Send an Email Using Twilio SMS in PHP with SendGrid
Time to read: 4 minutes
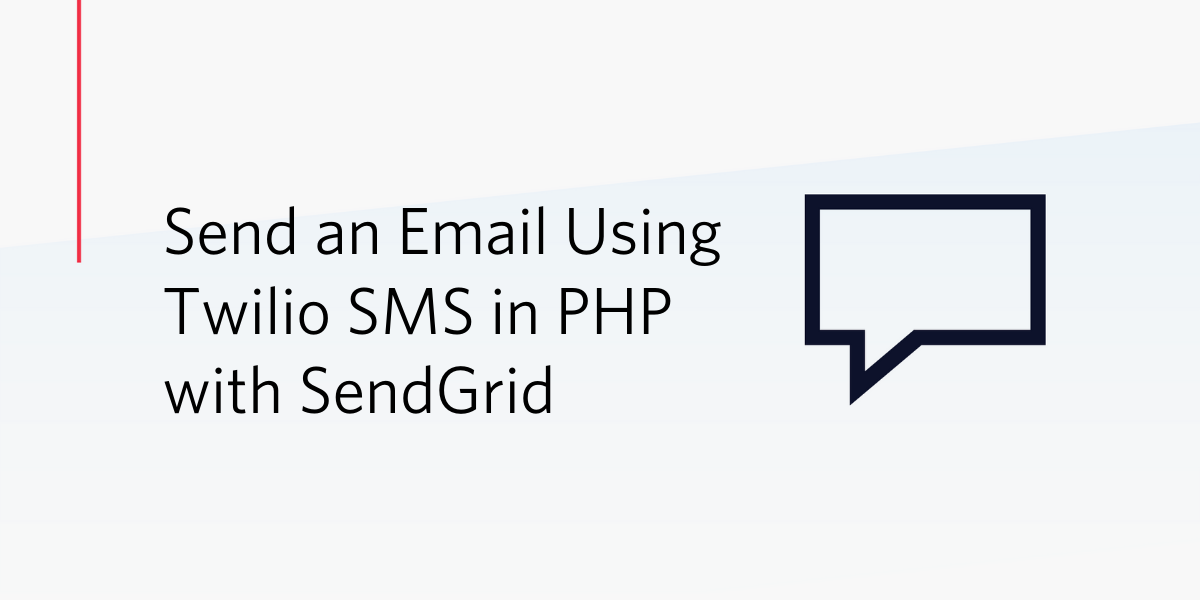
One of the most amazing and forgotten features of SMS is that it works with no internet access. If you’re one of the 3.5 billion people in the world that uses a smartphone, you might not be aware of the millions that don’t use their finger to swipe their screens. India alone accounts for 400 million feature phones still in use in the world.
This might be hard to imagine, but assume you’re in a tight situation and you need to send a quick email and have no internet access. Maybe it’s a job posting you saw or an opportunity that can’t wait until you reach your laptop. It would be helpful if you could still send an email using SMS! In this tutorial, we’re going to learn how to do just that; send email using SMS.
Technical Requirements
Your computer will need to be set up to with the following tools and packages:
- PHP 7 development environment
- Global installation of Composer
- Twilio Account
- Global installation of ngrok
- Sendgrid Account
Set Up Your Development Environment
To start you will need to create a folder in your computer where you will be keeping all the necessary files required to start sending emails using SMS. For this tutorial, I’ll call the folder sms_email
.
After creating this folder, the following empty files will need to be created inside of it:
.env
functions.php
webhook.php
Run the following command below to complete all actions simultaneously:
NOTE: The .env
file is a hidden file used to store secret and private keys on your server. It is not accessible by the browser, so we can safely store API keys or credentials in it and access them from anywhere within our app on the server-side.
Install the Required Packages
To enable the support of the .env
file, you’ll need to install the PHP dotenv package using Composer. Run this command in your terminal inside of the project directory:
The Twilio PHP SDK will now need to be installed in order to connect your project to Twilio’s APIs. Run the following command in your terminal:
Setup Twilio Credentials & Phone Number
If you have not already done so, take a few minutes and head to your Twilio account to create a new number. You will need to get the following information from the Twilio console to send and receive SMS from your project:
- Twilio Account SID
- Twilio Auth Token
- A Phone Number in E.164 format
Once you have located these items, add them to your .env
file.
Setup Twilio SendGrid Credentials for Sending Emails
SendGrid is a cloud-based SMTP provider owned by Twilio that allows you to send email without having to maintain email servers.
Only the SendGrid PHP package and a SendGrid API key are required to enable sending of emails within your application. First you need to generate a SendGrid API key.
Once you have your key, update your .env
as follows:
Now install SendGrid’s PHP package by running the following command in your terminal:
Create an Email Sending Helper Function
You are now going to create a simple helper function that accepts a few parameters, processes them, and sends messages to the recipient using SendGrid.
Below is a simple email sending function using SendGrid. Add the following code to the functions.php
file:
NOTE: The FROM_EMAIL
and FROM_NAME
variables will need to be added to your .env
file using your respective values.
Create a Twilio Webhook
When text messages are sent to your Twilio designated number, Twilio sends a response containing information about your SMS back to your application using a Twilio webhook.
You will need to create your own webhook to receive and process the email sending requests. In the webhook.php
file, copy and paste the following code into it.
If you’re made it this far, congratulations! You’re now only a few steps away from sending emails using SMS.
Setting up the Webhook URL
After setting up your webhook.php
function, you will need to assign a URL to the phone number on your Twilio settings page. From there, each time you send an SMS to your Twilio designated number, Twilio will send data to this URL and then to your functions.php
for processing.
For this tutorial, I am working from my local system and there’s no way to route my local URL to Twilio because my local dev environment is not connected to the internet. That’s where ngrok comes in. Ngrok allows your application to be accessed over the internet.
If you don’t have ngrok set up on your computer, you can see how to do so by following the instructions on their official download page.
In my own case, I’m going to run my PHP application using its built-in server.From the terminal, run the following command to start the server:
Now in a new terminal window, start ngrok by assigning it to the same port as the active PHP server:
Your terminal window should look like this:
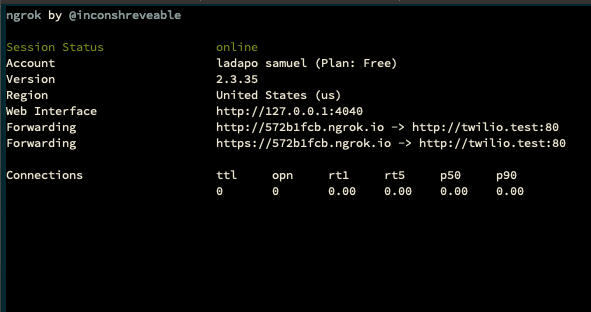
Your Twilio project will use the Forwarding URL provided above, followed by the path to our webhook file. For example, mine is:
The final step is hooking your Twilio number to the webhook we created. Navigate to the active numbers on your dashboard and click on the number you would like to use. In the Messaging section, there is an input labeled “A MESSAGE COMES IN”. Replace the URL with your webhook URL and click “Save”!
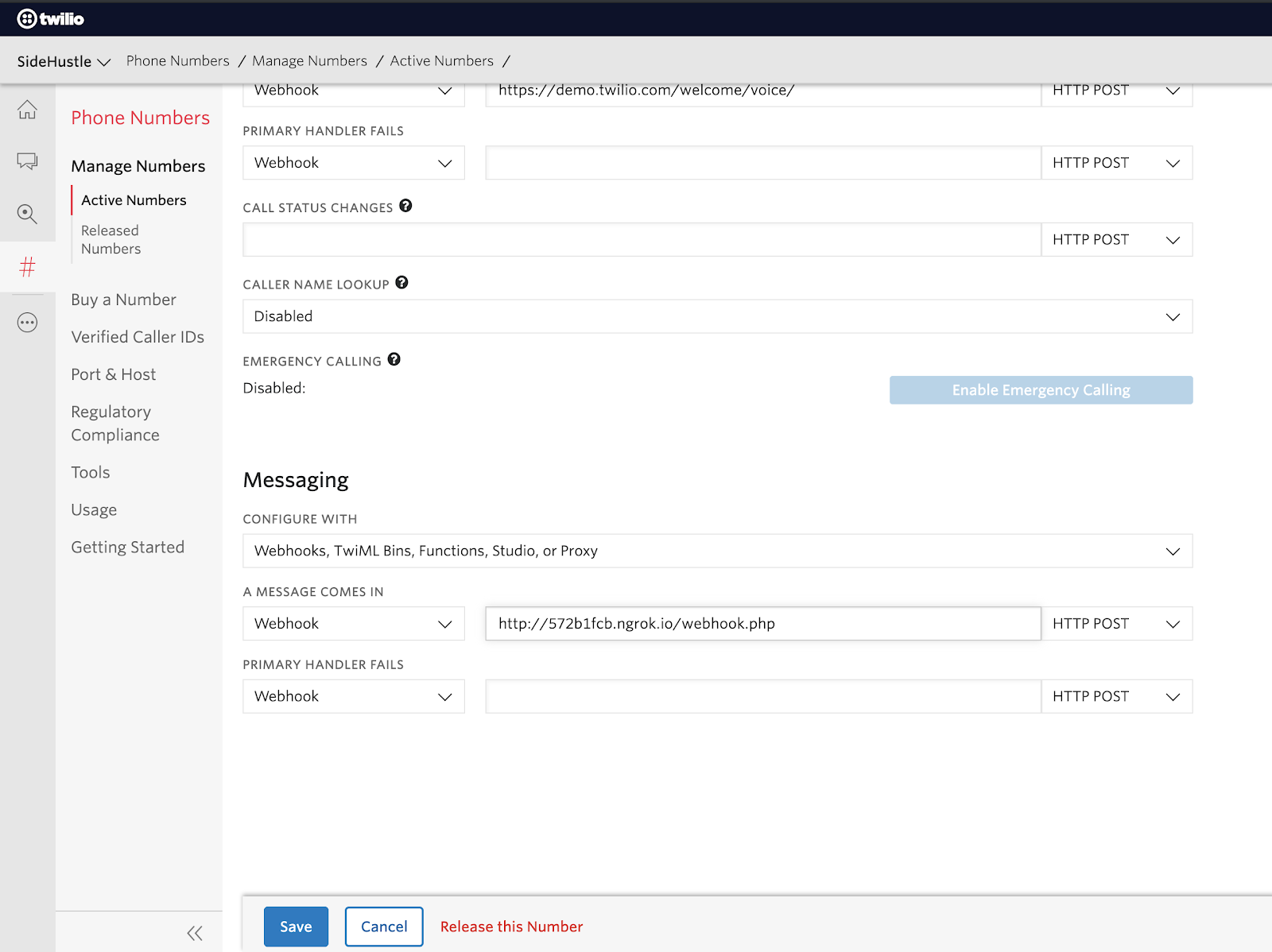
Testing Our App
Send an SMS with the following format to your Twilio Number: TO:ladaposamuel@gmail.com+SUBJ:Hello+MSG:I’m sending this email using Twilio SMS
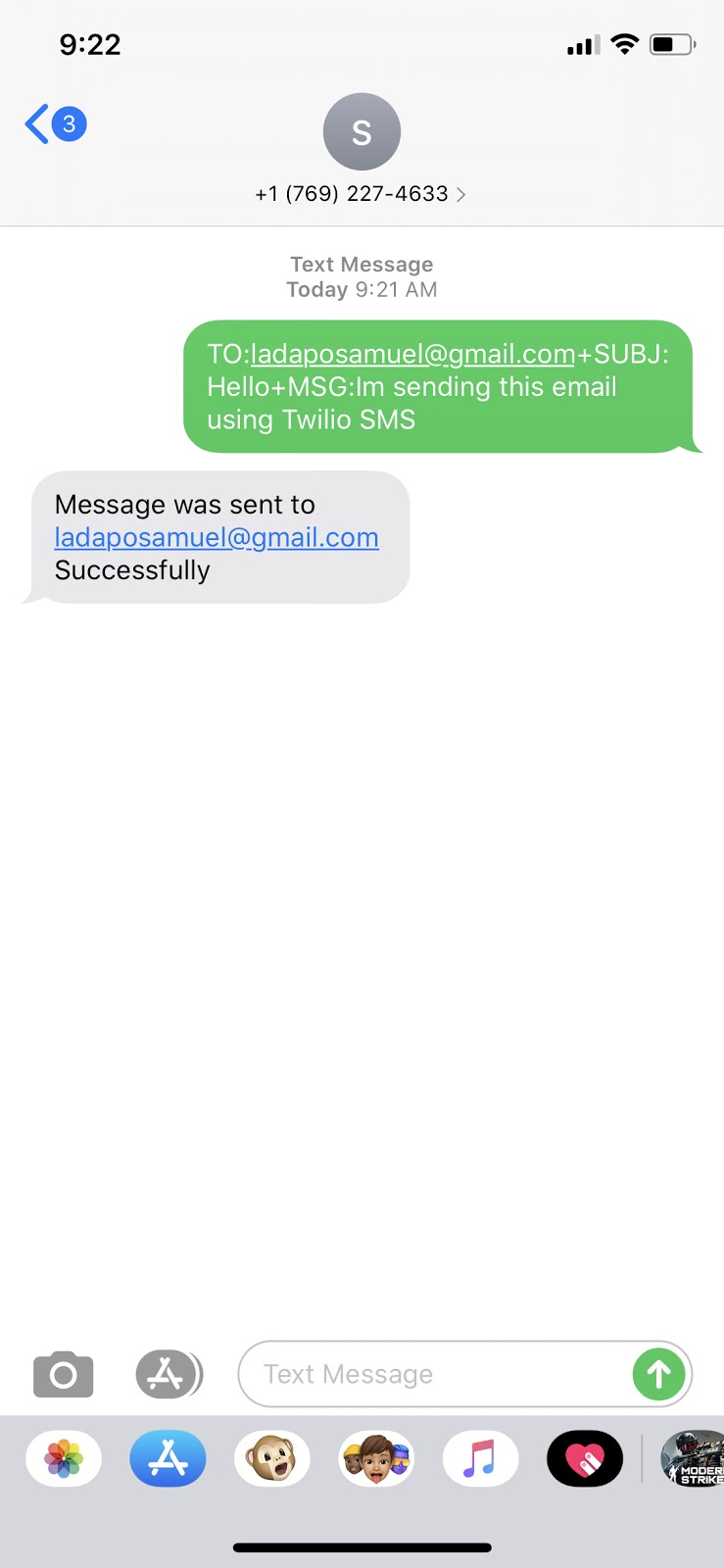
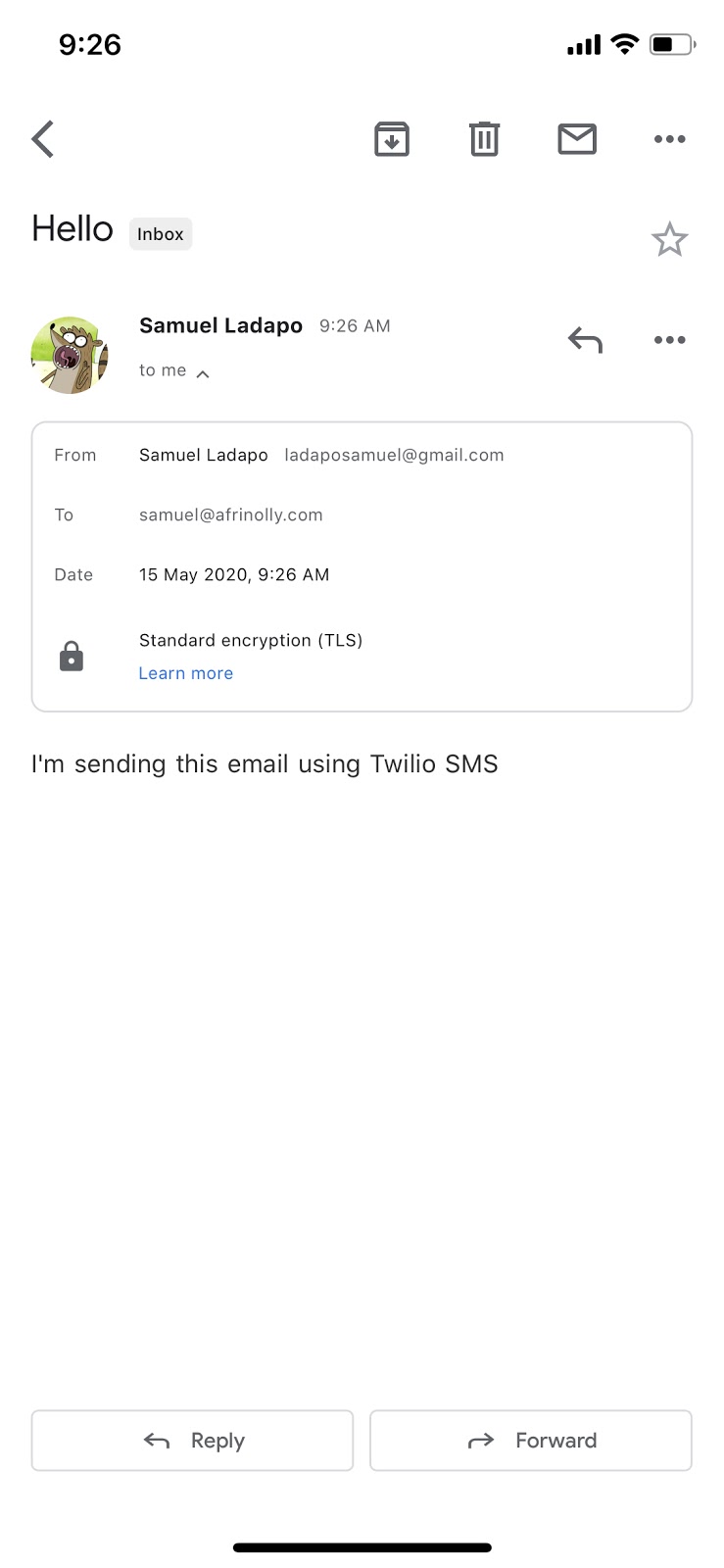
Conclusion
Good job! Now we can send emails using SMS. You can extend the project to be a little bit more fun by making the SMS seem interactive like a chatbot and also including allowing sending to multiple addresses.
Bonus
Github Repo: https://github.com/ladaposamuel/twilio_sms_email
Ladapo Samuel
- Email: ladaposamuel@gmail.com
- Twitter: @samuel2dotun
- Github: ladaposamuel
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.