Send an SMS from Twitch Chat with Node.js and Twilio Programmable Messaging
Time to read: 5 minutes
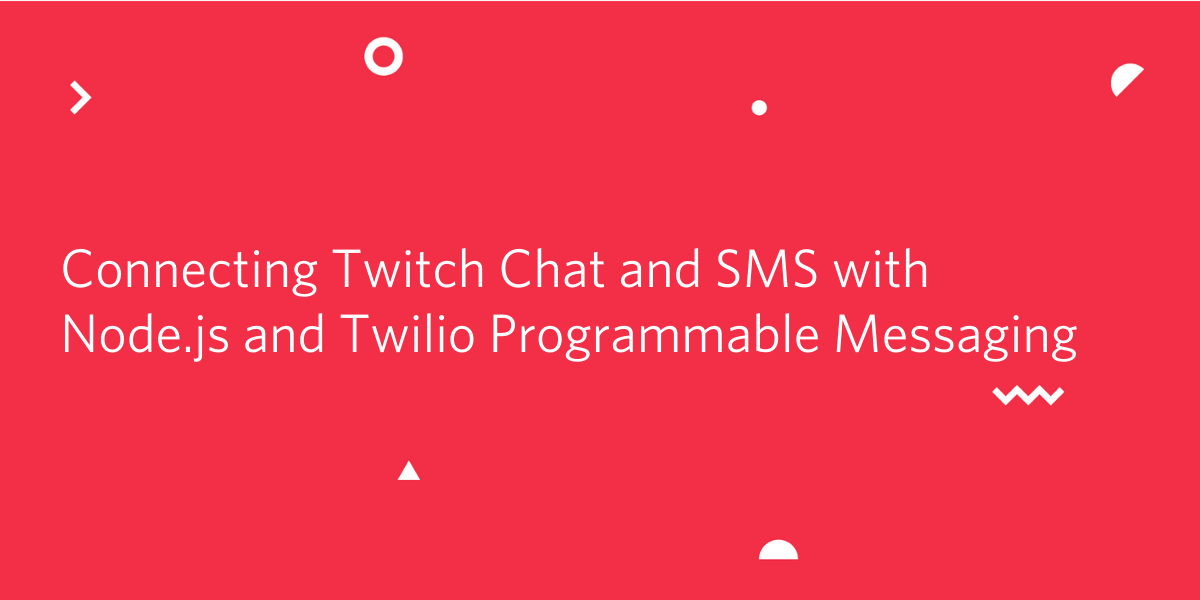
From watching people play your favorite games, to seeing awesome things built with code, to cooking up a storm in the kitchen, you can find a huge variety of content being streamed live on Twitch.tv. A mainstay of many Twitch channels is having a bot to engage with viewers in chat to automate things such as tasks for moderators or sharing contextual information with newcomers. Chatbots can even control Pokemon games!
With Twilio Programmable Messaging, you can write code to send and receive text messages. This could be useful for streamers for something like notifying subscribed users of when they're doing something particularly cool on stream.
Let's walk through how to create a chatbot to send SMS notifications to users on your own channel with JavaScript using Node.js.
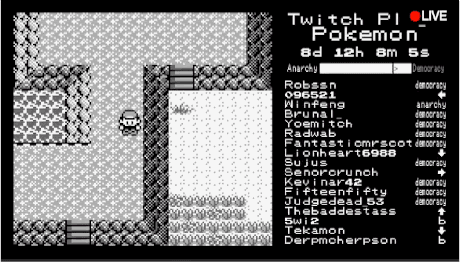
Taking care of dependencies
Before writing any code, you will need an up to date version of Node.js and npm installed.
Navigate to the directory where you want this code to live and run the following command in your terminal to create a package for this project:
The --yes
argument runs through all of the prompts that you would otherwise have to fill out or skip. Now that we have a package.json
for our app, run this command in the same directory to install the tmi.js library for Twitch chat as well as the Twilio helper library for Node.js:
Twitch chat can be accessed through IRC, and we can programmatically connect to Twitch IRC with tmi.js. You should now have everything you need to begin building your bot.
Creating a Twitch Chat Bot with Node.js
Now that we can generate text, let's work on creating a basic Twitch chat bot that interacts with viewers.
Connecting to a Twitch stream and reading chat messages
On the Twitch side of things, let's begin by making an anonymous, read-only connection to a Twitch channel's chat. No authentication credentials are needed to read messages sent to a channel, so we can just log all incoming messages with tmi.js without overcomplicating things.
Create a file called index.js
in the same directory as your package.json
. Add the following code to it for logging all messages sent to a given channel as they come in:
Replace the channel_name
with the Twitch username of whoever's stream you're testing with, and run the code with the command node index.js
from your terminal. You should see messages being logged which looks something like this:

Generating an Oauth token and sending messages to a channel
In order to send messages, from either your personal account or one you create specifically for your bot, you'll need a token to authenticate your chatbot with Twitch's servers. Go to https://twitchapps.com/tmi/ and log in with your Twitch credentials to authenticate TMI with your account.
After logging in, click "Authorize" and you should be given a token. Save this Oauth token you received in an environment variable to be used in your code later:
You can use this token to connect to Twitch chat with tmi.js and send messages from whichever Twitch account you authenticated with.
As an example, let's try some code to respond to people who say hello. Replace the code in index.js
with the following:
This time you'll have to replace your_username
with whatever Twitch account you're using to test this with, preferably one you created for your bot. I am just going to use my personal account for this, to avoid being rude and testing my bot in someone else's channel. But if you have any close friends who are streaming it can be fun to connect to their streams.
Run the code with index.js
again, and try saying "!hello" in the chat to get a response:
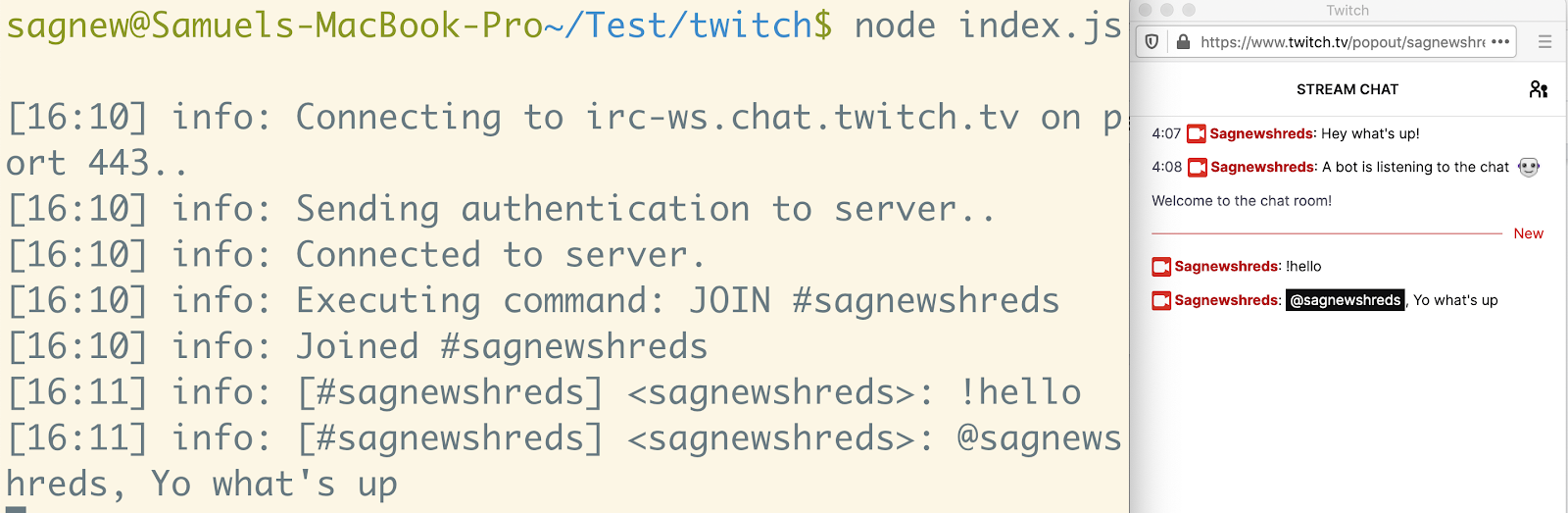
Because I am using the same account for both it may appear as if I'm talking to myself, but one of those messages is automated and one is not.
Working with Twilio SMS
With a minimal amount of code, you can send text messages in Node.js using Twilio. But before writing code, you'll first need a Twilio account and a phone number.
Getting a Twilio phone number
You can sign up for a free Twilio trial account here, and can get a phone number by navigating to the Buy a Number page, checking the "SMS" box and clicking "Search."
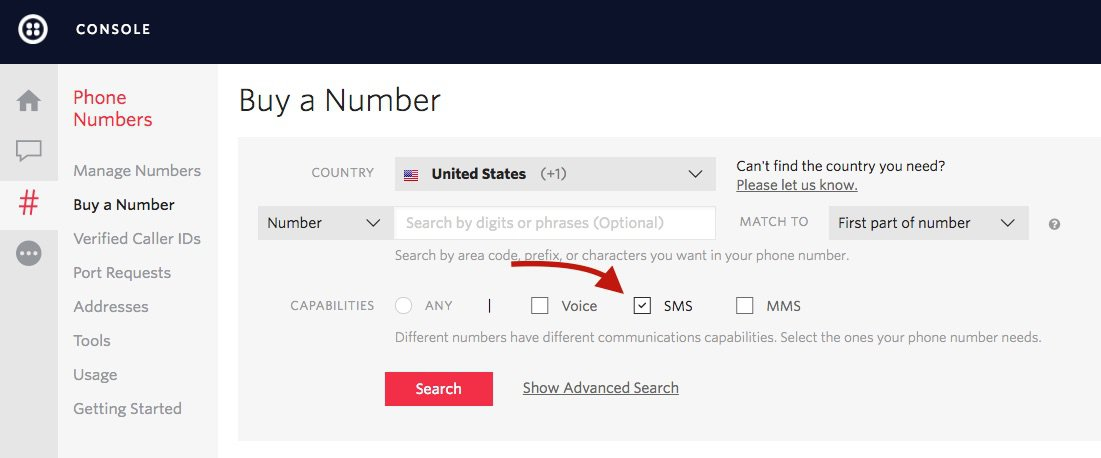
You’ll see a list of available phone numbers and their capabilities. Choose one and click "Buy" to add it to your account.
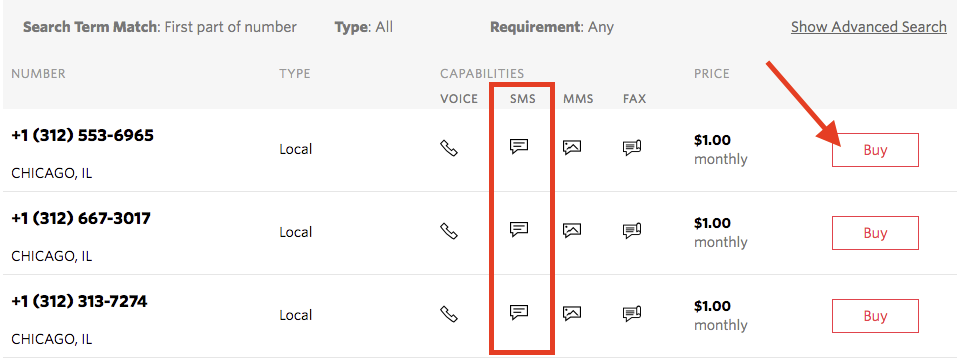
Sending an outbound text message
It's time to use the phone number you just purchased to programmatically send a text message. Create a file called sendSms.js
and add the following code to it:
Before running this code, make sure to grab your Account SID and Auth Token from your Twilio Console, and save them as environment variables named TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
, which the Twilio Node library will use when you run your code.
Next, replace the values in the code with your Twilio phone number and your personal phone number in this format: [+][country code][phone number including area code]
.
Run the code with the command node sendSms.js
from the directory that the file is in, and you should receive a text message!
Combining Twitch Chat and Twilio SMS
So now that we've written code to do these two things individually, let's bridge them together by creating a bot which a streamer can use to send notifications to people via SMS from their channel's chat. Perhaps the streamer would want to do this to let people know that a new speedrun attempt is about to begin, or that they are switching games. We'll make it so that the streamer can use a command to send any text to people who have previously signed up and provided their bot with their phone numbers.
Create a file called index.js
with the following code in it:
Run the code with index.js
and then go to your Twitch channel, type in a message like "!notify Hey everybody we are starting a new run!". If you've replaced all of the relevant variables in the code, you should receive a text message with whatever you typed in your Twitch chat.

The Next Level
We've covered some of the basics of creating a Twitch chatbot for you and your friends' channels and how to use it to interact with users over SMS via Twilio Programmable Messaging. You can continue working on this by integrating other APIs such as OpenAI's GPT-3, or adding new features that you think would be useful.
Feel free to reach out if you have any questions or comments, or just want to show off any cool things your Twitch bot can do.
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.