Identify Phone Number Line Type with Twilio Lookup to Alert Customers over SMS or Voice
Time to read: 3 minutes
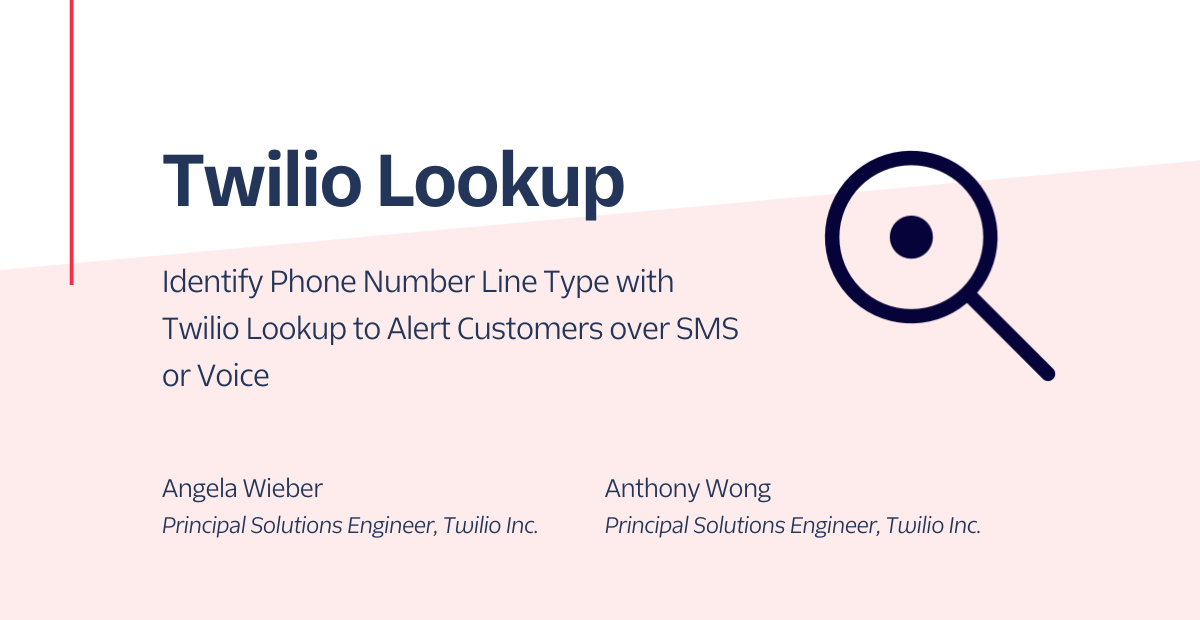
Although landlines have been decreasing in popularity with the rise of mobile phones, some households continue to use them as a primary means of contact. According to the National Center for Health Statistics, 10.4% of adults aged 65 and older have a landline alone without mobile service. This demographic is important to account for when sending critical alerts and time-sensitive notifications to customers.
In this tutorial, we are going to build a demo that checks an end user’s phone number type and sends an instant alert as a voice call or SMS depending on whether the device is a landline or mobile. You can build this in the Twilio console or programmatically. We’ll use the console in this blog post.
Prerequisites
What we will build
- A Twilio Function that uses the Twilio Lookup v2 API to determine a phone number line type. It returns eleven different types including:
mobile
,landline
,fixedVoip
,nonFixedVoip
,tollFree
, and more. The Lookup API is capable of determining even more information about a phone number, including its carrier, caller name, or even if the SIM card linked to the phone number has recently changed (which indicates potential account compromise), but for our purposes we only need the line type. - A Twilio Studio Flow that triggers the above Function and uses its output to decide whether to trigger a call or SMS to the customer.
- We will test the demo using API Explorer in the console by triggering the Studio Flow with a phone number you have access to.
Write the Function
First, we’ll build the function to return line type.
- Log in to the Twilio Console and navigate to the Functions tab.
- Functions are contained within Services. Create a Service by clicking the Create Service button. Name it “lookup”.
- Once you've been redirected to the new Service, click the Add + button and select Add Function from the dropdown. Rename the path from “/path_1” to “/lookup”.
- Copy the following code into your newly created function:
5. Click Save. Under Settings select Dependencies.
6. Update the “twilio” module version to “latest” version.
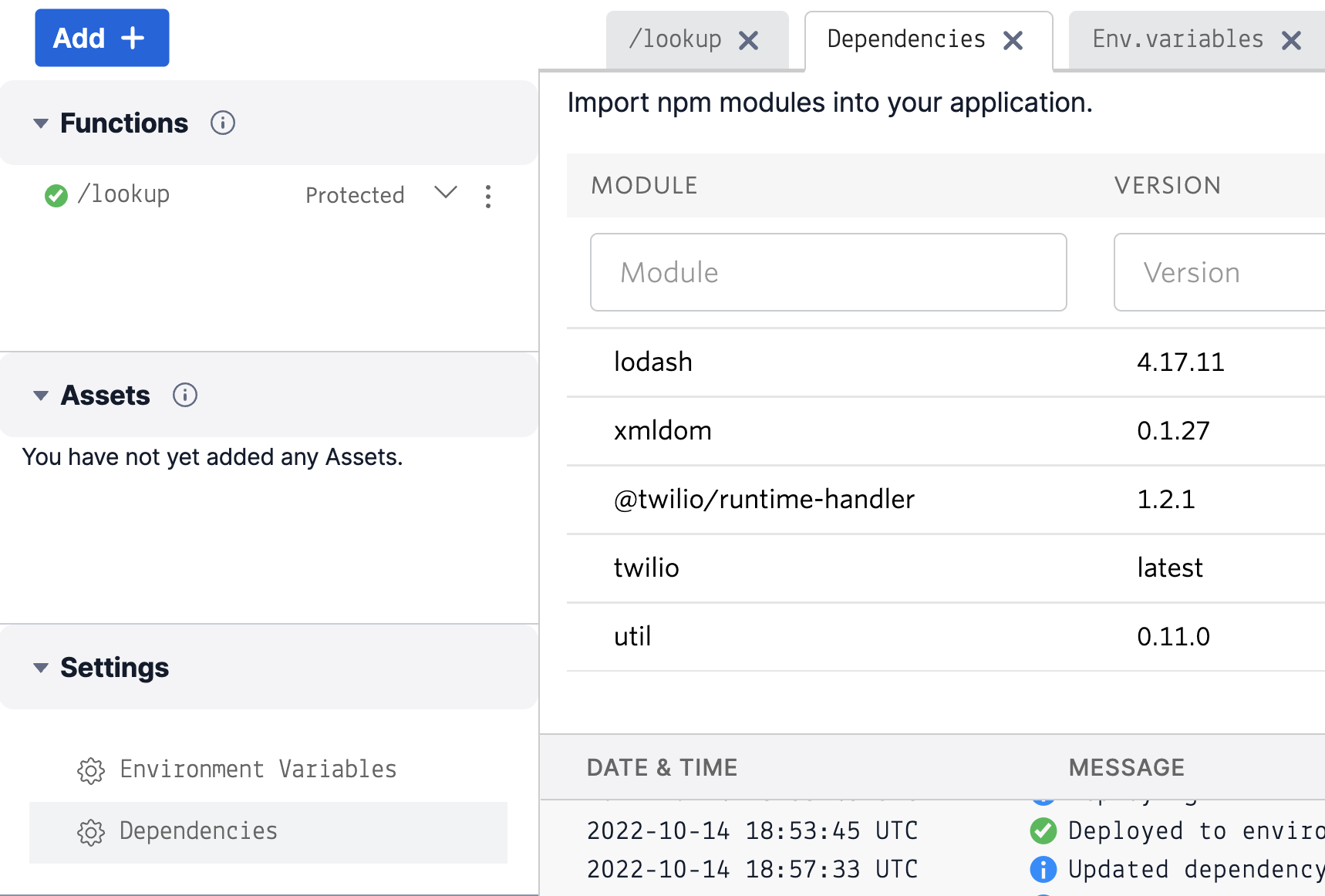
Finally, click Deploy All. Copy the URL for the function by selecting the three dots to the right of your function name, then clicking Copy URL. You’ll use this URL in the next step, so keep it handy.
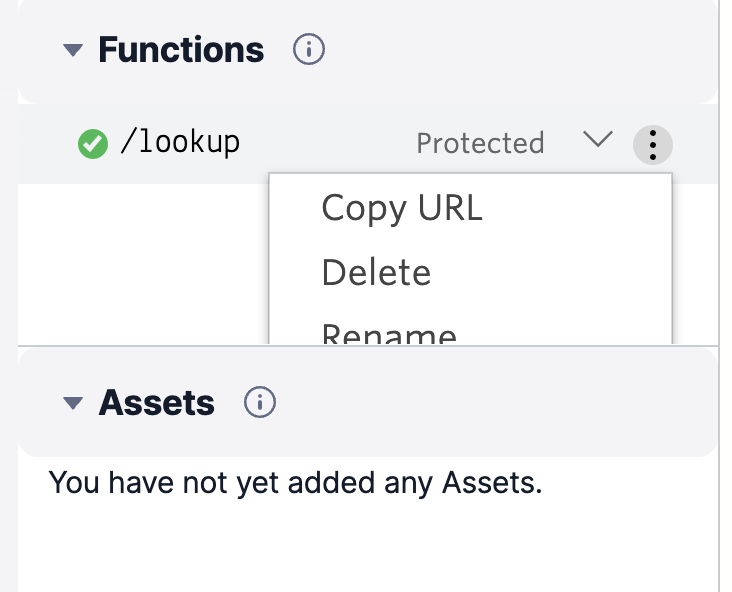
Build the Studio Flow
Now, we’re going to build the Studio flow which will decide whether to send an SMS or call the customer.
- Navigate to the Studio tab.
- Select the + Widget to create a new flow. Give it a descriptive name such as “Call or SMS”. Select Next.
- Scroll down to select “Import from JSON” and click Next.
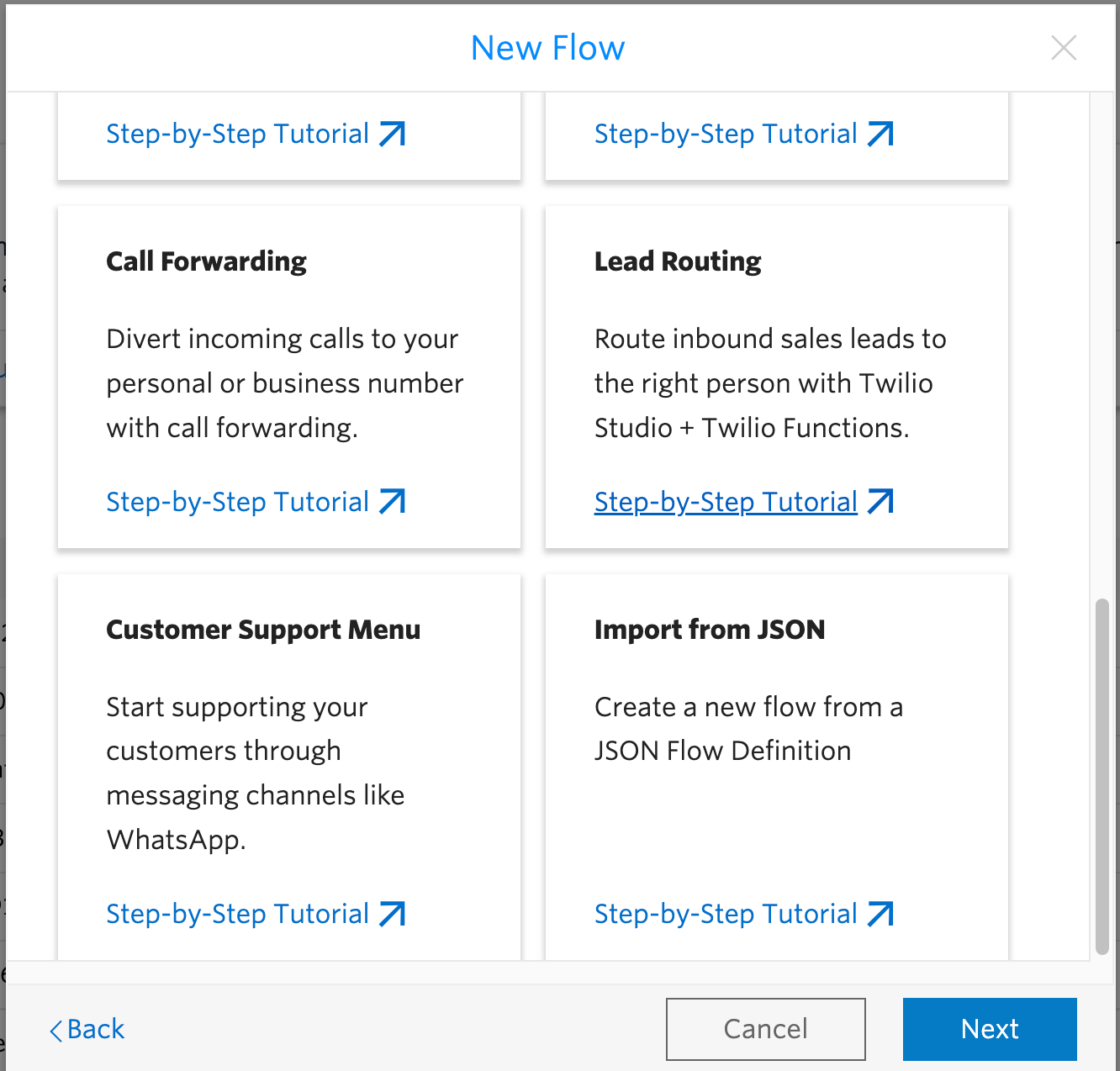
- Remove any existing JSON and copy the following into the box:
Your flow has been created! Now, there are just a few more steps to finish the flow.
- Click on the widget that is labeled “lookup”. You’ll need to edit this field to reference the function you created earlier.
- Scroll down to “Request URL” and paste the Function URL you copied in Writing the Function Step 7.
- Click “Save”. Select “Publish” to publish the latest changes.
- Return to the Studio Flows page and copy the SID of the Flow you just created.
Test your Demo
Your next step is creating a Studio Flow execution. An execution represents a specific person’s run through the Flow. The Twilio API Explorer allows us to make API requests within the console, so you can easily test out your Flow without setting up your own environment for running code.
- Navigate to the API Explorer tab.
- In the dropdown, begin typing “Studio” and select “Studio”.
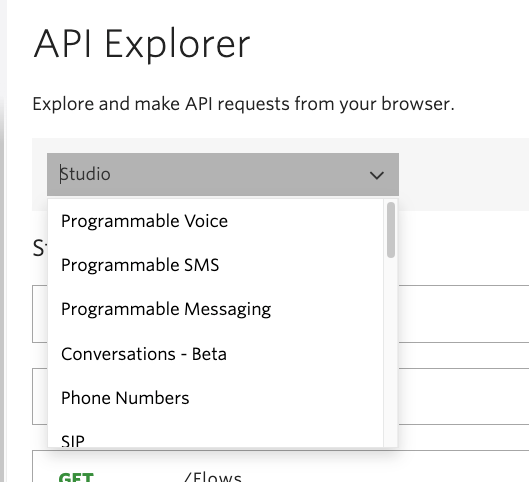
- Select the dropdown for Studio Flows.
- Scroll down and select “Create an Execution”
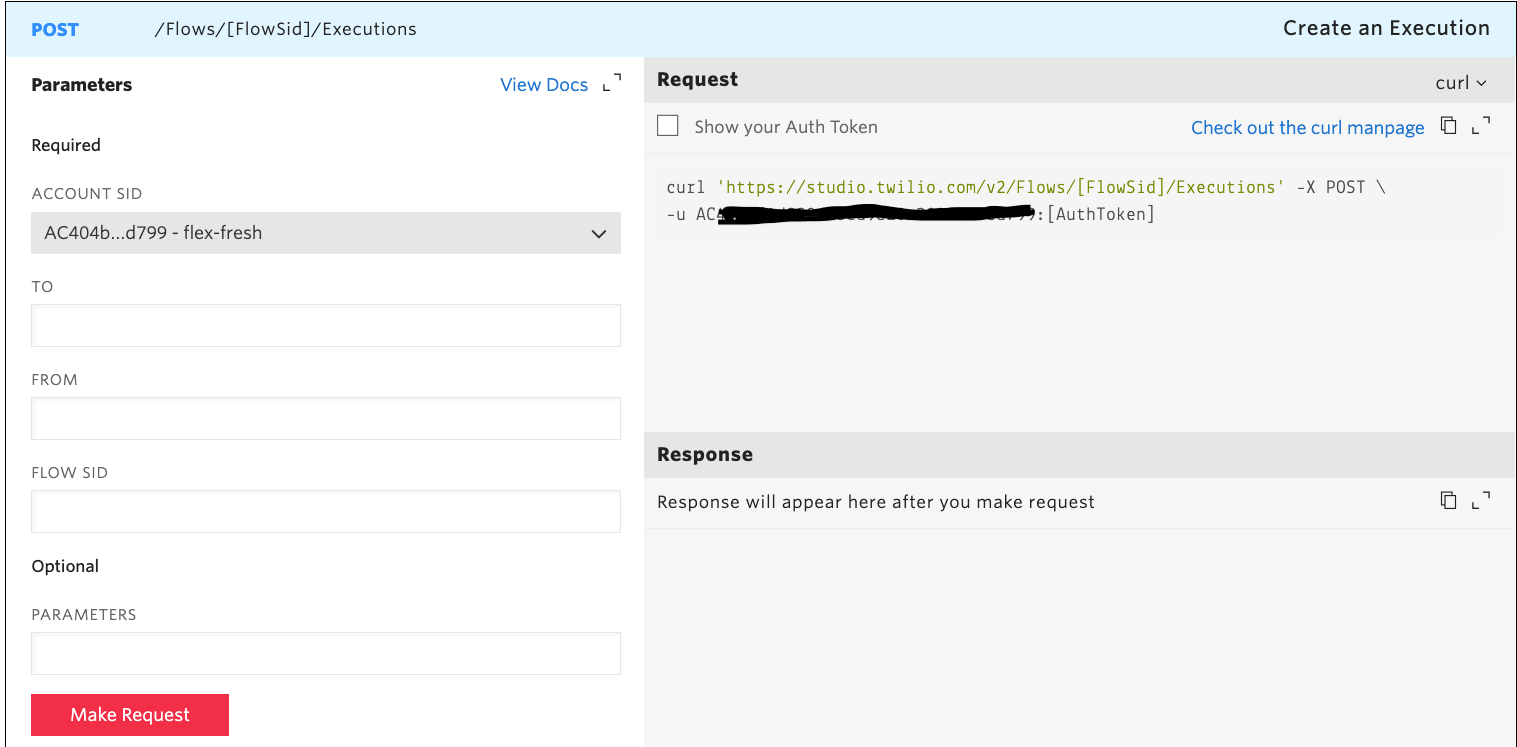
- Enter a phone number for a line you have access to in the “To” field.
- Enter a Twilio phone number that you own in the “From” field. If you do not own a Twilio phone number, scroll up to the Prerequisites section for instructions to buy one.
- Paste a Flow SID you copied above into the Flow SID section.'
- Click Make Request. Your device will receive an SMS or phone call, depending on whether your phone number is mobile or a landline.
Alerting customers based on line type
Congratulations! You now have a serverless Studio flow and Function which can alert customers via SMS or voice call depending on their phone number line type. You can integrate this Flow into your environment to send critical, real-time alerts using the API call referenced above.
To learn more about the power of Studio Flows as a low-code communications tool, check out this Quickstart guide or other existing templates when creating a new Studio Flow. Happy building!
Angela Wieber is a Principal Solutions Engineer at Twilio, where she loves building and educating on cloud-based technologies that enable critical communications. She is based out of Austin, TX, home of the best BBQ and live music venues. She can be reached at awieber [at] twilio.com
Anthony Wong is a Principal Solutions Engineer at Twilio. He’s focused on building integration between Twilio and companies like Salesforce, so adoption is one-click away. He can be found at anwong [at] twilio.com
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.