SMS and MMS Notifications with C# and ASP.NET MVC
Time to read: 2 minutes
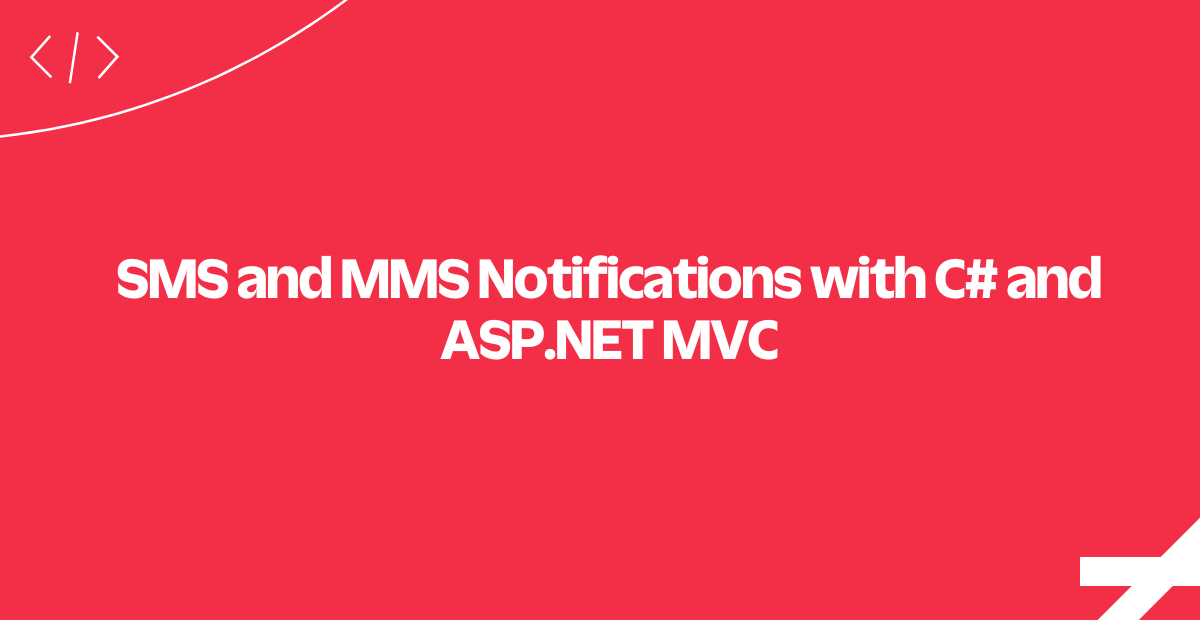
Need to hail your server administrators when something goes wrong with your servers? We've got you covered - today we'll cover adding notifications on server exceptions to your C# ASP.NET MVC application. We'll cover all the key plumbing to make it happen!
Why wait? Click the button below to move to the next step of the tutorial.
List The Server Administrators - and Whomever Else to Contact
Here we create a list of administrators who should be notified if a server error occurs. The only essential piece of data we need is a PhoneNumber
for each administrator.
With this list in hand, let's configure the Twilio REST client and see how we use it to send a notification on application exceptions.
Configure the Twilio C# REST Client
To send a message, we'll need to initialize the Twilio C# Helper Library. This requires reading our TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
defined at ServerNotifications.Web/Web.config
Now that we have our Twilio Client setup, let's see how to handle exceptions.
Handle All C# Application Exceptions
In an ASP.NET MVC application, we can handle errors at the application level. Note that any other exceptions caught in the code (using your own try/catch blocks) are not being captured and processed here.
Any uncaught exception will be handled here. Let's see how we can use this exception to hail our administrator list.
Creating a Custom Alert Message
Here we create an alert message to send out via text message. You might also decide to include a picture with your alert message... maybe a screenshot of the application when the crash happened? A meme to calm everyone down?
The error handling is setup to capture application wide errors and forward them to all the lucky administrators.
Let’s take a closer look at how the Notifier forwards these errors to the administrators.
Read the Administrators from the CSV File
With CSVReader
, we can open and parse our list of administrators from our CSV file. We use the method GetRecords
to read all the records.
We've seen how to load a list of administrators from a file. Now let's see how to send a message to each of them.
Send a Text Message Blast
There are the three parameters needed to send an SMS using the Twilio REST API: From
, To
, and Body
.
US and Canadian phone numbers can also send an image with the message. (Other countries can as well, but a shortened URL pointing to the image will be appended).
That's it! We've just implemented an automated server notification system that can send out C# ASP.NET MVC server alerts if anything goes wrong.
Let's see some other fine features you can add to your application, next.
Where to Next?
Twilio and C# are great together! Here are two more ideas for useful features:
Increase the security of your login system by verifying a user's mobile phone in addition to their password.
Send your customers a text message when they have an upcoming appointment, this tutorial shows you how to do it from a background job.
Did this Help?
Thanks for checking out this tutorial! Tweet us @twilio to let us know what you've built... and what you're building.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.