Creating a Emergency Outage IVR Response in Twilio Studio
Time to read: 10 minutes
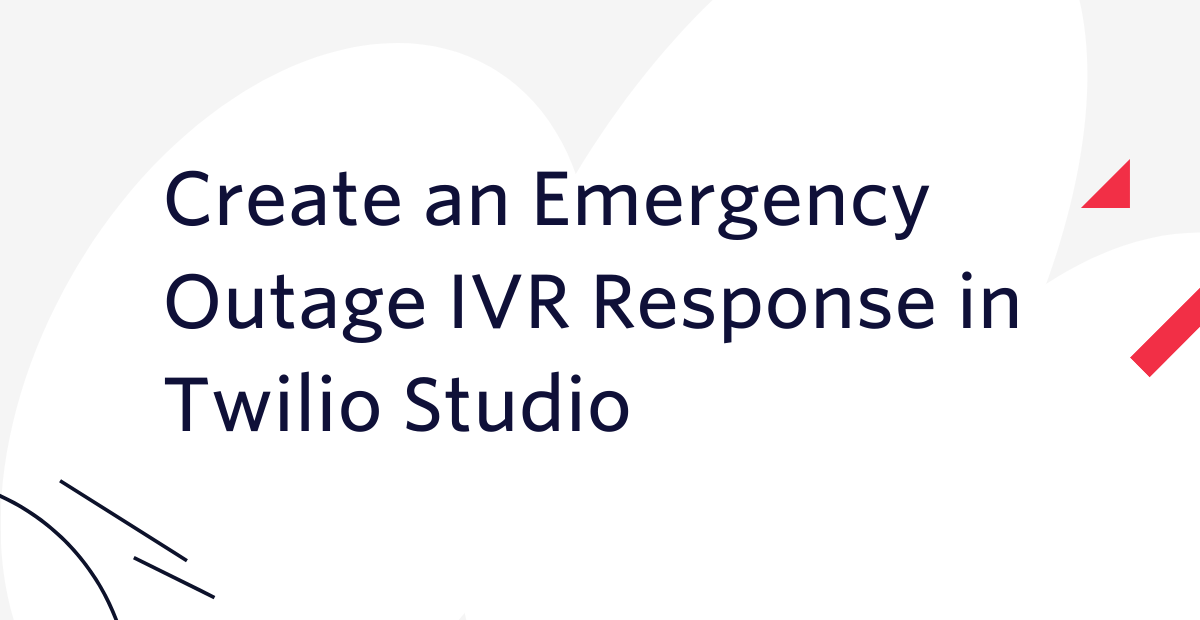
If you are part of a team that ensures the smooth operation of a contact center, you may be tasked with planning for the unknown. Surprises or rapidly changing situations are times when the value of a cloud-based voice solution shows through. In the case of an emergency — such as a weather-related closure, a facilities problem, or a personnel challenge — you may need to remotely activate a temporary change to your voice responder.
In this article, I will use Twilio’s IVR solution to create an emergency outage message that can play for incoming callers, notifying them of an unmanned queue. This message can save customers time and frustration and give you space to ensure the health and safety of your team. If you follow along with me through the steps I present here, you’ll have a working outage message for your selected Twilio phone numbers.
Tutorial prerequisites
To code along with this post you'll need a free Twilio account; sign into an existing account or sign up here.
In this blog we will use the following services:
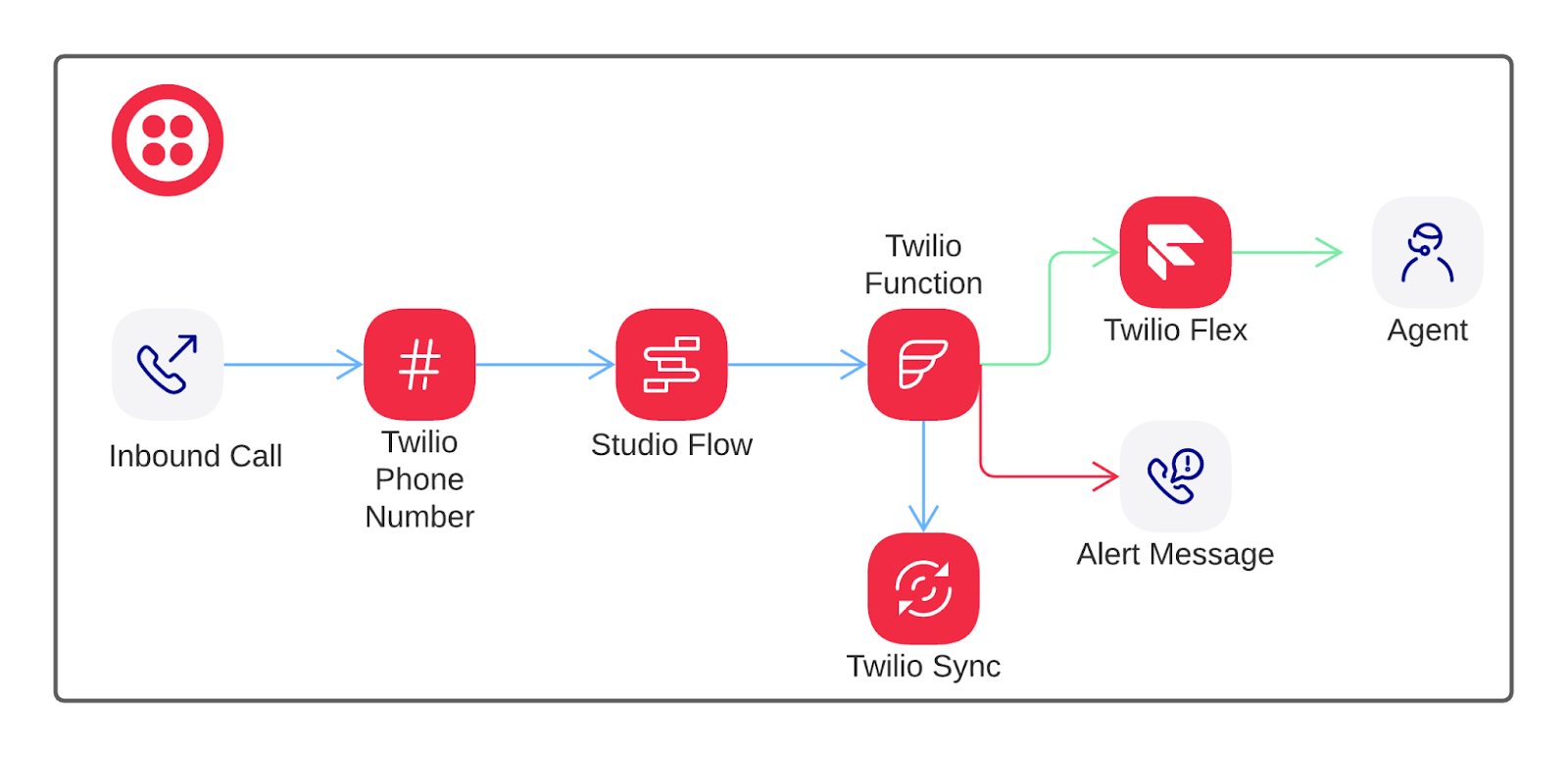
In this scenario, a call comes into a Twilio number and goes through a Studio Flow. The Flow runs a Function that reads a Sync Map to determine if there is an outage for a particular call center location. If there is no outage, the call can proceed. If there is an outage, an alert will play to the customer and the call will conclude.
SYNC
To set up Sync to use a Map to store our contact center outage data, I used the following steps. Click on Explore products, scroll down to the Developer Tools section and select Sync:
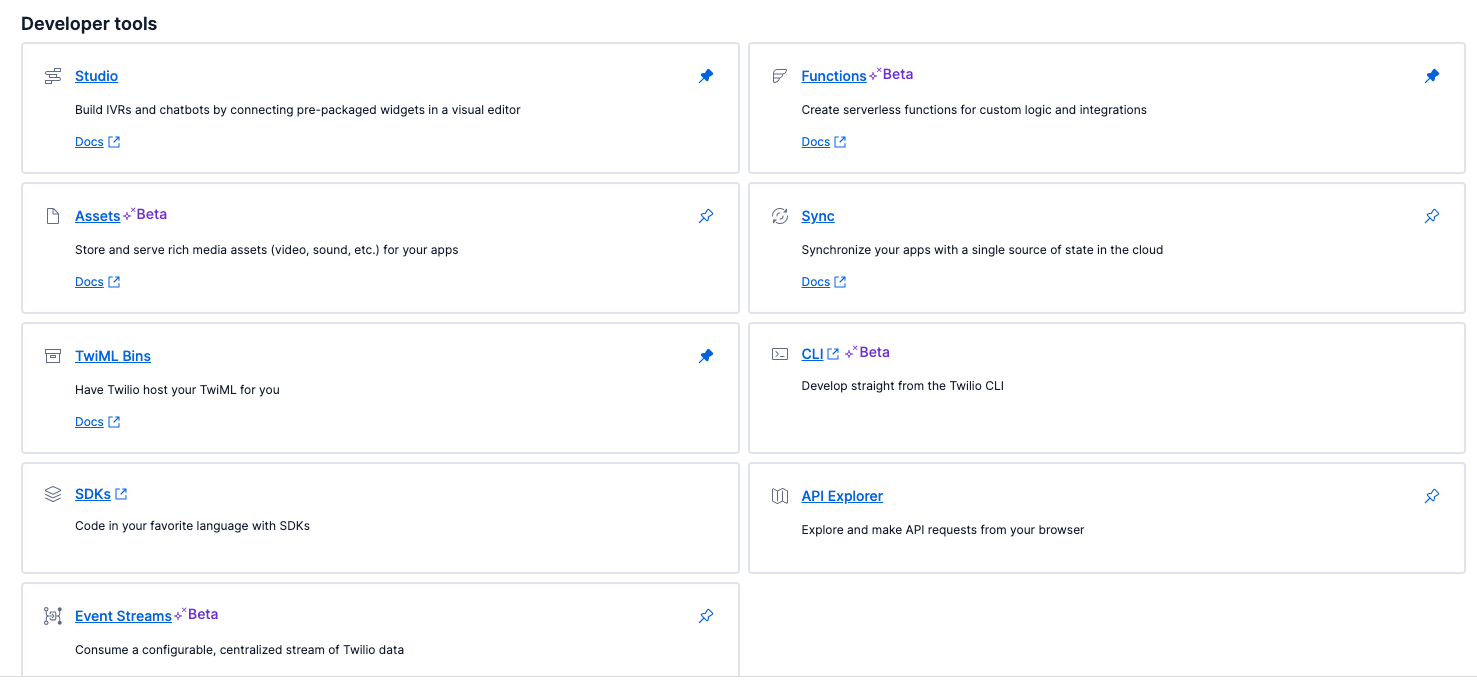
Note: You can pin Sync and Functions to your Navigation Pane for future use (by clicking on the pin in the Developer Tool box in the Sync section).
On the Get started with Sync page and click View Sync Services:
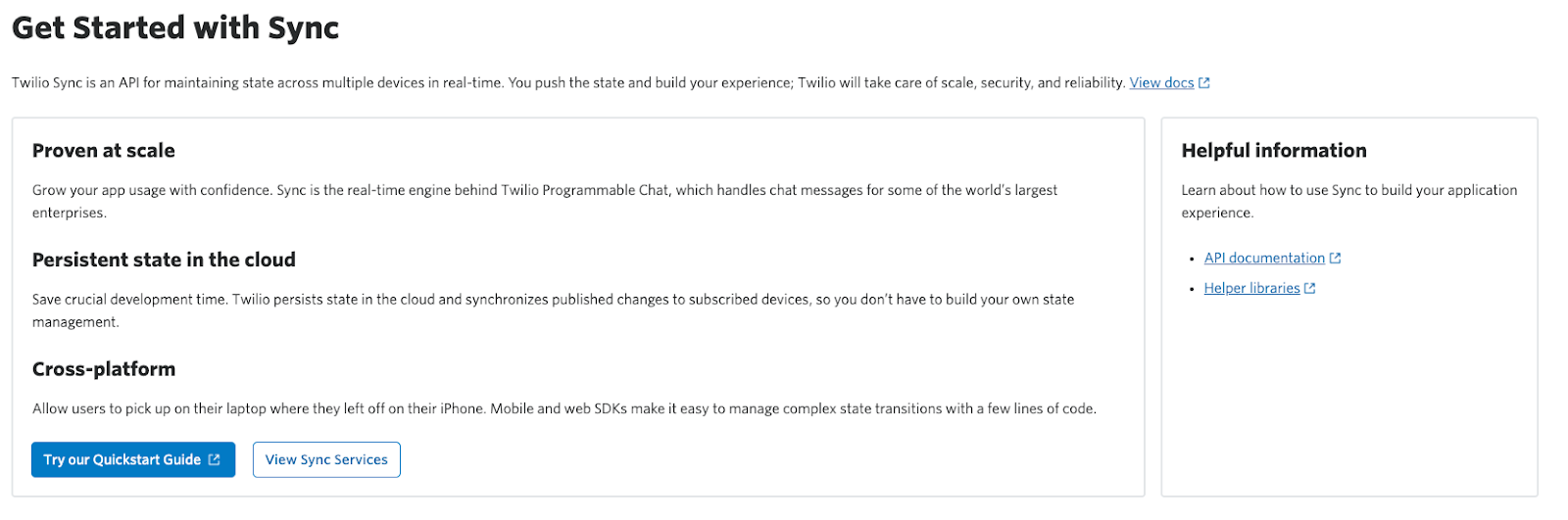
Select Create New Sync Service:

Give your new Service a name. I used EmergencyOutage. Click Create:
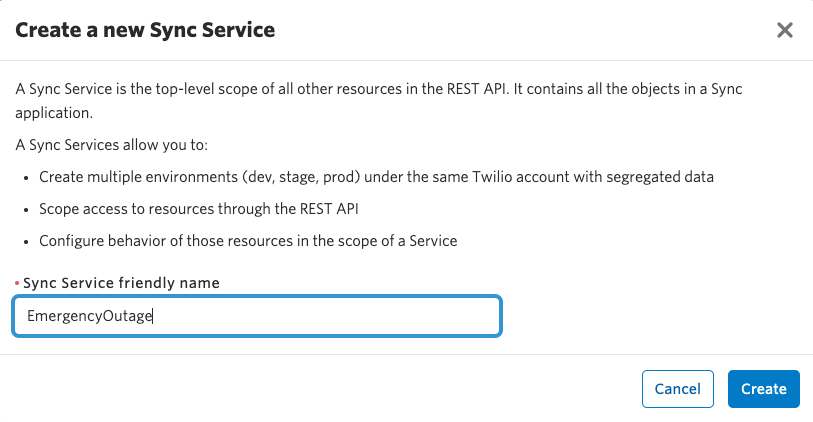
You have now created your service. Take note of the Sync Service SID as you will need this later. Click on Maps from the navigation menu:
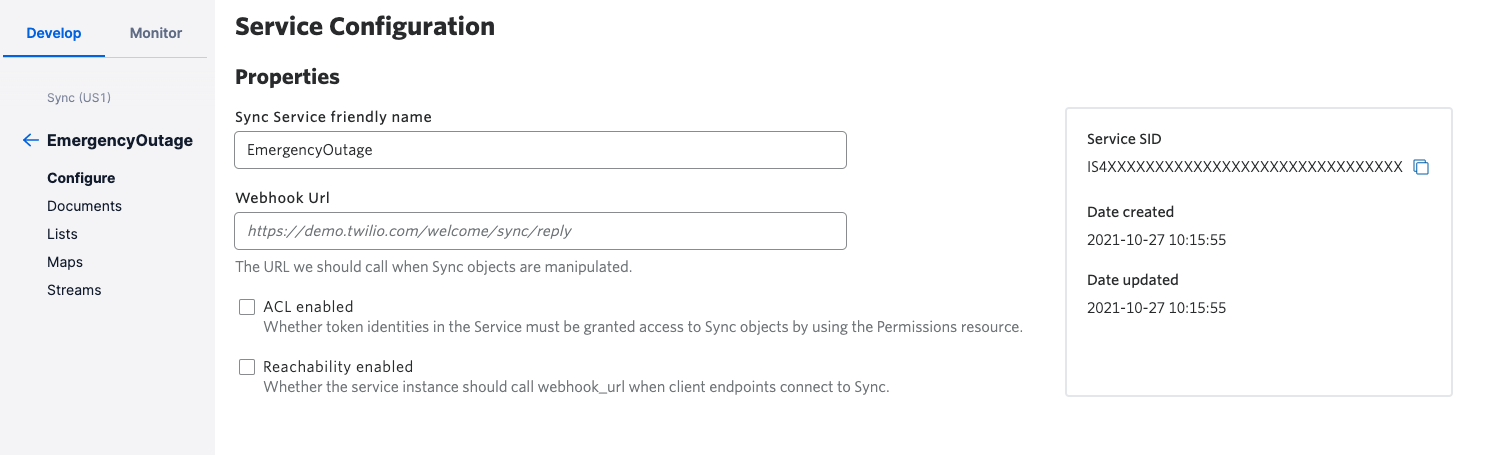
Select Create new Sync Map:

Give your new Sync Map a name. I used Outages. Click Create:
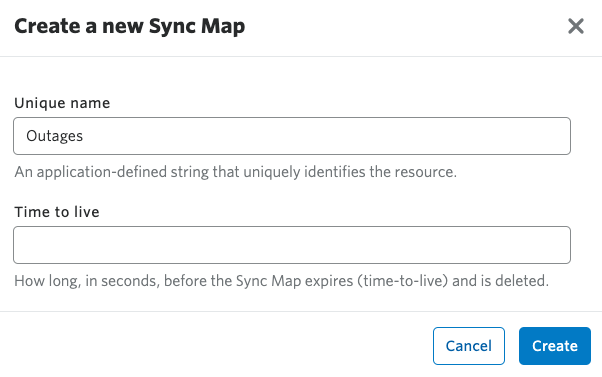
You have now created your Sync Map. Take note of the Sync Map SID which you will need later for the Function. Click on the name of your Map (Outages):
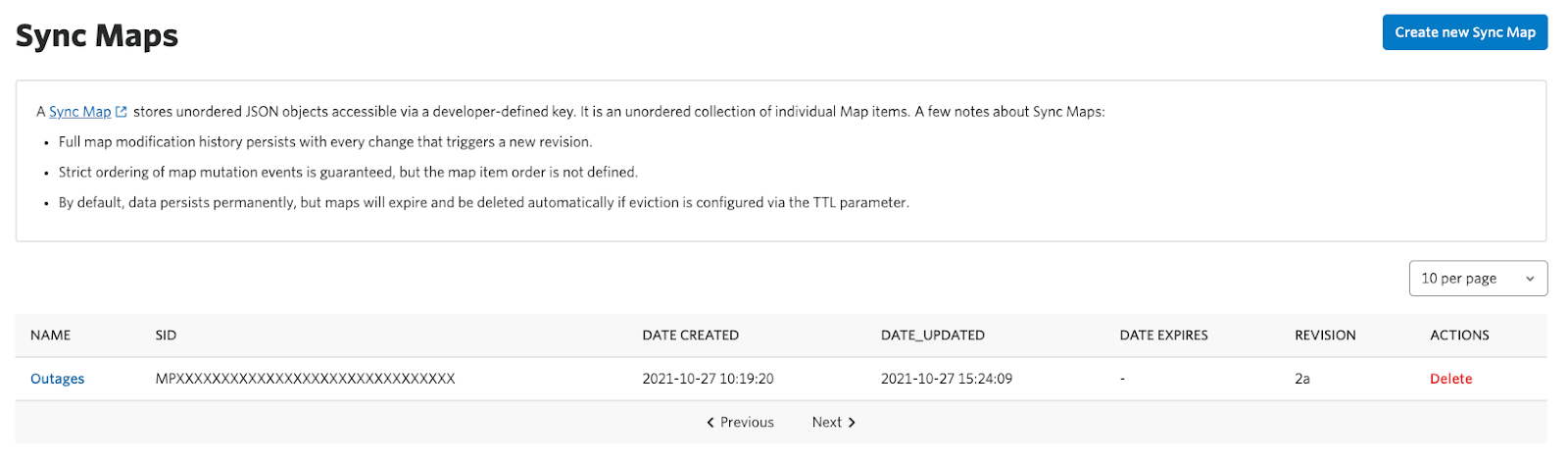
Click on Create New Map Item:
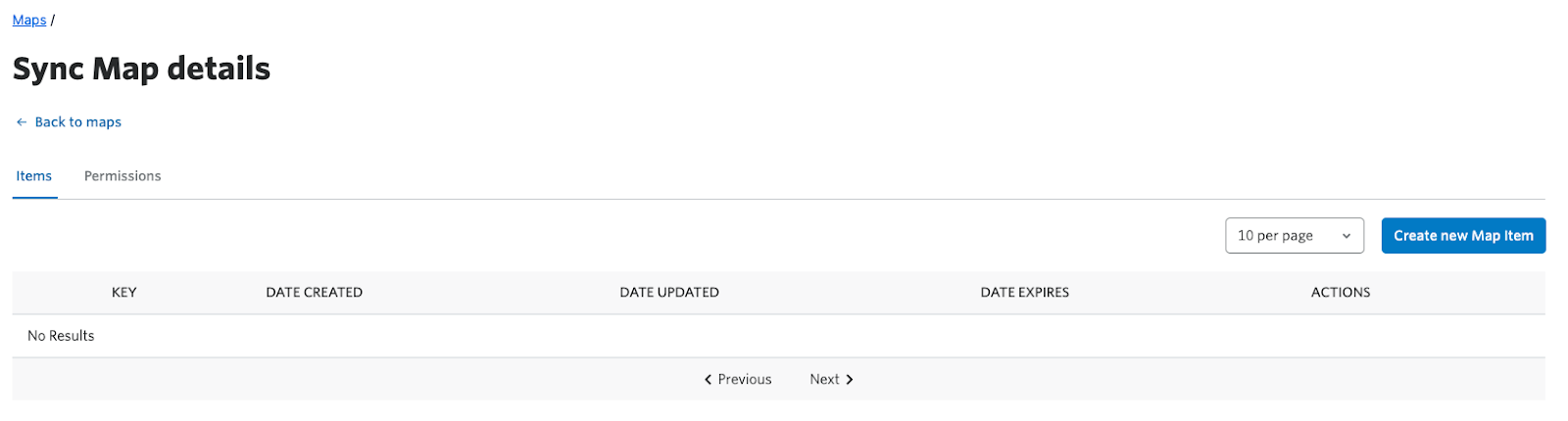
In the new item, the Sync Map Key is the physical location or identifier where your agents work; this is a name that makes sense to your environment and is typically the place affected by the outage. In the data field, set a JSON object to be {"Enabled": false} as you want the call center location to be on normal operation (i.e. no emergency).
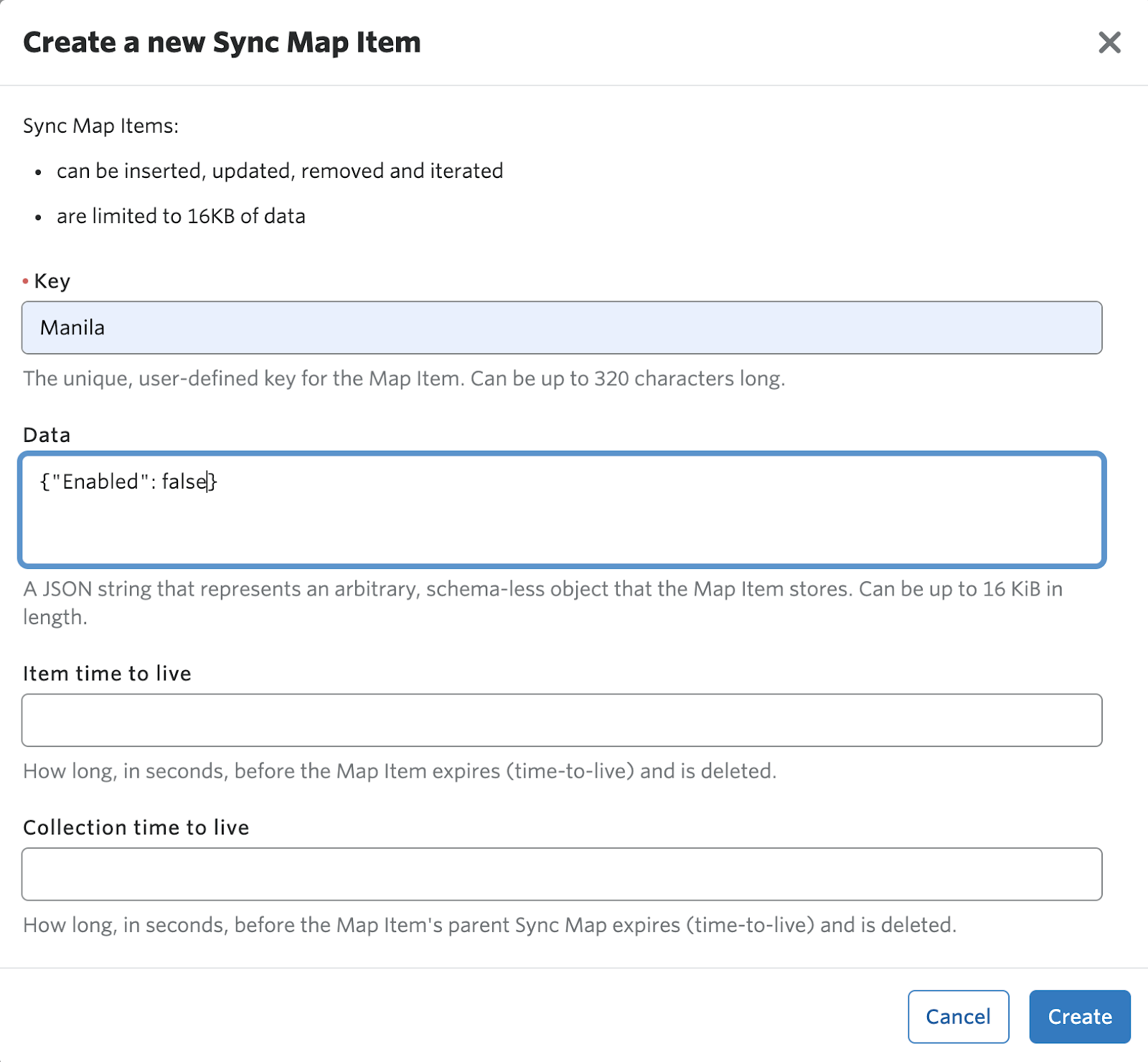
Note: The Sync Map Key is case-sensitive and you will need it for the Parameters of your Function in your Studio Flow in the next steps.
You have now created your new Sync Map item:
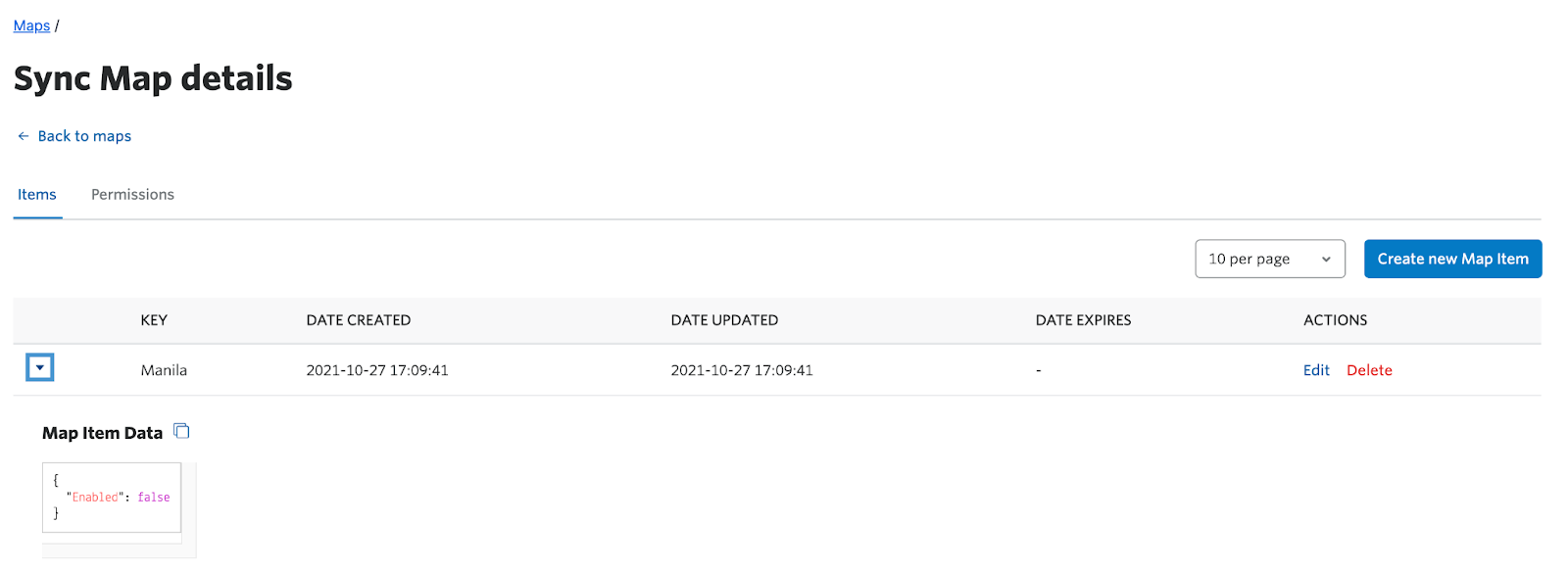
This sets up your first call center Sync Map to be under normal operation.
Function readUpdateOutage
In this section you will outline the Function you need to read your newly created Sync Map. This function will also be used in later steps. I will call it readUpdateOutage as that is its intended purpose. In the Navigation Pane expand Functions and select Services:
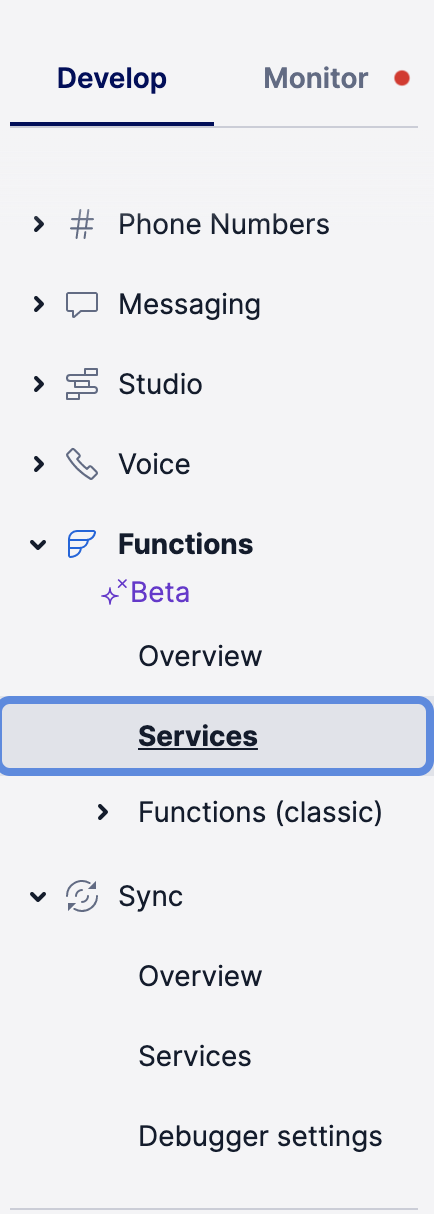
Click on Create Service:

Give your new Service a name. I used Emergency Outage. Click Next:
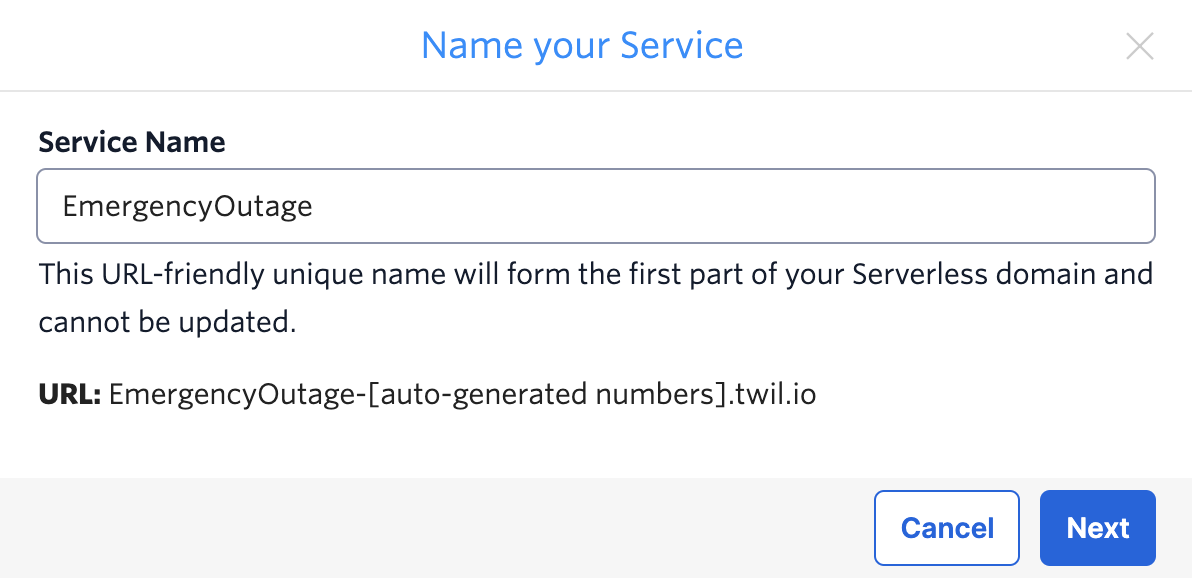
Click on Add + and select Add Function:
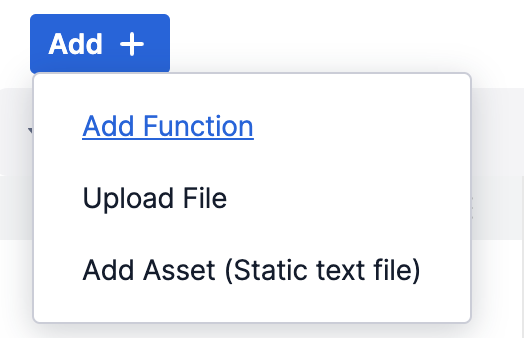
Enter your Function path. I used readUpdateOutage as that is relevant to what the Function does:
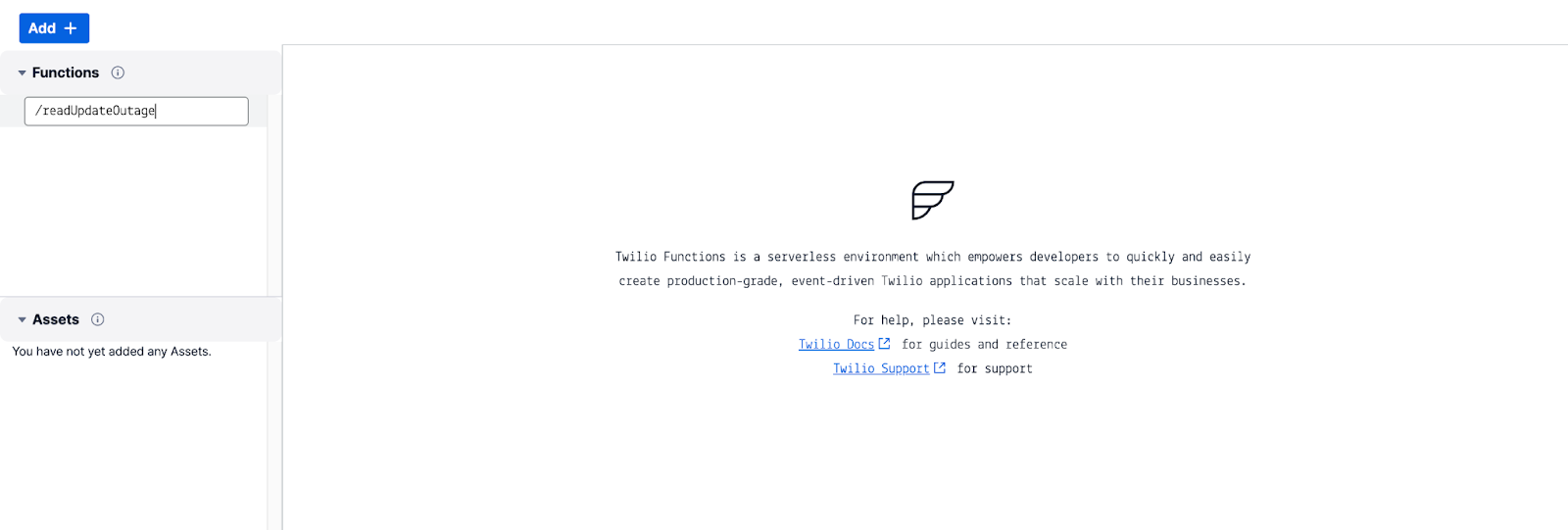
Leave the Function as Protected, and paste in the following code:
This code reads or updates the Sync map. It reads to check if there is an outage and updates the Sync Map to add or cancel an outage.
Click on Save:
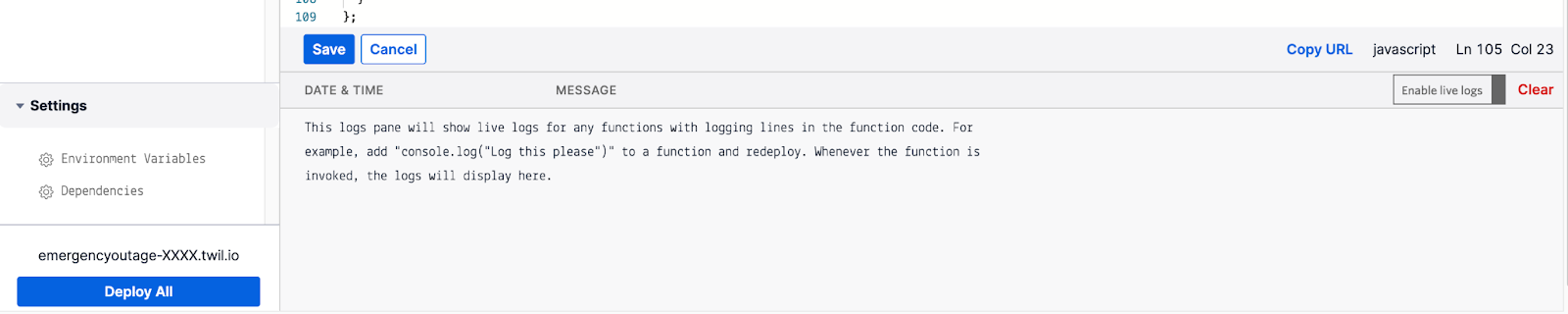
The Function is still not ready to deploy. You need to add the Environment Variables first. Select Environment Variables from the Settings section as shown above. Enter syncServiceSid as the Key, and use the noted Sync Service SID from earlier (in the format of ISXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX) then click Add:

Enter mapId as the next Key and use the noted Sync Map Id from earlier (in the format of MPXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX) and click Add:
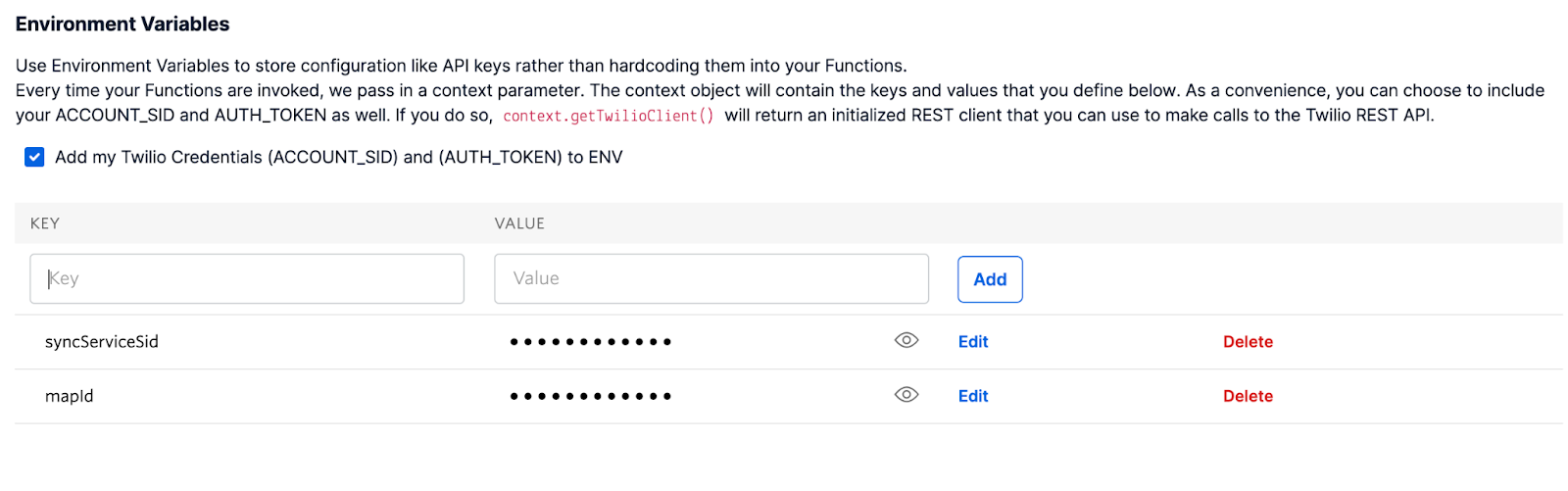
Note: Keep the Add my Twilio Credentials (ACCOUNT_SID) and (AUTH_TOKEN) to ENV ticked for use in the Function.
For this Function you will use a Module called Moment to make it easier to determine dates for your outages. You need to add this Module to Dependencies. Click on Dependencies in the Settings section:

Enter moment as the Module, leave the Version as blank, and click Add:
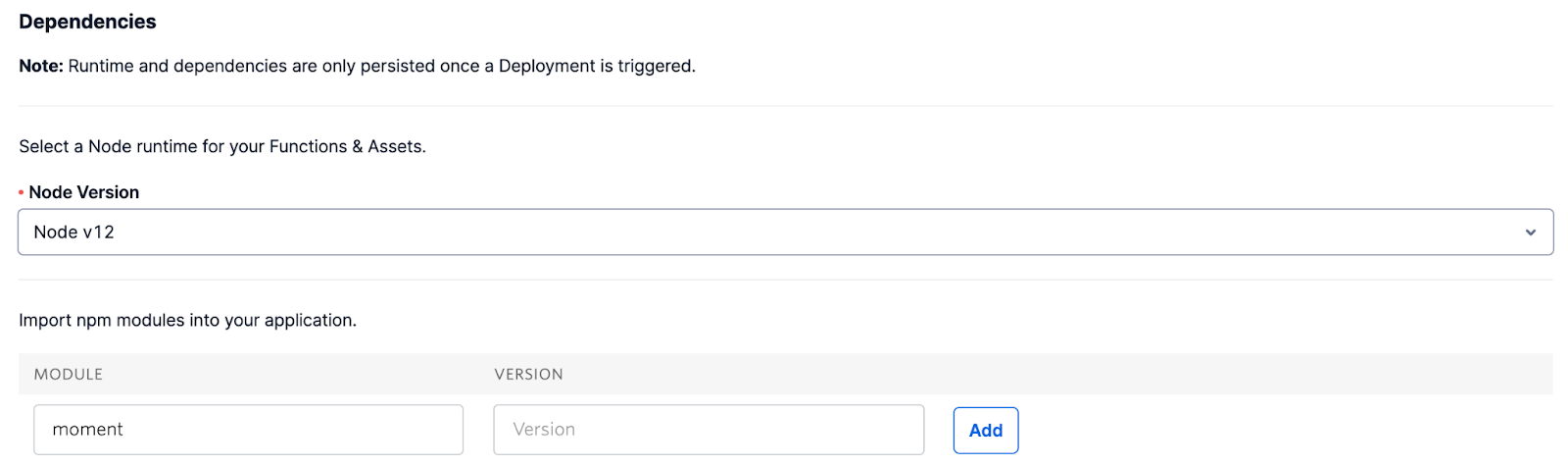
And now you are ready to Deploy All:

Your new Function is ready to be used in your Studio Flow.
Demo Flow
The following Studio Flow is a simple demo to test your outage as read from your Sync Map. The first step is your Check Emergency Function to readUpdateOutage. It requires some Parameters to perform properly. The Parameters include location which should be set to your call center physical location or identifier (which will match the Sync Key we created earlier). The second step is your operation for the Function to perform, which is set to read. The EmergencySplit step checks the results of the Function to determine if there is an outage:
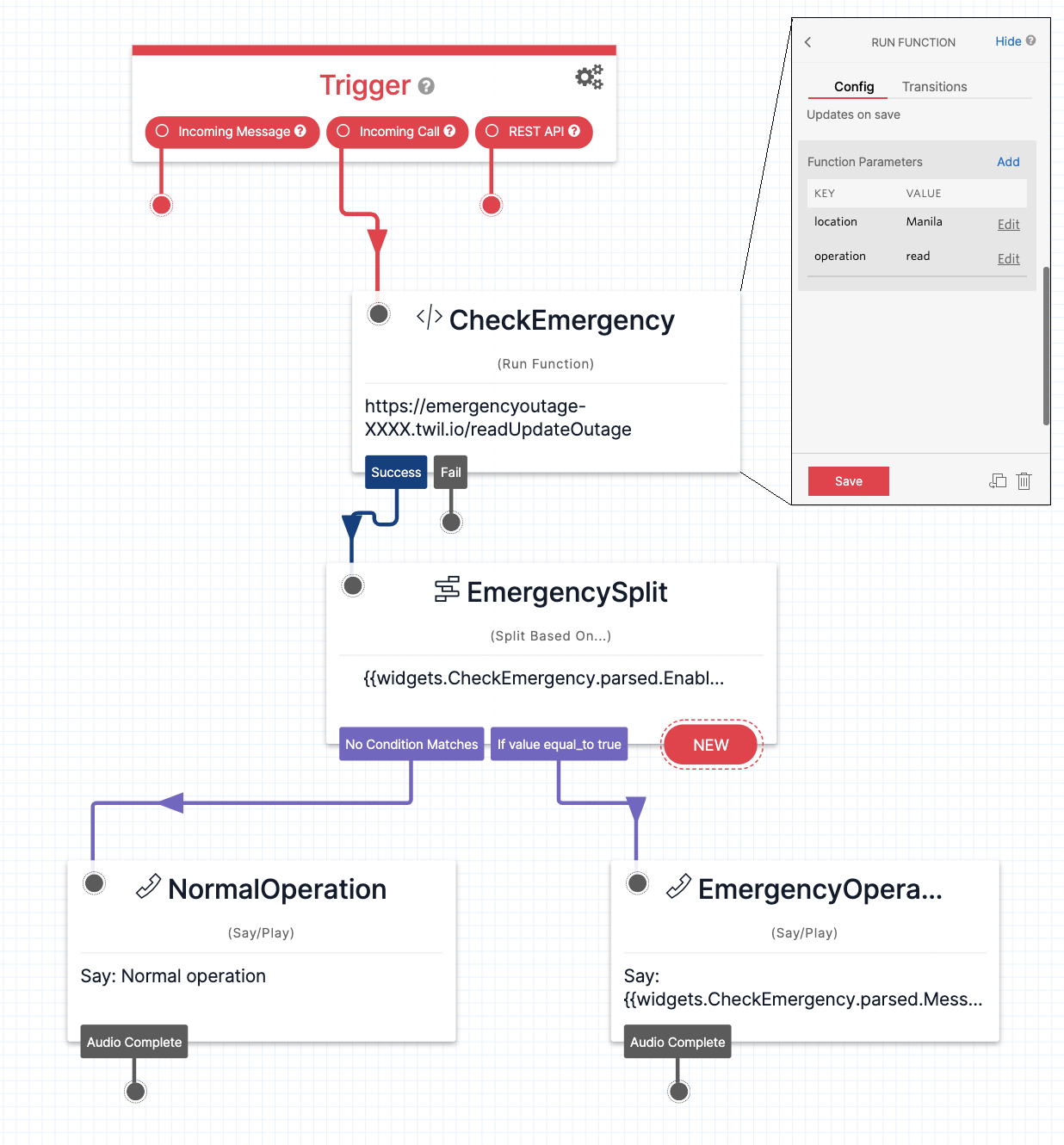
Demo Flow JSON
Instead of recreating the Studio Flow as shown, you can Import a JSON because copy-and-pasting JSON code is easier than dragging and dropping Twilio Studio widgets. To do this, open Studio > Flows from the Navigation Pane and click on the Plus icon.
Give your new Studio Flow a name. I used EmergencyDemo. Click Next.
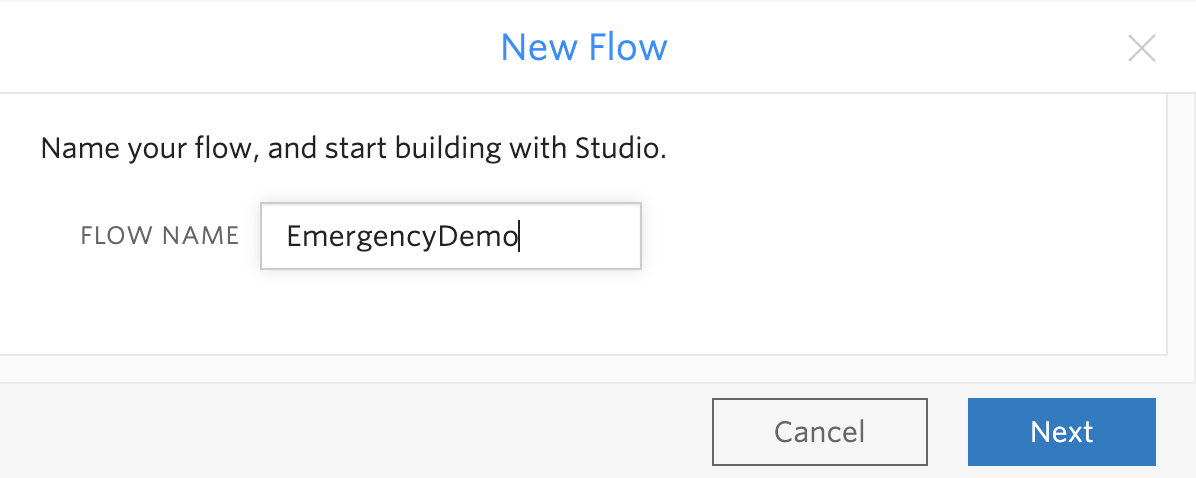
On the Template window, scroll to the bottom and select Import from JSON:
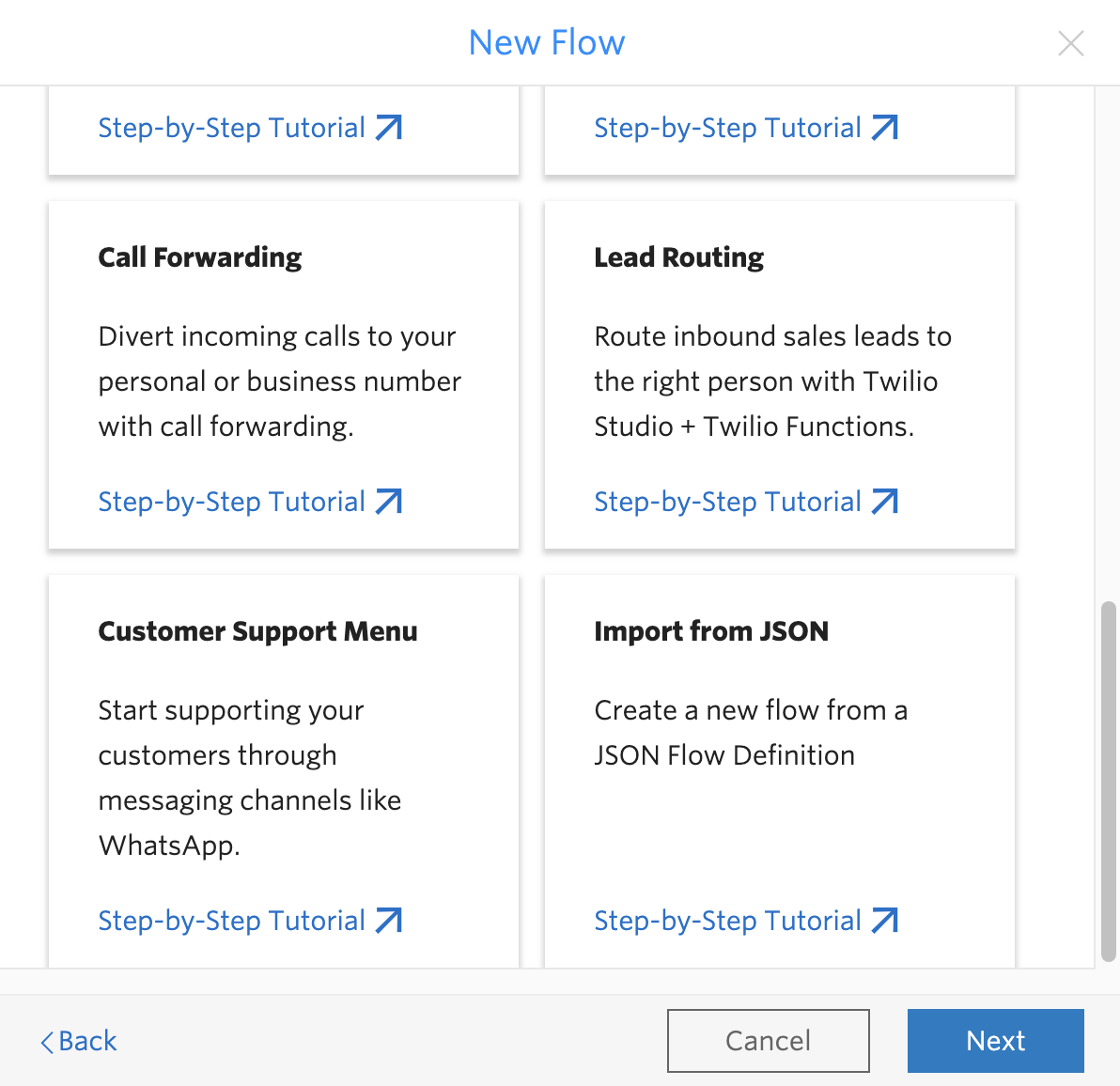
On the JSON Flow definition window, remove the existing {}, paste in the following JSON, and click Next:
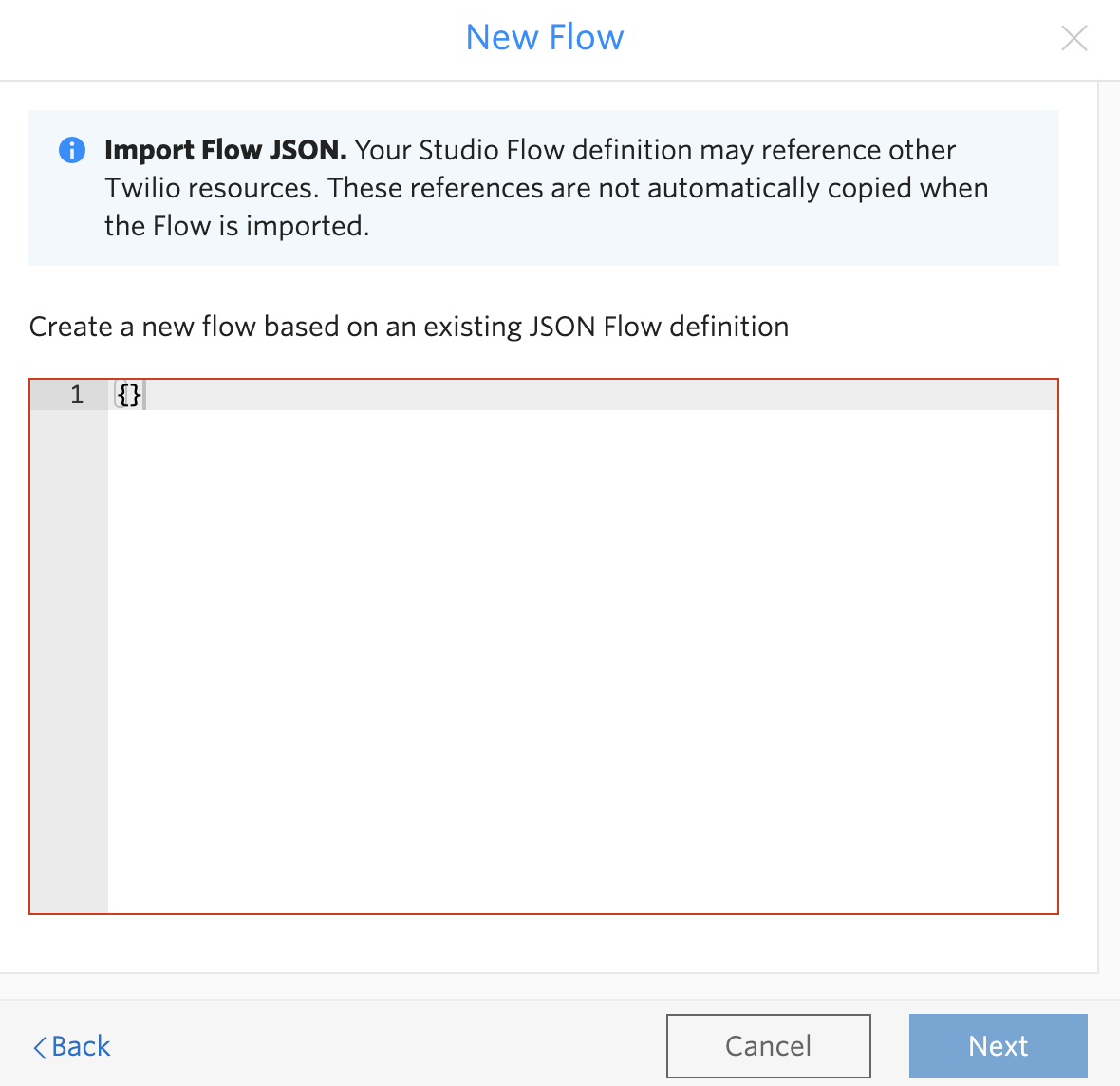
This JSON object is the call flow as pictured above. It runs a Function to check for an emergency. If there is no emergency, it proceeds to normal operation. If there is an emergency, it plays the emergency message.
Now you see our EmergencyDemo flow, and you can see an exception (red circle with exclamation mark) on the CheckEmergency Function block of your flow:
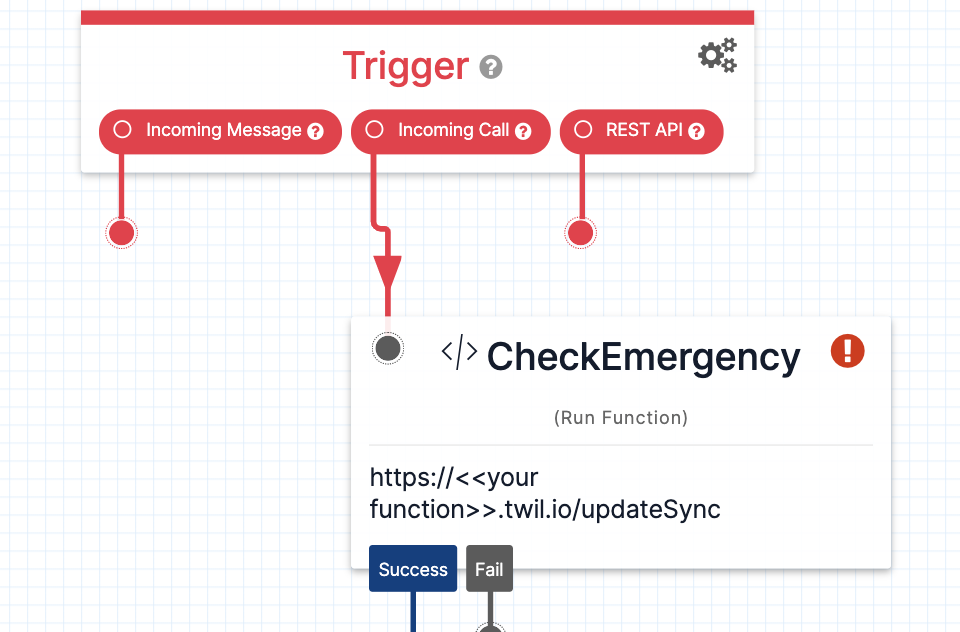
This exception happened because you need to update the block with the Function you created. Simply click on the CheckEmergency block, and configure the Function with the Service = EmergencyOutage, Environment = ui and Function = /readUpdateOutage. Then click Save as shown:
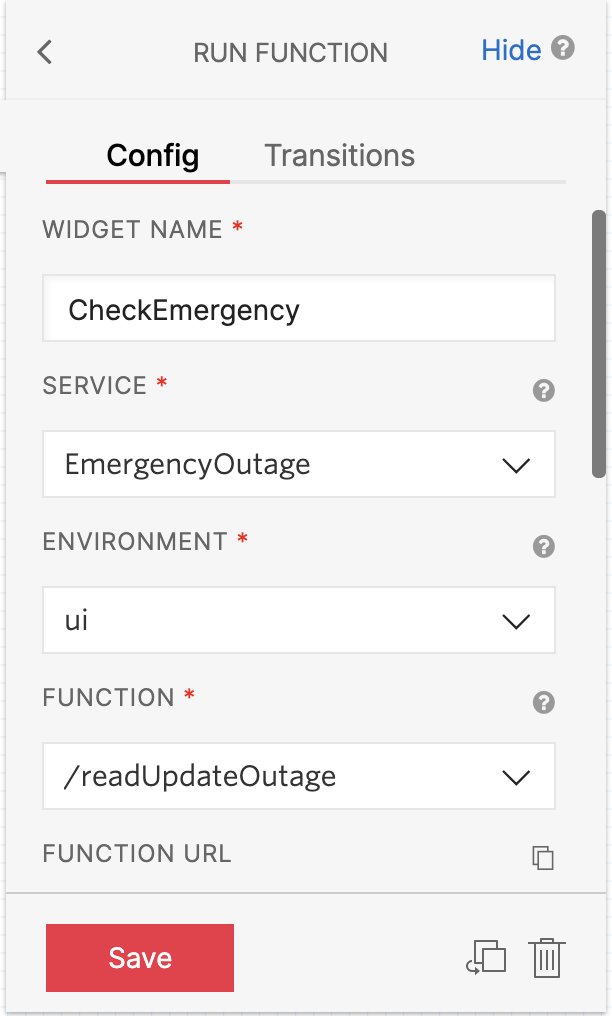
Double check your location in the Function’s Parameters section, and make any changes to the location necessary:
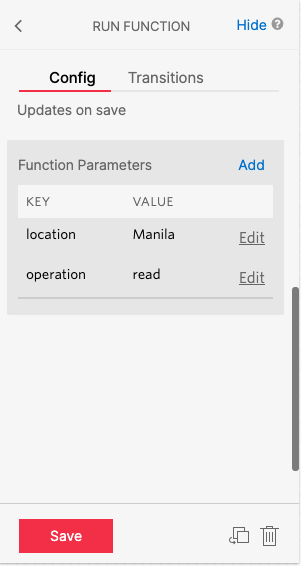
Then Publish the Flow:

Your Studio Flow is now ready to Assign to a Phone Number.
Assign Flow to Phone Number
In the Navigation Pane, select Phone Numbers > Manage > Active numbers:
Select the Number you wish to use with your EmergencyDemo flow (or optionally buy a new number) and scroll down to the Voice & Fax section. In the A CALL COMES IN section, select Studio Flow, select EmergencyDemo, then click Save:
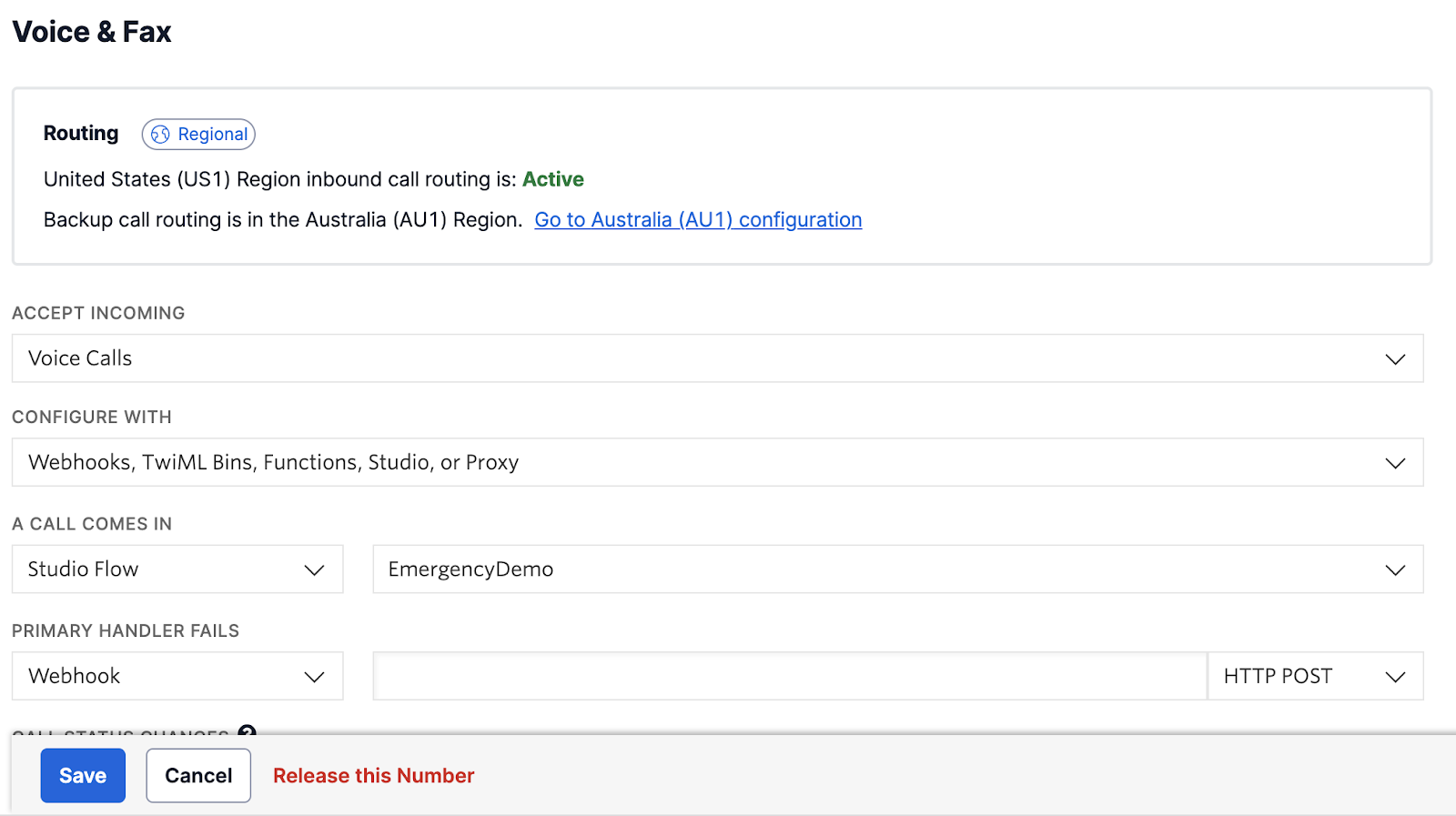
Testing the solution
In order to test the solution you can simply call your number you just configured with the Studio Flow. You should hear “Normal Operation” and then the call will hang up. In a real-world example you would then provide a menu of phone options you would like your customer to hear and interact with.
Let’s set an outage:
Using the Navigation Pane open the previously created Sync Map (Outages) and select Edit for the Map Item (Manila):
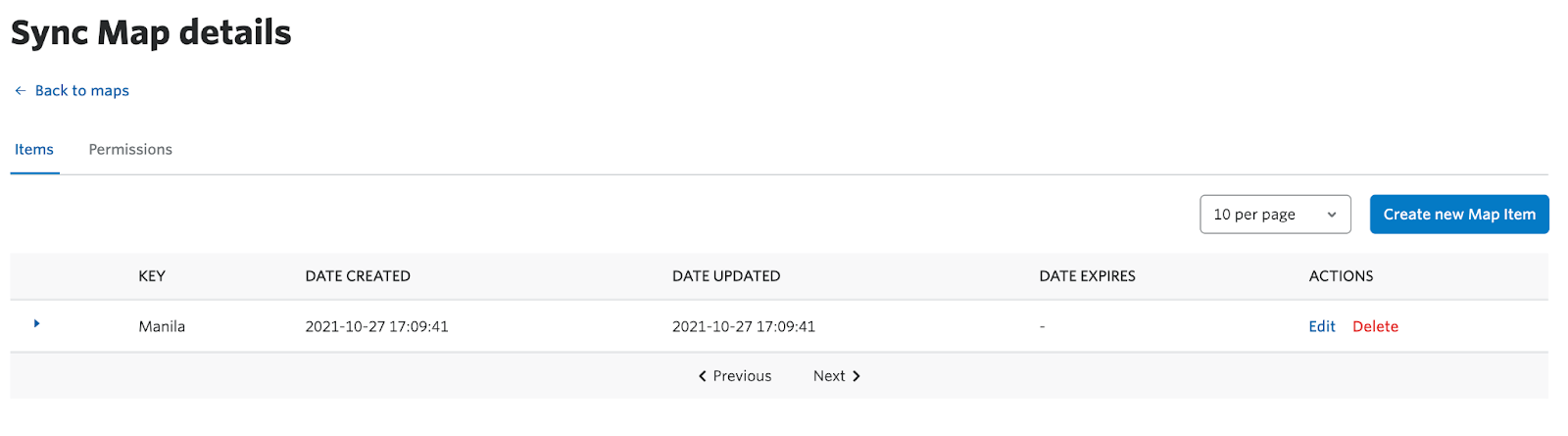
Update the Data field with the following and click Save:
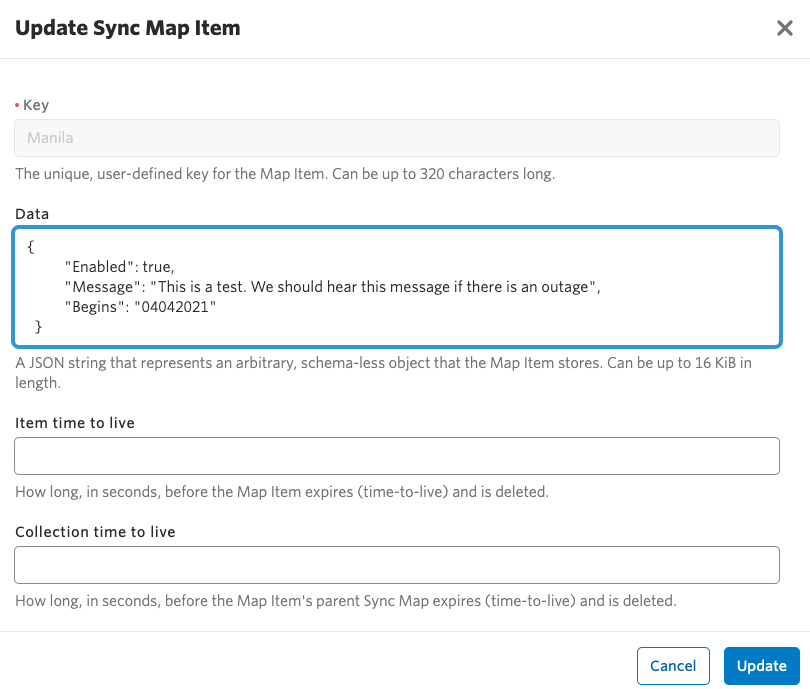
Note: If the Begins date is in the future, the outage will not be enabled.
Call your number again with the Studio Flow. You should hear “This is a test. We should hear this message if there is an outage” and then the call will hang up. In a real-world example you would then configure your intended response such as voicemail, or re-queueing the call to another location.
Conclusion
This solution makes it easy to set an outage and play a message for your callers after a few easy steps. It helps alleviate the frustration of waiting in an unmanned queue by disconnecting the call immediately, or setting expectations on wait times, or even re-directing the queue to give callers the best experience.
Optional Part 2: Creating a Remotely Activated Emergency Outage Message in Twilio
In the event of an emergency, you may not have the time to update the Sync Map to enable the emergency. Having a remote way to update this message saves time in these events. For this option you can use SMS messaging to enable or disable the emergency outage remotely. A correctly formatted message from a recognised number is all you need to perform this task.
To enable an Emergency you will send a SMS message in the following format:
<<Location>>: Message
For example:
Manila: Due to unseen circumstances, our call center is unavailable. Please try again later.
If the number is on the SMS approved list, you will receive a message asking when you want the outage to start to which you can reply with a date in the format of DDMMYYY (07112021 for 7 November 2021). This also allows us to schedule a message in the future for such things as public holidays.
If the date entered is not valid, the user is prompted again and reminded about the format.
If the date is in the past, they will receive an SMS like ***Warning: Start Date is in the past*** Outage message for <<Location>>: <<Message>> starting on <<Start Date>>
If the date is in the future, they will receive an SMS like ***Warning: Start Date is in the future, no outage will be enabled until that time*** Outage message for <<Location>>: <<Message>> starting on <<Start Date>>.
To remove the outage we simply message <<Location>>: remove (e.g. Manila: remove) to disable the message.
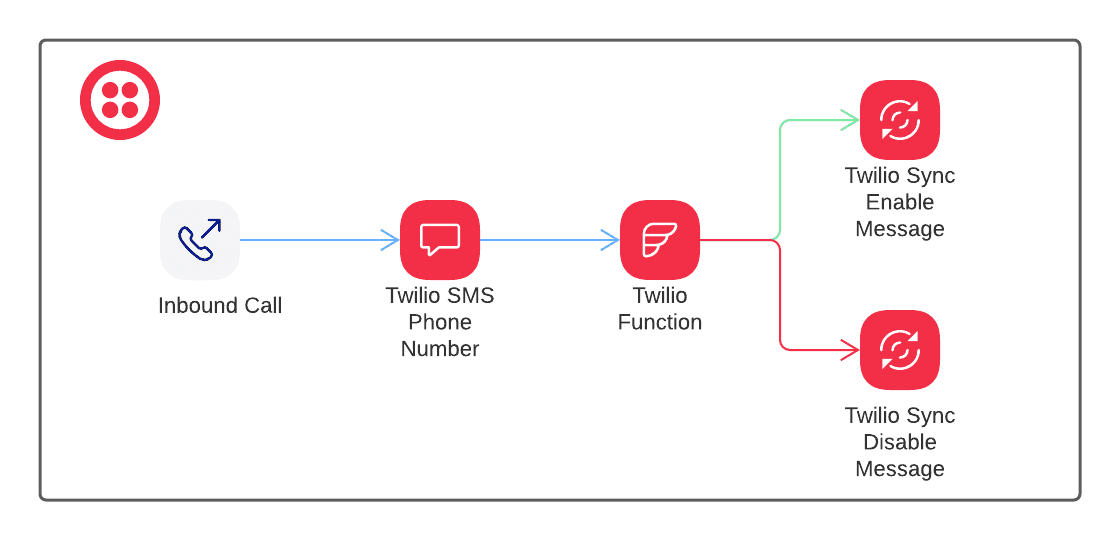
Function splitLocationMessage
In this section you will outline the Function needed, check the incoming SMS, and break it down into Location and Message. In the Navigation Pane expand Functions and select Services.
Select your EmergencyOutage Service:
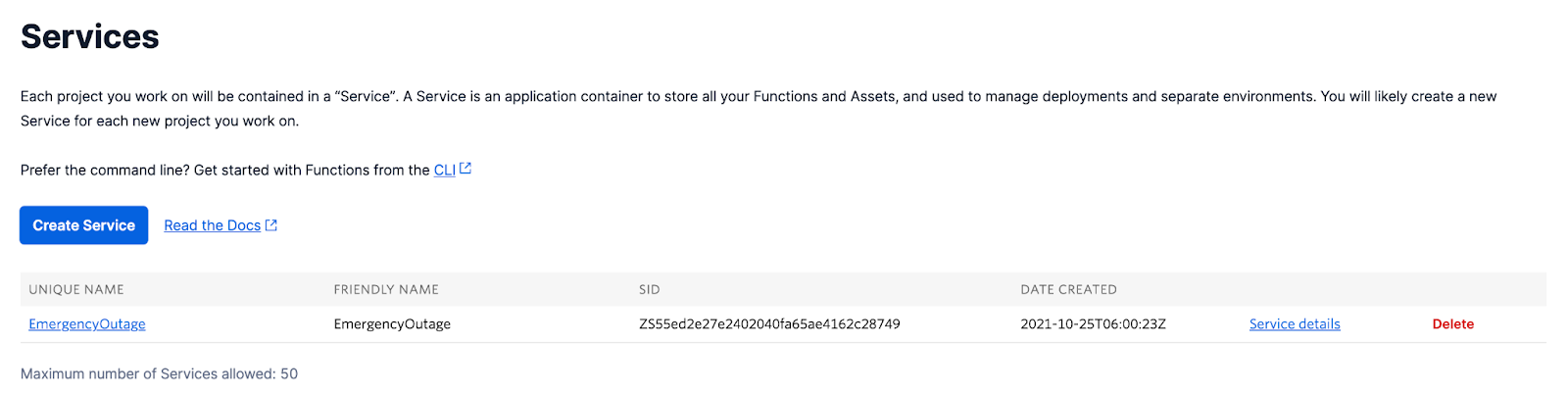
Click on Add + > Add Function and provide /splitLocationMessage as a Function Path:
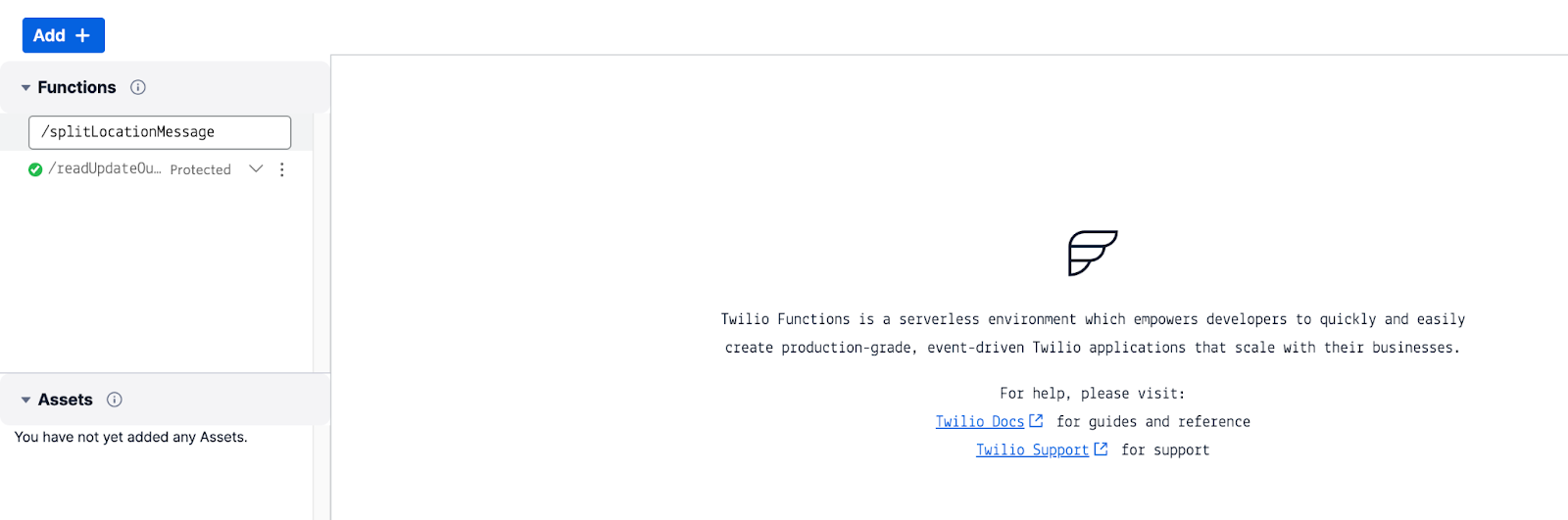
Leave the Function as Protected, and paste in the following code. Then click Save:
This code looks at the SMS message and splits it into 2 parts (separated by the colon ":"). The first part is then assigned to location and the second part is assigned to the message. Both parts return to the flow.
Function checkDateFormat
Like the previous task, you will create a new Function in your existing Service. Click Add + and use /checkDateFormat as the path:
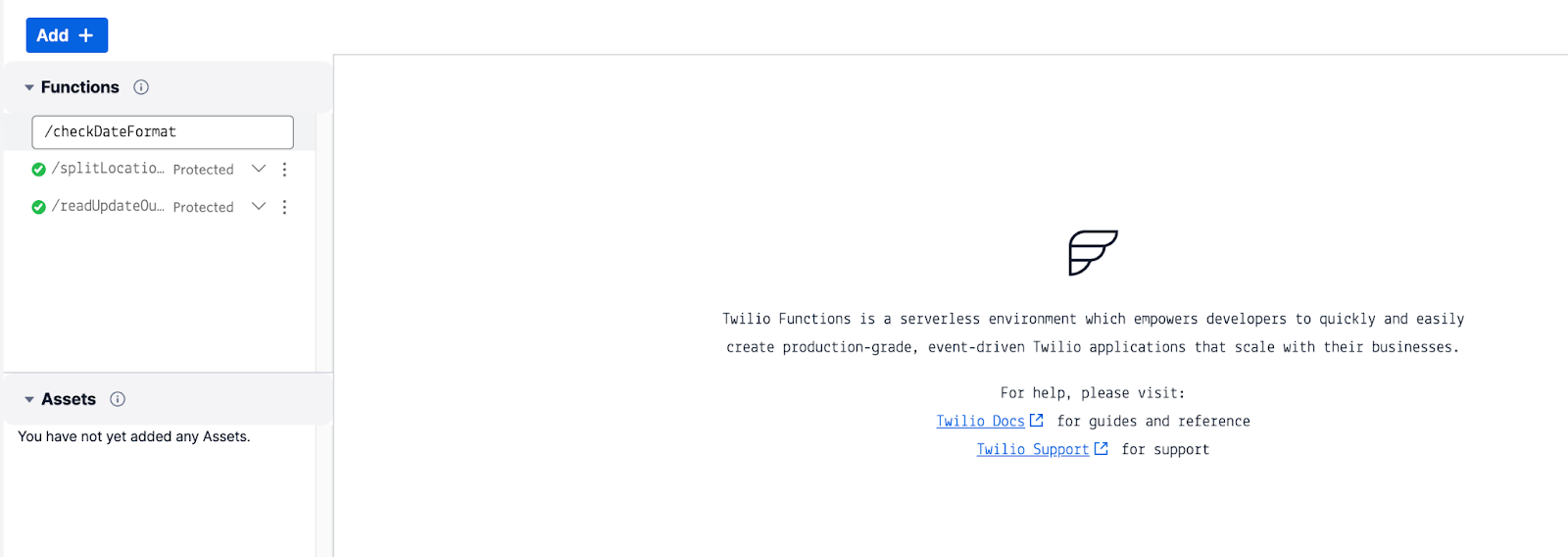
Leave the Function as Protected, and paste in the following code. Then, click Save:
This code checks the date to make sure the date is a valid format. It also checks if the date is today, in the past, or in the future, then the details pass back to the flow.
Click Deploy All:
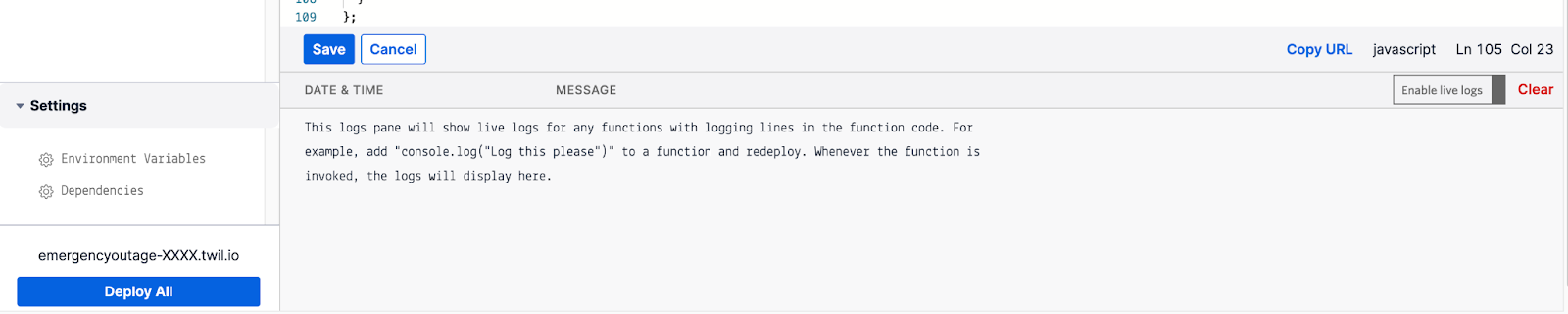
Your Functions are ready for the Studio Flow.
Outage Flow
The outage flow pictured below will enable you to trigger and remove an emergency outage with a text message.
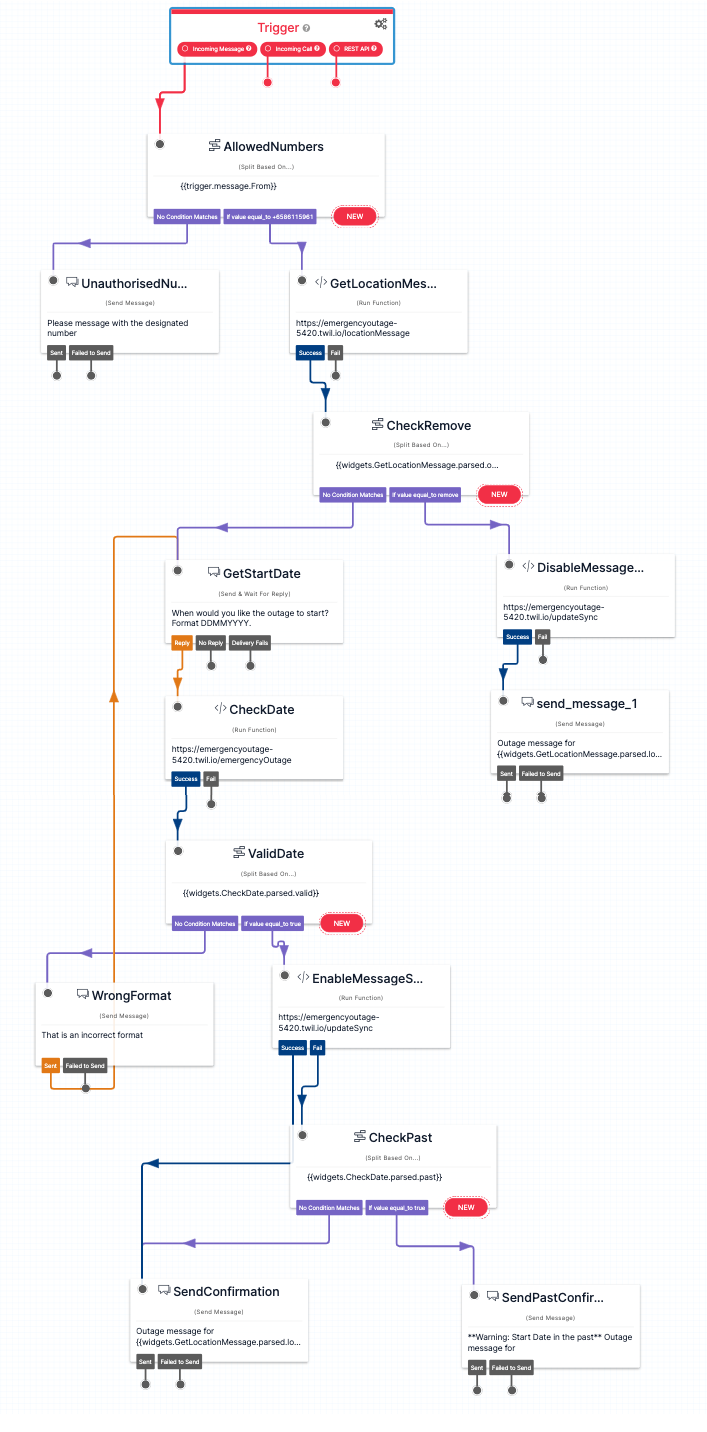
Outage Flow JSON
Instead of recreating the Studio Flow as shown, you can Import a JSON.
To do this open Studio > Flows from the Navigation Pane and click on the Plus icon. Give your new Studio Flow a name. I used UpdateOutage. Then click Next:
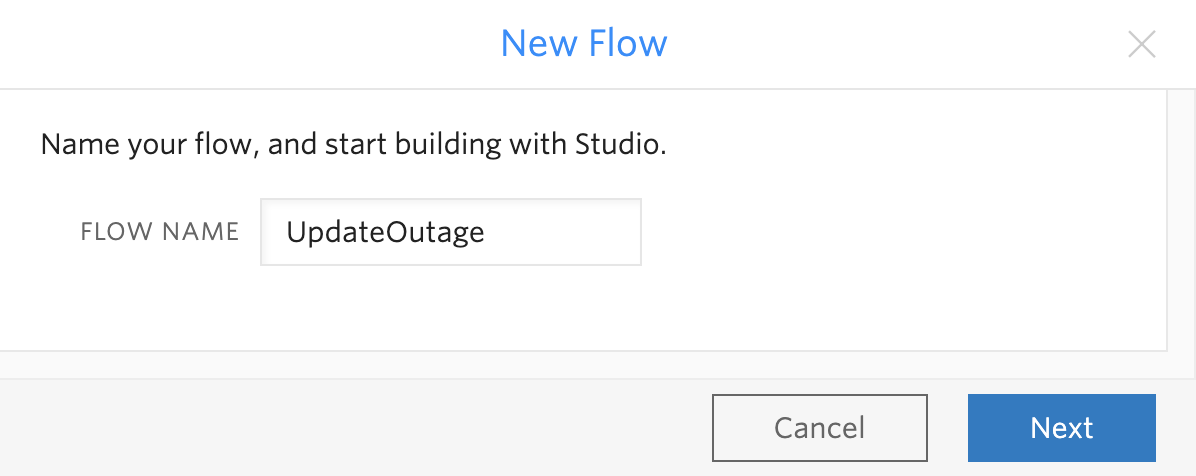
On the Template window, scroll to the bottom, select Import from JSON, and paste in the following code:
This JSON object is the flow pictured above. It checks the SMS messages for both the location and message. If the message includes “remove,” then the function will remove the outage details. If the message indicates an outage, then it will ask for the date. It’ll check the date to see if it’s in the past, present, or future, and send back a message to the admin based on the results.
Outage Flow Exceptions
Your UpdateOutage flow is created, and there are 4 exceptions (Red circle with exclamation mark) on the following blocks of the flow that need to be resolved.
Get Location Message
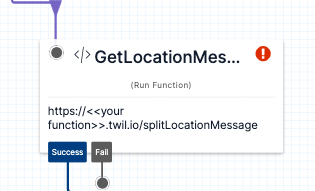
Click on this block and set the SERVICE to EmergencyOutage, the ENVIRONMENT to ui and the FUNCTION to /splitLocationMessage, then click Save:
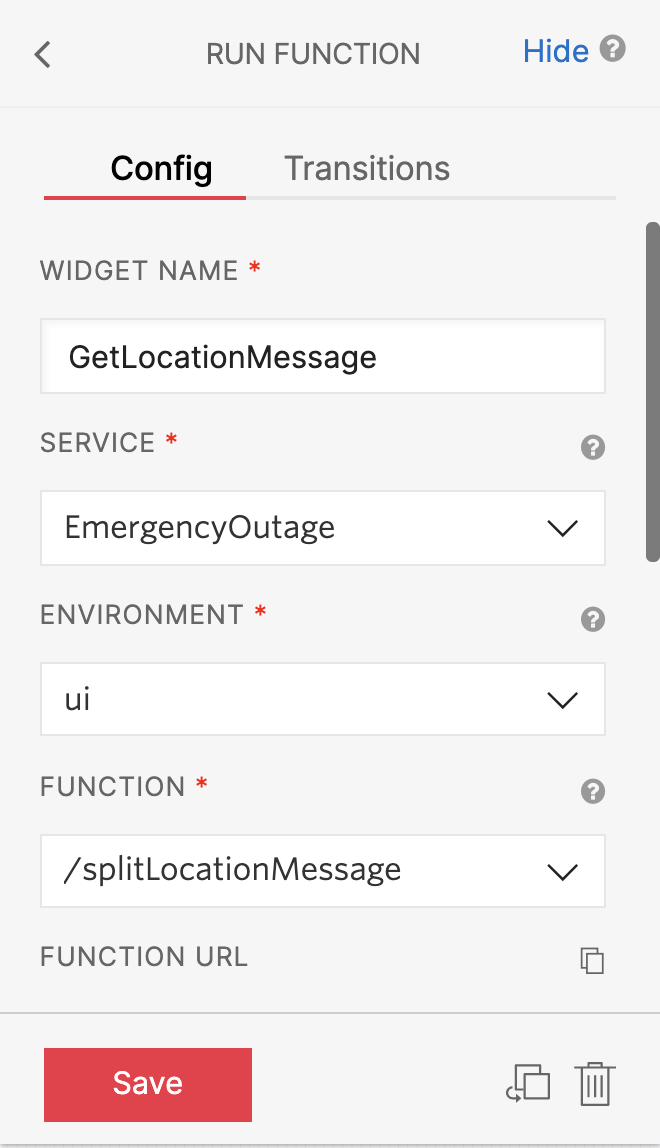
Disable Message Sync
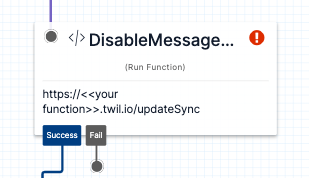
Click on this block and set the SERVICE to EmergencyOutage, the ENVIRONMENT to ui and the FUNCTION to /readUpdateOutage, then click Save:
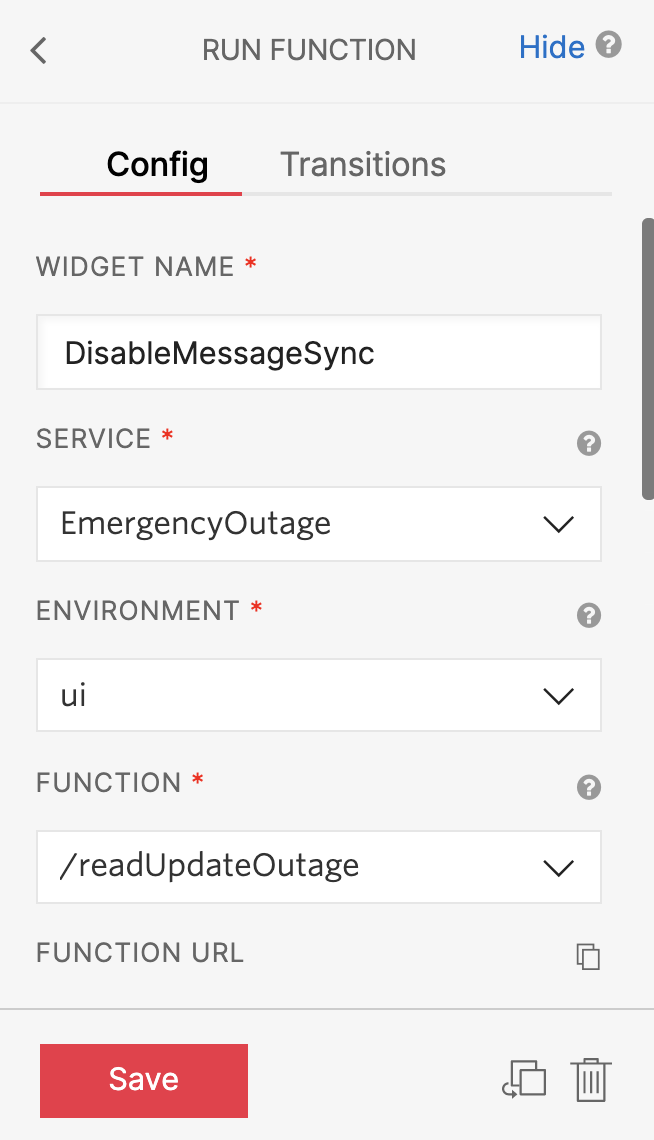
Check Date
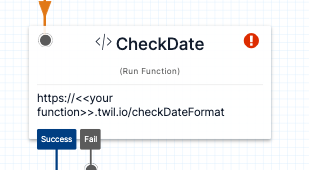
Click on this block and set the SERVICE to EmergencyOutage, the ENVIRONMENT to ui and the FUNCTION to /checkDateFormat, then click Save:
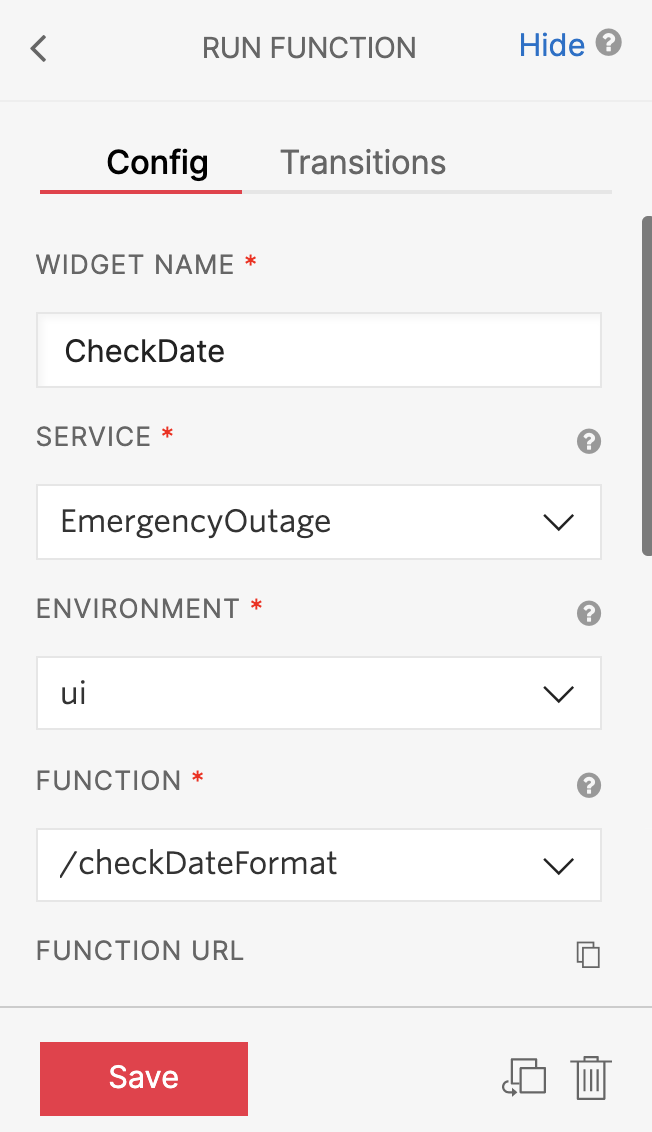
Enable Message Sync
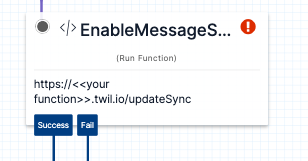
Click on this block and the SERVICE to EmergencyOutage, the ENVIRONMENT to ui and the FUNCTION to /readUpdateOutage, then click Save:
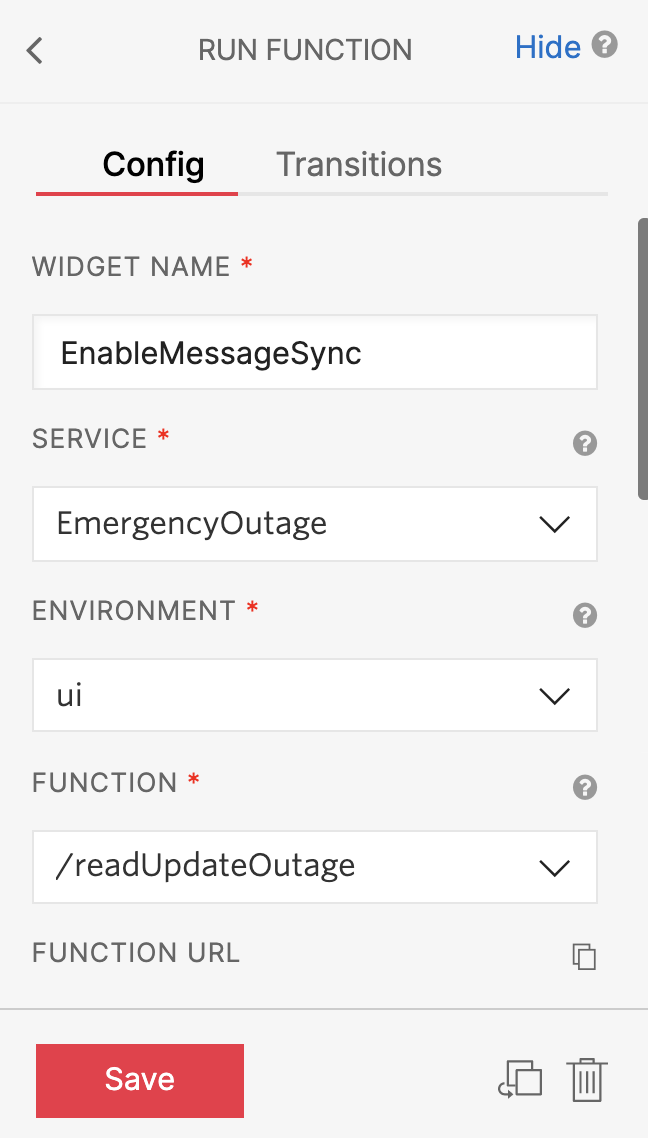
Allowed Numbers
You also have to update the AllowedNumbers block to reflect the mobile number for your admins. Each admin’s phone number should have its own Condition, and point to the GetLocationMessage as shown. Once changed click Save:
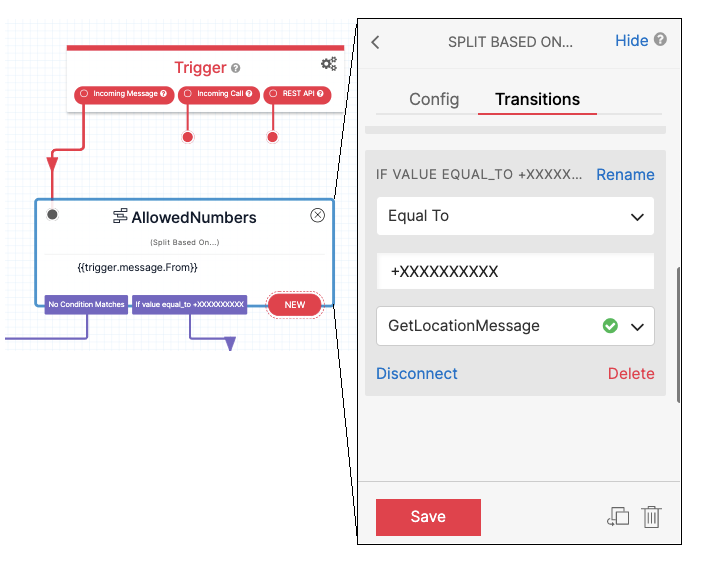
Then Publish the Flow:

Assign Flow to Phone Number
In the Navigation Pane, select Phone Numbers > Manage > Active numbers:
Select the Number you wish to use with your UpdateOutage flow (or optionally buy a new number) and scroll down to the Messaging section. In the A MESSAGE COMES IN section, select Studio Flow, select UpdateOutage, and then click Save:
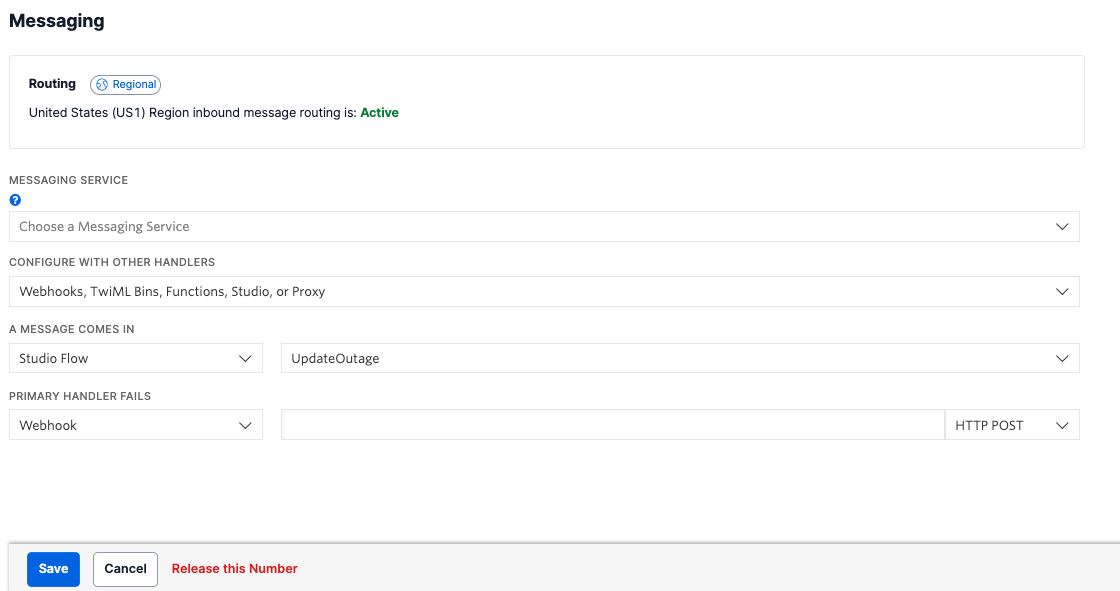
Testing the solution
In the first part of this blog post, you called the number and heard “Normal Operation”. To enable the outage, you can send an SMS containing “Manila: This is a test outage message” (replacing “Manila” with the location you used) to your configured number in the previous step. You will get a follow up message asking when to start the message. Enter today, or a date in the past in the format of DDMMYYYY (e.g. 07112021 for 7 November 2021). Then call the Demo Studio Flow number you configured in the last blog. You should hear the “This is a test outage message” prompt.
To remove the outage, send an SMS containing “Manila: remove” (replacing “Manila” with the location you used) to the Update Outage phone number, then call the Emergency Demo phone number again. You should hear the “Normal Operation” message again.
Lastly you can test entering a future date to start our outage by texting “Manila: This is a second test outage message”. In the follow-up, enter a future date. Then call the Demo Studio Flow number you configured in the last blog. You should hear the “Normal Operation” message again. However, if you call on the date you specified in the outage flow, you will hear “This is a test outage message.”
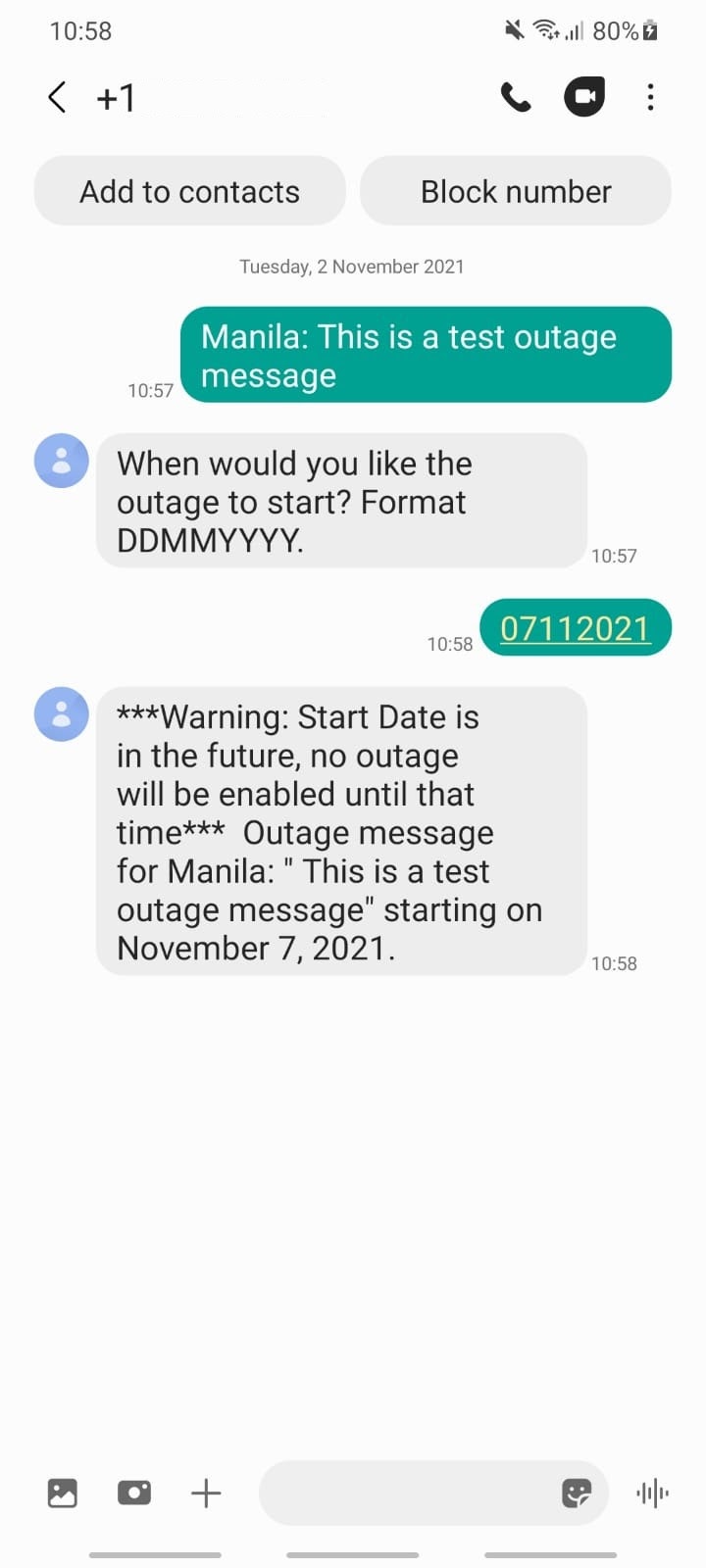
Conclusion
With this solution in place, you now have the ability to quickly activate or deactivate an emergency outage notification no matter where you are using a simple SMS message. Your customers will not need to wait in frustrating unmanned queues and you can operate with maximum efficiency in rapidly changing situations.
Robert is a Senior Solutions Architect at Twilio working in the Professional Services team in Singapore. You can reach him at rhockley [at] twilio.com
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.