How to Send SMS Text Messages with AWS Lambda and Python 3.6
Time to read: 5 minutes
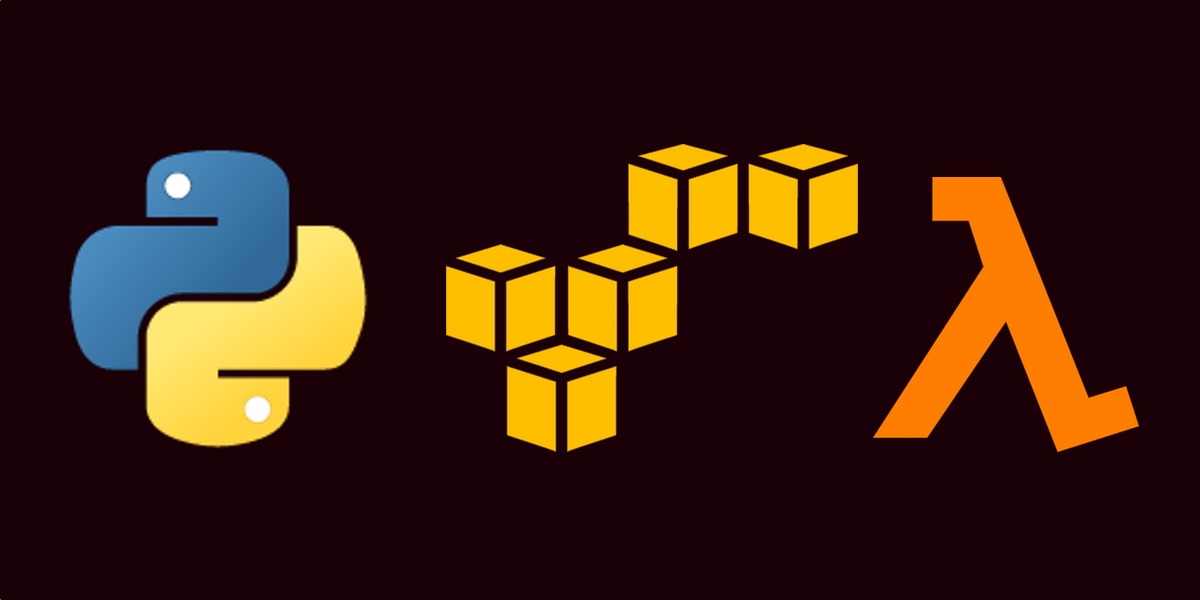
Amazon Web Services (AWS) Lambda is a usage-based service that can run arbitrary Python 3.6 code in response to developer-defined events. For example, if a new JPEG file is uploaded to AWS S3 then AWS Lambda can execute Python code to respond to resize the image on S3.
Let’s learn how to use AWS Lambda with a manual test event trigger to send outbound text messages via a Python Lambda function that calls the Twilio SMS REST API.
Getting Started with AWS Lambda
Sign up for a new Amazon Web Services account, which provides a free tier for new users, or sign into your existing AWS account.
After you sign up go to the main Console page that looks like the following screen.
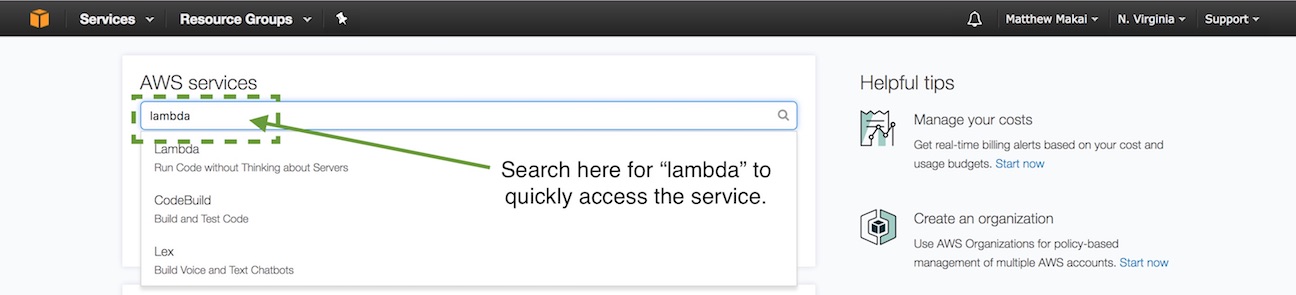
As shown in the screenshot, use the search box with the text “lambda” to find Lambda on the list of services. Click on Lambda to get to the main AWS Lambda page.
Click the “Create a Lambda function” button. The “Select Blueprint” page will appear.
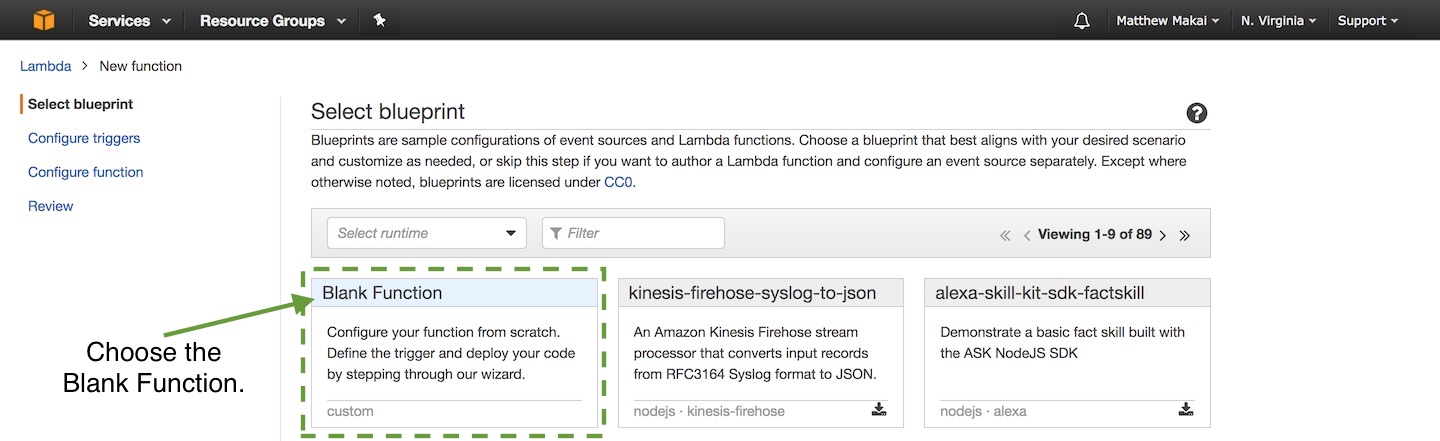
Click on the “Blank Function” option to advanced to the “Configure triggers” page. A trigger fires and runs the Lambda function when a developer-defined event happens. I found it confusing at first that the trigger is optional and you don’t actually need to configure a trigger to move to the next step. In our case we’ll test the Lambda function manually after we’ve set it up so click the “Next” button to move on.
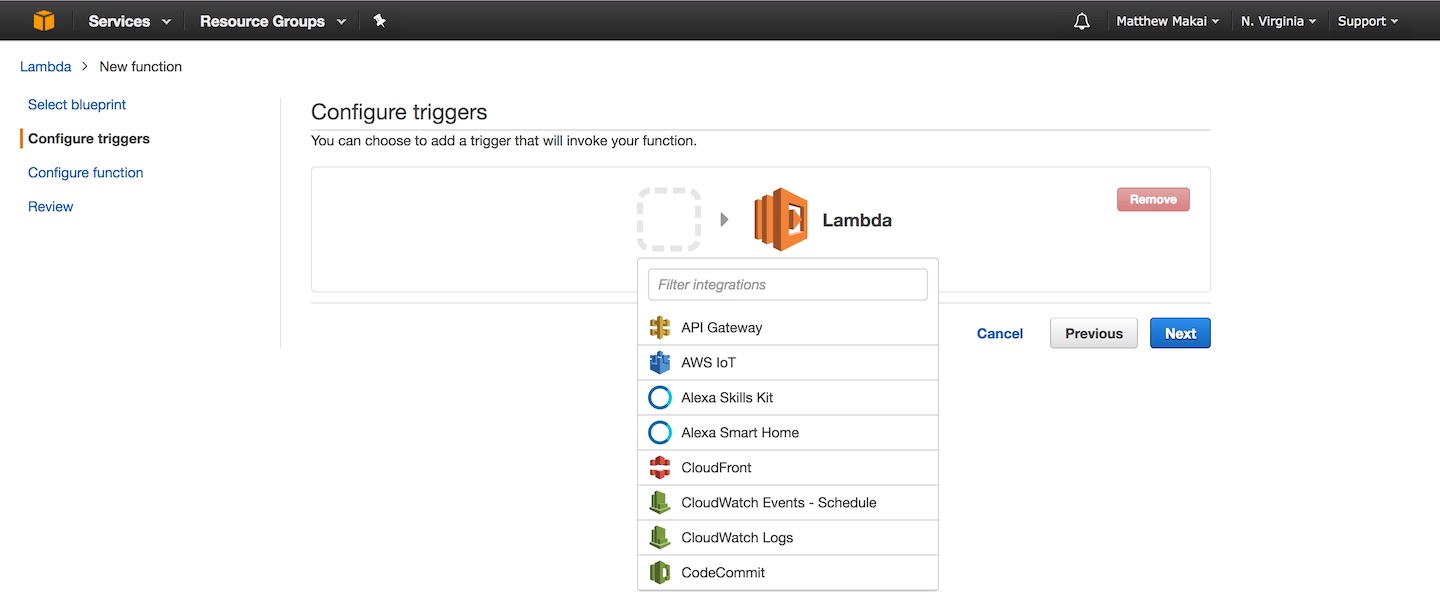
The “Configure function” screen appears next where we can write some code.
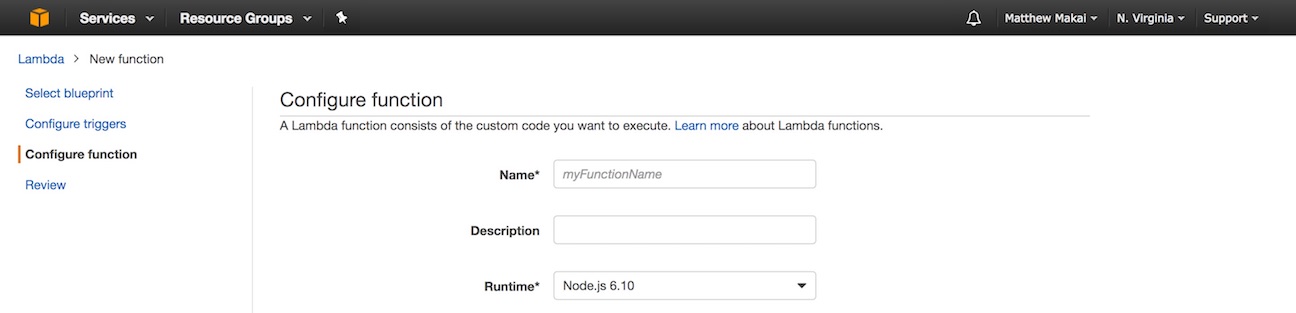
Preparing the Lambda Function
Enter a “sendsms” as the name of the Lambda function and “Send an SMS text message to a phone.” for the description field. In the Runtime drop-down, select Python 3.6 for the programming language.
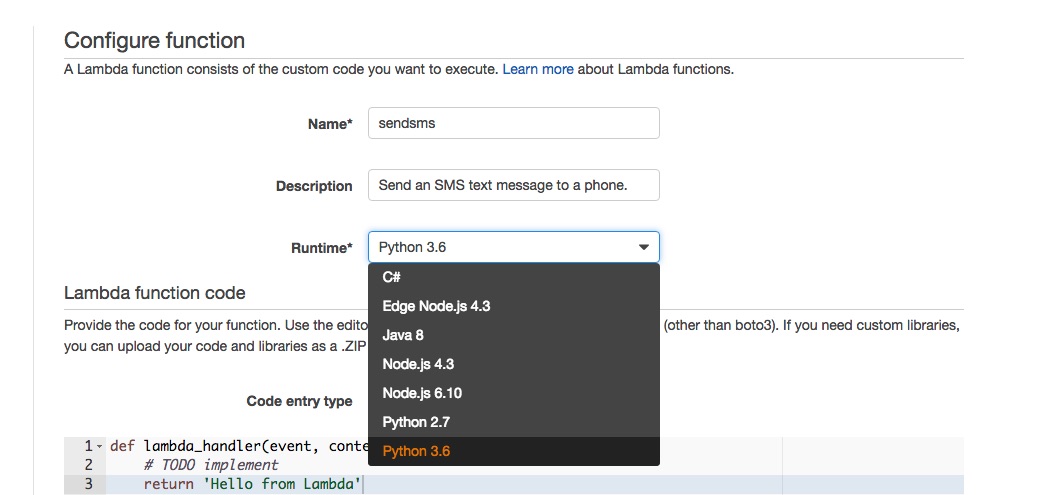
Below the Runtime drop-down there is a large text box for code, pre-populated with a stub
lambda_handler
function. The “Code entry type” drop-down can be changed to upload a ZIP file but for our short function we will stick to the “Edit code inline” option. Replace the default function in the text box and paste in the following code.
The above code defines the lambda_handler
function, which AWS invokes to execute your code. The Python code expects two environment variables that are read by the os
module with the os.environ.get
function.
Within the lambda_handler
function we check that all values have been set properly, otherwise we exit with a readable error message. If the appropriate values are in place we populate the URL with the user’s Twilio Account SID as specified in the API reference documentation. We then use Python 3’s urllib standard library functions to perform an API request to Twilio. If Twilio returns the expected HTTP 201 response there will not be an exception so we return from the function with the “SMS sent successfully!” value.
Below the code input area there is a section to set environment variable key-value pairs. Enter two environment variable keys named TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
.
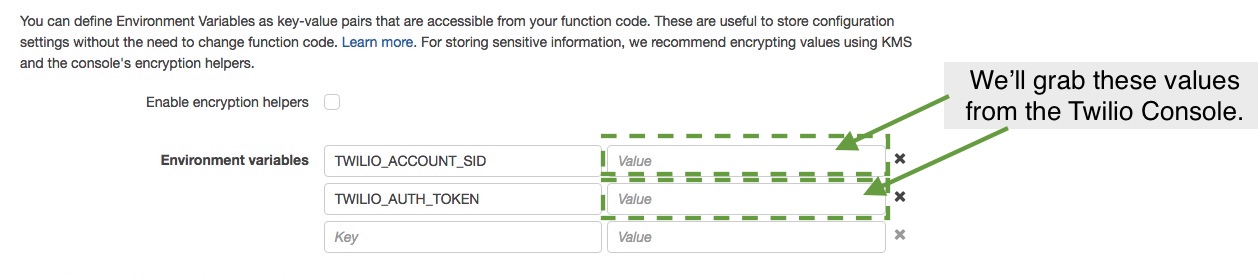
Head to the Try Twilio page and sign up for a free Twilio account so that we can grab Twilio credentials for populating the environment variable values.
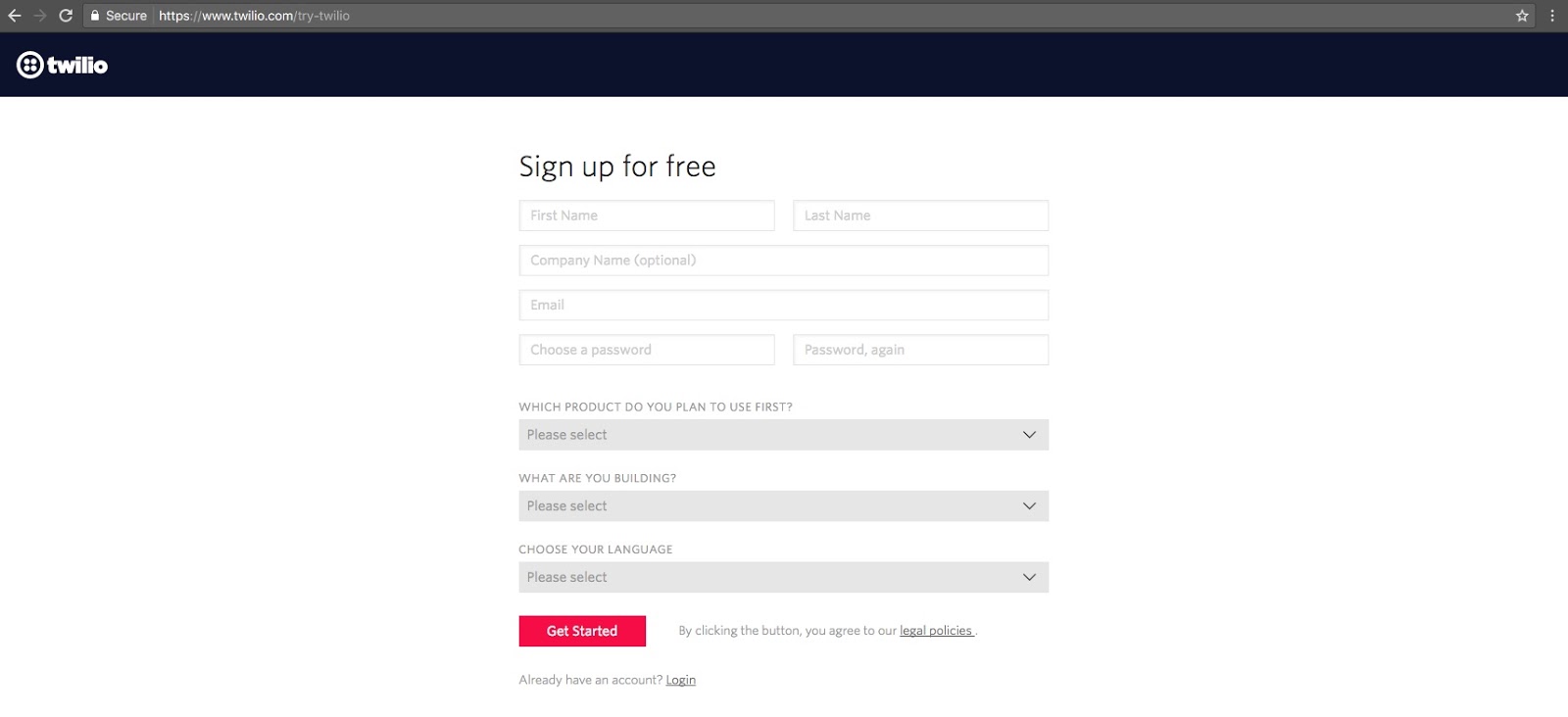
After signing up, you’ll get to the main Twilio Console where you can grab your Account SID and Auth Token. Copy the values and paste them into the environment variable values on the AWS Lambda configuration page.
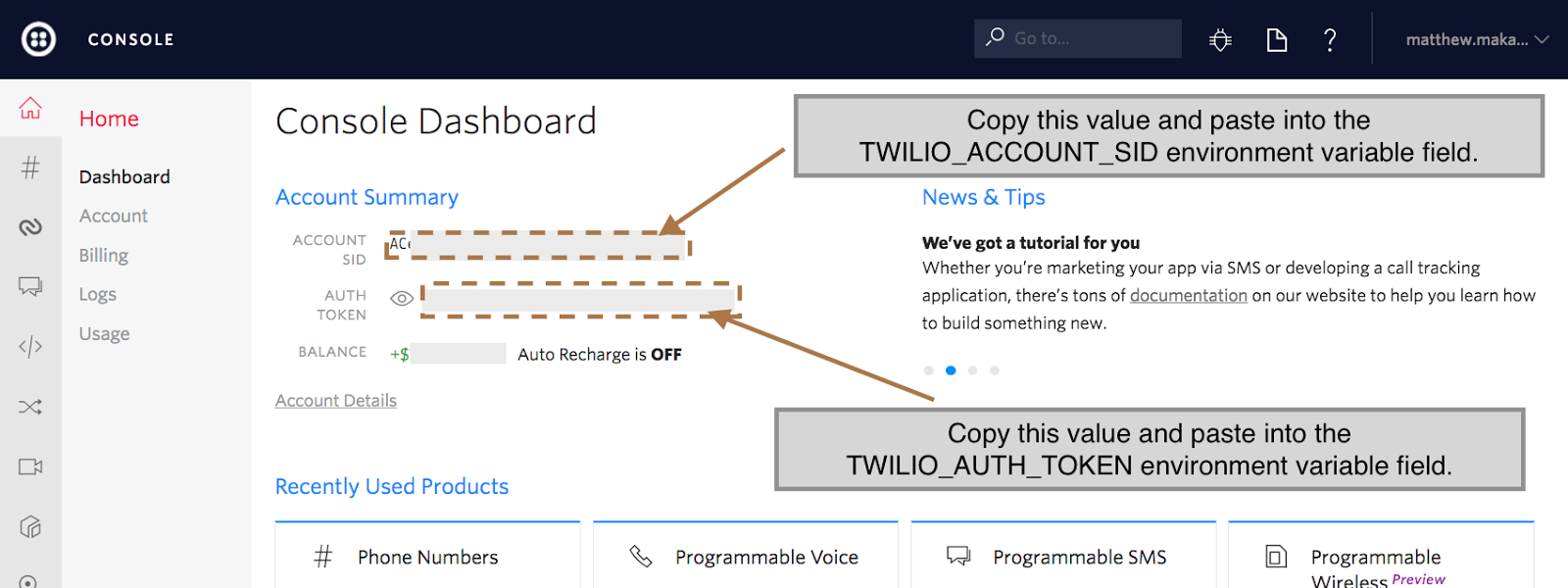
The Python 3.6 code and the environment variables are now in place. We just need to handle a few more AWS-specific settings before we can test the Lambda function. Scroll past the environment variables to the “Lambda function handler and role” section, which contains a few more required function configuration items.
Keep the default handler set to lambda_function.lambda_handler
. Select “Create a new Role from template(s)” from the drop-down then for the “Role name” field enter “dynamodb_access”. Under “Policy templates” select the “Simple Microservice permissions”.
The “Simple Microservice permissions” allows our Lambda to access AWS DynamoDB. We will not use DynamoDB in this tutorial but the service is commonly used either as permanent or temporary storage for Lambda functions.
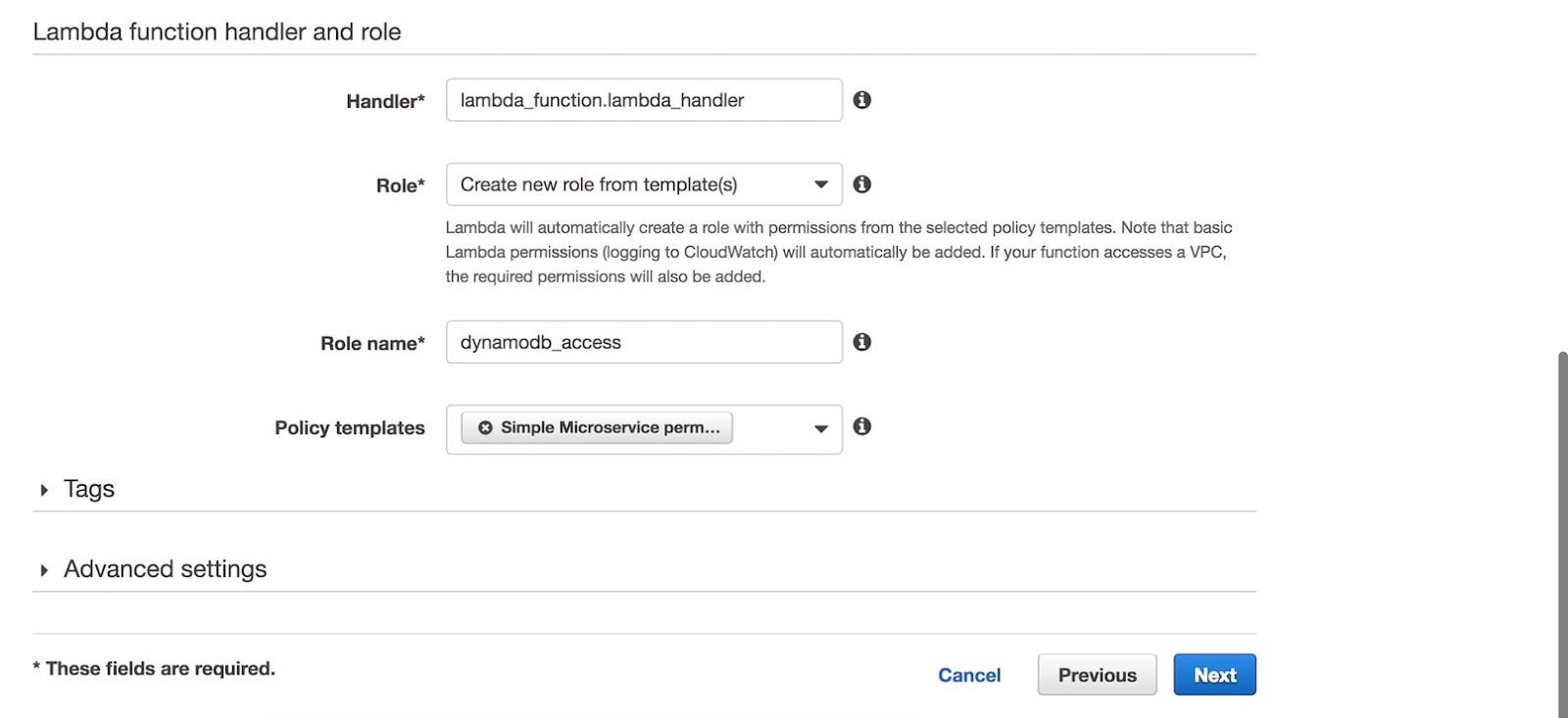
Our code and configuration is in place so click the “Next” button at the bottom right corner of the page.
Testing the Lambda Function
The next screen is to review the settings you just configured. Glance through the name, environment variables and other fields to make sure you didn’t typo anything.
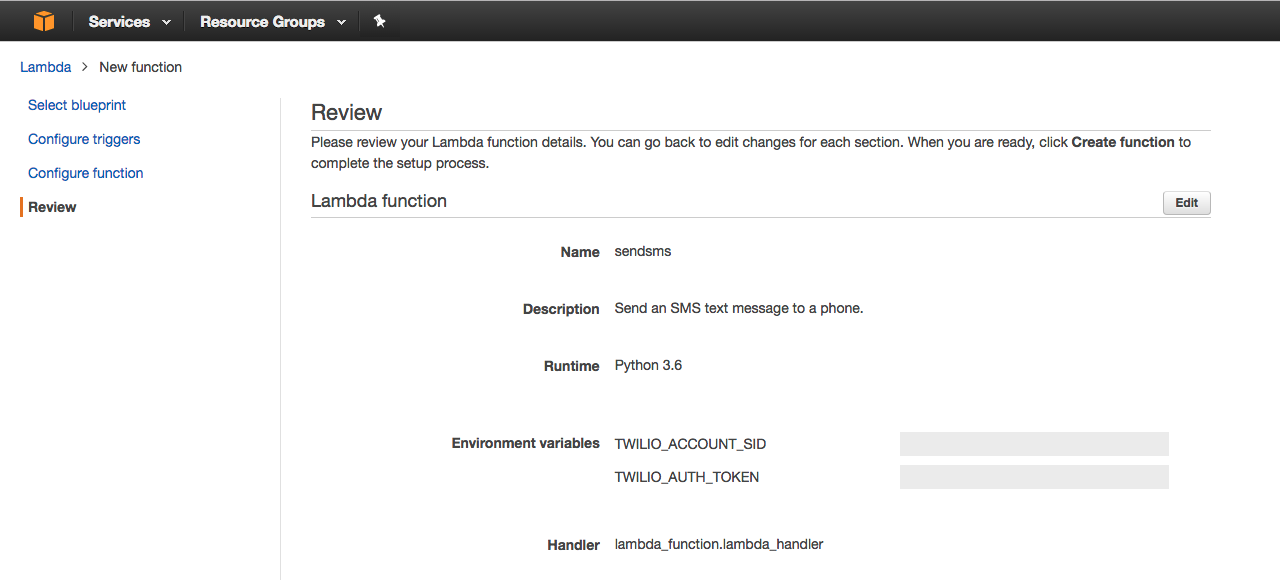
Scroll down press “Create function”.
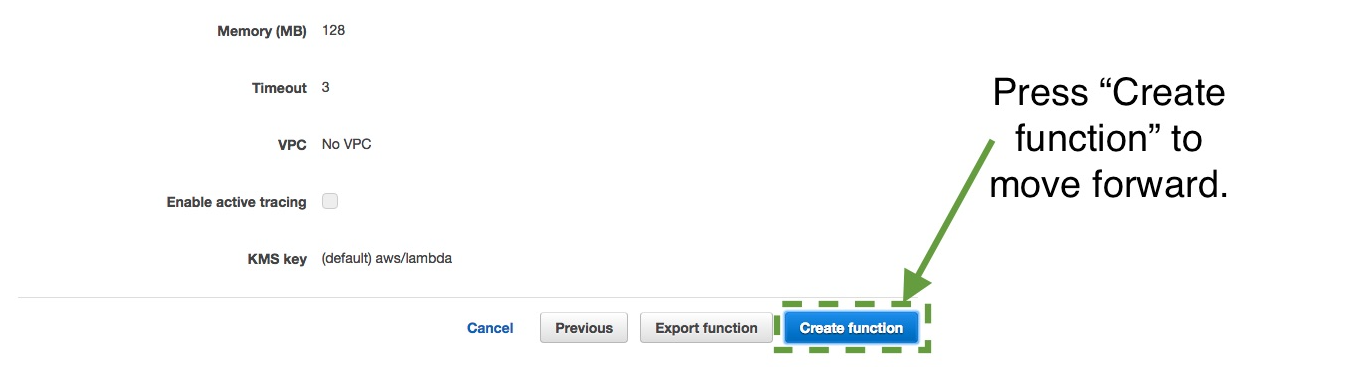
A success message will appear on the next page below the “Test” button.
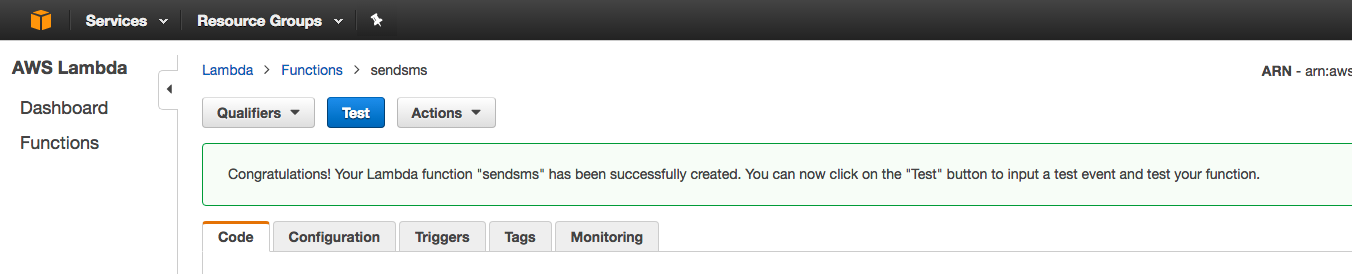
Click the “Test” button to execute the Lambda. Lambda will prompt us for some data to simulate an event that would kick off our function. Select the “Hello World” sample event template, which contains some default key that we will replace. Modify the template so you have the following input test event keys:
"To": "recipient phone number"
– the number in the format “+12023351493” that you want to send a text message to. If you have a trial Twilio account this should be your verified phone number. If your Twilio account is upgraded then this can be any phone number."From": "twilio phone number"
– the number in the format “+19732644156” that you own on Twilio, which will be used to send the outbound SMS"Body": "Hello from AWS Lambda and Twilio!"
– the message you want to send from the Twilio number to the recipient phone number
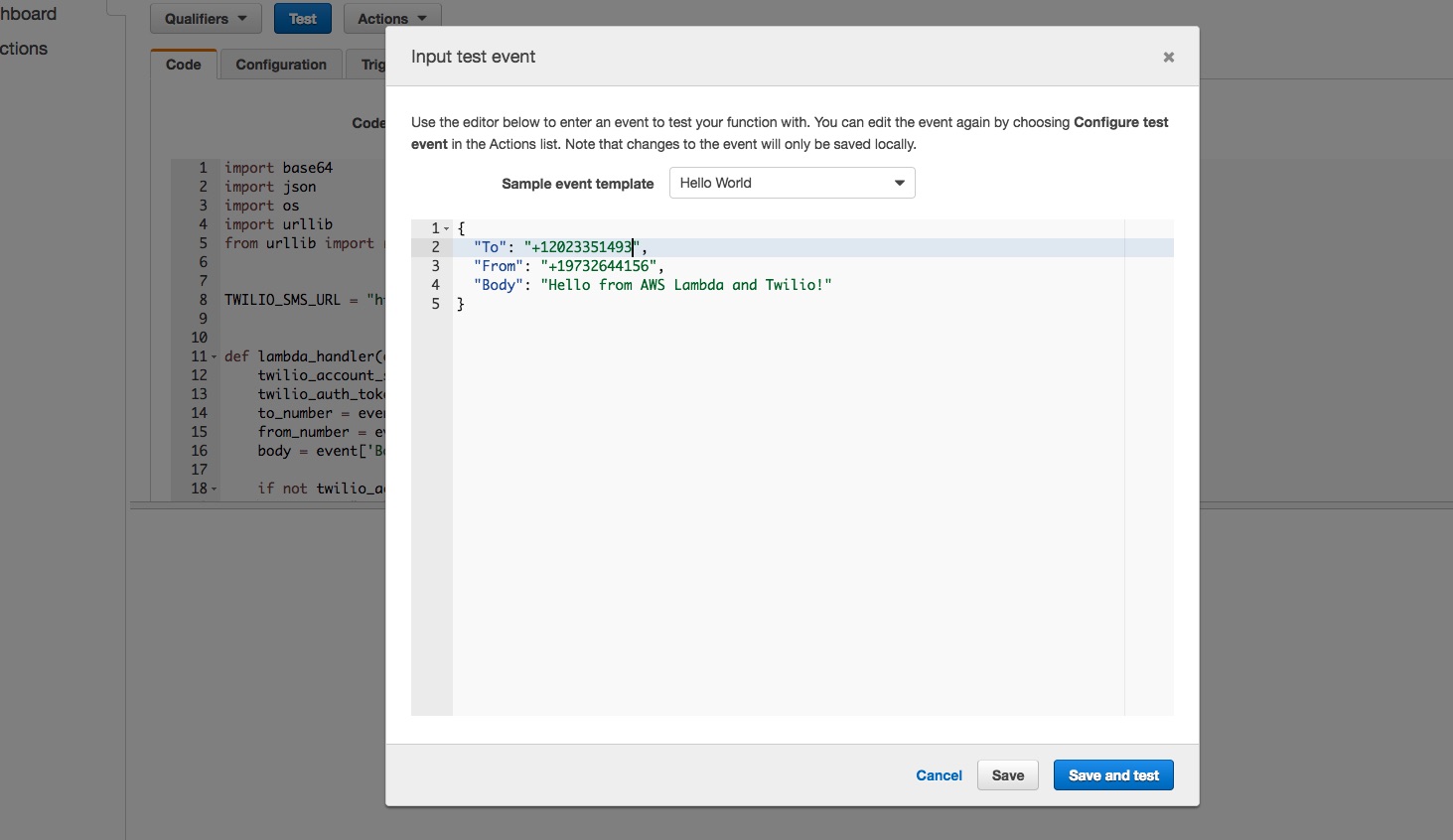
After entering your test event values, click the “Save and test” button at the bottom of the modal. Scroll down to the “Execution result” section where we can see our output.
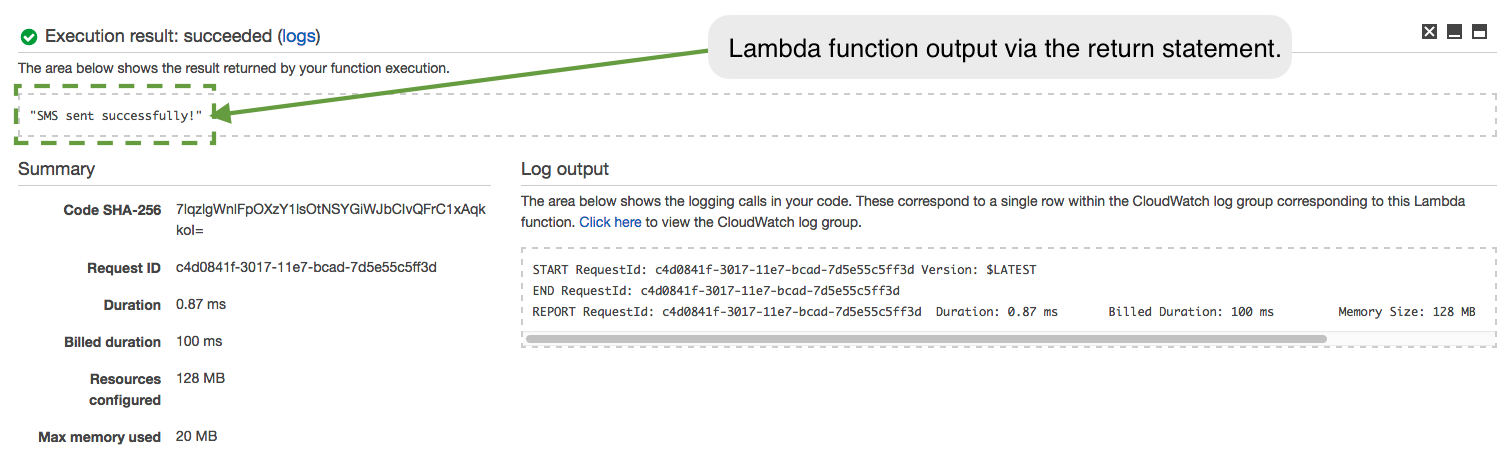
We can see the return value of the Lambda function and in just a moment our phone should light up with the received message like this:
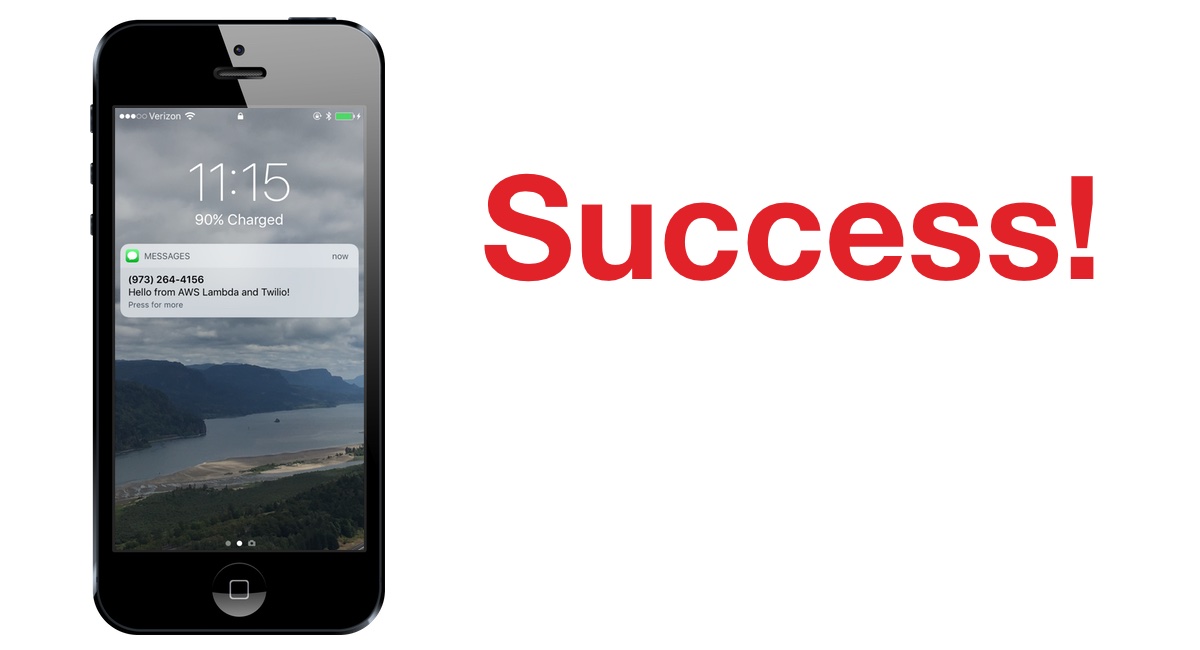
Awesome, our AWS Lambda function is working and we can modify it for whatever alert SMS notifications we need to send.
Next Steps
You just configured and ran some serverless infrastructure to call the Twilio API via the serverless AWS Lambda platform. There is a lot more you can do with Python and AWS Lambda. Check out the following tutorials for more code projects:
- “This Is Fine”… Or Not: Get Server Outage Alerts via SMS with Amazon Lambda
- Texting robots on Mars using Python, Flask, NASA APIs and Twilio MMS
- How to Create Your First Python 3.6 AWS Lambda Function
- Appointment Reminders with Python and Flask
- Build Your Own IVR with AWS Lambda, Amazon API Gateway and Twilio
Questions? Drop a comment down below or contact me via
- Twitter: @mattmakai
- GitHub: mattmakai
- Email: makai@twilio.com
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.