Making Voice Calls with Java and Twilio
Time to read: 4 minutes
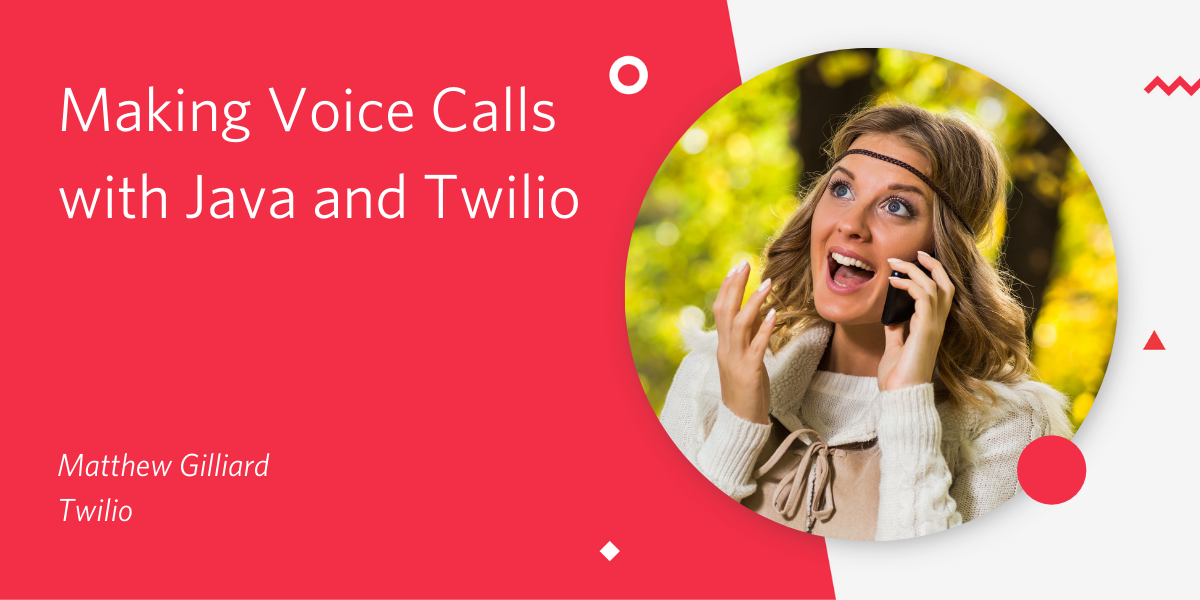
The capability to make outbound calls from code has a long history at Twilio. It will only take you a few minutes to set up a Java project to do just that and see the magic for yourself. To follow along with this post you'll need:
- An installation of Java 8 or newer - I recommend SDKMAN! for installing and managing Java versions.
- A Java IDE - I like IntelliJ IDEA but if you have a favourite that's cool too.
- A Twilio account (if you don't have one yet, sign up for a free account here and receive a $10 credit when you upgrade)
- A Twilio phone number that can send and receive voice calls. You can buy one on your Twilio console.
The easiest way to call the Twilio API is with the Java Helper Library. In this tutorial I'll give examples using the Apache Maven build tool, which will download and manage the dependency and package the project. You can get up to speed with Maven in 5 Minutes, and other tools like Gradle will work, too, if that's what you prefer.
If you want to skip to the end, check out the completed project on GitHub.
Creating a new project
The first thing you need to do is create a new project. Create a new directory and open your IDE.
A new Maven project
You can create a new Maven project in IntelliJ IDEA using these settings:
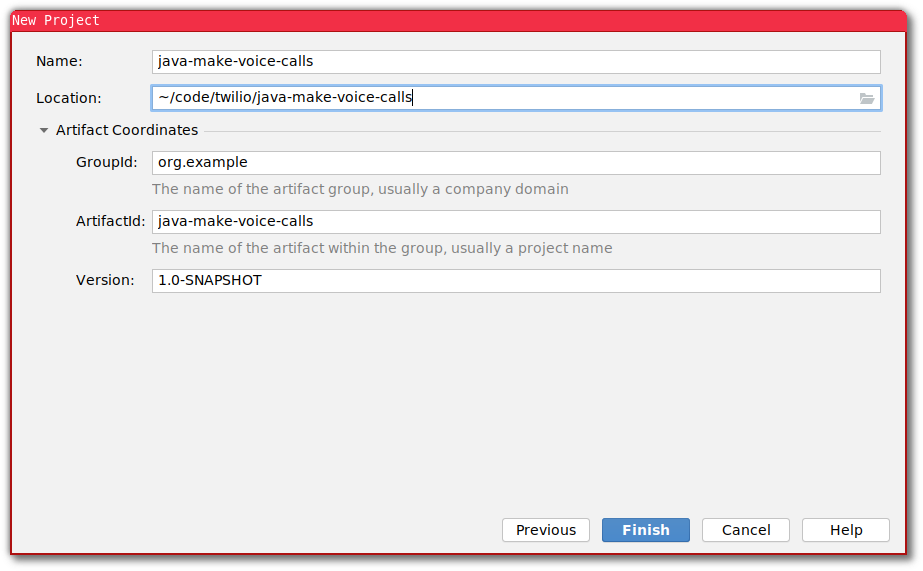
It's also possible to create the same setup from the command line by creating a directory for your source code using the standard directory structure with mkdir -p src/main/java
and a file called pom.xml
with this content:
To finish up the project's configuration, we need to tell Maven to download the Twilio Helper Library for Java and to use Java 8 (any newer version would be fine too). Update the pom.xml
file to make sure that the following properties and dependency are present:
On to the code
Inside src/main/java
create a class called TwilioMakeVoiceCall
with a package of org.example
. The directory structure will be:
Start off the TwilioMakeVoiceCall
class with a main
method:
In the main method, you need to do two things:
- Authenticate the Twilio client with your Account SID and Auth Token
- Call the API to make someone's phone ring
Let's see what that code looks like.
Authenticate the Twilio client
You'll need your ACCOUNT_SID
and AUTH_TOKEN
from your Twilio Console. You should keep these secret, so I'd always recommend reading these from environment variables rather than hard-coding them. I use the EnvFile plugin for IntelliJ IDEA, and there are alternatives for other IDEs or you can set them in your OS. Once they are set in the environment, add this code in the main()
function body:
Make your first call
After you have authenticated your Twilio client, use the following code in your main
method to make a phone call:
[this code including imports on GitHub]
Replace the notes on lines 7 and 8 with real phone numbers, in E.164 format.
This code does three things:
- Creates some instructions for what Twilio should do during the call
- Creates the call, using those instructions and the "to" and "from" phone numbers
- Prints out the call SID
Let's look at these in a bit more detail.
Instructions for the call
Twilio takes instructions in a language we call TwiML, short for Twilio Markup Language The TwiML generated by the code above is:
This is the "Hello World" of TwiML - there are a lot more possibilities. You can Say messages, Play recordings, Record the call, Gather speech or DTMF tones, create Conference calls, Stream audio to another service, and do a lot more.
Creating the call
The Call.creator(...)
method above takes the "to" and "from" numbers as well as the TwiML code. In this case, we've provided the TwiML directly as a String
. Another option is passing a URL instead of TwiML. Twilio will make a request to that URL, passing in metadata about the call, and the response should contain the TwiML that will be used.
There are a few options for hosting TwiML on Twilio:
- TwiML Bins: fixed TwiML, useful for quick prototyping or simple services.
- Twilio Functions: a serverless JavaScript platform which can have complex logic or call out to other online services
For the ultimate flexibility you can host your own web service using any stack you like. As long as you return valid TwiML with a content-type of application/xml
you're good to go.
The call SID
Every call that Twilio makes or receives is given a String Identifier (SID). You can use the SID to look up the details of the call (status, duration, cost) in the Call Logs page on the Twilio console.
Summing up
Now you know how to make calls with Java and Twilio something you could learn next might be how to handle inbound calls. Or perhaps you'd like to learn how to send and receive SMS messages.
It's time to put your imagination to work — what will you build next? Whatever you make with Java and Twilio, I'd love to hear about it! Please get in touch:
I can't wait to see what you build!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.