How to Receive an SMS in Node.js with Twilio and HyperDev
Time to read: 4 minutes
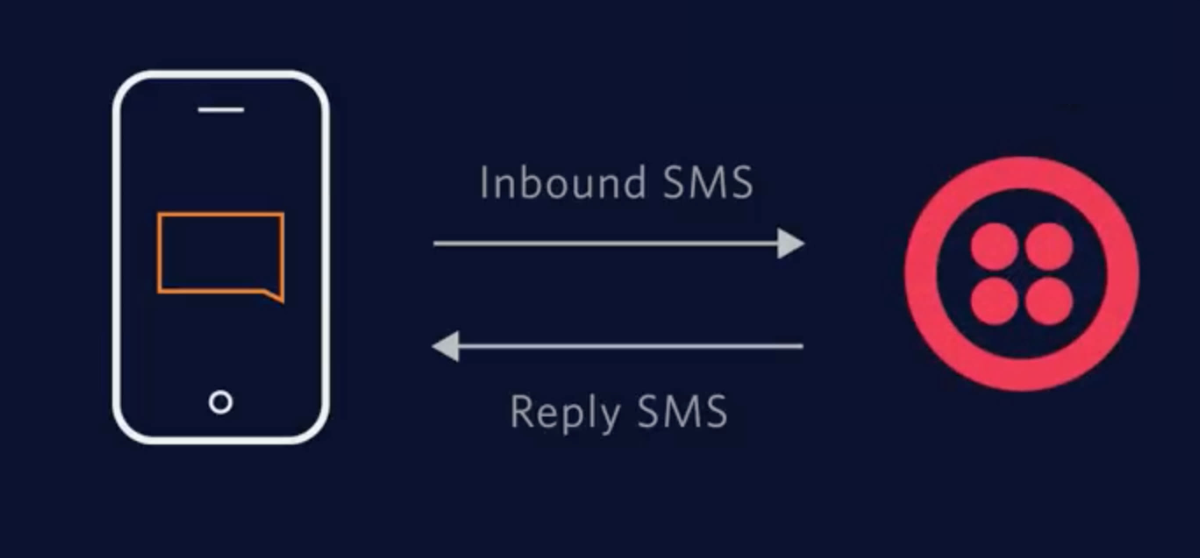
When someone texts your Twilio phone number, Twilio makes an HTTP request to your app. Details about the inbound message, such as what it said and the number it was sent from, are passed in the parameters of that request.
In this post we’ll look at how to receive and parse that request in JavaScript using Node.js and Express. Then we’ll look at how to reply to the inbound SMS with a message of your own.
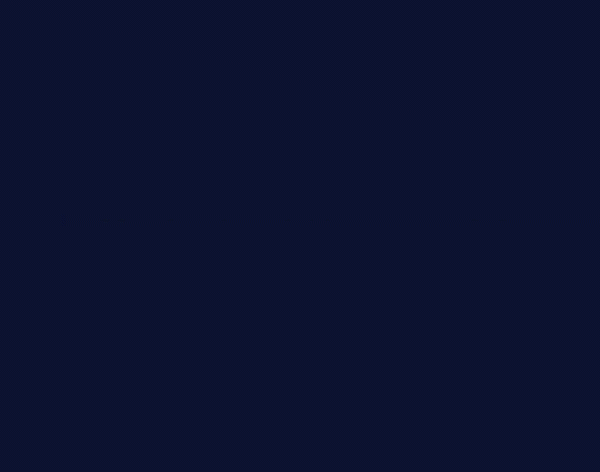
Setup your Node.js Server
For those who like to skip to the punchline, here’s all the JavaScript you’ll need:
To get this app up and running quickly, we’ll use HyperDev, a service that lets you write and run Node apps directly from the browser.
Open this HyperDev project, click the Remix button to create your own copy of the project, then open server.js. Let’s walk through this JavaScript one chunk at a time.
First we create an Express app and include body-parser to parse the inbound HTTP request.
Then we:
- create a route to receive a POST request at /message
- log request.body to the console. This contains the all message details from Twilio.
- send an HTTP response back to Twilio. This response takes the form of TwiML and tells Twilio to send a reply message.
Finally, we:
- Serve up a homepage. Okay, this is just so I could give you that fancy “Remix” button. You don’t really need this.
- Start the server. You do need this.
Click the logs button above the file-tree. Here’s where we’ll see our request body along with any errors that happen during development.
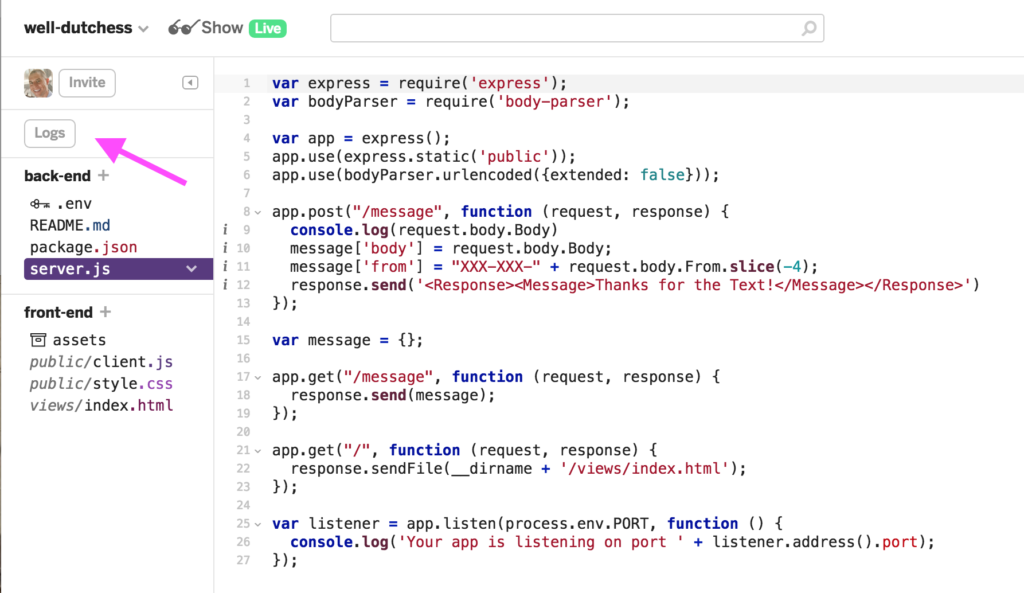
Click the Show Live button above the Logs button. Your project is now running at http://your-space.hyperdev.space (actually, it’s been running this whole time). Copy your app’s URL to the clipboard.
Setup Twilio
You’ll need a Twilio account. You can sign up for a free trial account here.
Buy a number. At the end of that process you’ll be asked to Setup Number. Scroll to the Messaging section and find the line that says “A Message Comes In.” This is where you plug in the URL of your Node app.
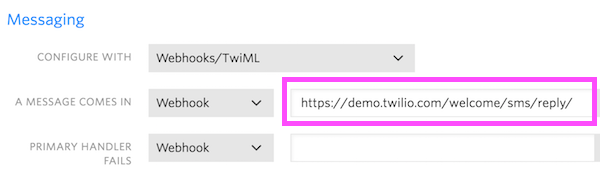
Paste your HyperDev project’s URL into the box and append the /message. (i.e., https://your-space.hyperdev.space/message). Click Save.
Receive an SMS
Send an SMS to your shiny new Twilio number and check out your HyperDev logs. You’ll see something that looks like:
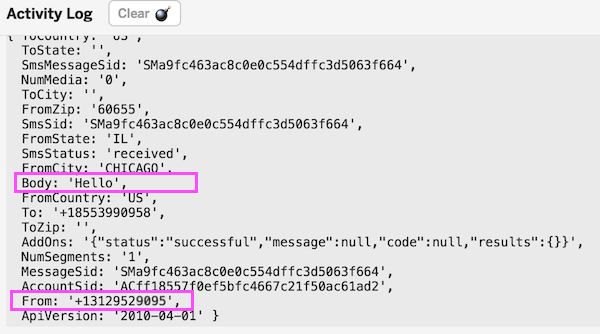
Whoa! That’s a lot of data. The two most useful pieces are:
- the body of the inbound message. This is in response.body.Body.
- the phone number the message was sent from. This is in response.body.From.
Let’s simplify that output a bit. Go back to server.js and update the /message route to look like this:
HyperDev will automatically save the file and restart your server when you make a change. Text your number again and check out the much easier to read logs.
Next Steps
How easy that was? Receiving a text message is as simple as setting up a basic web server and writing a few lines of JavaScript. Replying is as easy as sending back three lines of XML. And with HyperDev, writing and deploying a Node app is as easy as opening a browser.
If you’d like to dive deeper into the world of Twilio SMS, I’d recommend you check out:
- The Node.js Helper Library
- The Twilio REST API
- This in-depth tutorial on how to send SMS and MMS notification in Node
If you have any questions, drop me a line at gb@twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.