A Simple Way to Receive an SMS with PHP and Twilio
Time to read: 4 minutes
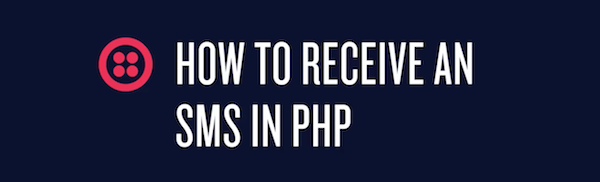
In this tutorial we’ll receive and reply to a text message in PHP. If you’re the type who likes to skip to the punchline, create a file named message.php and paste in this code:
To learn more about how this works, watch this one minute video, or just keep reading.
Twilio’s HTTP Request
When someone texts your Twilio number, Twilio makes an HTTP request to your app. Your app parses the request, then sends back an HTTP response with instructions for what Twilio should do next.
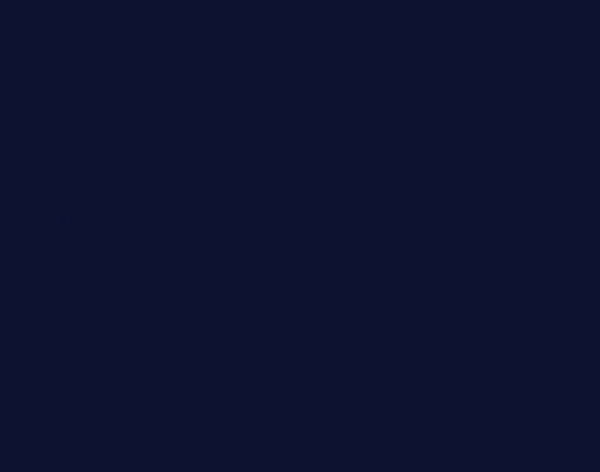
Details about the inbound SMS, such as the body of the message and the number it was sent from, are passed via the request parameters. To receive and parse Twilio’s request, create a file called message.php and pluck the From and Body from the $_POST parameters:
A common mistake is to forget to capitalize the first letter of the keys ( ‘From’ , ‘Body’), so be careful there.
When Twilio makes an HTTP request to your server, it expects an HTTP response in return. This response takes the form of TwiML, a subset of XML used to relay instructions back to Twilio. Thus, we start our response by setting the Content-Type header to text/xml.
Valid TwiML has instructions nested inside opening and closing <Response> tags (even if you don’t want Twilio to do anything, you should still send back an empty <Response></Response>). In this case, we use the <Message> tag to reply with an SMS that uses the $number and $body.
And that’s all the code we need to receive and reply to a text message. That said, there’s lots more you can do with TwiML. If you’d like to learn more, check out the docs.
Your message.php needs to have a publicly accessible URL for Twilio to reach it, so push it to a server or use ngrok to open a tunnel to localhost. Then we just need to point a Twilio number at that URL.
Setup Twilio Phone Number
Sign up for a free Twilio account if you don’t have one.
Buy a phone number, then click Setup Number. Scroll to the Messaging section and find the line that says “A Message Comes In.”
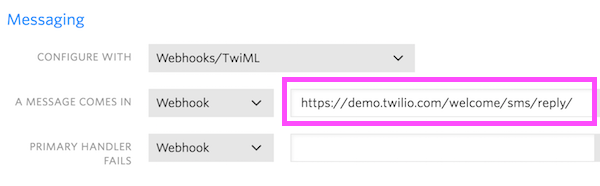
Fill in the full path to your file (i.e., https://yourserver.com/message.php) and click Save.
Now, send an SMS to your shiny new phone number number and revel in the customized response that comes back your way.
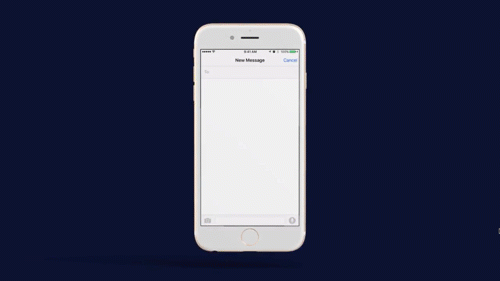
Next Steps
If you’d like to dive deeper into the world of Twilio SMS, I’d recommend you check out:
If you have any questions, drop me a line at gb@twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.