Food Glorious Food! Using Location Data in WhatsApp to find nearby healthy restaurants using Twilio and JavaScript.
Time to read: 4 minutes
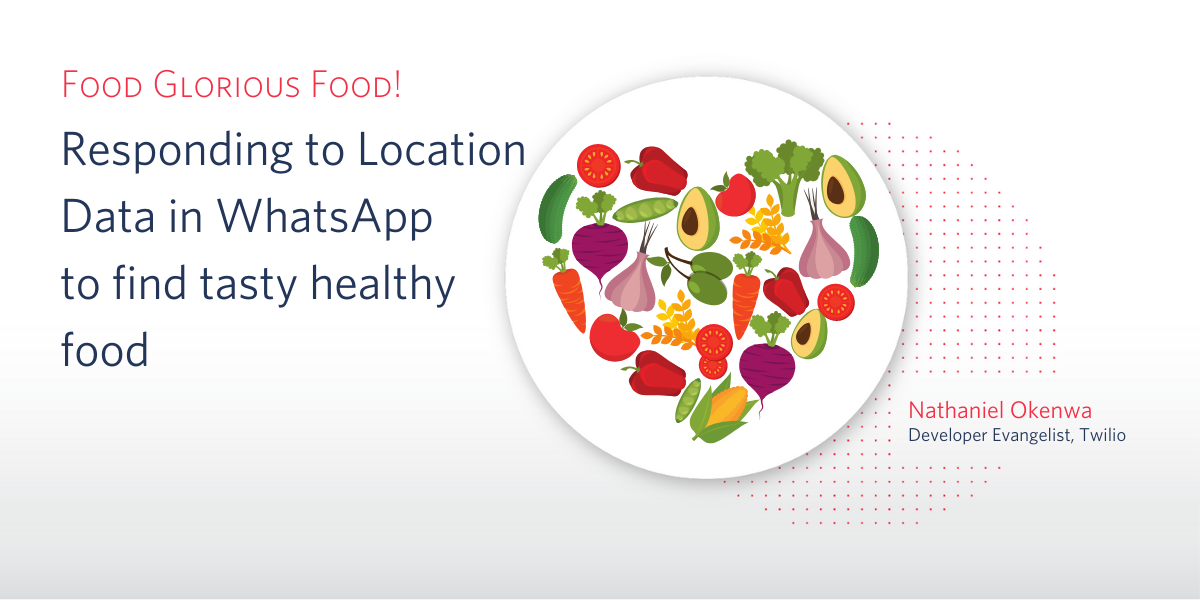
The life of a Developer Evangelist can be very unhealthy. I travel to many different countries and end up ordering fast food to my hotel room far more frequently than I would like. This year, I’m trying to take control of my diet, particularly when on the road. However, I also suffer from decision paralysis. Looking at a long list of restaurants and filtering through them to find restaurants with healthy options can often be tedious and frustrating.
The amazing thing about being a developer is that I can build tools to solve my problems. Today I’m going to show you how I created a bot to find the closest restaurants serving healthy food, no matter where in the world I am! Follow along to build your own.
The Twilio API for WhatsApp makes gathering location data from users easy, enabling us to respond appropriately. Therefore, we’ll use WhatsApp as the channel for our bot.
What We'll Need
- A Twilio Account (Get one here for Free)
- A Travel API with restaurant information (I’m using the Zomato API)
Setting Up Our Function
In this project, we’ll use Twilio Functions as they runJavaScript code directly on Twilio. They are also one of the easiest ways to have code respond to an incoming message.
Head on over to the Twilio Functions page and create a new function using the "blank function" template. Give the function a name and set its path to /food
. Make a note of the whole URL as we will use it later. Be sure to Save the function. By pressing the Save
button at the bottom of the page
We are going to use axios to make HTTP requests to the Travel API. To add this to our environment, navigate to the Configure section on the left-hand side of the Twilio Console and add axios
to our dependencies. I built this using axios version 0.19.1.
We can also add any environment variables we need here such as the API credentials for Zomato. I’ll be adding an environmental variable called ZOMATO_API_KEY
that I’ll be using in my code.
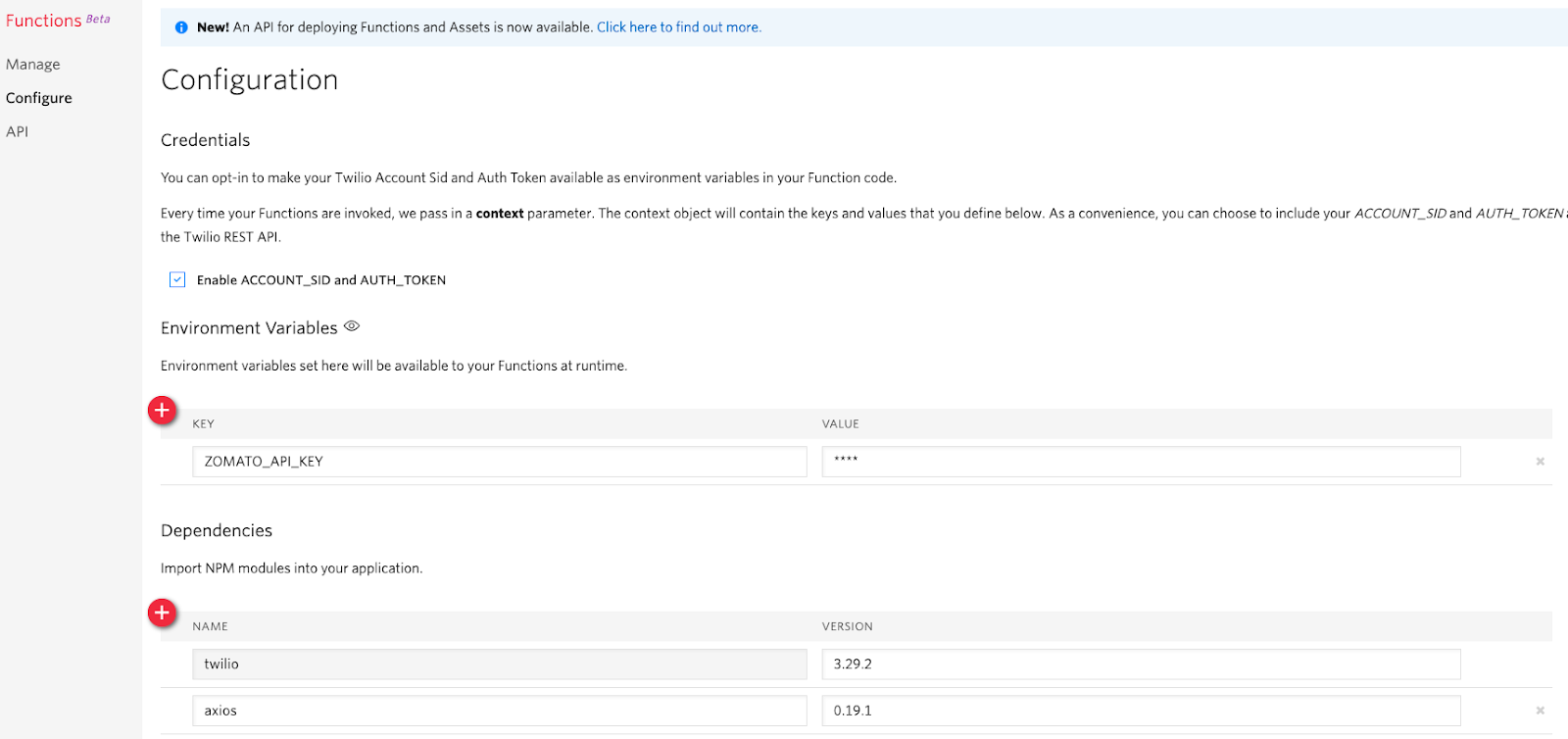
Getting Location Data from WhatsApp
WhatsApp enables users to share their location data with ease. When Twilio receives the location data in a WhatsApp message, it will make a request to our Twilio Function. It surfaces the location data as request parameters with latitude, longitude and an address if available.
To get started with the Twilio API for WhatsApp, you will need to set up a Twilio Sandbox number. After you have completed setup, make sure you have used your personal sandbox keyword to join your sandbox conversation from your WhatsApp App.
Once set up, configure the "When a message comes in" field to make a POST request to the URL we noted earlier in the Twilio Function.

Navigate back to the Twilio Function and get ready to write some code!
This above code will first check that the message contains location data. If we do have a location, let’s reply with the coordinates that we received. If it does not, then we will send a message asking for a location..
Save the function and test it with a WhatsApp message containing your current location to your Sandbox Number. To do this, click on the ‘attachment’ icon and select location and select to Send your current location
. Live Location is currently not supported on the Twilio WhatsApp API.
You should receive a reply like this.
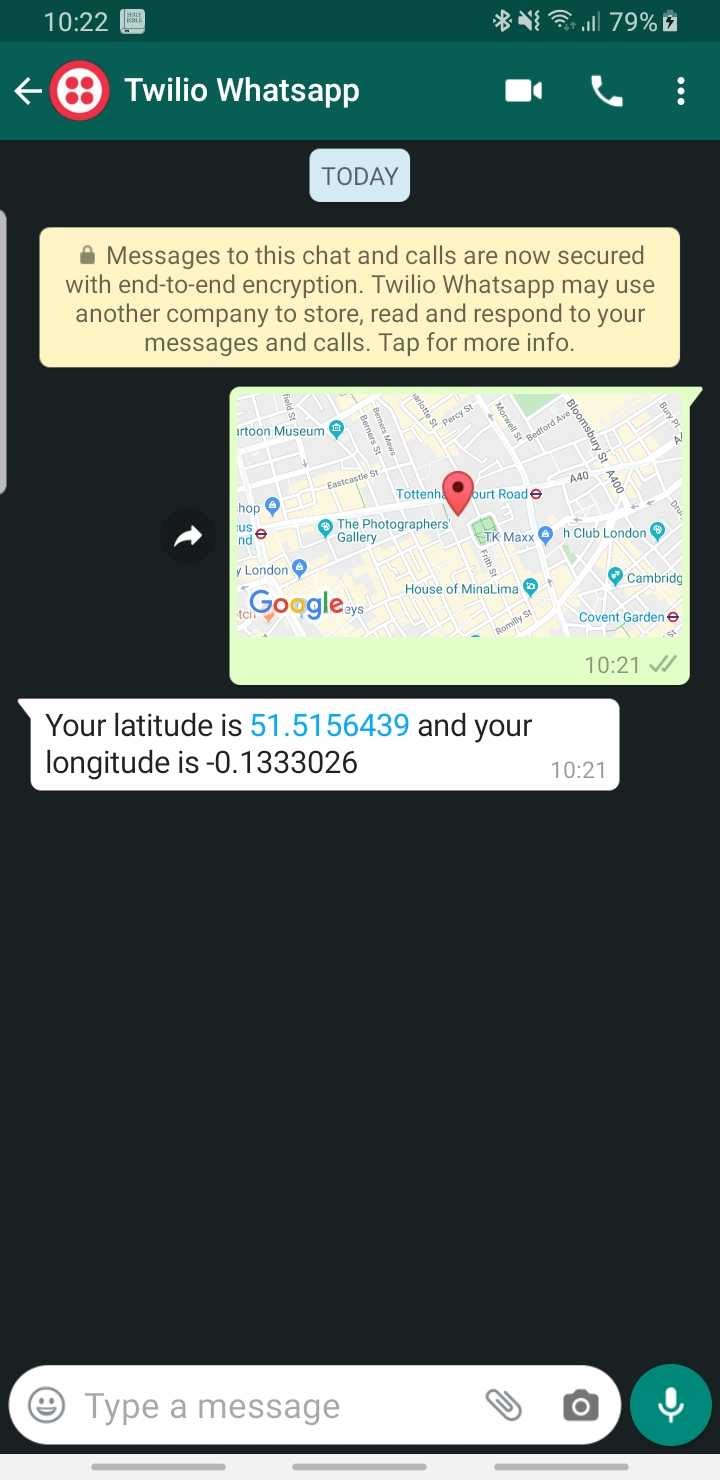
Let’s find some food
Now we can use our location coordinates to find nearby restaurants. I’ll be using the Zomato API but you can easily replace this with an API for anything as long as it can take GPS Coordinates as parameters to return places.
We need to make axios
available in order to make requests to our travel API.
We can now make a call to the travel API and grab a list of restaurants that serve healthy food cuisines. I have used the search endpoint provided by the Zomato API. This endpoint returns an array of restaurant objects that contains information about each restaurant.
Iterating through this array, we can create messages containing the name of each restaurant and a URL to get further details on a given restaurant.
You may notice that I have grabbed my API Key from the context object. This is because I set it up as an environment variable when we configured the environment for our Twilio Function.
I have also shared the code in a gist on Github, if you prefer to copy and paste it.
Save the Twilio Function and test it out by sending another location to the WhatsApp sandbox. You will receive a list of healthy restaurants near you!
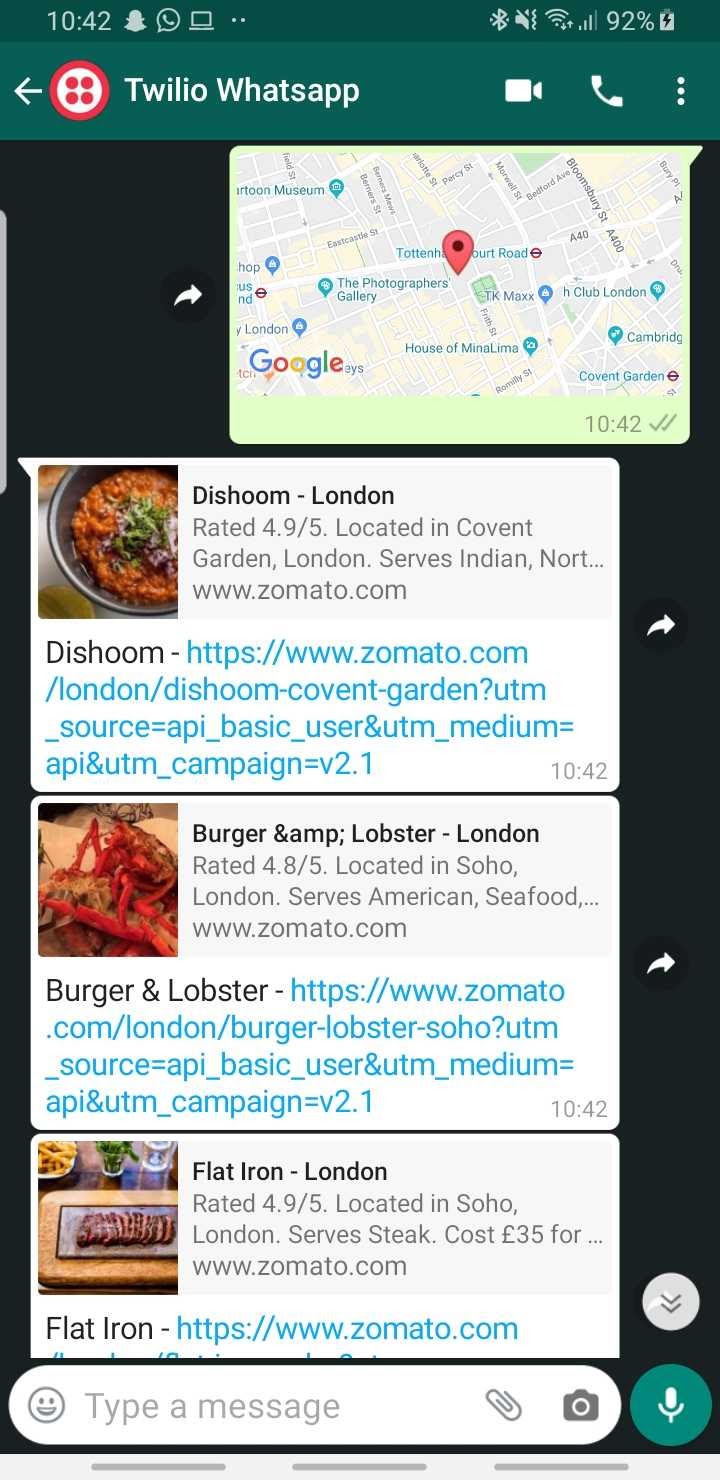
Wrapping Up
I tested this WhatsApp bot to find my lunch today and I must say that I didn’t realise how many healthy restaurants were nearby my office. I look forward to using this bot whenever I’m travelling and I need to find some healthy food to eat.
There are so many other ways you can use location data to serve users over WhatsApp chat. Whether you want to be able to locate a user quickly, or give information specific to where a user is, the Twilio API for WhatsApp is a good place to start.
I can’t wait to see what you build with Twilio WhatsApp. Let me know what you’re working on at:
- Twitter - @chatterboxCoder
- Email - nokenwa@twilio.com
- GitHub - nokenwa
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.