Send Scheduled Emails from Python and Flask with Twilio SendGrid
Time to read: 3 minutes
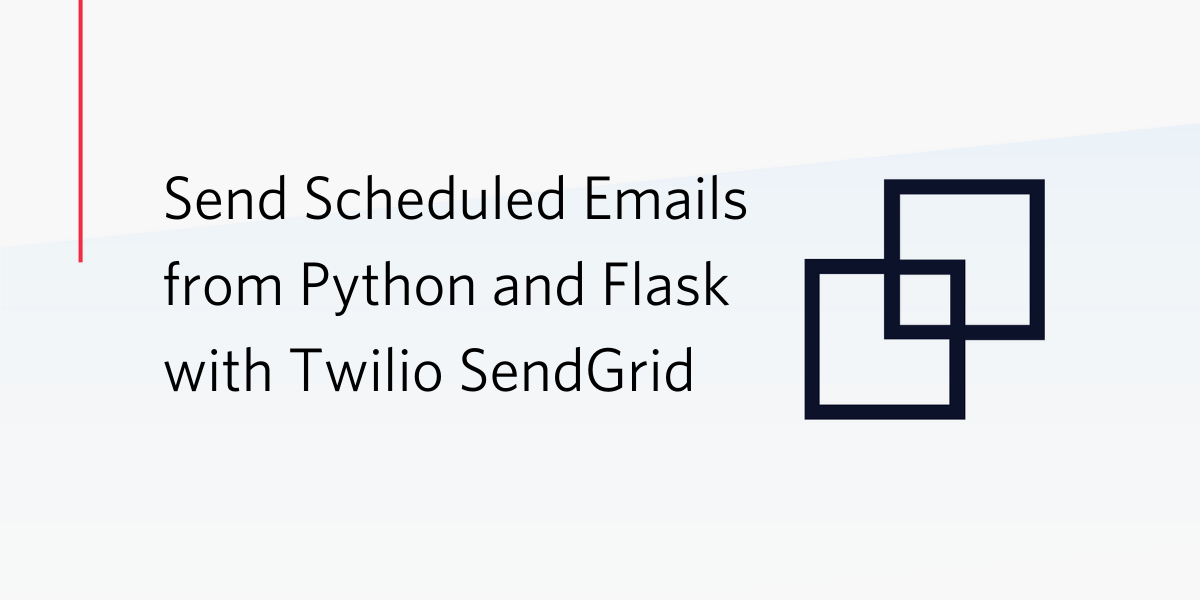
Twilio SendGrid eliminates many of the complexities of sending email. In a previous tutorial, you learned how to use SendGrid’s SMTP server to send emails to your users from a Python and Flask application. But how do you schedule your emails so that they are sent at a specific time?
In this short tutorial you will learn how to use SendGrid’s email scheduling options, which will save you from having to implement your own background scheduling.
Requirements
To work on this tutorial you will need the following items:
- Python 3.6 or newer. If your operating system does not provide a Python 3.6+ interpreter, you can go to python.org to download an installer.
- A free Twilio SendGrid account. If you are new to Twilio Sendgrid you can create a trial account. With a trial account you can send 100 emails per day forever.
Create a Flask project
Find an appropriate location for your project and create a directory for it:
Now create a Python virtual environment where the dependencies of the project are to be installed. For Mac and Unix users, the commands are:
For Windows users, the commands are:
For this project, you are going to use Flask, the Flask-Mail extension and the python-dotenv package. Install them all in your virtual environment:
For the purposes of this tutorial, the following Flask application will suffice. Create and open a file named app.py and enter the following code in it using your favorite text editor or IDE:
This short application configures all the email related settings so that you can send emails through your SendGrid account. Note how the email password is sourced from an environment variable. You will define this variable in the next section.
SendGrid configuration
To send emails with SendGrid you need to authenticate with an API key. If you don’t have one yet, log in to your SendGrid account, then click on the left sidebar, select Settings and then API Keys. Click the “Create API Key” button and provide the requested information to create your key. For detailed step-by-step instructions, follow the basic tutorial first.
Once you have your key, create and open a .env file in your Flask project directory, and paste the key as follows:
Send a test email
You are now ready to send a test email. Open a Flask shell with the following command:
Now you can configure and send a test email from the Python prompt. First, create a message object and set the subject, sender and recipients:
Here you should replace youremail@example.com
with a valid email address you have access to. If you prefer, you can use different email addresses for the sender and the recipient. You can also use multiple recipient addresses if you like.
Next, set the body of the email:
The body
attribute defines a text-only email. If you want to also provide a rich-text version in HTML, you can assign it to the msg.html
attribute.
Your message is now complete. You can send it as follows:
In a few seconds, the email should arrive in your inbox. The original Flask email sending tutorial has a “If Your Emails Aren’t Delivered” section that describes how to troubleshoot emails that aren’t delivered.
Schedule an email
You are now ready to schedule an email, from the same shell session. Create a new message:
And here comes the magic. You can use the send_at
extension from SendGrid to provide a delivery time in Unix timestamp units. In the following example, the email is scheduled to be sent two minutes later:
That’s it! The X-SMTPAPI
custom header is an extension supported by SendGrid’s SMTP server that allows applications to pass additional sending instructions as a JSON blob. The expression time() + 120
refers to the current time plus 120 seconds, or in other words, two minutes from now. Send the email like you did before:
Nothing will happen immediately, but about two minutes later you should have the scheduled email in your inbox.
The send_at
option is documented in detail. In the same page you can also learn about send_each_at
, which allows you to provide a list of timestamps, one per recipient in a multi-recipient email.
Conclusion
I hope this was a useful trick that you can add to your email sending toolbox. The X-SMTPAPI
header supports several other extensions, so be sure to check its documentation to learn about more cool email features.
Happy email scheduling!
Miguel Grinberg is a Principal Software Engineer for Technical Content at Twilio. Reach out to him at mgrinberg [at] twilio [dot] com if you have a cool project you’d like to share on this blog!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.