Chuck Norris jokes via WhatsApp with C# and Azure Functions
Time to read: 3 minutes
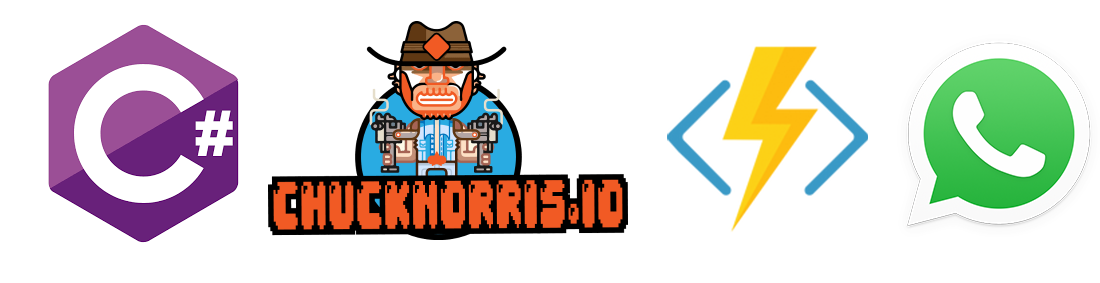
Setting up a .NET application takes time and sometimes you just want to write a quick bit of code that sends you a Chuck Norris joke via the Twilio API for WhatsApp! So that’s what we’re going to do with the help of Azure Functions.
What you’ll need:
- A Twilio account (sign up for a free Twilio account here)
- An Azure account (sign up for a free Azure account here)
- The WhatsApp Sandbox Channel installed in your account (go through this process to activate the sandbox)
Azure Functions allow you to write code without having to worry about infrastructure or servers, so they are perfect for setting up a simple webhook for an incoming SMS or call from Twilio.
Create an Azure Function
From the Azure portal, click the Create a resource button, found at the top of the menu on the left. Search for a Serverless Function App and then click on the matching result.
Give your app a name, I called mine twilio-whatsapp-jokes
, create or reuse a resource group, and choose the Location that best suits you. You can then click Create at the bottom of the panel.
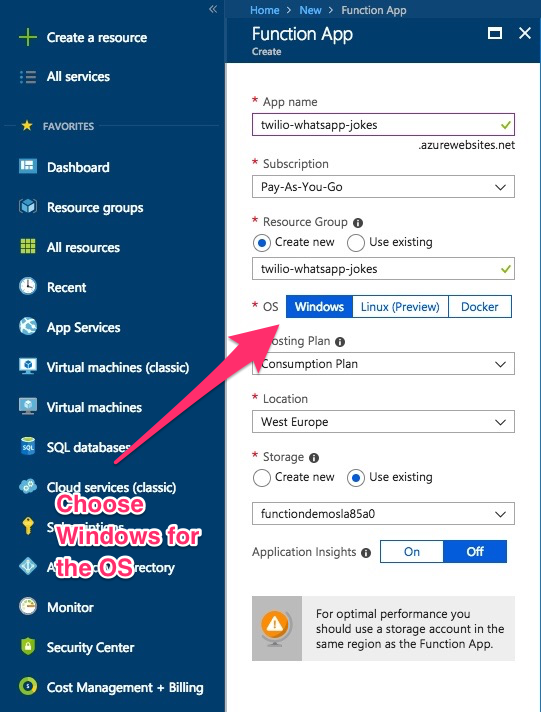
Once that has deployed, go into the Functions from the menu on the left. If you can’t see Functions then click on All services, it’s below Create a resource, search for Function Apps and then click the star to the right of the result to favourite it. Now it should be on the panel on the left.
You should see your newly created Function App with three submenus; Functions, Proxies and Slots. Click on the plus button beside Functions to add a new function to our Function app.
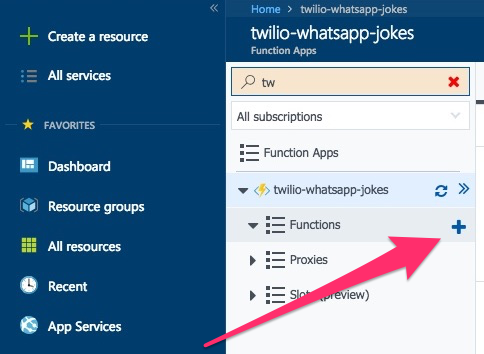
You’ll be given some options for quickstarts but we are going to create a Custom Function, so go ahead and click on that link.
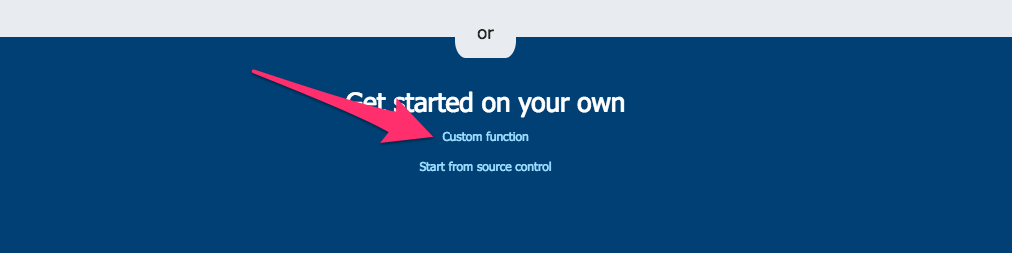
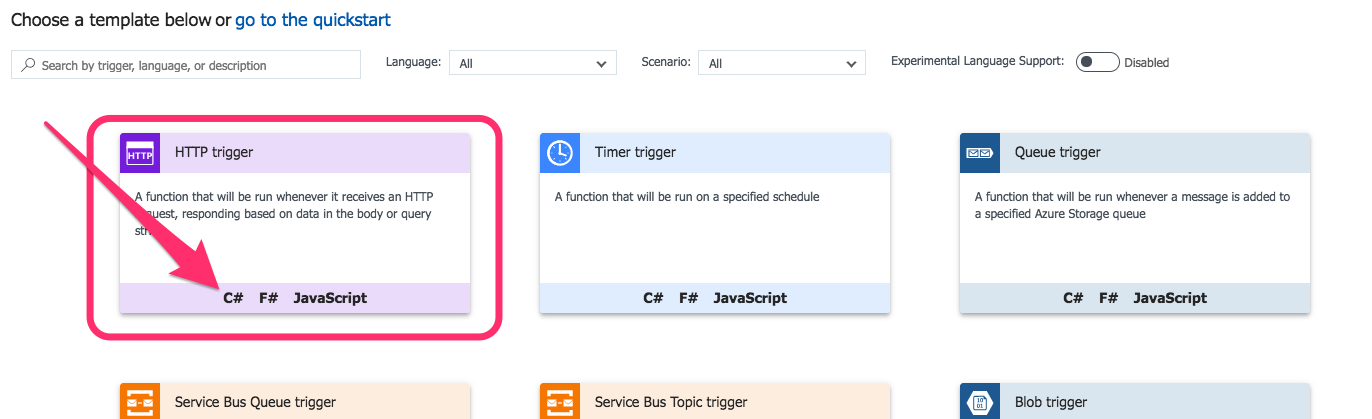
The link will take you to a screen with loads of template types. The one we want, HTTP trigger, should be first, select the link for C#. A panel will slide out from the right of the screen asking you to name your function, do this and then click create.
You should now see your newly created function listed under your function app. Click on the Get function URL button and save the URL for later.
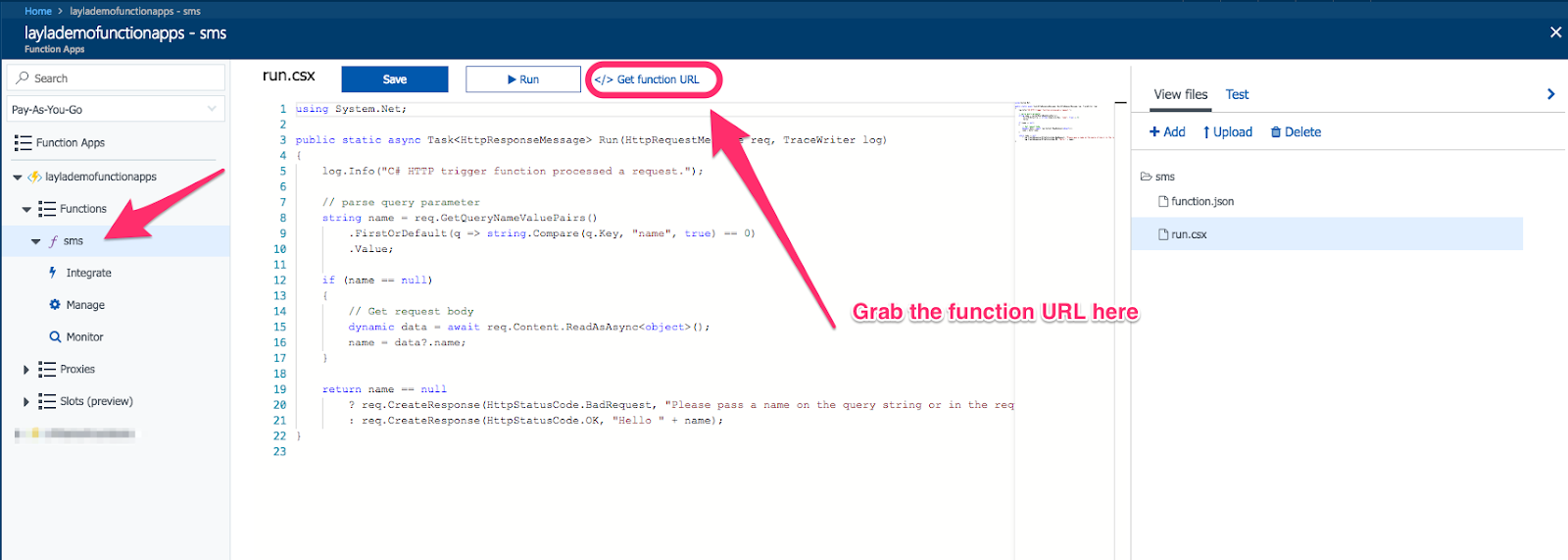
On the far right, you will see a panel, expand this and click on the View Files tab. We need to add a new file called to project.json
enable us to bring in NuGet packages. Once added, copy and paste the code below in and save it.
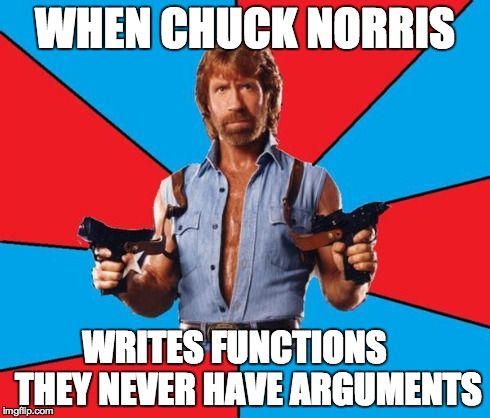
We will need a method to fetch a random joke from the Chuck Norris API, one of my favourite APIs!
So, let’s add another file to the function called GetJoke.csx
and add the following code. We are using an HTTP client to make the call to the Chuck Norris API. If our mine, is successful, we go ahead and deserialize the returned JSON string to a dynamic
object and return the value
.
Azure Functions come with some external assemblies, like Newtonsoft.json
already included, we just need to reference them using the #r "Newtonsoft.Json"
notation. This also means we don’t need to add them to the project.json
file.
Click on the run.csx
file. This is our entry point so we will handle the incoming API call here.
Update the file with the following code where we set up our usings
. Note how we reference our GetJoke
method with #load "GetJoke.csx"
.
Now, all we need to do is grab a joke using GetNewJokeAsync
and create a new TwiML
response to return our joke.
Now that we have our working function we can add the function URL we saved earlier to the Twilio API for WhatsApp configuration, under A Message Comes In.
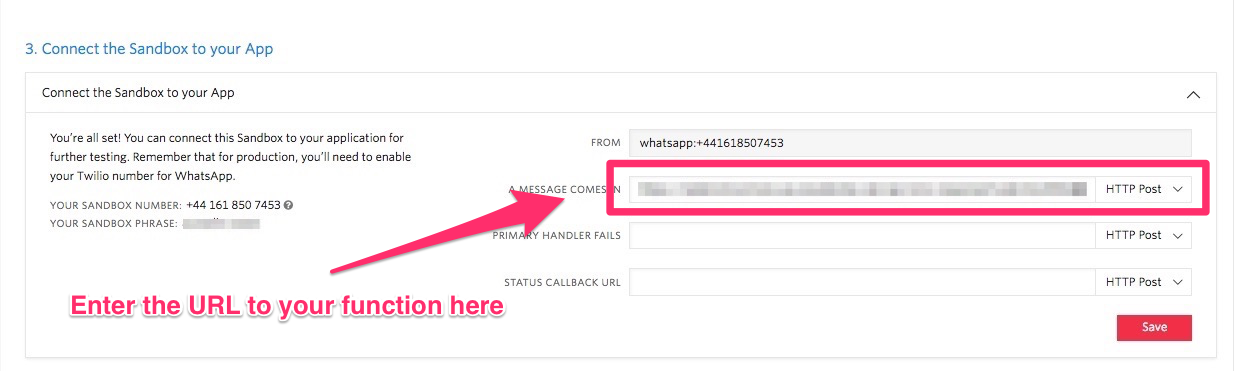
Time to retrieve a joke
Send your message to the Twilio WhatsApp number and you should receive a random Chuck Norris joke.
Your day has now been made!
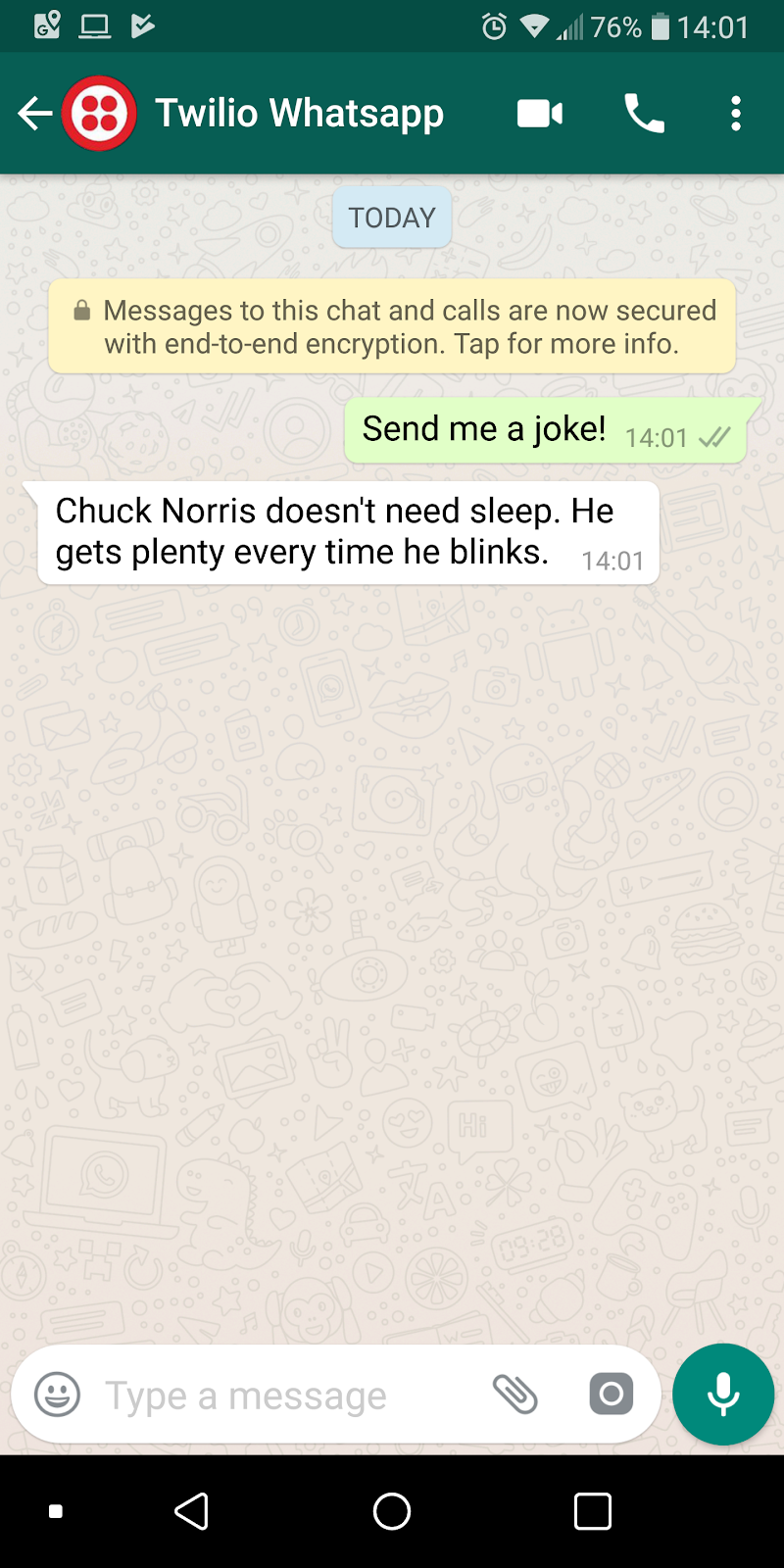
What else can we do?
Well, an improvement on this app would be to text in a joke category and then return a joke that fulfils that. You can check out one of my other Chuck Norris projects to see how you might do this and this blog to see how you can extract the message body from the request.
Azure functions are great for small sections of code that you want to reuse across different projects like token generators for example.
Azure functions on the Consumption plan will scale automatically so they are also great for high load, sporadic jobs.
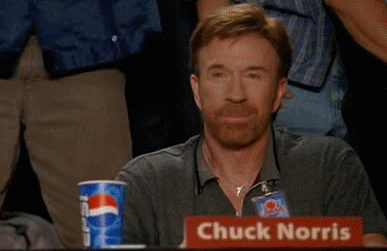
Let me know what cool ideas you have for Twilio and Azure functions and feel free to get in touch with any questions. I can’t wait to see what you build!
- Email: lporter@twilio.com
- Twitter: @LaylaCodesIt
- GitHub: layla-p
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.