Adding MP3 to Voice Calls using Twilio in Node.js
Time to read: 7 minutes
Adding MP3 to Voice Calls using Twilio in Node.js
You might already know how to send voice messages during calls through Twilio Programmable Voice, but did you know you can also play MP3 audio files in a Twilio voice call? Whether it’s your favorite jingle, a meme soundbite (rickroll is go-to) or even a full song, you can stream it directly to your Twilio calls in just a few lines of code.
In this tutorial, you’ll learn how to use the TwiML <Play>
verb to play MP3 audio in incoming and outgoing voice calls in Node.js using Twilio Programmable Voice. If you just want to play MP3 in either an outgoing call or just an incoming call, I’ve separated the tutorial into two sections so you can jump to the part that fits your use case.
Prerequisites
- A Twilio account - Sign up with Twilio for free here
- A Twilio number - Read our docs here on how to obtain a Twilio number
- Node.js installation - Download Node.js here
- ngrok installation - Download ngrok here
- Not required for outgoing phone calls
Set up Node.js Application
In this section you’ll set up your application by initializing your Node.js project, installing the needed dependencies and importing your Twilio credentials as environment variables.
Initialize Node.js project
Start off by scaffolding out the application where you’ll be initiating and receiving Twilio calls from. Open your terminal/shell, navigate to a preferred directory where your project will be held, and enter the following command to initiate a new Node project:
Open the mp3-call project directory in your preferred IDE and create a file called index.js within the directory. This file is where you’ll be writing the code that will initiate and/or receive calls from.
Install Dependencies
The next step is to install the dependencies required for this project. Run the following command on your terminal:
The twilio
package will be used to access the Twilio Programmable Voice API to send phone calls. dotenv
will be used to access environment variables from a .env file, which is where you will store your Twilio credentials and Twilio number. The express
package will be used to build your server which will capture incoming phone calls and host your local MP3 file
Import Environment Variables
You’ll need your Twilio Account SID, Auth Token and phone number to initiate and receive phone calls from your Node.js application. Log in to your Twilio Console, scroll down to the Account Info section and you’ll see Account SID, Auth Token and phone number:
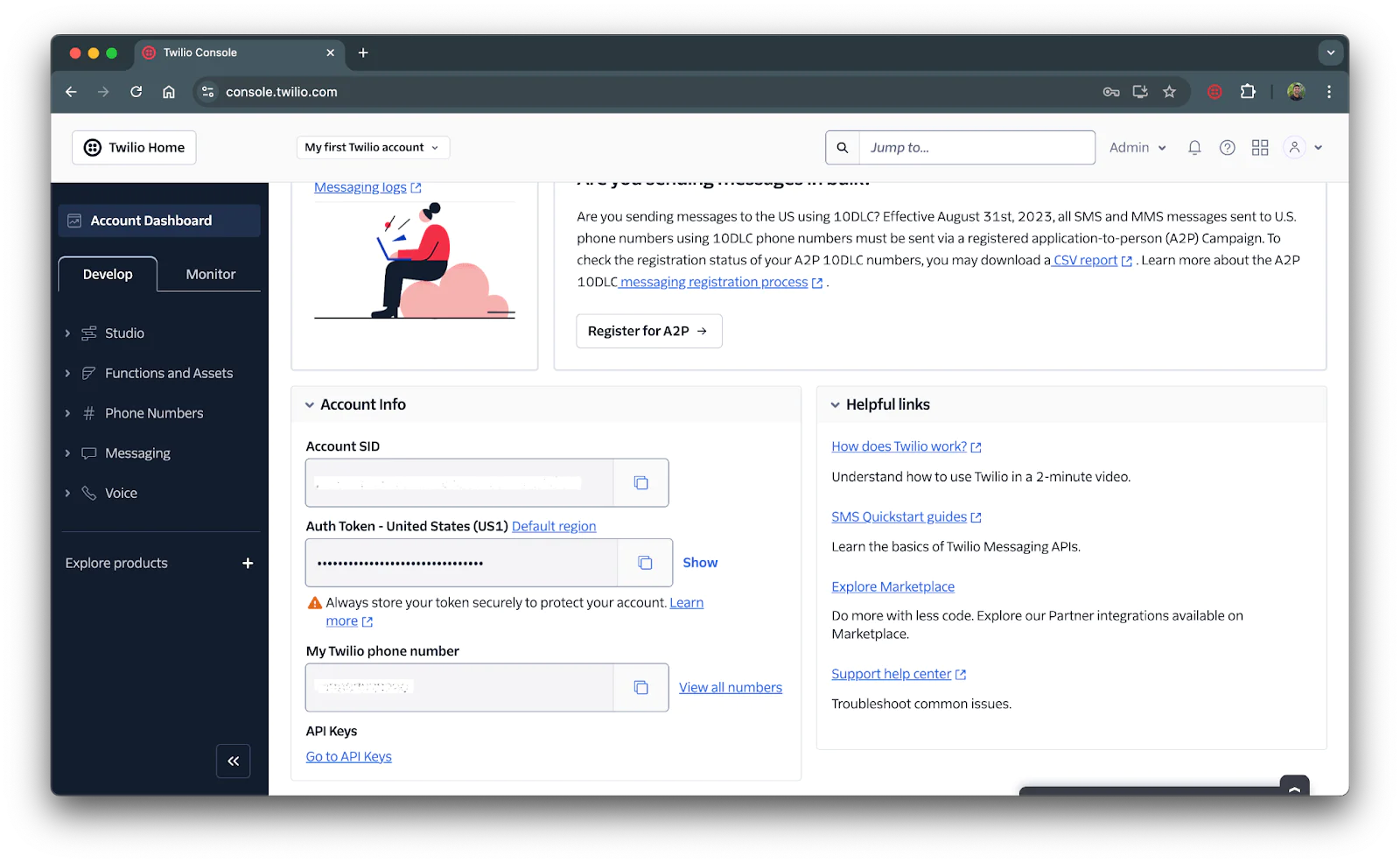
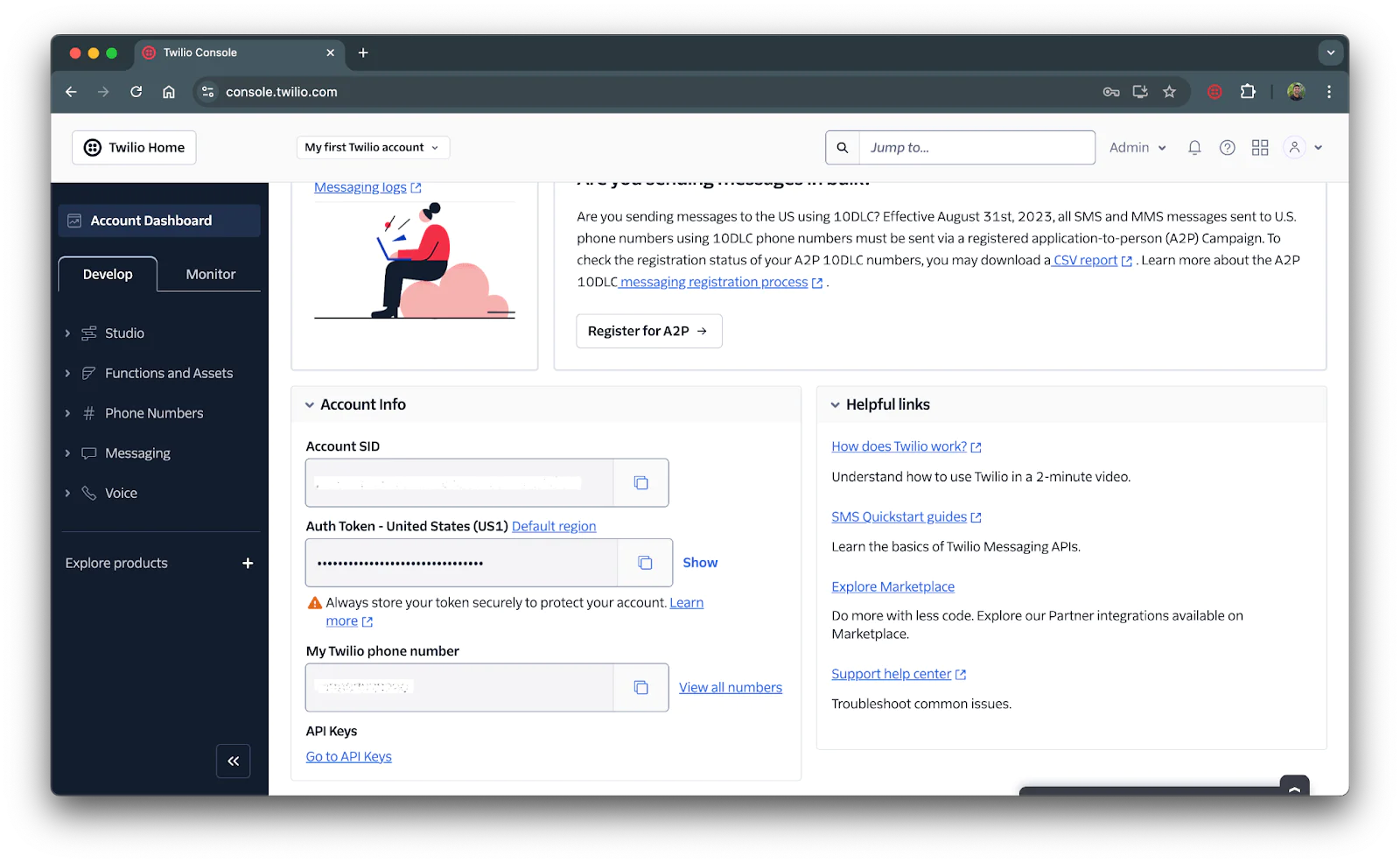
Save this screen and head back to your IDE to create a file named .env. Copy the following into your .env file:
Replace the XXXXXX
placeholders with their respective values from the Account Info section on your Twilio Console and save the file.
Hosting your MP3 File
This section is optional if you’re looking to just test out playing any MP3 audio in phone calls or already have a hosted MP3 file. We have a demo MP3 file hosted on our server that you can use. You can copy and use the following URL for the next section:
However, if you have a local MP3 file that you want to use in your phone call, you’ll first need to host it to have a way for Twilio to publicly access this file through a public URL. I’ll go over two ways of hosting your MP3 file: Twilio Assets and through your Node.js Application with Express.
For the sake of simplicity, I recommend using Twilio Assets.
Option 1: Twilio Assets
Twilio Assets is Twilio's static file hosting service that allows you to quickly upload and serve the files needed to support your applications. Head over to your Twilio Console and on the left sidebar click on Function and Assets > Assets (classic):
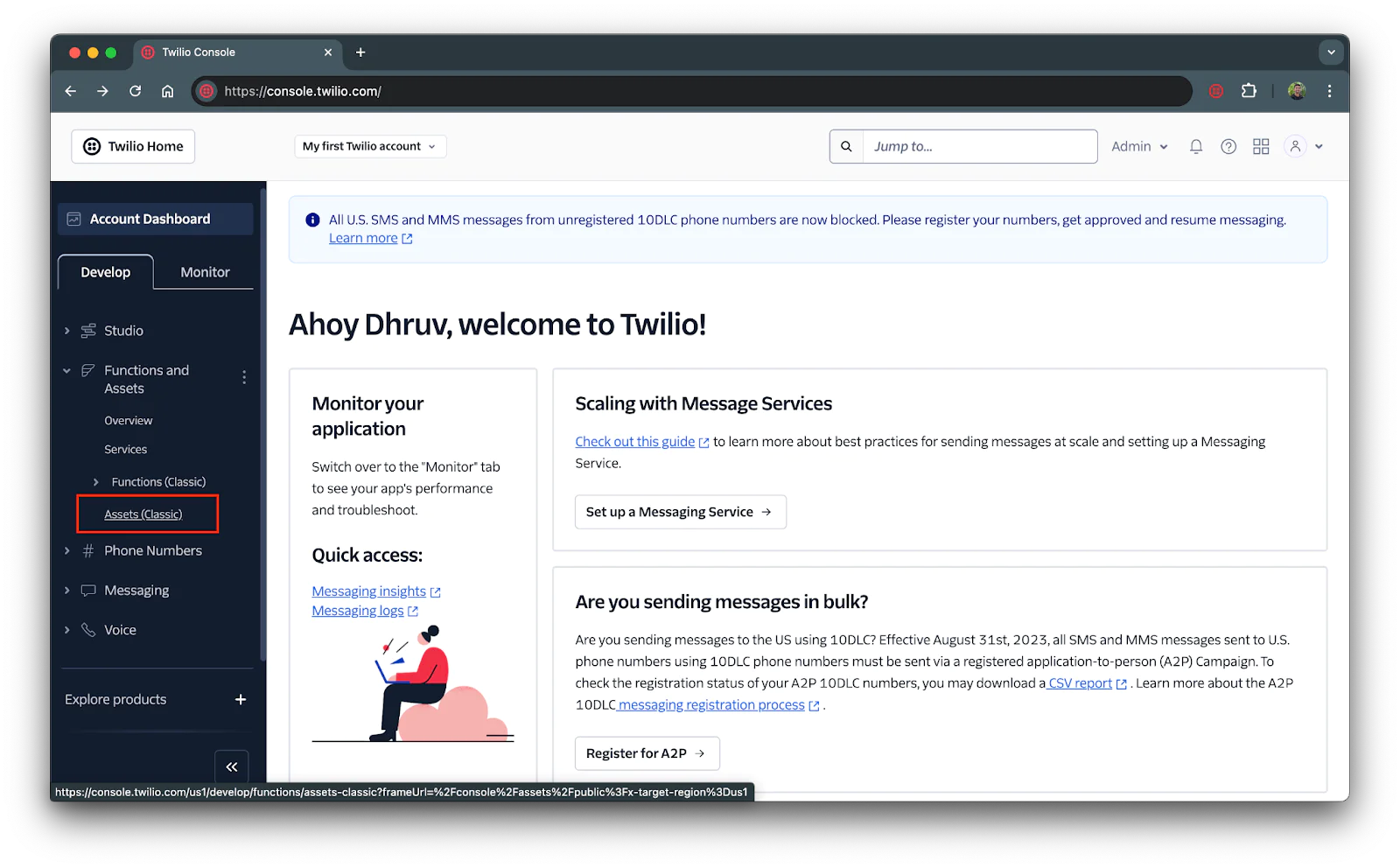
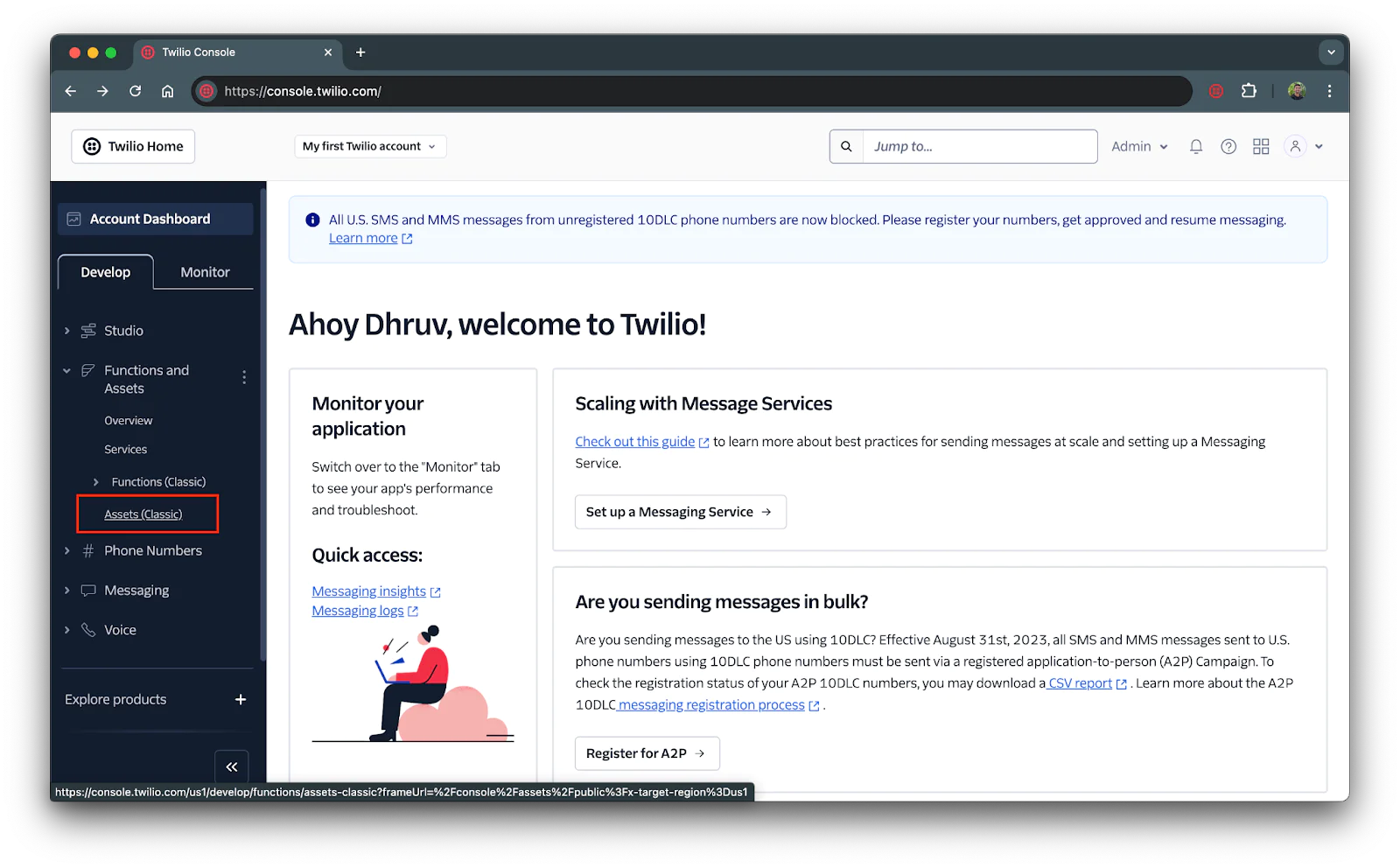
Next click on the blue button that says Add an Asset and select the MP3 file from your computer.
Once selected, click on the blue Upload button:
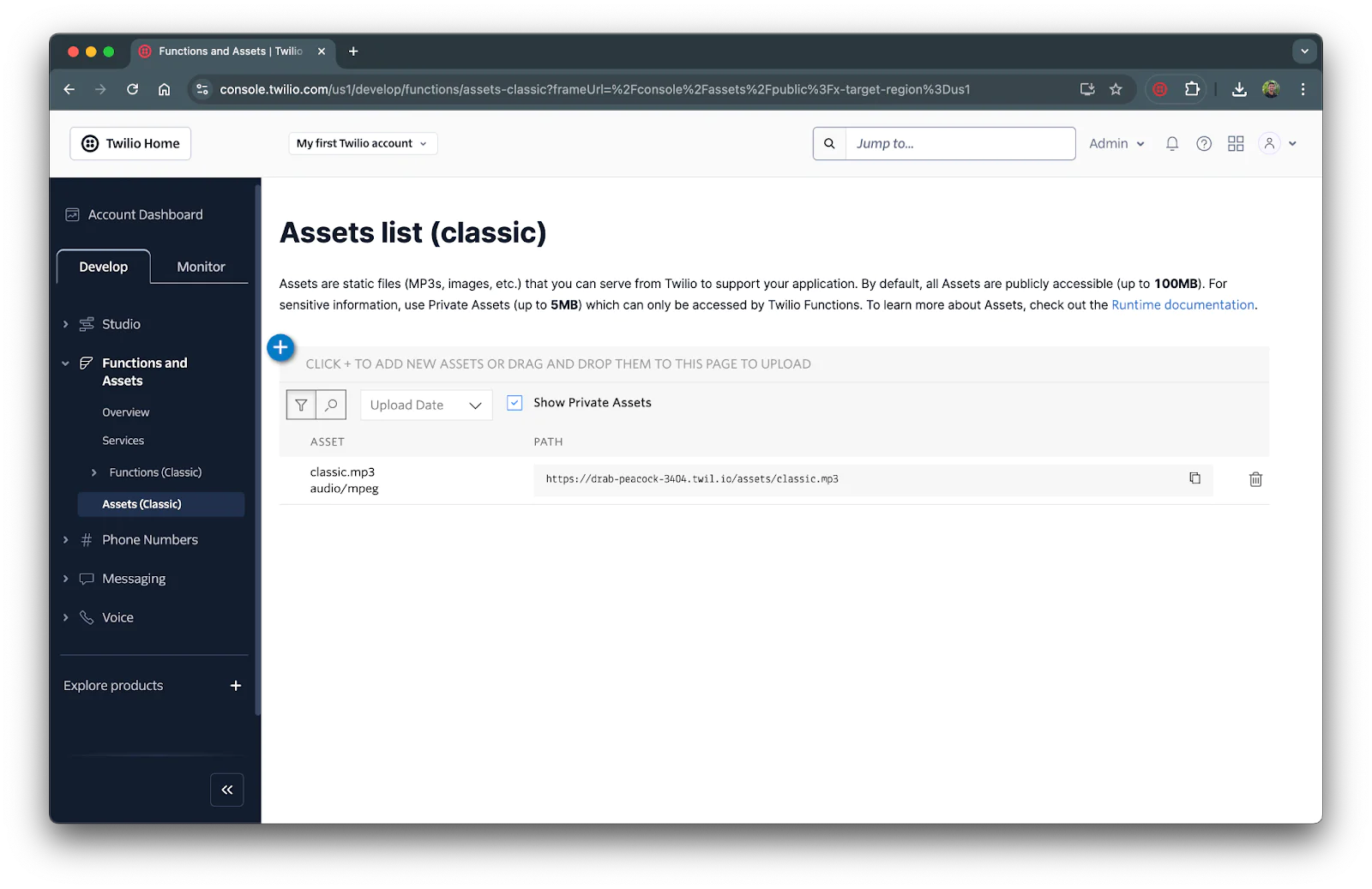
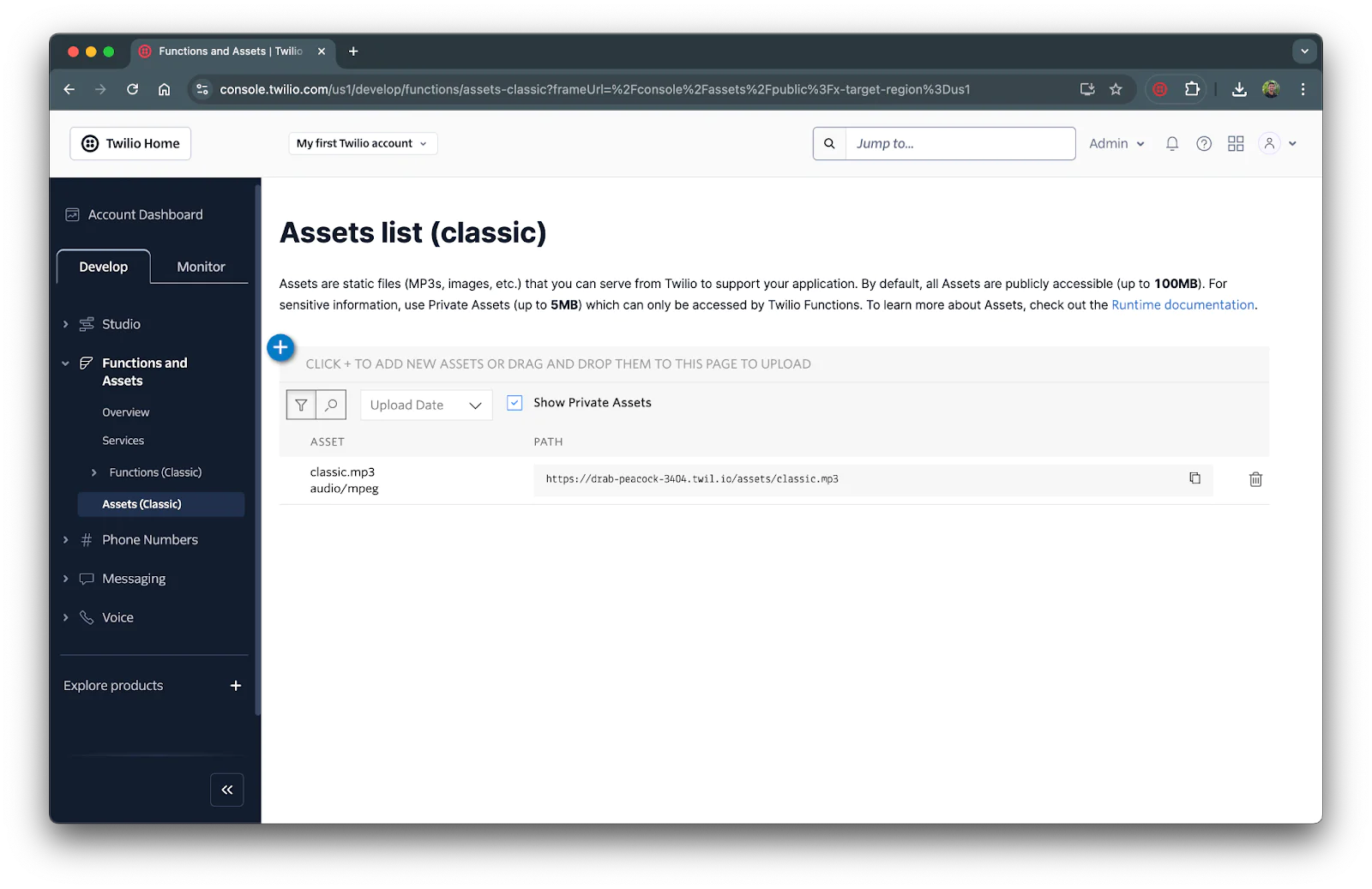
This URL will be used to access your MP3 file from your Node.js application so copy and store it for the next section.
Option 2: Express
You can also host your MP3 file on your Node.js application when it's being used as an Express server; their express.static
middleware function allows you to serve static files.
Within your root project directory (mp3-calls), create a folder called /public and place your local MP3 file within this folder. Ensure to name the file to something recognizable like classic.mp3.
Open your index.js file and place the following code at the top of the file to have Express serve files from the /public directory:
Save the file and open your terminal to execute the following command:
You’ll see App listening on port 3000!
printed on your console which means your local server is live! Open your browser to localhost:3000/classic.mp3 (replace classic.mp3 with your MP3 file name if it's named something else) and you should be able to listen to the MP3 file on your browser.
However, this server is only locally hosted on your computer which means its not publicly accessible. ngrok will be used to connect your Express server to the internet by generating a public URL that will tunnel all requests directly to your local server on your computer.
Open a new tab on your terminal and execute the following command to spin up a tunnel to your local server:
After executing the command, your terminal should look like the following:
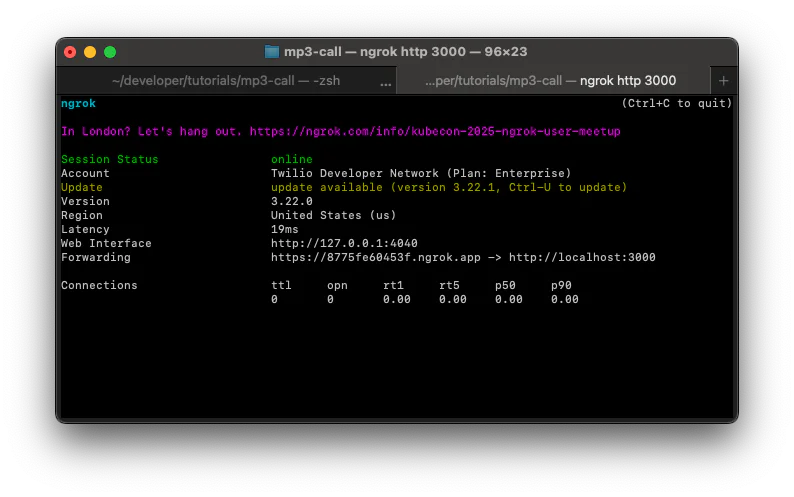
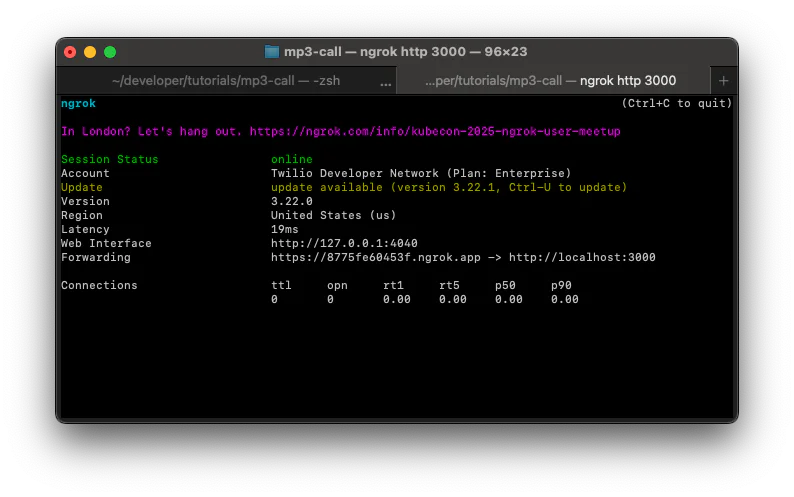
Copy the Forwarding URL, append the name of your MP3 file name to it and open it up in the browser. This will be the URL you’ll use to play your MP3 file in your Twilio phone call.Navigate back to the terminal tab where you ran your Node.js application and click CTRL + C
to stop the server so you can add more to the code.
<Play> TwiML Verb
Twilio's Markup Language (TwiML) will be used to instruct and process phone calls to and from your application. TwiML provides a set of simple verbs and nouns which is used to tell Twilio what to do with your calls.
For this application, you’ll be using the <Play> verb which plays an audio file to the caller from a given URL. Here’s a list of the supported file types that can be used on a call.
In addition to playing audio files, you can use the digits
attribute to play DTMF tones if you need to test an IVR system. The loop
attribute can be used to specify the amount of times to play the audio file during a call.
MP3 on Incoming Calls
Now that we have everything set up to play an MP3 file on a voice call, lets put it to use on incoming phone calls.
Writing and running the code
Replace the existing code in index.js with the code below:
This code will handle all incoming calls to your Twilio phone number. It will start an Express server on port 3000 and listen to all incoming HTTP requests to the /mp3 route. This route will create a twiml
voice response that uses the <Play>
verb to play the MP3 file to the call by referencing your URL where the file is hosted. Save the file and navigate to your terminal to test out the code.
Run the following command on your terminal to create your local Express server:
Creating an Ngrok tunnel
If you already created an ngrok tunnel by following Option 1: Express section of the tutorial, you can skip this section. ngrok will be used to connect your Express server to the internet by generating a public URL that will tunnel all requests directly to your local server on your computer.
Open a new tab on your terminal and execute the following command to spin up a tunnel to your local server:
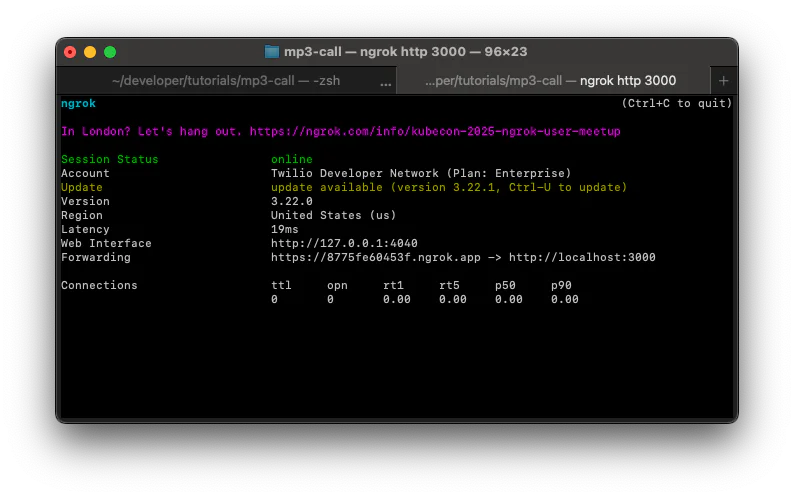
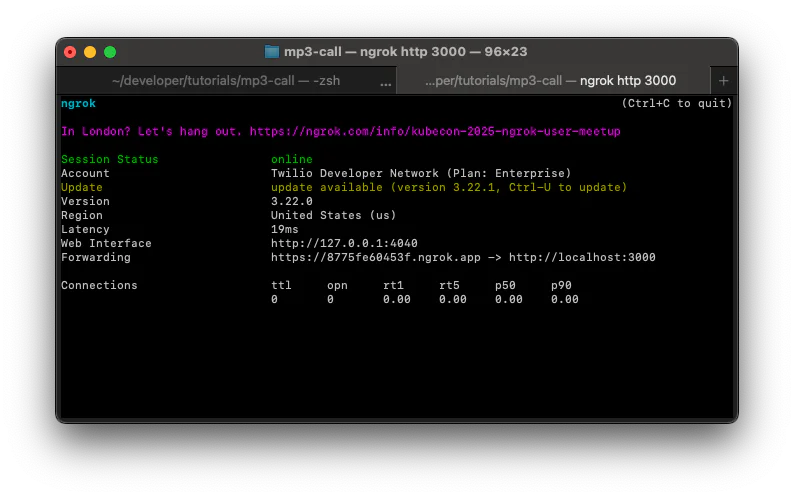
Copy the Forwarding URL in your terminal to use in the next section.
Connect Node.js application to Twilio number
You’ll now hook up your Node.js server to your Twilio number using the forwarding URL; this URL will forward all requests to your Node.js application.Navigate to the Active Numbers section of your Twilio Console. You can head there by clicking Phone Numbers > Manage > Active numbers from the left tab on your Console.
Now, click on the Twilio number you’d like to use for your phone service. Within the Voice Configuration section, select Webhook for the “ A call comes in”dropdown and then within the next textbox, enter your forwarding URL given by ngrok followed by "/mp3" (see below how the URL should look like).
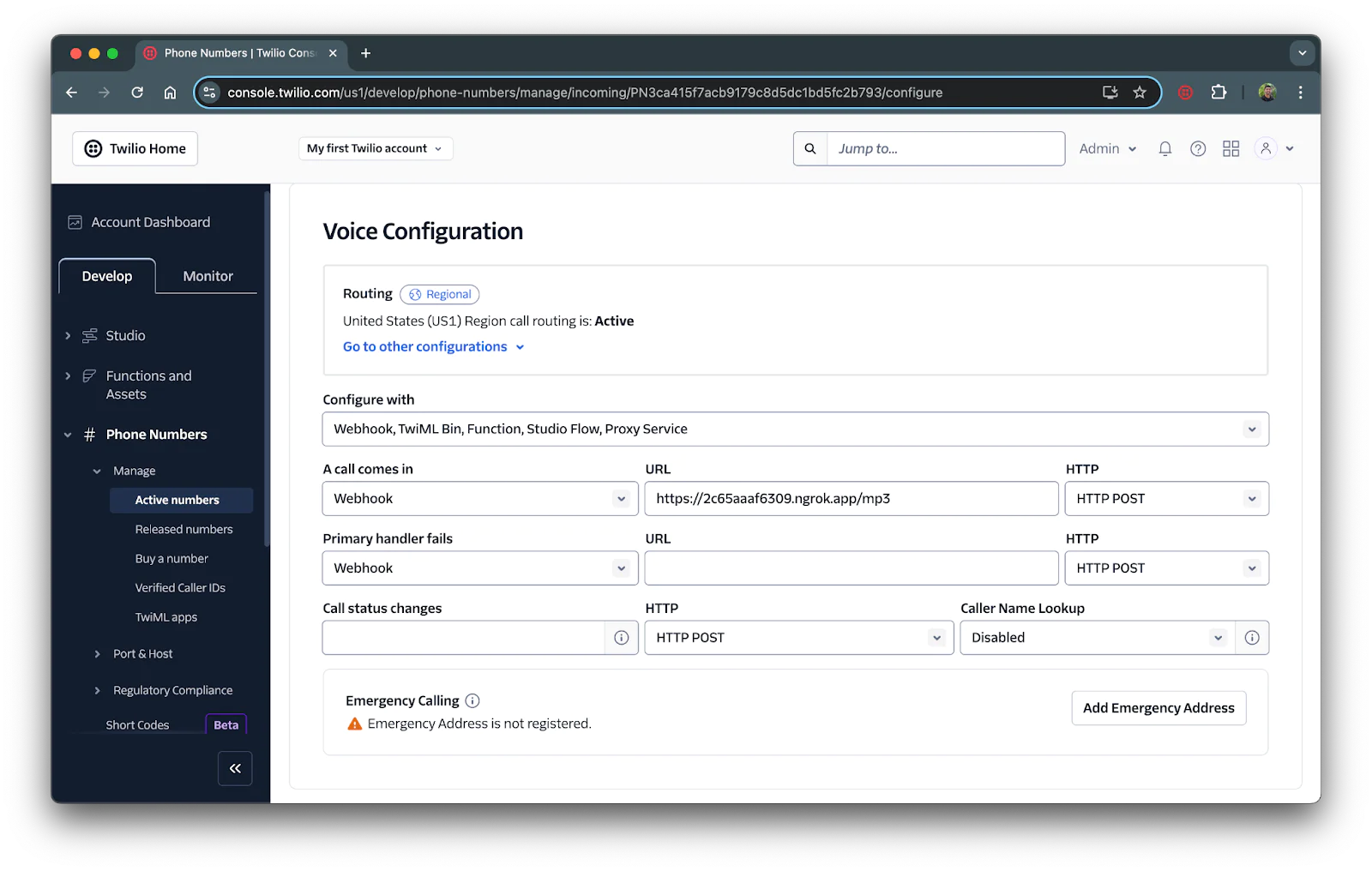
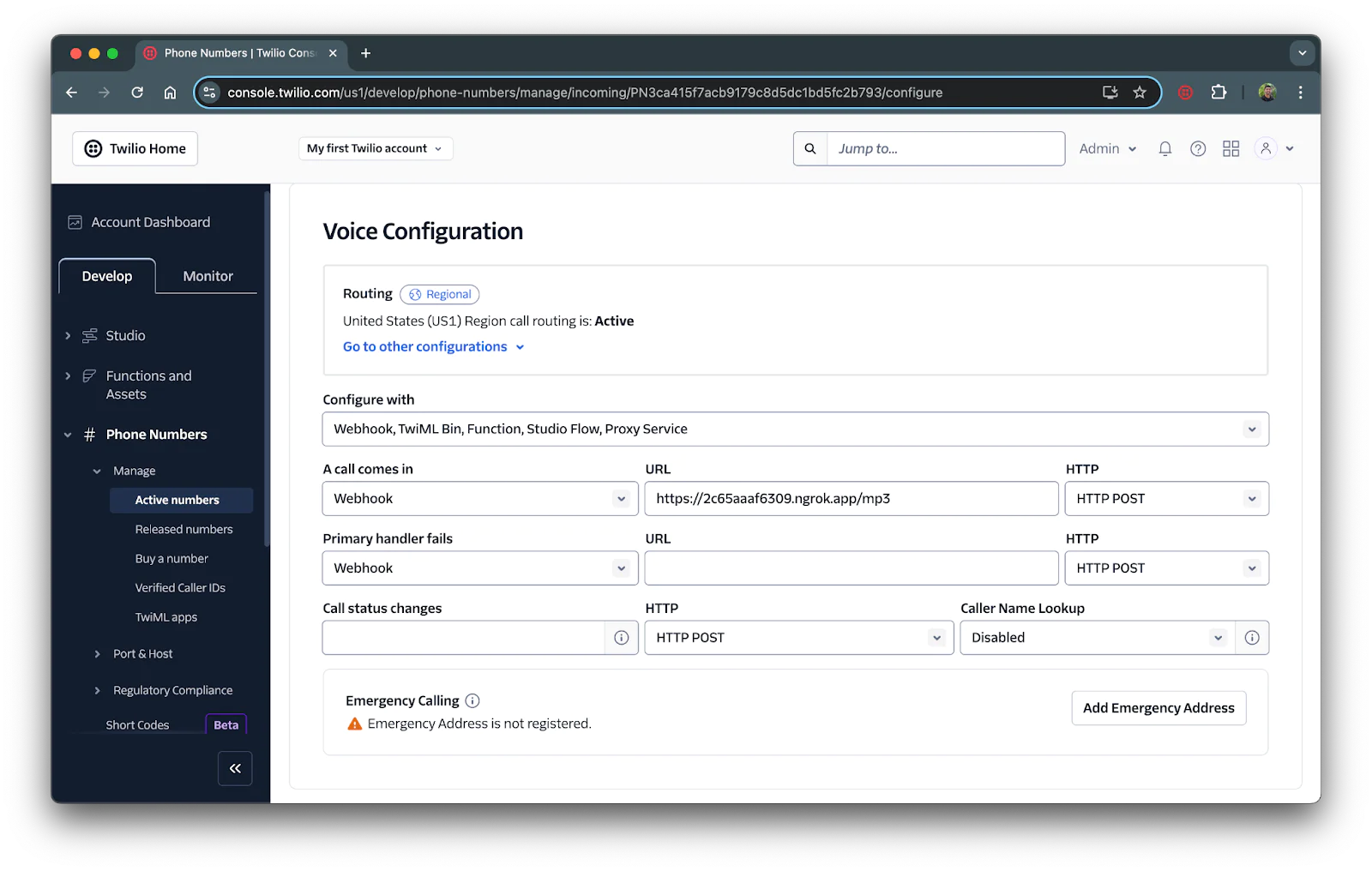
Once added, scroll down and click Save configuration.
Test the call
Your Twilio number will now be set to play the given URL. Call your Twilio number to test it out!
MP3 on Outgoing calls
Making outgoing calls with Twilio is less tedious than responding to incoming calls. For this section, you won’t need to start an Express server or create an ngrok tunnel (unless you’re hosting the MP3 file on your Node application).
Writing the code
Replace the code within index.js with the following (or add the code to outgoing.js if you need to keep index.js to host your MP3 file):
Replace the URL on line 3 with your MP3 URL and the phone number on line 8 with your number.
Test the call
Save the file and navigate to your terminal. Run the following command to run the file (use outgoing.js instead of index.js if that’s the file name you used):
Wait a moment and you’ll receive your MP3 call!
Conclusion
Now that you know how to play MP3 during your Twilio Voice calls, what's next? Try expanding the application by using the <Gather> verb to let callers choose which MP3 file to play; it’s like giving them a song on speed dial!
You can explore our other TwiML verbs and see what interactive interactions you can build for your voice applications. You can use the <Record> verb to build a song identifying voice service or you can use the <Say> verb to convert text-to-speech with an Amazon Polly or Google voice.
Happy Building!
Dhruv Patel is a Developer on Twilio’s Developer Voices team. You can find Dhruv working in a coffee shop with a glass of cold brew or he can either be reached at dhrpatel [at] twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.