Anpassbare Sprachfunktionen hinzuzufügen war noch nie so einfach
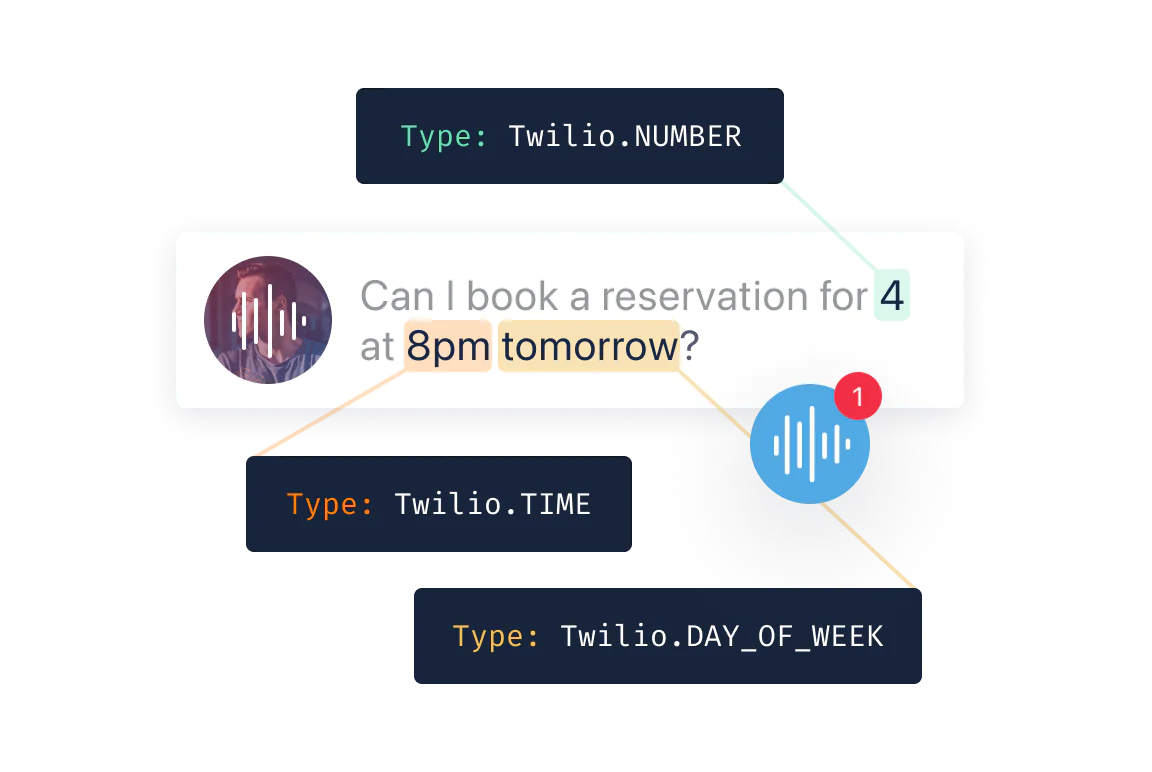
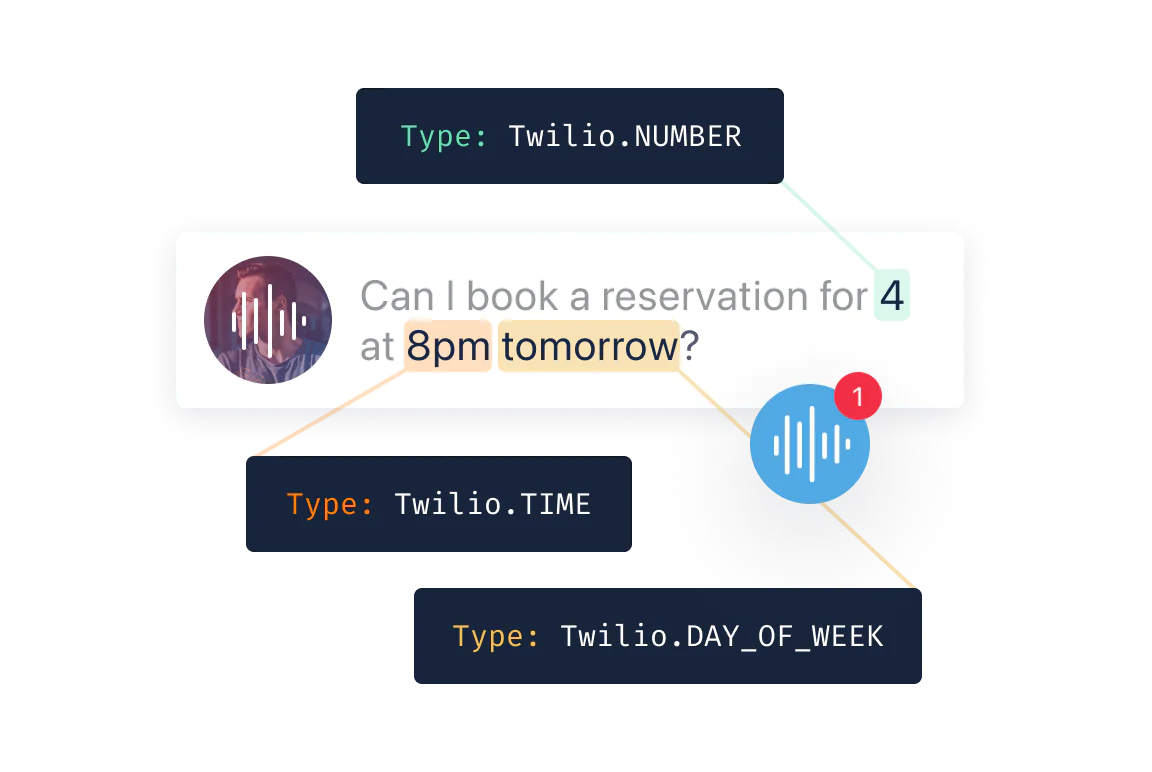
Konzentrieren Sie sich auf Ihre Kunden, nicht auf den Aufbau von Sprachanruffunktionen
Twilio Voice stellt Ihnen die Tools zur Verfügung, die Sie benötigen, um maßgeschneiderte Spracherlebnisse zu schaffen, die die sich ständig ändernden Kundenerwartungen erfüllen und übertreffen. Mit unserer Infrastruktur für Unternehmen können Sie sich ganz auf die Bereitstellung unvergesslicher Kundenerlebnisse konzentrieren und müssen nicht bei Null anzufangen.
Und mit unseren innovativen APIs, SDKs und Entwicklerressourcen haben Sie alles, was Sie brauchen, um eine unvergessliche Sprachanwendung zu entwickeln.