Getting Started With the Box API in PHP
Time to read: 3 minutes
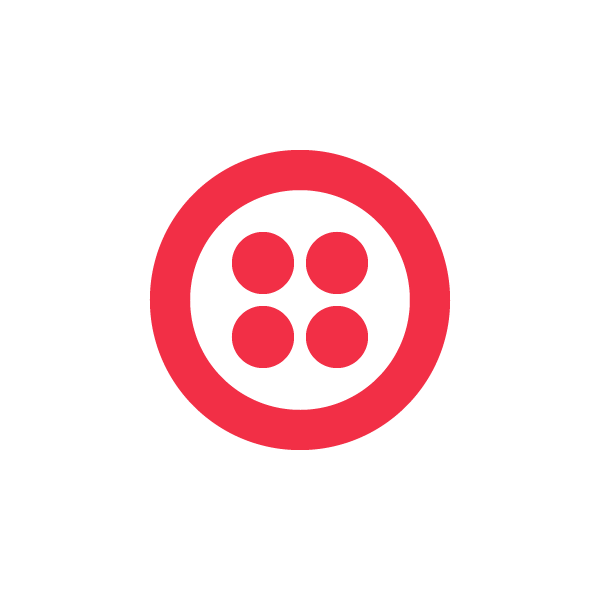
We’re running a contest this week with our friends at Box, so I thought I’d write up a quick tutorial on how to get started using the Box API in PHP. In this short tutorial, we’ll create a simple app that adds comments to a file by SMS.
To get started, you’ll want to setup your project on Box. Head over to http://www.box.net/developers/services and click on the “Create New Application” button on the top right of the page.


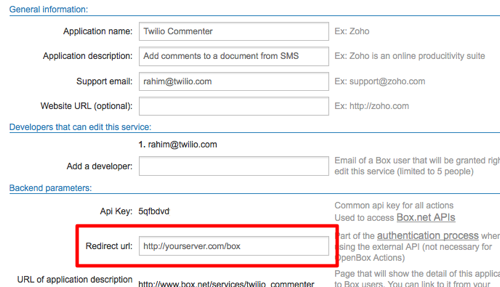
Now, on to the code. First, grab Box’s PHP helper library. After unzipping, you’ll need to make one minor edit to boxlibphp5.php. Edit line 33 and change
var $_debug to
false. Upload boxlibphp5.php and class.curl.php to your server (no need for box_config.php, we’ll take care of that ourselves). Create a file called index.php and paste in:
[php]
require_once(‘boxlibphp5.php’);
$api_key = ‘XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX’;
$auth_token = ”;
$box = new boxclient($api_key, $auth_token);
$ticket_return = $box->getTicket();
if ($box->isError())
{
echo $box->getErrorMsg();
}
else {
$ticket = $ticket_return[‘ticket’];
}
// If no auth_token has been previously stored, user must login.
if ($ticket && ($auth_token == ”) && ($_REQUEST[‘auth_token’]))
{
$auth_token = $_REQUEST[‘auth_token’];
}
else if ($ticket && ($auth_token == ”))
{
$box->getAuthToken($ticket);
}
// Save the auth token to a file (don’t do this in production)
file_put_contents(‘creds.txt’, $auth_token);
$box = new boxclient($api_key, $auth_token);
$tree = $box->getAccountTree();
// Dump the file tree to output
print_r($tree);
?>
[/php]
Be sure to replace
$api_key with your API key.
When you browse to index.php, you’ll be redirected to Box which will ask you to log in and add your application. Once you do that, you’ll be redirected to index.php, the authentication token will be saved to creds.txt, and you’ll get a dump of your Box account’s file tree. If this is a new account for you, it’ll be blank. Upload a file and refresh. Take note of the file_id of the file you want to comment on. It’ll look something like 869161393.
(Note: the code is missing all the bells and whistles like properly storing the authentication token, pretty layouts, and actually letting users choose file elegantly because I wanted to keep the focus on working with the API. Be sure to dress it up in your app.)
Next, create a file called handle-incoming-sms.php and paste in:
[php]
header(‘Content-type: text/xml’);
require_once(‘boxlibphp5.php’);
$api_key = ‘XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX’;
$auth_token = file_get_contents(‘creds.txt’);
$box = new boxclient($api_key, $auth_token);
$params = array(‘api_key’ => $api_key,
‘auth_token’ => $auth_token,
‘target’ => ‘file’,
‘target_id’ => ‘XXXXXXXXX’,
‘message’ => $_REQUEST[‘Body’]
);
$response = $box->makeRequest(‘action=add_comment’, $params);
if ($response[1][‘value’] == ‘add_comment_ok’)
{
echo ‘Comment added!’;
}
else {
echo ‘Woah, there was an error–bummer.’;
}
?>
[/php]
Replace
$api_key with your API key and
target_id with the ID of the file you want to comment on. Grab a Twilio phone number and set its SMS URL to handle-incoming-sms.php and you can now comment on the file through SMS!
Now go out and build something cool using Twilio and Box and you could win a MacBook Air, a Galaxy Tab, or a Parrot AR Drone in this week’s contest!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.