4 ways to schedule Node.js code
Time to read: 5 minutes
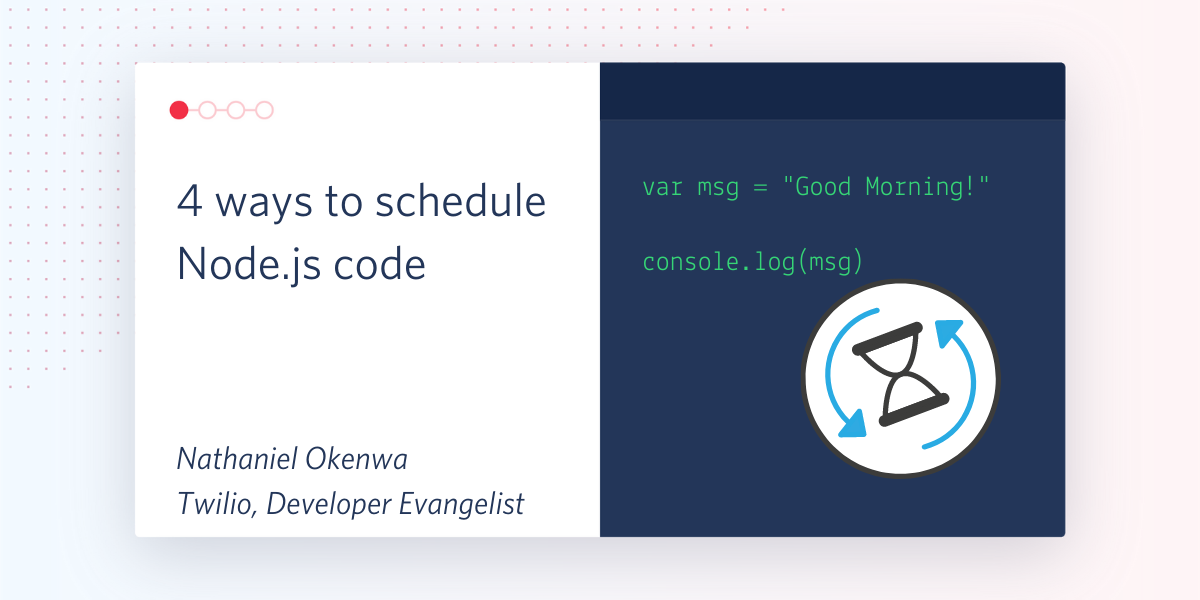
Have you ever needed to run some sort of scheduled tasks with JavaScript? I am constantly trying to find ways to automate repetitive tasks and the power of code is extremely useful in offloading the mental burden of a lot of these monotonous tasks. Also many applications need to run important tasks on a regular basis such as administration, system maintenance, daily backups or even sending emails or other communications. This is where cron steps in!
Cron Expressions
Cron is used to schedule time-based jobs, enabling them to run automatically at a certain date or time. Cron expressions are strings used to define the schedule upon which a task should be executed. Here is a link to help you build your own Cron expressions. Let’s say I wanted to schedule a Node.js script to run every morning at 9am. My cron expression would look something like this:
Let’s break this down. The first 5 parts of the expression are made up of strings that correspond to minute, hour, date, month and day of the week. If you want your command to run every x unit of time’ just place a *
in its place. So the above cron expression will run the command at 0 minutes after the 9th hour every day, every month, every day of the week.
If we wanted to schedule our command to run at intervals we can use a /
. For example if I wanted to run a command every other day at noon the expression would be:
Now that we understand cron expressions, let’s look at 4 ways we can use cron to get our daily texts up and running.
Running Locally with Crontab
Unix Systems
Crontab is a software utility built into Unix (and family) operating systems. A list of jobs lives in a crontab file. If you are on a Unix-like OS (Linux, macOS etc.) you can edit your crontab file by running this command.
Each entry in a crontab file consists of six fields separated by spaces. The first five form a cron expression and the sixth is the command to execute. Let’s say we want to run an ‘index.js’ file using node. Usually in our command line we would run the command:
However for our cron entry we need to include three things: our cron expression, the absolute path to node (usually /usr/local/bin/node
) and the absolute path to our index.js file. Our cron entry will look something like this.
Try adding your own javascript code as an entry to your crontab file. You can check what jobs are currently scheduled at any time by running the command”
This is the quickest way to schedule node.js code and we retain access to all our local resources, however there are some limitations. Firstly your computer has to be on at all times for your scheduled code to run. Not everyone wants to keep their machine churning at all hours, so let’s look at some ways to run our code on the internet.
Windows
If you’re on Windows, you have 2 choices. You could install the Windows Subsystem for Linux and and run the command:
This allows you to run a Linux environment and have access to the crontab scheduler just as above. Alternatively, you can use Windows Scheduled Tasks from powershell or cmd. In order to use the Scheduled Tasks, you will need to write a batch file(.bat) that triggers node. A very simple file could look like this:
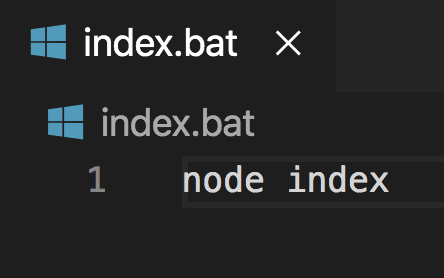
Windows Scheduler does not use cron expressions but you can still schedule jobs to run at a set recurring pattern. To create a scheduled task to run our batch file that will trigger our Node.js code we would run the following command:
Let’s break this down. This command will create a new task with:
- /tn - Task Name. A task name called
myTask
(p.s. This must be unique among tasks) - /tr - Task Run. The absolute path to the script file
- /sc - Schedule Type. I have chosen daily but other options include (MINUTE, HOURLY, WEEKLY and more…)
- /st - Start time. When the first task should be triggered. Subsequent tasks will run at the set interval
There are many more options to add even more complexity to the task schedule. The Microsoft Documentation has a comprehensive guide on how to use schtasks
Cloud Servers
If we want to have our code to execute on the internet we require some sort of infrastructure service. There are many popular services out there such as AWS EC2, Google’s Compute Engine, DigitalOcean Droplets, Azure Virtual Machines and so many more. I am not going to go into a deep dive into the differences between the services. However after going through the initial setup on each of these services you should end up being able to use the crontab tool just like we did locally.
Cloud servers are great as they are online 24/7 and I doubt that the internet is going to collapse anytime soon (knocks wood). However, setting up and maintaining a server can be time consuming. Also since our server is “always on” we’re technically always paying for it, although this should not exceed most providers free tiers.
Cloud Functions
Maintaining a server is significant overhead and a lot more work than many of us would like to deal with. There is also an additional problem that we are paying for our server year-round even if we run zero jobs in that time. Some cloud services offer cloud functions that can be triggered on a timed schedule.
Here are a few examples:
If you can’t find your cloud host among this list it doesn’t necessarily mean there is no way to configure cron scheduled jobs. Make sure to check their documentation for more on it. Just like cloud servers, our cron functions are available all the time and even better, we only pay for what we use
Cloud Triggers
Now this option is very similar to the cloud functions we spoke about earlier, hosting our JavaScript code in some cloud function. Some services will trigger a HTTP request to any given URL on a schedule and are really useful as they allow us to get around vendor lock-in. This is my favourite option, as many of the cloud function services that I love don’t have built in cron functionality. All we need to do is host our code with our favourite cloud function service. I personally love Twilio Functions. We can then trigger our cloud function on a schedule and voila, we have scheduled Node.js code up and running.
Here are some of my favourites:
With cloud triggers, it doesn’t matter where your code lives as long as it is possible to trigger it with a HTTP request. This is particularly useful when you have different functions across vendors. However, it also means there is a new place to manage scheduled jobs and is another thing you have to keep track of.
Summary
There are all sorts of ways to schedule your Node.js code to run at regular intervals. Each of the different methods of setting up your cron jobs have different pros and cons, and developers will have different preferences based on their needs. Personally, I love to use Twilio Functions with Google Cloud Scheduler. In the world of serverless, I no longer need to run a server to schedule my code. Awesome!
I can’t wait to see how you schedule your JavaScript code. Let me know what you’re working on at:
- Twitter - @chatterboxCoder
- Email - nokenwa@twilio.com
- GitHub - nokenwa
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.