How to Send Emails in Python with Sendgrid
Time to read: 2 minutes
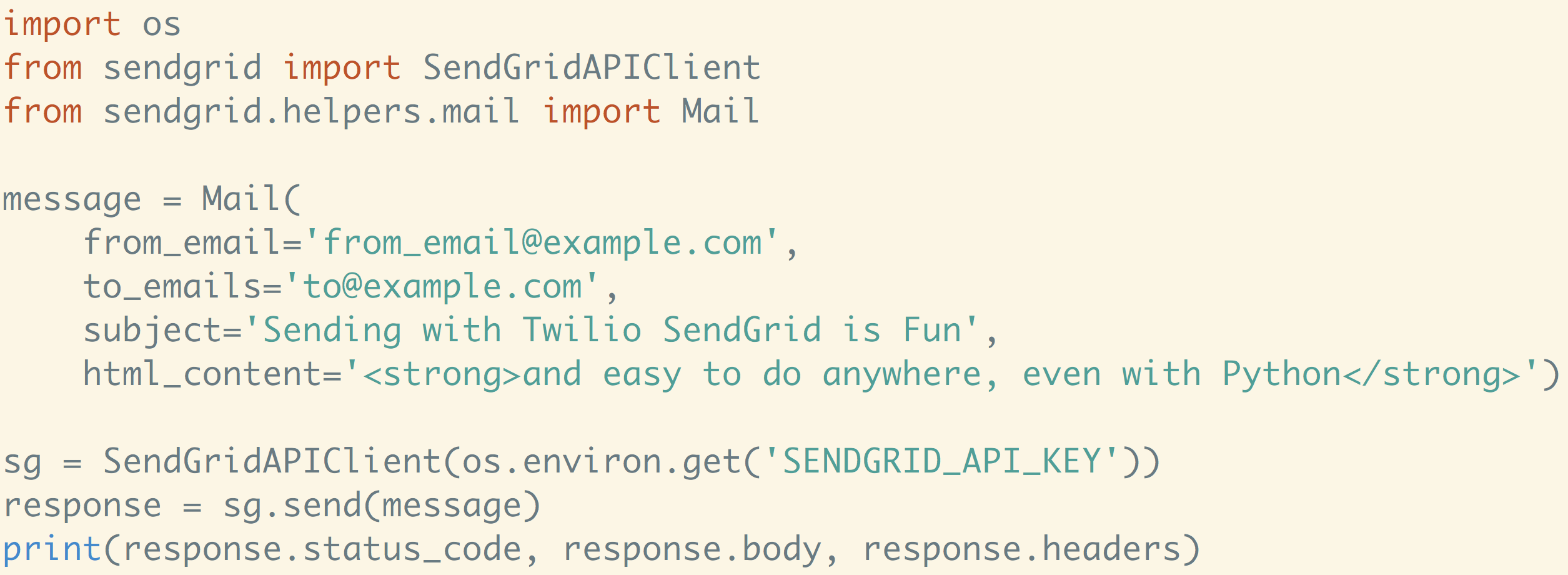
So you're building a Django or Flask app and you need to programmatically send some emails. The Twilio SendGrid API for sending email is a great solution to this problem. If you have a SendGrid account and an API key set as an environment variable, here is all the code you need to send an email in Python:
Let's walk through how to get this running step by step.
Development Environment Setup
Make sure we have the right software installed that we'll need to use for the rest of this post. Throughout this post you will need:
- Python 2 or 3 installed (do this first if you haven't already)
- A free SendGrid account
- The SendGrid Python library
Here is a good guide to follow on setting up your development environment if you are going to be doing more web development with Python.
Sign up for SendGrid and create an API key
The first thing you need to do is create a SendGrid account. You can choose the free tier for the purpose of this tutorial. Once you have an account, you need to create an API key as seen in this screenshot. You can name it whatever you want, but once it is created make sure you save it before moving on!
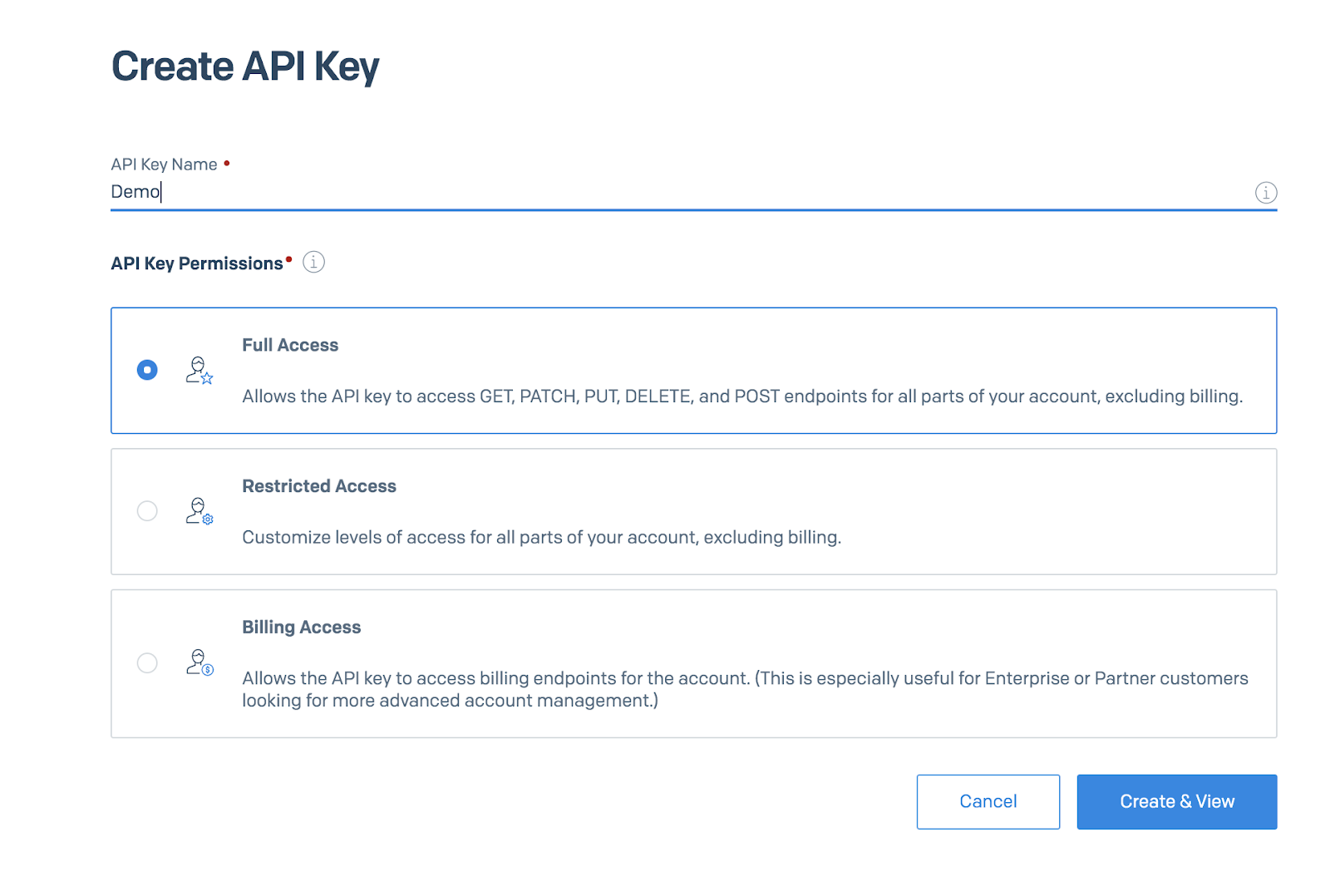
A good way to save this API key is to set it as an environment variable that you can access from your Python code in order to avoid writing it directly in your code. Set the value of the SENDGRID_API_KEY
environment variable to be the API key from your SendGrid account. Here's a useful tutorial if you need help setting environment variables. We will use this later on.
Sending an Email with Python
Now that you have a SendGrid account and an API key, you're ready to dive into some code and send emails! Start by opening your terminal and navigating to the directory where you want your project to live.
First install the virtualenv package then run the following command:
After either of those steps, activate the virtual environment:
Install the SendGrid Python helper library in the virtualenv:
Now create a file called send_email.py
in this directory and add the following code to it:
Before running this code, make sure you have the SENDGRID_API_KEY
environment variable set, and remember to replace the to_emails
value with your own email address to verify that your code worked correctly.
Finally, in your terminal run the following command to run this code to send yourself a picture of an email:
Check your inbox and you should see something like this!
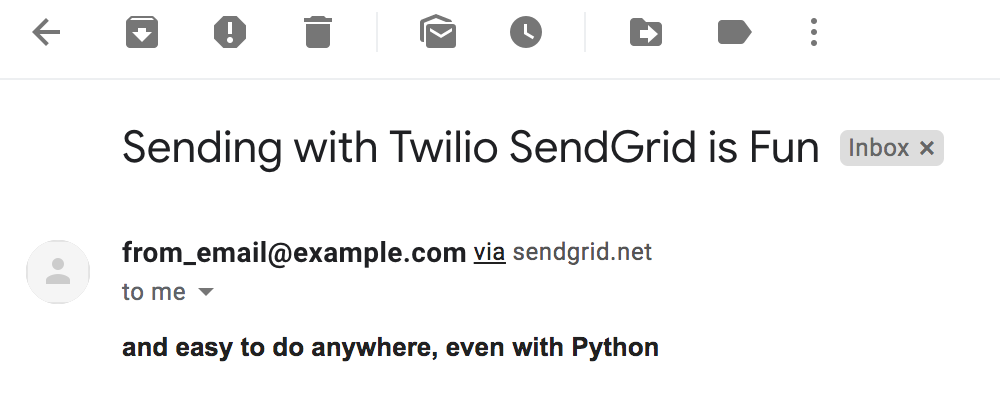
What next?
You just successfully sent your first email using Python, but what if you want to do more than just send emails? You can also process and respond to incoming emails using SendGrid's Inbound Email Parse Webhook. You can also check out the SendGrid docs for a ton of other cool features and uses.
I’m looking forward to seeing what you build. Feel free to reach out and share your experiences or ask any questions.
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.