How to Build a Conference Line with Twilio, ASP.NET Core, and C#
Time to read: 2 minutes
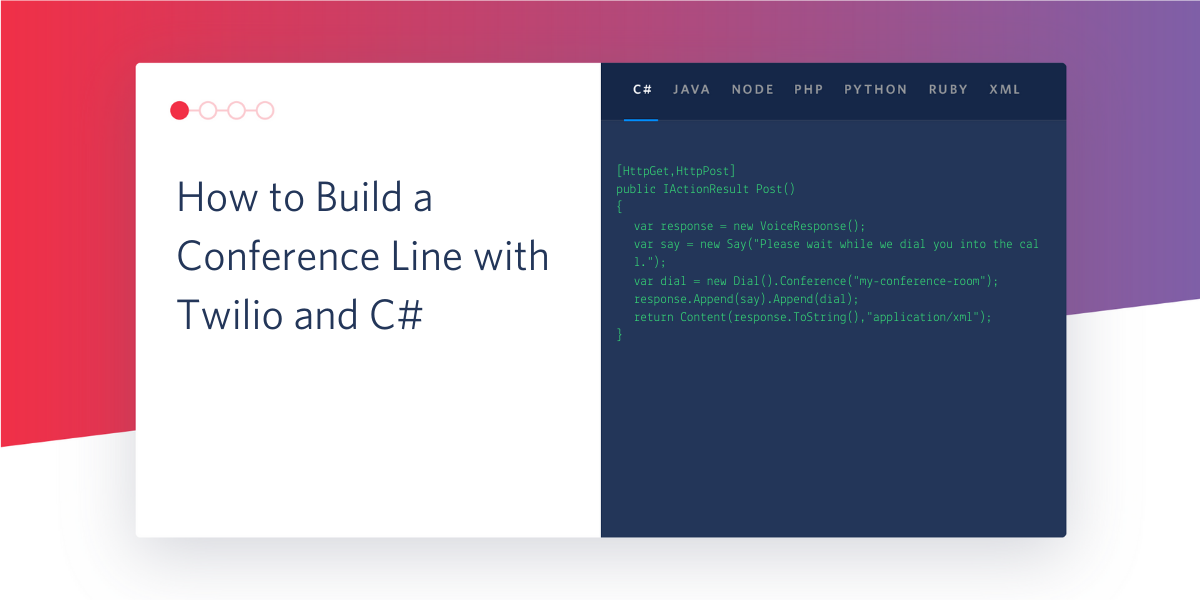
Another conference call, another app, another PIN, another log-in. Joining conference calls should be as simple as dialing a phone number, without needing to enter random conference IDs. In this post, we will walk through how you can build a conference line that anyone can join, using Twilio with C# and ASP.NET Core.
Developer Environment Setup
Let's make sure you have the software you need to build this conference line. For this, you will need:
Create the ASP.NET Core Project
It will create an ASP.NET Core Web API project, open it in Visual Studio Code, add the Twilio helper library, and close your command window.
Create a Route to Receive the Incoming Call
Next you'll create an API route by adding a new controller to the Controllers directory. With your project open in Visual Studio Code, create a new file in the Controllers directory named CallController.cs. Copy the below code into that file:
There are a few things happening here:
- The
[Route("api/[controller]")]
creates the URL path `https://localhost:5001/api/call` - The
[HttpGet,HttpPost]
attribute sets up the Post() method to execute whenever HTTP Post requests are issued to the URL - It creates a
VoiceResponse
containing a Say verb and a Dial verb that adds the caller to a conference room named `my-conference-room` - Finally it returns the
VoiceResponse
object as XML representing valid TwiML that Twilio understands and can interpret.
Running Your App
With all of this in place, it's time to run the app. In the VS Code terminal window, type dotnet run
. This will both compile your application and run it on ports 5000 & 5001.
For Twilio to reach your application, you can either deploy this code or run ngrok, which will provide a URL that allows Twilio to call into your local machine. In a new terminal window, type ngrok http https://localhost:5001
. This should give you a Forwarding URL to use in Twilio's console:
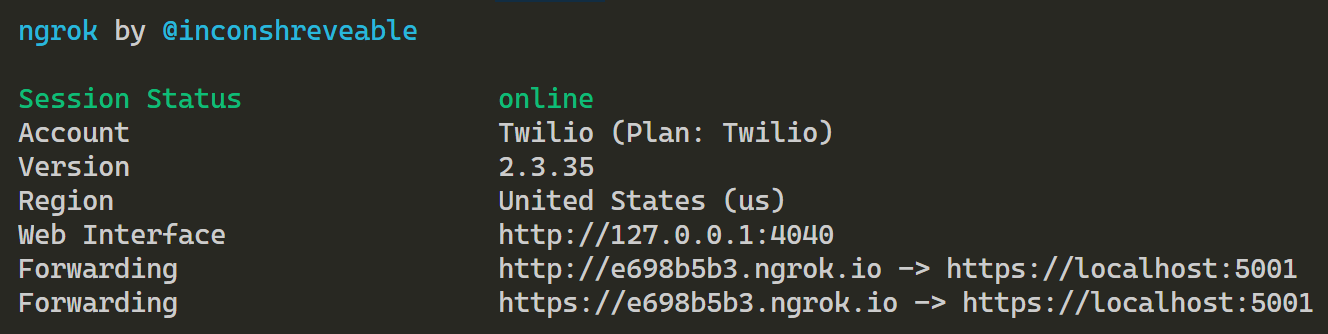
Before we update the Twilio console, open a browser window and visit your ngrok URL/api/call. For instance, using the above url - you would visit https://e698b5b3.ngrok.io/api/call and should see:
Connect Your ASP.NET Core App to Twilio
With your app running and an ngrok URL, head over to the Twilio console where you can purchase and configure a phone number. After you purchase a phone number, head to the Voice & Fax area of the console and update the incoming webhook to point to your ngrok url as in the photo below:
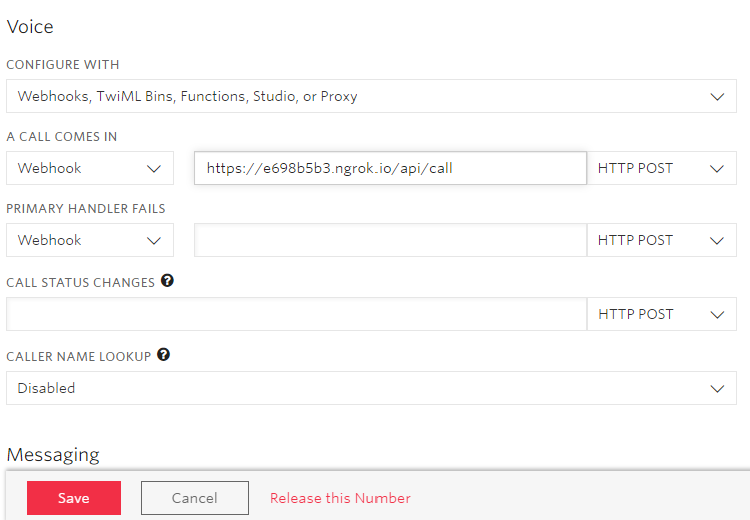
Give It a Try
Now that you know how to create a basic conference call, try building off of it with other features from our Conference TwiML docs such as moderating a conference call, changing the wait music, or perhaps the entry voice. If you want to explore more of what Twilio has to offer, definitely check out TwilioQuest where you can learn to unlock the power of communications with code. Feel free to reach out and share what you build. I can't wait to see what you build.
- Email: corey@twilio.com
- Twitter: coreylweathers
- Twitch: cldubya
- Github: clw895
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.