How to build a Conference line with Twilio and Node.js
Time to read: 2 minutes
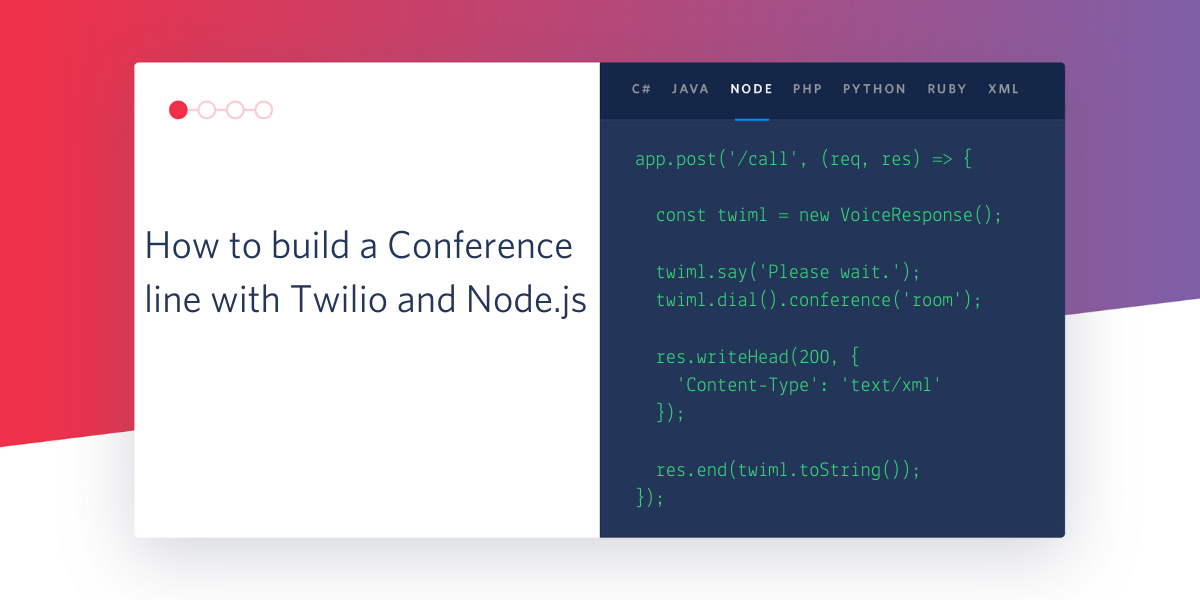
Another conference call, another app, another PIN, another log-in. Joining conference calls shouldn’t take more than simply dialing a phone number. Lets walk through how you can quickly build a conference line anyone and everyone can use with Node.js.
Development Environment Setup
Start by making sure you have the right software installed that you'll need to use for the rest of this post. You will need:
- Node.js and npm installed (do this first if you haven't already)
- A free Twilio account and a Twilio phone number
- The npm modules for Express and the Twilio Node library
Here is a good guide to follow in general if you are going to be doing more with Twilio and Node.js and have any more questions.
To install these npm modules, navigate to the directory where you want this code to live and run the following command in your terminal to create an npm package for this project.
The --yes
argument just runs through all of the prompts that you would otherwise have to fill out or skip. Now that we have a package.json for our app, let’s install the necessary libraries with the following shell commands:
After this you should be ready to write some code!
Setting up an Express server to receive the incoming call
Open a file called index.js
in the same directory as your package.json and add the following code to it:
This code sets up an Express app, defines a route on the app to handle incoming phone calls, and then has it listen on port 3000 for incoming requests. In the /call
route, we are responding to the HTTP request Twilio sends when a phone call is received with some TwiML that tells Twilio to greet the caller, and then dial them into a conference line.
You can run this code with the following command:
Leave this Express app running in your terminal because we will use it in a little bit.
Setting up your Twilio account
Before being able to receive phone calls, you’ll need a Twilio phone number. You can buy a phone number here (it’s free while you're just testing your code).
Your Express app will need to be visible from the internet in order for Twilio to send requests to it. We will use ngrok for this, which you’ll need to install if you don’t have it already. In your terminal run the following command:
If you’ve just installed ngrok and that previous command didn’t work, you might have to run it like ./ngrok http 3000
from the directory that the ngrok executable is in.
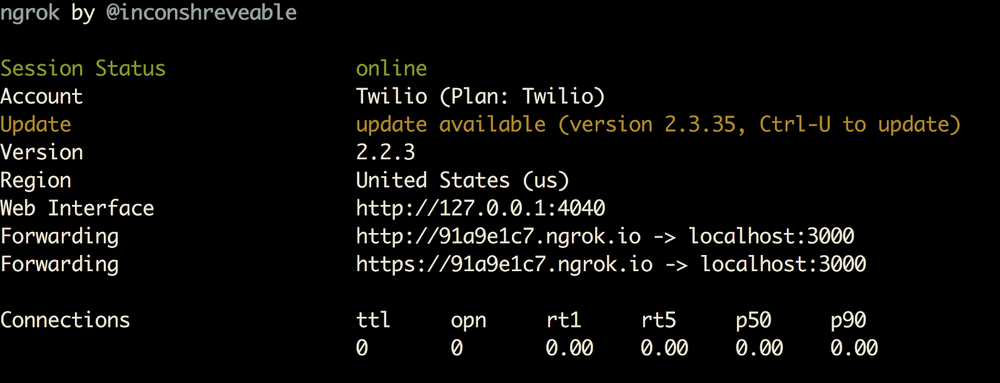
Ngrok provides us with a publicly accessible URL to the Express app. Configure your phone number in your Twilio Console as seen in this image by adding your ngrok URL with "/call" appended to it to the "A Call Comes In" section:
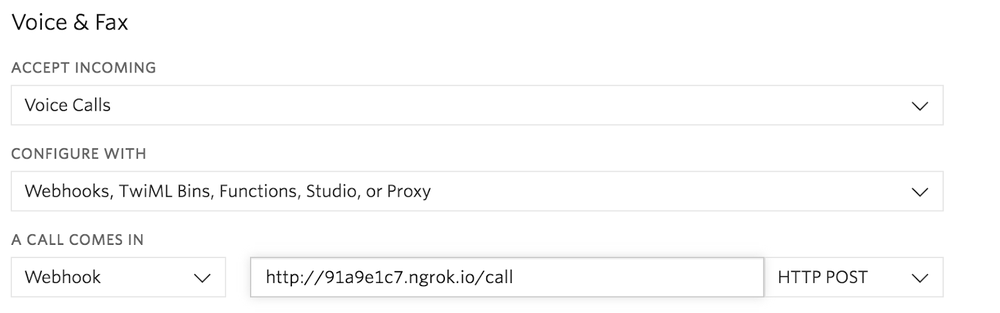
You are now ready to receive a phone call to your new Twilio number. Give your new conference line a spin and have some friends join you to test it out!
Give your friends and colleagues a call
Now that you know how to create a basic conference call, try building off of it with other features from our Conference TwiML docs such as joining a Conference on mute, silently bridging calls, or making a conference call moderated.
I’m looking forward to seeing what you build. Feel free to reach out and share your experiences or ask any questions.
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.