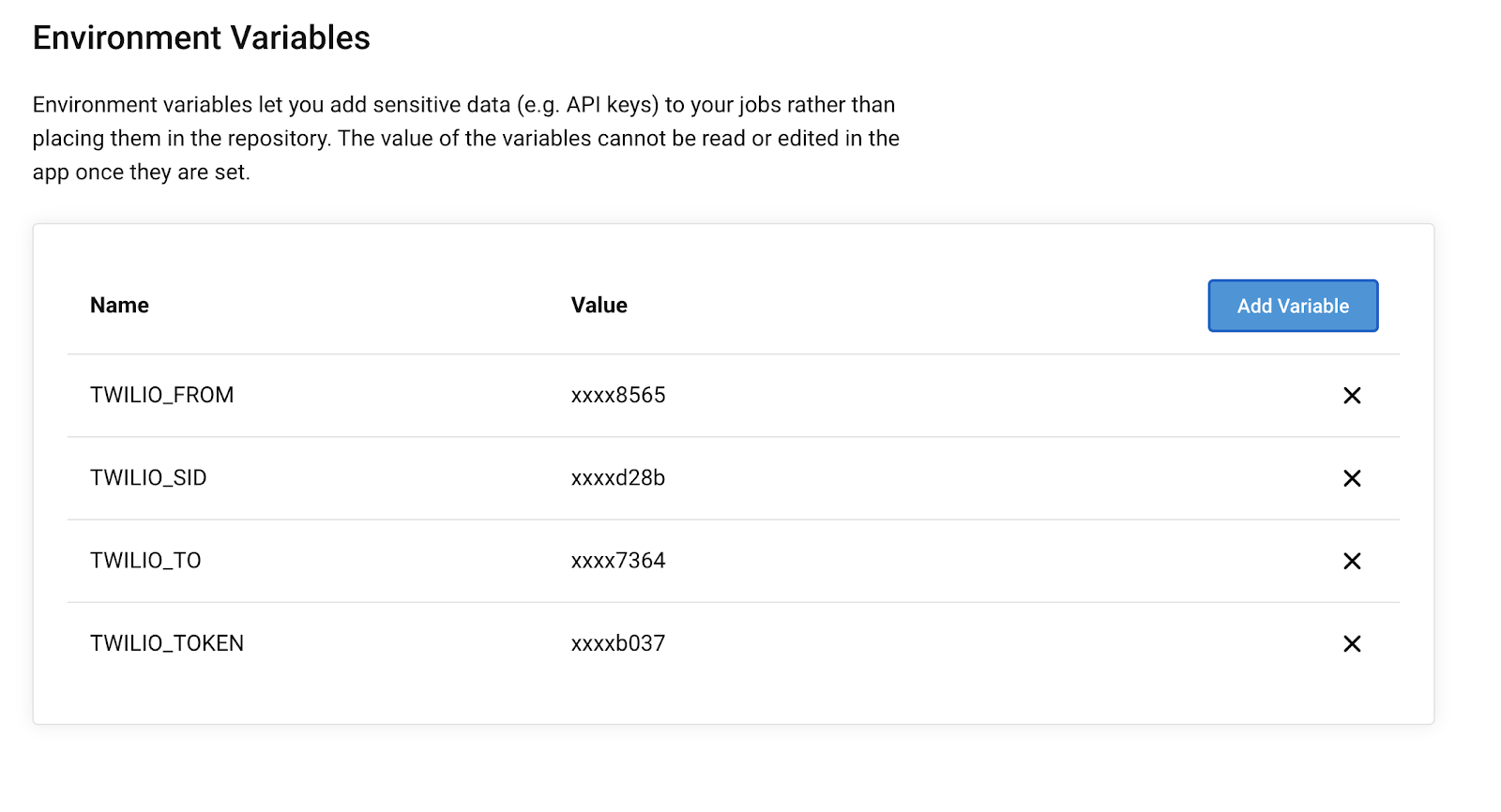
Update Configuration File
CircleCI uses Orbs available in its Orbs registry for almost all integrations with 3rd party applications. In this case, we will make use of a CircleCI Twilio orb created for the purpose of sending custom Twilio notifications through SMS. To achieve that, open .circleci/config.yml
file and update its content as shown here:
We have included a new Orbs section that points to Twilio Orb. Also we included two commands named twilio/sendsms
and twilio/alert
to send an SMS for a successful and failed build respectively.
Test for Failed Build
To test for a failed build, we will intentionally make our test fail by altering the register
endpoints within routes/api.php
as shown here:
Now commit all of the code changes and push to the repository. Of course, the build will fail and you will receive this SMS:
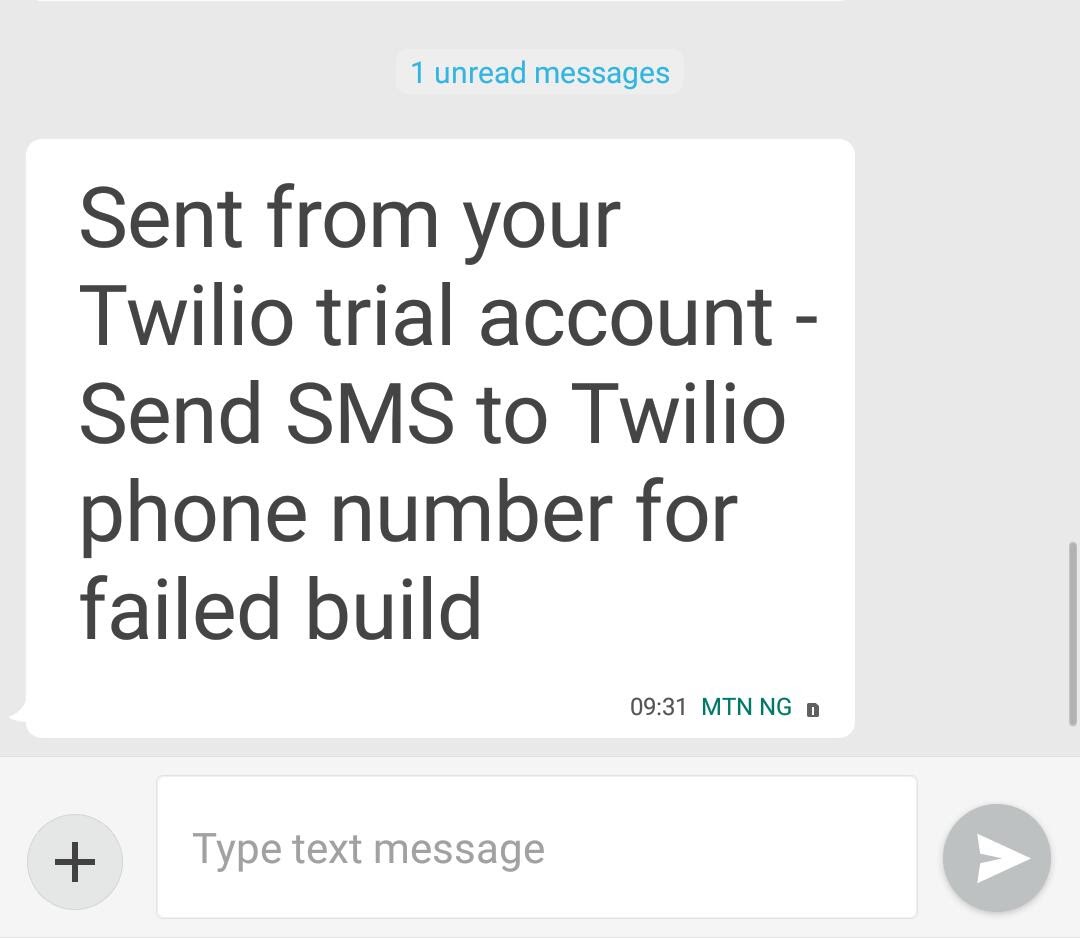
Testing for a Successful Build
Open the routes/api.php
file again and uncomment the register
endpoint:
Commit changes and push the updated code to the repository again.
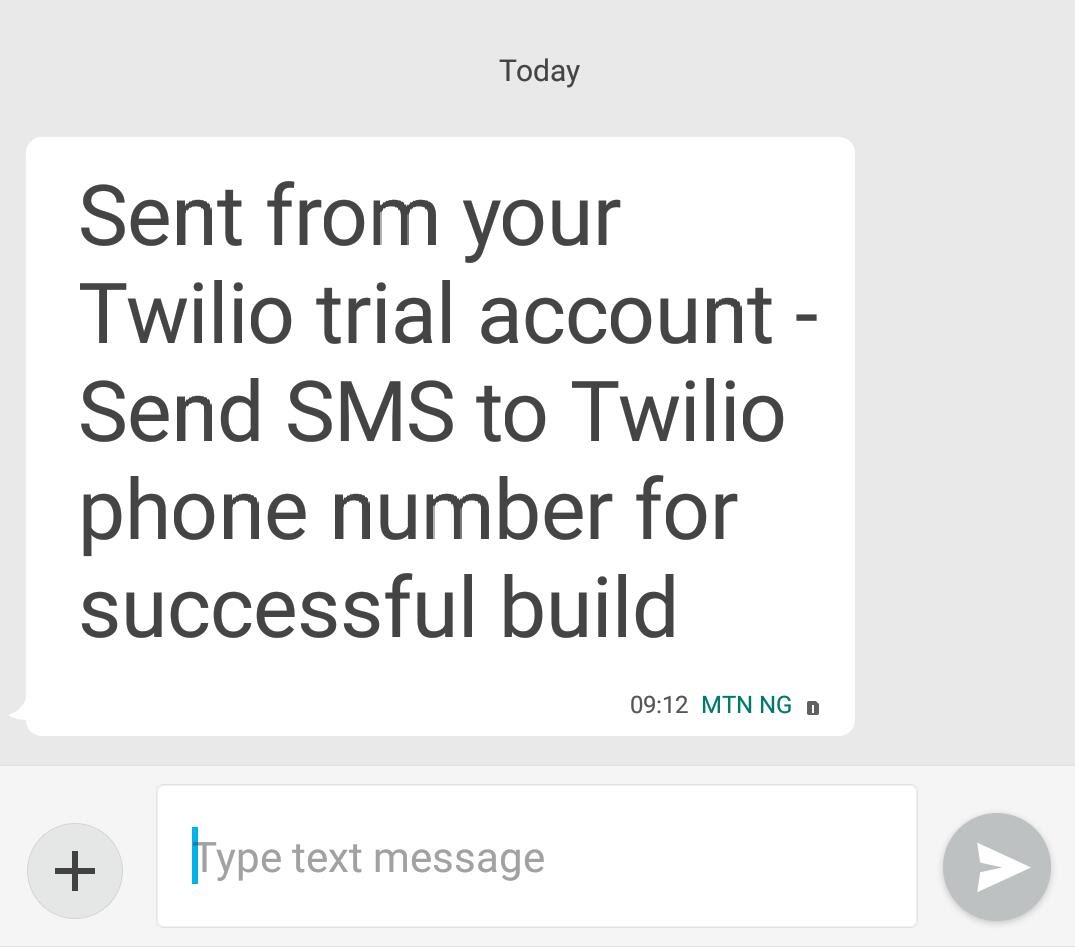
Conclusion
Irrespective of the number of tests written within your projects, being able to keep track of the build status of your pipeline is very important. If you are a project manager or software team lead, the knowledge gained here can help you keep track of the build statuses of your projects.
Download the source code of the project built here from this repository, and feel free to explore its current features by adding more.
Olususi Oluyemi is a tech enthusiast, programming freak, and a web development junkie who loves to embrace new technology.
- Twitter: https://twitter.com/yemiwebby
- GitHub: https://github.com/yemiwebby
- Website: https://yemiwebby.com.ng/
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.