Instant Lead Alerts with C# and ASP.NET MVC
Time to read: 2 minutes
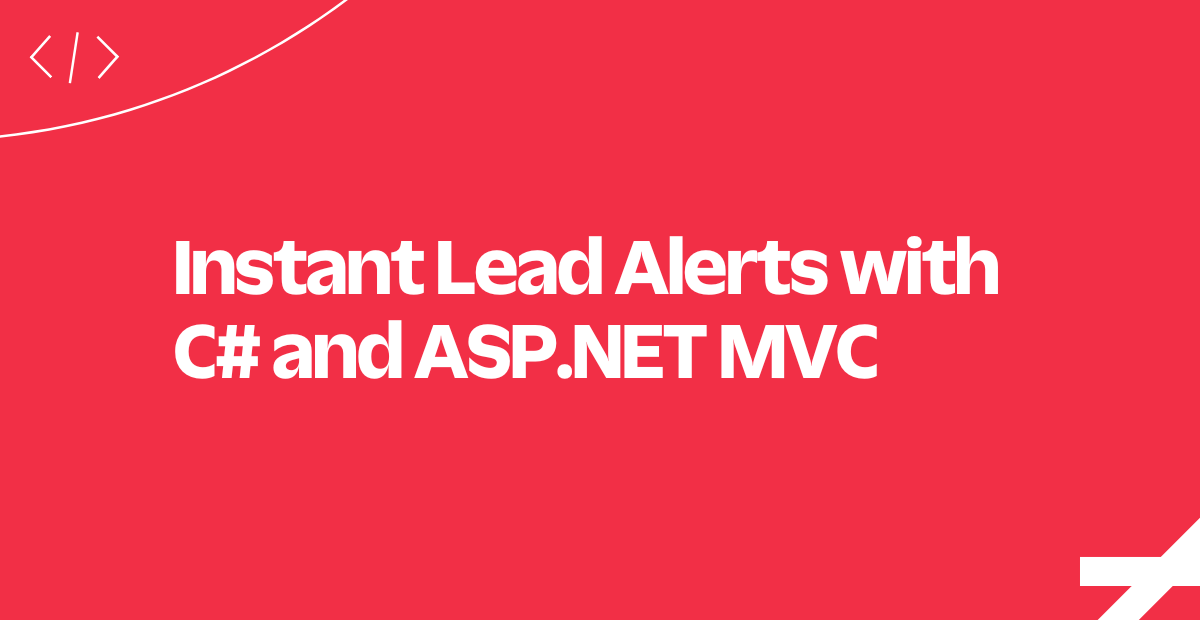
You probably already have landing pages or product detail views in your web application which you're using to generate some excellent leads for your business. Would you like to let the sales team know right away when you've got a new qualified lead?
In this tutorial we'll use Twilio Programmable SMS to send a message when a new lead is found for a C# and ASP.NET MVC application.
In this example, we'll be implementing instant lead alerts for real estate.
We'll create a landing page for a new house on the market and notify a real estate agent when a user requests more information.
Populate Landing Page Data
To display a landing page for our house, we'll first need some data about the fictional house we've put on the market.
For demonstration purposes, we've hard-coded the information we need as you can see in this code sample.
Now that we have the route that will provide our data let's look at how we will render it.
Rendering the Landing Page
In our template, we insert data about the house.
We also put a form into the sidebar so a user can request additional information about the house and provide contact details.
Now that we have rendered our listing let's see how to setup a Twilio REST Client to send messages.
Create a Twilio REST API Client
Here we create a helper class with an authenticated Twilio REST API client that we can use anytime we need to send a text message.
We initialize it with our Twilio account credentials in our Local.config
file. You can find them in the console:
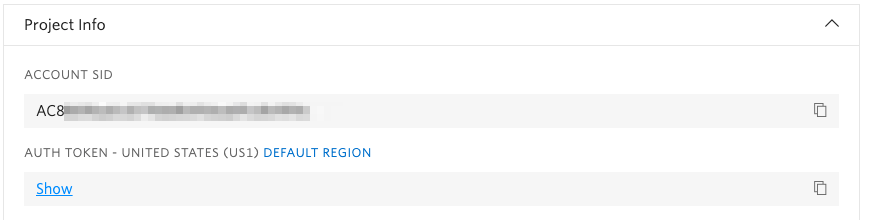
Credentials and PhoneNumbers are just a thin wrapper for values from Local.config
. Here's how it might look:
public static string AccountSID
{
get
{
return
WebConfigurationManager.AppSettings["TwilioAccountSid"];
}
}
Now that our Twilio REST Client is ready, let's see how we handle a new lead.
Handle a POST Request
This code handles the HTTP POST
request from our landing page when our customer fills out a form.
It uses our MessageSender
class to send a SMS message to the real estate agent's phone number, which is set as an environment variable.
We include the lead's name, phone number, and inquiry directly in the body of the text message we send to the agent.
That's it! Now the agent has all the information they need to immediately follow up on the lead.
We've just implemented an application to instantly route leads to a salesperson using text messages. Let's look at some other possible features for your application on the next slide.
Where to Next?
There's a lot you can build easily with .NET and Twilio! Here are just a couple ideas for what to do next:
Twilio Client allows your website users to make and receive phone calls directly from their browsers.
Call Tracking helps you measure the effectiveness of different marketing campaigns.
Did this help?
Thanks for checking out this tutorial! Let us know what you've built - or what you're building - on Twitter.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.