Ajouter des fonctions vocales customisables n'a jamais été aussi simple
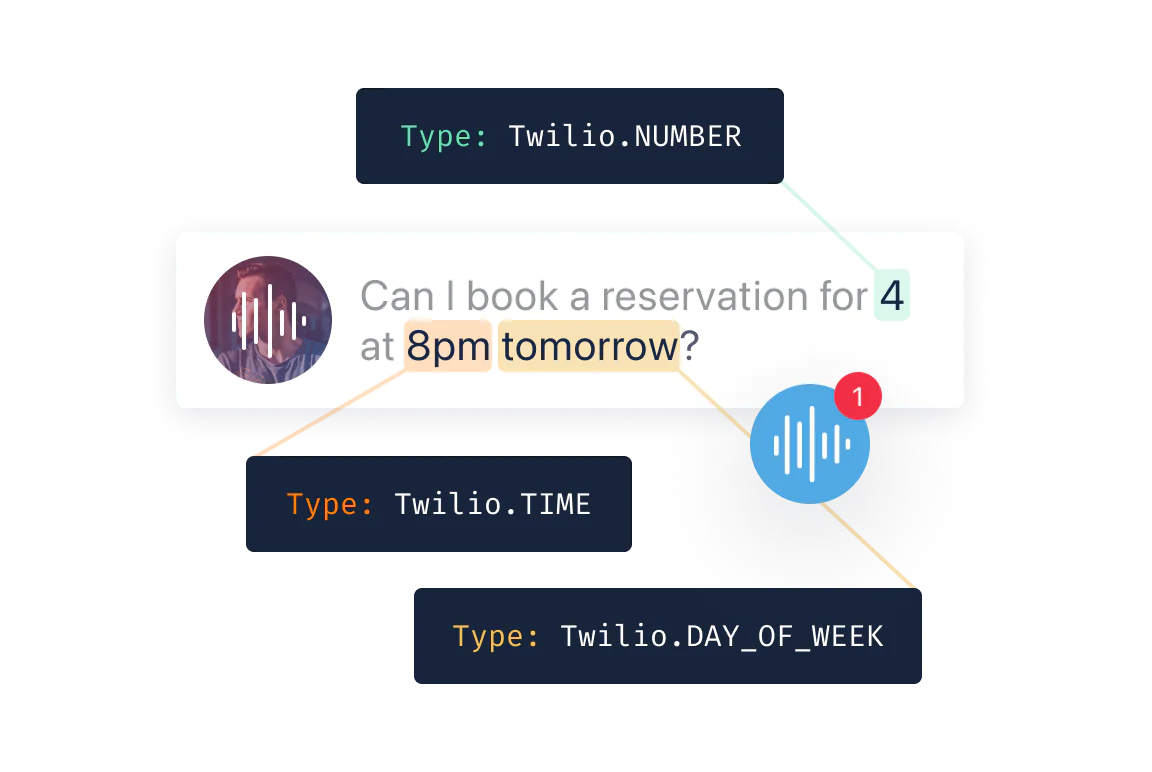
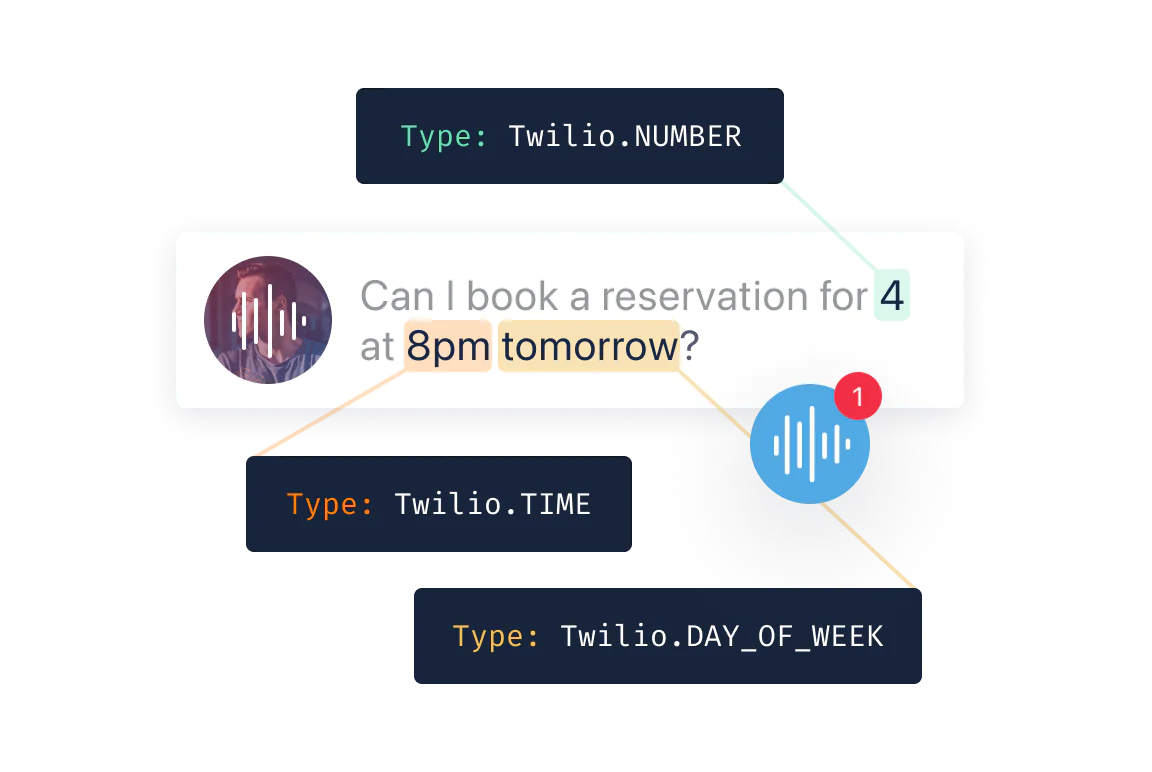
Concentrez-vous sur vos clients, pas sur la création d'une expérience vocale à partir de zéro
Twilio Voice vous fournit les outils nécessaires pour construire des expériences vocales sur mesure visant à satisfaire et dépasser les attentes des clients en constante évolution. Grâce à notre infrastructure de qualité professionnelle, vous pouvez consacrer plus de temps à affiner l'expérience client au lieu de perdre du temps à construire ces expériences à partir de zéro.
Et grâce à nos API innovantes, nos SDK et nos ressources pour les développeurs, vous bénéficiez de toute l'assistance nécessaire pour commencer à construire une application vocale remarquable.