How to Send an SMS with a Click of a Button with Java
Time to read:
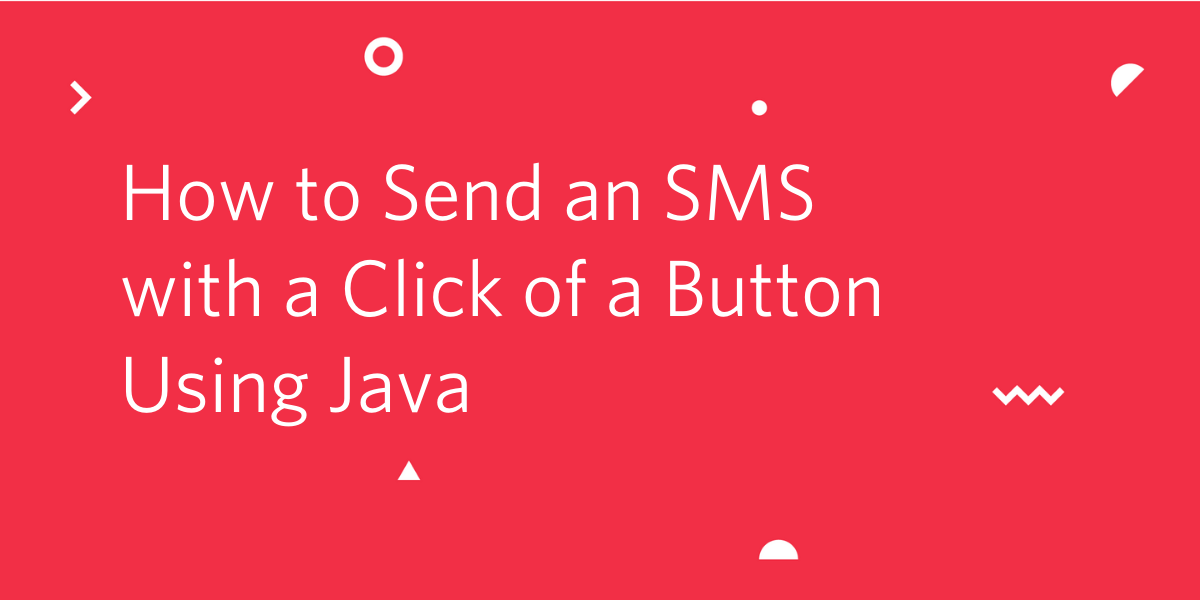
Twilio is all about powering communication and doing it conveniently and fast in any language.
With the help of Twilio and Java, you can deliver a quick message to someone without having to pick up your mobile device. Using Java also gives you the fun opportunity to create Graphical User Interfaces (GUIs) to complement your user's experience in sending an SMS in a more fun and interactive way.
In this article, you'll be implementing a small button GUI that will fire an SMS to your mobile phone device once the button is clicked.
Tutorial requirements
- A free or paid Twilio account. If you are new to Twilio get your free account now! (If you sign up through this link, Twilio will give you $10 credit when you upgrade.)
- Some prior knowledge of Java or a willingness to learn.
- A smartphone with active service to test the project.
Configuration
We’ll start off by creating a directory to store the files of our project. Inside your favorite terminal, enter:
In this article, we will be downloading the standalone Twilio Java helper library. Download a pre-built jar file here. The latest version of the jar files at the time this article was published is twilio-8.18.0-with-dependencies.jar. Scroll down to download the file and save it to your java_sms_button project directory.
Buy a Twilio phone number
If you haven't done so already, purchase a Twilio number to send the SMS.
To do so, log in to the Twilio Console, select Phone Numbers, and then click on the red plus sign to buy a Twilio number. Note that if you are using a free account you will be using your trial credit for this purchase.
In the Buy a Number screen you can select your country and check SMS in the capabilities field. If you’d like to request a number from your region, you can enter your area code in the Number field.
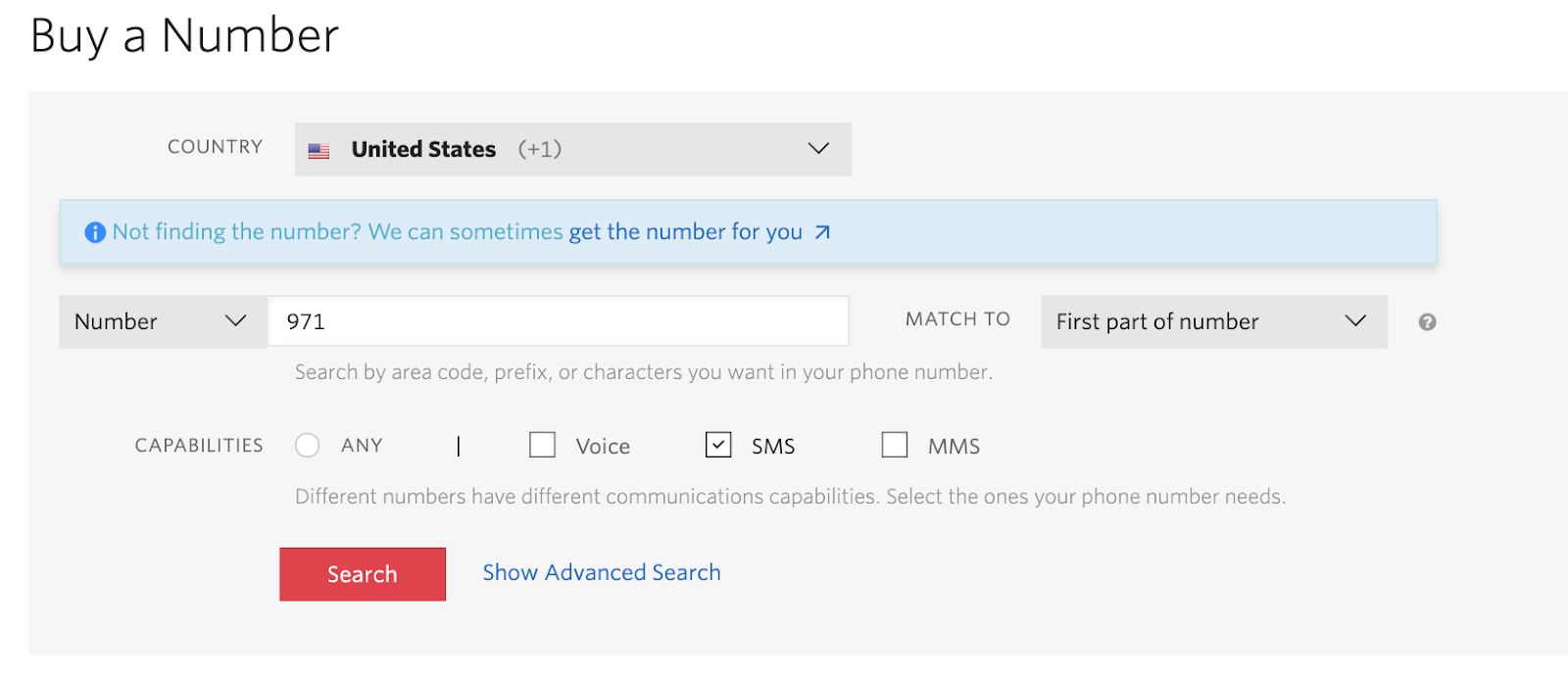
Click the Search button to see what numbers are available, and then click “Buy” for the number that you like from the results. After you confirm your purchase, click the Close button.
Create a button GUI
The goal is to build a clickable button in a GUI so that a user can click to send an SMS to a cell phone number.
Make a new file named SMSButton.java in your project directory and paste the following code to import the necessary packages to handle events:
The javax.swing package is the new version of the AWT
package that allows you to develop GUI applications. The package is necessary to utilize components that create buttons for this project. Although the AWT
package is not used directly, the javax.swing
package requires the java.awt.event package to handle events from the button component.
A class named SMSEventClicker
that implements an ActionListener
is created. The constructor for the class is also defined so that the GUI and button properties object can be used when the user clicks on the SMS button event.
Before this code can be compiled, the functions for createButtonGUI()
and buttonProperties()
must be written. Copy and paste the following code under the class constructor:
This function utilizes the JFrame class from the javax.swing
package in order to create the GUI window container that will pop up and hold the clickable button. Elements of the content pane include the title, visibly, and bounds to determine the width and height. End the function by using the setDefaultCloseOperation
to stop the application once the user closes the window.
Copy and paste the following code to define the buttonProperties
function right beneath the button GUI function:
Similarly, the frame class is called again to create a button with boundaries. However, the important thing here is that the button has to be given specific instructions on what to do. The goal is to have a user click on the button that fires an SMS to a phone number.
Override the button clicking action
As seen earlier in the SMSEventClicker
class definition, the ActionListener
interface is implemented. This allows developers to determine the actions that happen when a user clicks on the button against the ActionEvent
.
A method called actionPerformed()
is invoked when the user clicks on the component, therefore, this method needs to be overridden so that new instructions can be implemented instead.
Go ahead and override the method by copying and pasting the code under the existing code:
Replace <YOUR_PHONE_NUMBER>
and <YOUR_TWILIO_NUMBER>
with your actual numbers, using the E.164 format.
The overridden method ignites the Twilio REST API in order to send an SMS to your personal phone number from your Twilio phone number. This is made possible by creating a new message
instance of the Message
resource. After the message is sent, the message SID is printed on the terminal for reference.
Save the file. Feel free to add to these functions and alter the appearance of the GUI window container and button properties as you please.
Send an SMS with Java and Twilio
In order to finish off the code and send the SMS to a phone number, you must configure the environment with your Twilio account credentials and reference it within the code.
You can find your account credentials on the Twilio Console.
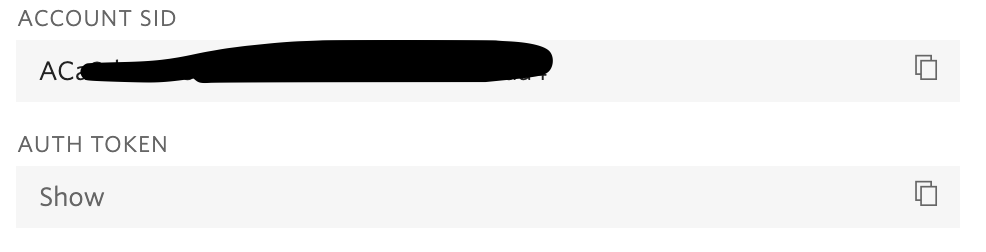
If you are using macOS or Linux, run the commands below to export your account credentials into a twilio.env file. If you decide to commit this project directory, make sure that you do not accidentally commit your credentials to a public repository.
For Windows developers, you would have to replace export
with set
, as seen below:
Feel free to create a .env and .gitignore file as well for your convenience.
Head back to the SMSButton.java file and retrieve the environment variables by creating a new public class that reflects the file name. Copy the following class definition and paste it beneath the definition for the SMSEventClicker
class:
This class is necessary to both retrieve the system environment variables and initiate the Twilio client that will utilize the variables so that the SMS can be sent out successfully.
To end the class definition, an object of the SMSEventClicker
is created. Once the code is compiled, the JVM will start executing the Java program and all of the GUI and button definitions defined earlier in this article will be invoked.
The full code is on my GitHub repository for your reference.
Compile and run the Java program
Save your file and compile the SMSButton
class by typing this command in the terminal:
If you are using macOS or Linux, use the following command:
For Windows developers, use the following command to activate the GUI on your desktop:
Notice that the only difference between the two commands is that the Windows command uses a semicolon instead.
In just a moment, you will see a screen show up with the title "Send an SMS". Go ahead and click the button!
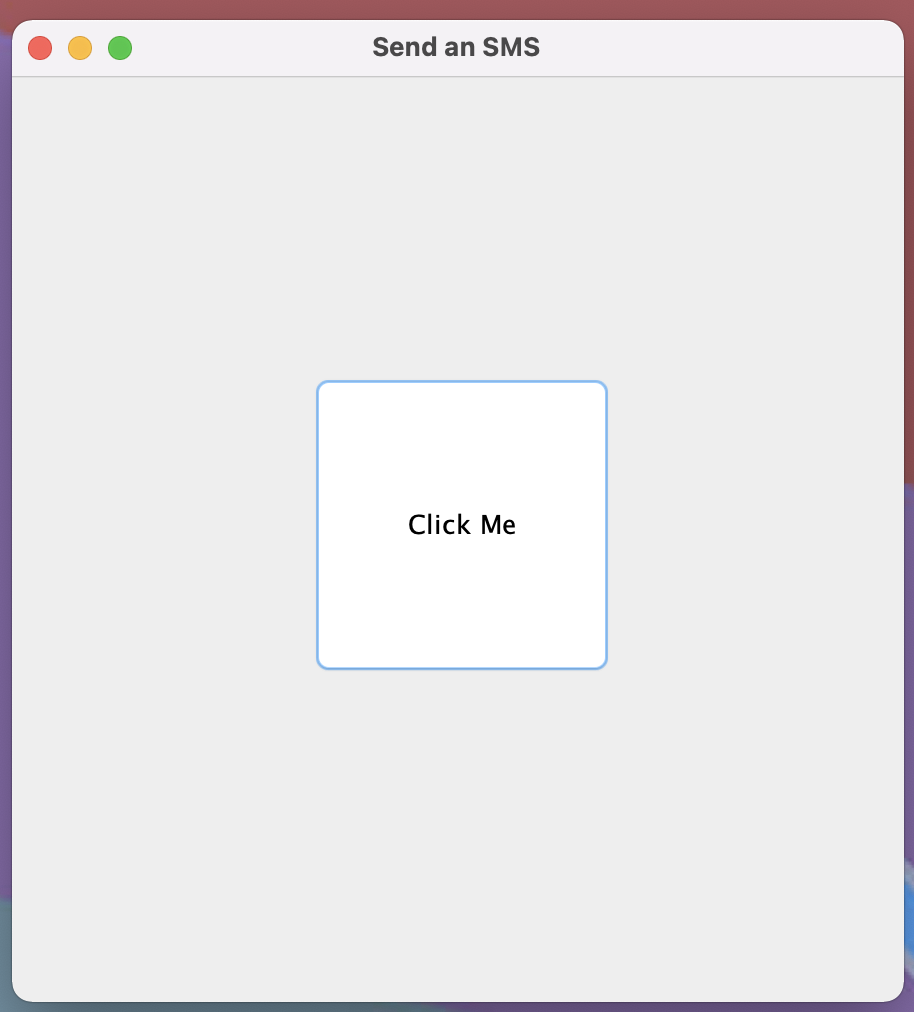
After clicking the button, check your phone for a text message.
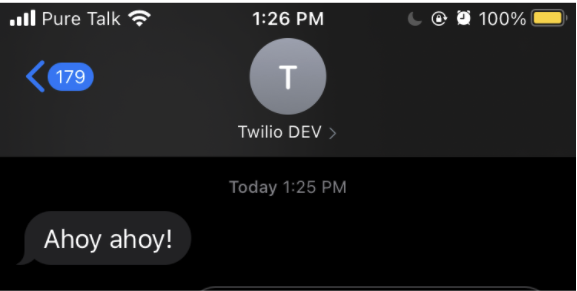
What's next for sending SMS with Java?
Congratulations on writing a short Java program that sends an SMS once a user clicks on the button on screen!
There's so much more you can do with Twilio and Java's many packages. Perhaps you can figure out how to reply to inbound SMS in Java with a click of a button as well.
Explore some other neat Java projects with Twilio:
- Learn how to send bulk SMS with Twilio
- Make the button send a WhatsApp message using Java
- Allow the button to trigger a voice call with Java and Twilio
Let me know about the projects you're building with Java and Twilio by reaching out to me over email!
Diane Phan is a software developer on the Developer Voices team. She loves to help programmers tackle difficult challenges that might prevent them from bringing their projects to life. She can be reached at dphan [at] twilio.com or LinkedIn.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.