How to Receive Alerts from Home Assistant with Twilio SMS
Time to read: 5 minutes
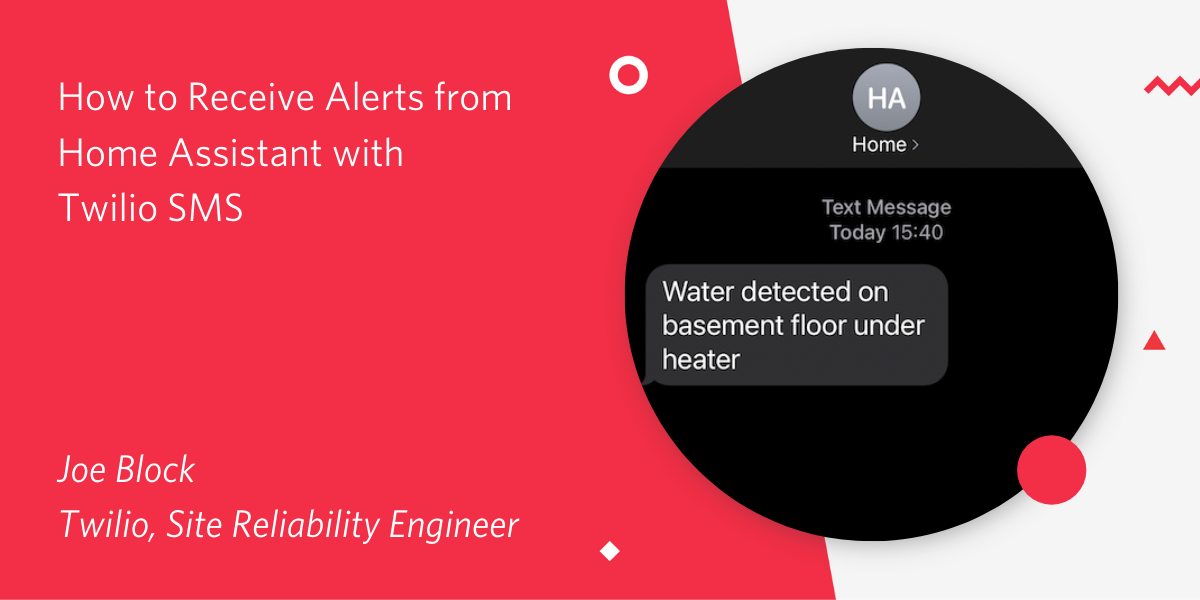
Introduction
Home Assistant is an open source home automation system that allows you to control Internet of Things devices like smart bulbs, smart switches and sensors. In this article, I’ll show you how to use Twilio SMS to have your Home Assistant (HA) alert you via SMS when it detects problems in your home.
Prerequisites
To follow along with this post, you’ll need:
- A Twilio account - if you don’t already have one, sign up for a free Twilio trial account that you’ll use later to test the notification system we’ll be setting up.
- A working Home Assistant installation, with some sensors connected to it. I used a Zigbee water sensor (an Aqara Water Leak Sensor, specifically) for this tutorial but any sensor that you have connected to your Home Assistant will also work - you could also use a garage door sensor and get alerted when the garage door is open for more than ten minutes - the possibilities are only limited by your imagination.
- Enough familiarity with Linux and your Home Assistant installation to be comfortable editing the configuration files.
Background
Last year I set up Home Assistant on a Raspberry Pi in my basement to automate my lights and begin integrating Internet of Things devices into my home. I recently had a very messy water heater leak in my basement, and I wanted to get alerted if it happens again.
I bought an Aqara water sensor so that my Home Assistant system could detect a future water leak and alert me before I had another big mess in the basement. This article covers how I use the Twilio Programmable SMS service to get text alerts before there’s another giant puddle of water on the floor.
Build the Twilio SMS program to receive alerts from Home Assistant
In this article, I’m going to assume that you already have a working Home Assistant installation, that it is in the /config
directory in a docker container, or – if you’re running directly on your hardware – on the native OS filesystem. You can find instructions for installing HA in a Docker container on the Home Assistant website.
To make it easier for you to copy the article’s examples into your own HA configuration, all the code and yaml
files are in this article is on Github in the article repository.
Make sure that you also sign up for a Twilio account and buy a Twilio Phone Number for this project as well.
Set up your Twilio Credentials and Phone Number
Log in to your Twilio console. You’re going to need a phone number to use for outgoing SMS messages - click on the (...) on the sidebar and select # Phone Numbers. You should see the number you have purchased earlier. If you have more phone numbers, choose a number that you would like to use for this project.
Second, you’ll need your ACCOUNT SID and AUTH TOKEN from the Twilio Console in order for the helper script to be able to send SMS. Put them into the /config/twilio-credentials.sh file in the following format:
These values will be used by the SMS helper script that we’re about to write. Don't forget to replace the TWILIO_NUMBER
with the one you just bought.
Create the helper script
While you can run a curl
command directly out of Home Assistant, I find that it’s more convenient to debug problems in the code if I have Home Assistant call external scripts. This way I can make sure to test the code one part at a time before I even attempt to integrate them with Home Assistant.
Create a folder named helpers inside of the /config directory. Make a new file named send-sms and paste in the following code:
Once you’ve created the script, make it executable by running chmod 755 /config/helpers/send-sms
or Home Assistant won’t be able to run it.
Send a test message to your device by running the command /config/helpers/send-sms YOUR_PHONE_NUMBER 'Congratulations, you have sent an SMS from your HA server'
in your terminal window. Replace YOUR_PHONE_NUMBER with your personal cell phone number in E.164 format.
Check your phone to see if the message went through. After you confirm that the script is sending SMS messages, let’s get Home Assistant to send them automatically when certain conditions are met.
Add numbers to Home Assistant Secrets file
I didn’t want to hard-code my Twilio number or my cellphone’s number into the examples, so I’m storing them as Home Assistant secrets. I’m adding them as two keys, twilio_number
and demo_phone
. Set the demo_phone
variable to the phone number of your personal device.
Make Home Assistant Send SMS Messages
As I mentioned earlier, I want to have my Home Assistant send me a text message when it detects water on the basement floor. Here’s what I want it to look like:
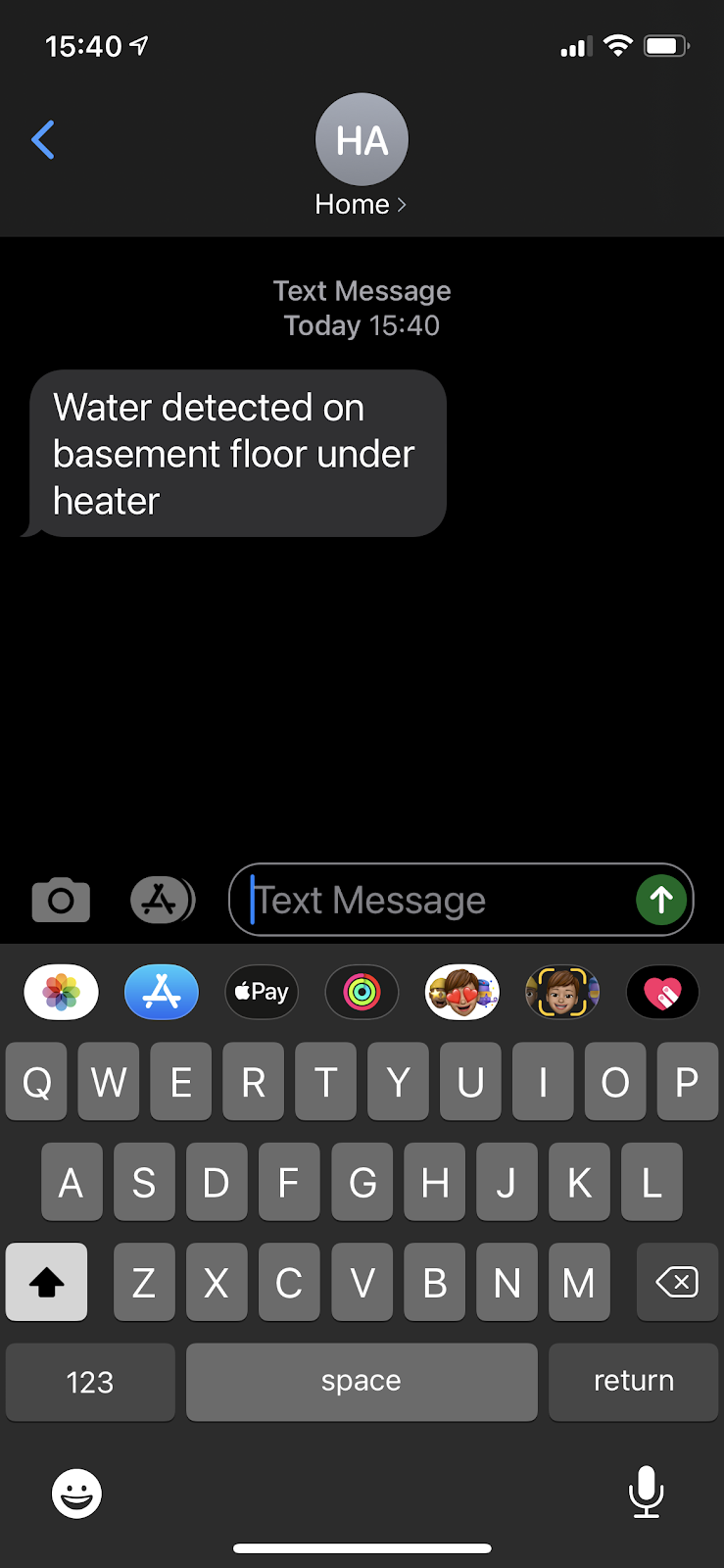
We need to define a shell command to call the send-sms script we just created. Edit the configuration.yaml file inside the config directory and add the following shell command section:
Now that we’ve defined a new twilio_sms
custom shell_command
service, we can refer to it anywhere in the HA configuration that we’re allowed to use actions. To demonstrate, I’m going to define two new automations - one that is triggered when water is detected on our water sensor, and another that is triggered when the sensor is dry again.
I added my Aqara sensor to Home Assistant using Home Assistant's documentation to connect other hardware devices. I configured it so that the sensor shows up in Home Assistant’s entity list as binary_sensor.water_01_zone
.
Home Assistant stores custom automations in the automation.yaml file. Open it and add the following entries. I’m using two different automations so HA can send one message when water is detected, and a different “all-clear” message when the floor is dry to let us know that the leak has stopped.
HA water sensors have a state of off when they’re dry, and on when they detect water. For our water alert example, I’m triggering one automation to happen when the state of the water sensor changes from off to on and another when it changes from on back to off. HA allows for very flexible triggers, so if you want to explore more options using other sensor types like motion sensors or door sensors, you can find detailed documentation for triggers on their site here.
Conclusion: Building a Twilio SMS program to receive alerts from Home Assistant
And that’s it, we’re done! Feel free to check out my GitHub repository for the project files and make sure that you have everything. The next time (though: hopefully there won’t be a next time) that there’s water on the basement floor, I’ll get an SMS immediately instead of discovering in the morning that it has been leaking hundreds of gallons of water onto the floor all night.
Joe is an SRE at Twilio. He currently lives in Denver after spending a decade in the SF Bay Area automating computer fleets for a variety of tech companies. When he’s not working you can usually find him hiking somewhere or cooking. Among his other projects, he maintains lists of resources for ZSH, Git and Home Assistant on GitHub.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.