Better Twilio Authentication with API Keys
Time to read: 4 minutes
Twilio generates an Account String Identifier (SID) and an Auth token when you create a Twilio account. With these credentials, you can perform all functions available in the Twilio API.
Anytime you hand over the Account SID and Auth Token to a device or a colleague, you increase the risk of those credentials becoming compromised. Luckily, Twilio provides capabilities to minimize this risk. This article covers:
- Auth Tokens
- Subaccounts
- API Keys
Auth Tokens
When you create a new account or subaccount, Twilio generates an Account SID and Auth Token for that account. You can find these credentials on the dashboard page of your account:
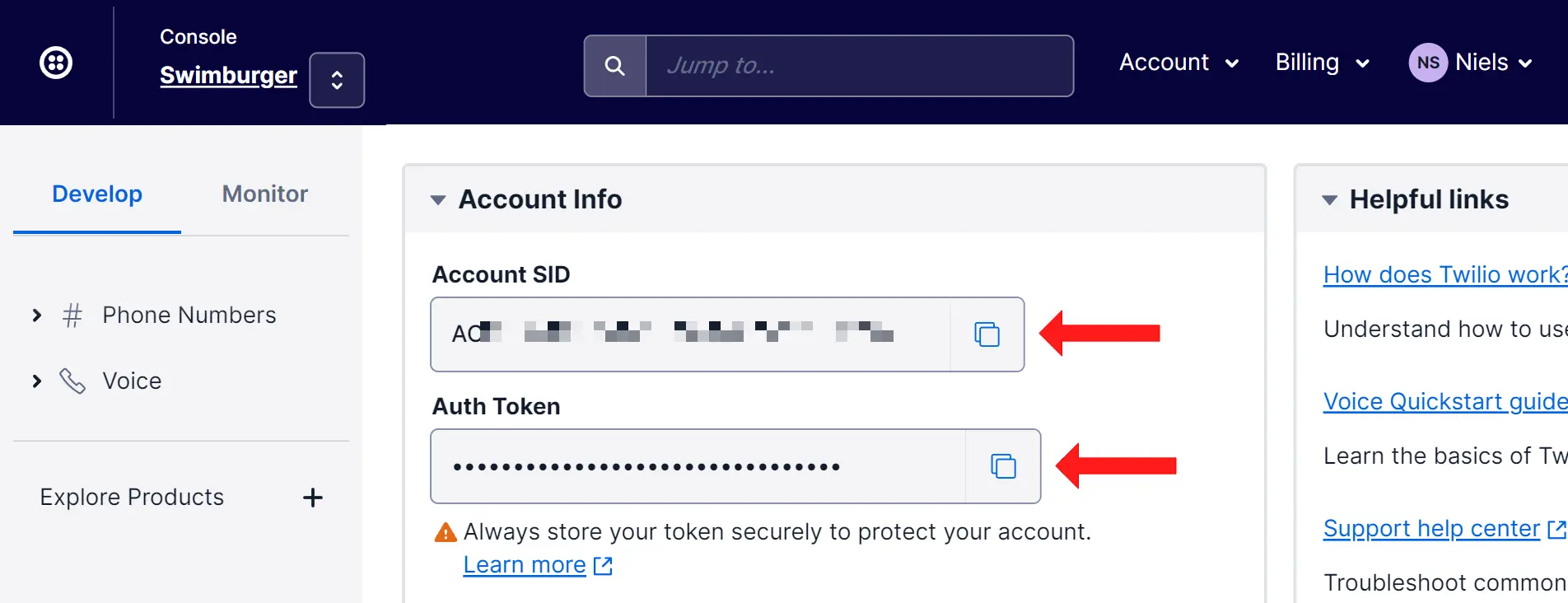
These credentials are frequently used to communicate with Twilio via the CLI, SDK's, or using the API directly. There is only one Auth Token by default, so you should avoid sharing this Auth Token to minimize the risk of it becoming compromised. If the Auth Token is compromised for some reason, rotate it by creating a secondary Auth Token so that the leaked token becomes useless.
Follow the steps below to create a secondary Auth Token:
- Click on the Account link in the top-right navigation
- In the submenu, click on API keys & tokens
- Scroll to the bottom of the page and click Request a Secondary Token
Once you have a secondary token, you can promote this token to the primary token. This will remove the old primary token and render it useless, as seen below.
Unfortunately, you can only rotate these tokens using the Twilio Console. It is not possible to rotate API Credential tokens using the API. However, you can rotate API Keys which will be covered later in this post.
Subaccounts
Subaccounts are just like accounts, but they are owned and can be managed by the parent account. Instead of using the Auth Tokens of the parent account, you can use the Auth Tokens of the subaccount. If the auth token of the subaccount is compromised, the token cannot be used to access resources of the parent account or other subaccounts.
You can create a subaccount using the Twilio Console by following these steps:
- Click on the Account link in the top-right navigation
- In the submenu, click on Subaccounts
- Click on the plus (+) icon if you have other subaccounts already, otherwise click the Create new Subaccount button
- Enter a friendly name for the Subaccount
- Click the Create button
You can also create subaccounts using Twilio's CLI, SDK, and API as documented in the Twilio Documentation.
API Keys
API Keys are the preferred way to authenticate to Twilio's services. There are two types of API Keys: Standard and Main API Keys.
Standard API Keys give you access to all the functionality in Twilio's API, except managing API Keys, Account Configuration, and Subaccounts.
Main API Keys have the same access as standard keys, but can also manage API Keys, Account Configuration, and Subaccounts. Main API Keys give you the same level of access as if you were using Auth Tokens.
You can create API Keys using the Twilio Console by following these steps:
- Click on the Account link in the top-right navigation
- In the submenu, click on API keys & tokens
- Click on the Create API key button
- Enter a friendly name for your API Key
- Select the region closest to you
- Select whether the key type should be standard or main
You can also create standard API Keys using the CLI, SDK, and API as documented in the Twilio Documentation. You have to be authenticated with an Auth Token or a Main API Key to manage API Keys.
Rotate API Keys
One of the advantages of using API Keys instead of API Credentials is that you can use the API to create and delete API Keys. This way, you can programmatically rotate API Keys as a preventative measure.
Here is how you would rotate the API Keys using the Twilio CLI and PowerShell:
Note: Make sure you have installed the Twilio CLI before running this code.
WARNING: Make sure you develop and test your application to ensure API Key rotation is handled gracefully.
You can create as many API Keys as you need, as opposed to API Credentials where you can only have two (primary and secondary) tokens per account. So instead of passing API Credentials to your teammates and applications, you should give them API Keys. This way you can safely revoke the API Keys when they are no longer used.
Move from Auth Tokens to API Keys
If you are already using the Auth Tokens in your code, you can switch to using API Keys with only a few lines of changes. Please note, you'll want to grab some of your Auth Token from the Twilio console and save them locally in environment variables for the code to run. For more information on how to do that, follow the instructions on storing Twilio credentials securely.
Here's how you would authenticate and send an SMS with the API Credentials using C#:
First, pass in your Account SID as the username
parameter, and Auth Token as the password
parameter to TwilioClient.Init
. Then, send a text message using MessageResource.Create
.
Update the parameters passed to TwilioClient.Init
to authenticate with your API Key instead of the API Credential:
First, pass in the API Key SID to the username
parameter instead of the Account SID. Then, pass in the API Key Secret to the password
parameter instead of the auth token. Lastly, pass in the Account SID to the accountSid
parameter.
Summary
You can authenticate with Twilio's API using the Account ID as the username and the primary or secondary auth token. If the primary token is compromised, you can promote the secondary token to the primary token which will make the old primary token unusable.
You can protect your credentials by segmenting your account with subaccounts. If an auth token or API Key for a subaccount is compromised, the token can only be used to access resources on the subaccount.
API Keys are now the preferred way to authenticate with Twilio's API. You can create as many API Keys as you need and remove them if they are compromised or no longer used.
Niels Swimberghe is a Belgian Full Stack Developer and technical content creator working in the USA. Get in touch with Niels on Twitter @RealSwimburger and follow Niels’ blog on .NET, Azure, web development, and more at swimburger.net.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.