Make a Phone Call from a Bash Script using Twilio Programmable Voice
Time to read: 4 minutes
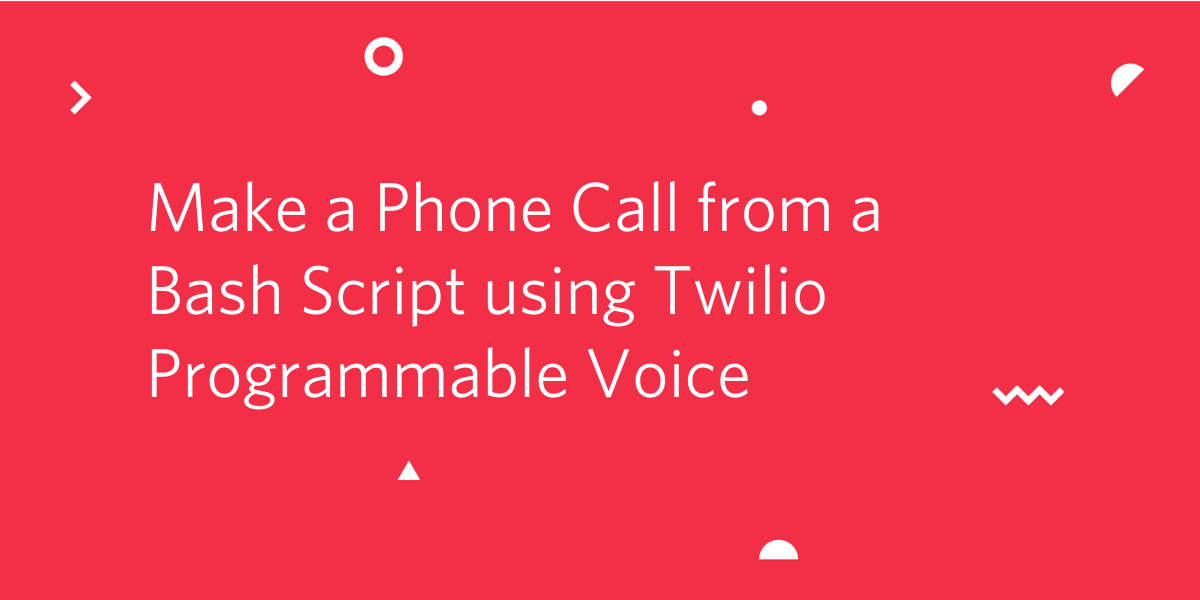
Twilio Programmable Voice allows you to make voice calls directly from your application or script. In this tutorial, I’ll show you how to use it to make a voice call that plays a text-to-speech message from Bash or other shell scripting languages.
Prerequisites
- A Twilio account. If you are new to Twilio, click here to create a free account now and receive $10 credit when you upgrade to a paid account. You can review the features and limitations of a free Twilio account.
- A phone with active service that can receive phone calls, to test the project.
Set up your environment
In this section you are going to set up a brand new project. To keep things nicely organized, open a terminal or command prompt, find a suitable place and create a new directory where the project you are about to create will live:
To be able to access the Twilio service, the shell script will need access to your Twilio account credentials, to log in and authenticate. The most secure way to define these credentials is to add them as environment variables.
The information that you need is the “Account SID” and the “Auth Token”. You can find both in the main dashboard of the Twilio Console, under “Account Info”:
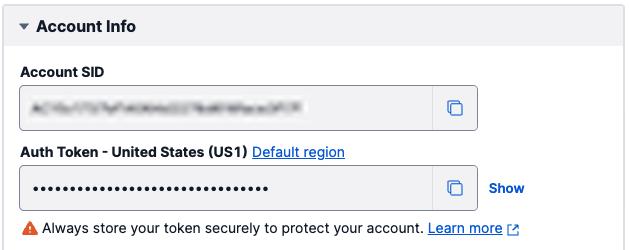
In your terminal, define two environment variables called TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
and set them to your account credentials:
If you want to learn more about environment variables, check out the How to Set Environment Variables tutorial.
Buy a Twilio phone number
To be able to make a phone call, you need to have a phone number associated with your Twilio account to call from. Log in to the Twilio Console, and select Phone Numbers. If you already have one or more numbers listed, you can use one of those. If you don’t have a number yet, click on the “Buy a number” button to buy a Twilio number. Note that if you are using a free account, you will be using your trial credit for this purchase.
In the “Buy a Number” page, select your country and check Voice under the capabilities field. If you’d like to request a number from your region, you can enter your area code in the Number field.
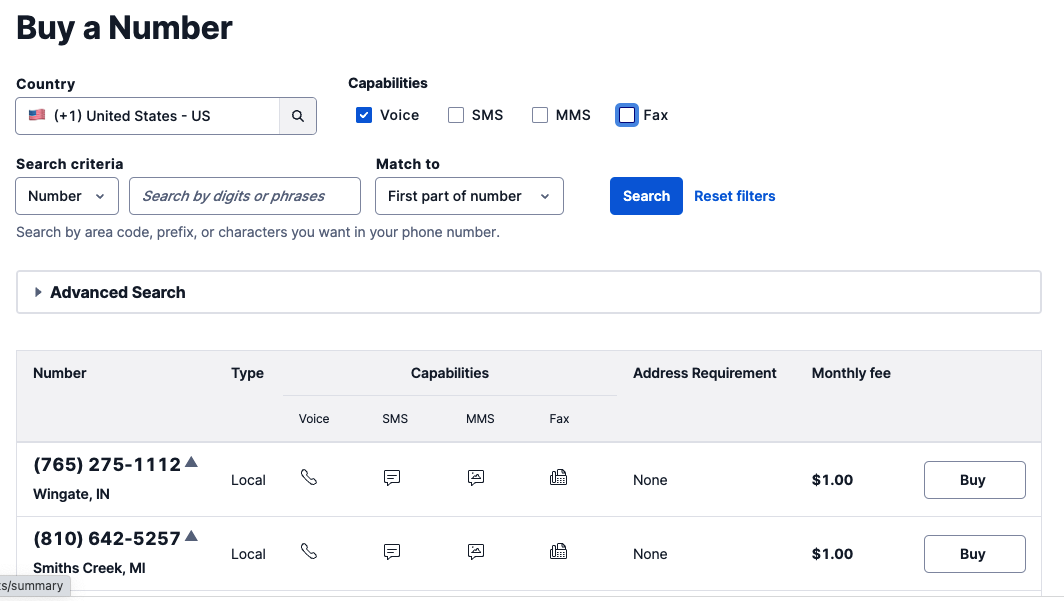
Click the “Search” button to see what numbers are available, and then click “Buy” for the number that you like from the results. After you confirm your purchase, click the “Close” button.
Create a TwiML Bin
TwiML is Twilio’s markup language, an extension to XML that is used to provide instructions to Twilio on how certain events need to be handled. When a call is made from the Twilio phone number you purchased above, you have to provide a URL that returns TwiML instructions, and Twilio will follow these instructions to handle the phone call.
You can host TwiML directly through Twilio, in a TwiML Bin. Navigate to the TwiML Bins section of the Console.
Click the “Create new TwiML Bin” button (or the “+” button) to create a new bin. This will direct you to a new page where you can configure your bin.
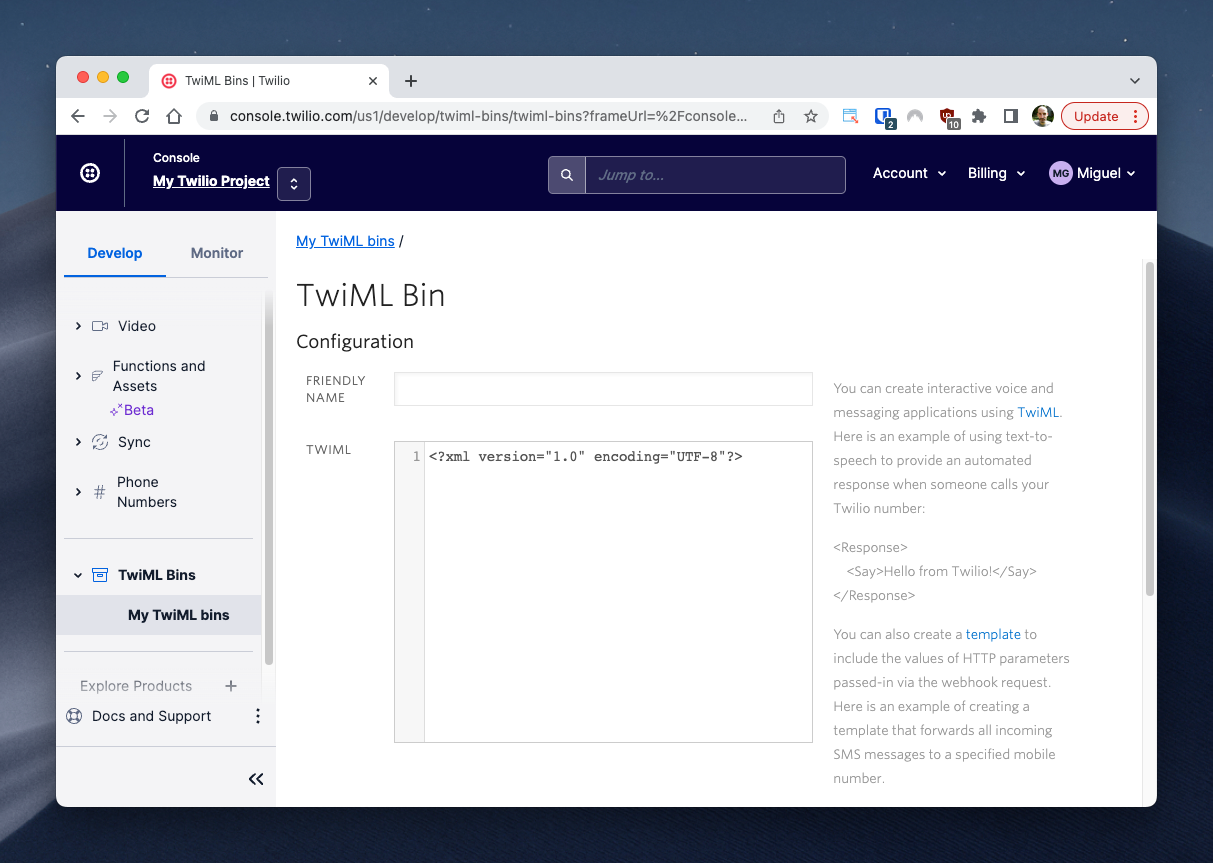
Give your bin any friendly name of your choosing, such as bash-call. Then copy and paste the following TwiML into the TwiML field, replacing anything there already:
Scroll down and click the “Create” button. The page will refresh and then at the top of the new page, you will see the “SID” and “URL” values assigned to your new TwiML bin. Copy the URL field to the clipboard.
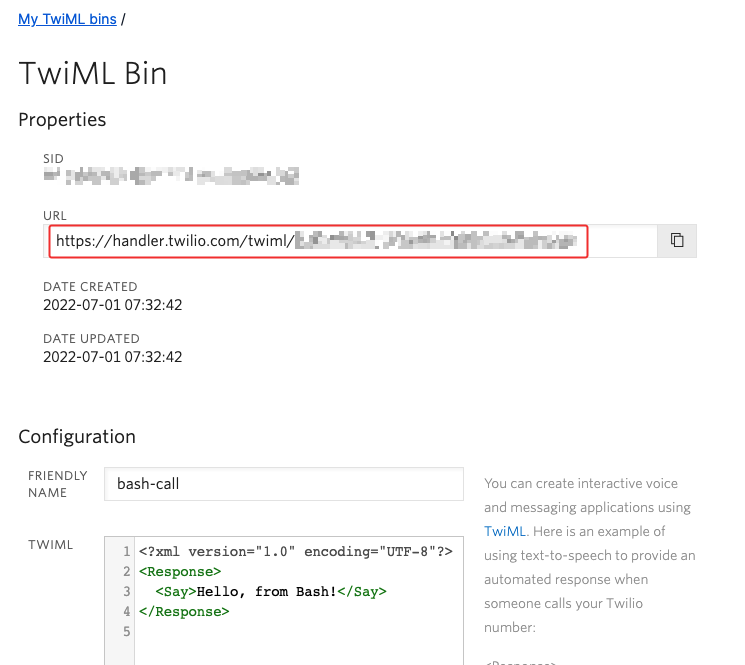
Making a phone call from a shell script
Fire up your text editor or IDE and create a new file in the phone-call directory you created at the start of the tutorial. You can call it call.sh or any other name that you like.
Enter the following code in this file:
Make sure you update the following parts of the above code snippet:
- Replace
<TWIML-BIN-URL>
with the URL you just copied from your TwiML bin - Replace
<YOUR-PHONE-NUMBER>
with your personal phone number - Replace
<YOUR-TWILIO-PHONE-NUMBER>
with the Twilio phone number you purchased earlier
For the two phone numbers, enter them in the E.164 format, which includes a plus sign, followed by country code, area code and local number.
Head back to your terminal, make sure you have defined the environment variables with your Twilio credentials as indicated above, and then run the script as follows:
The script will prompt you to press Enter to make a phone call. A few seconds after you press this key, your personal phone will ring. When you answer the call, you’ll hear “Hello, from Bash!”, which is the message entered inside the <Say>
element in the TwiML bin.
The phone call was triggered with a request sent to the Twilio API. The curl
command was used to make this request. This command should be pre-installed in your system, but in the odd case it isn’t, you can install it from here.
Now that you have made your first phone call, feel free to go back to your TwiML bin in the Console and edit the message you want the recipient to hear.
Conclusion
In this tutorial you’ve learned how to make a phone call from a shell script. But this is just the beginning. Be sure to check the TwiML reference to find out many more cool things you can add to your scripted phone calls. An interesting improvement you can attempt is to replace the Url
parameter, which provides a link to the TwiML Bin, with the Twiml
parameter, which accepts TwiML code directly. This would allow your script to dynamically generate the message that is played when the recipient picks up.
I can’t wait to see what you build!
Miguel Grinberg is a Principal Software Engineer for Technical Content at Twilio. Reach out to him at mgrinberg [at] twilio [dot] com if you have a cool project you’d like to share on this blog!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.