Send Inspirational Quotes Every Morning in PHP Using Cron, SendGrid, Twilio SMS and Quote APIs
Time to read: 4 minutes
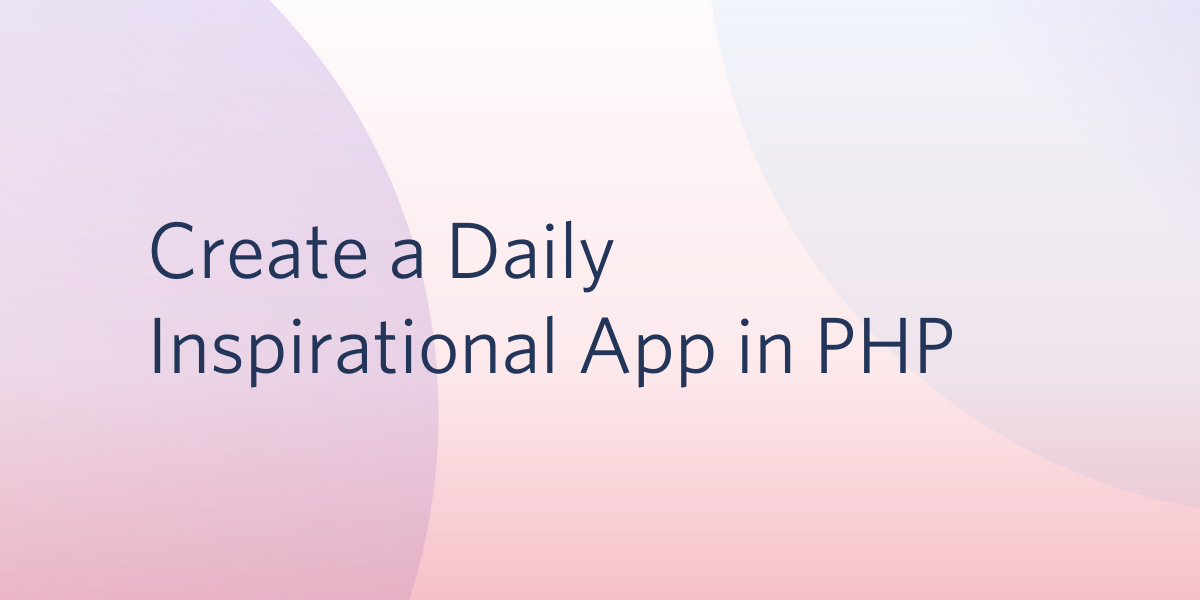
Ever had one of those mornings when you woke up unmotivated, but read a quote and the energy returned? That is the power of positive and motivational quotes.
Motivational quotes have a way of making people feel inspired and energized. This phenomenon can be attributed to a combination of factors such as motivational psychology, wordsmithing, and self-selection.
In this tutorial, I am going to teach you how to create an app that automatically sends you motivational quotes every morning. Before we start, here is a motivational quote to uplift you as you follow along.
“Believe in yourself! Have faith in your abilities! Without humble but reasonable confidence in your own powers, you cannot be successful or happy.” – Norman Vincent Peale
Getting Started
To complete this tutorial, you will need the following:
Create a New Laravel Project
Let’s create a new Laravel project by running the following command:
Set up the Twilio SDK and SendGrid Mail Driver
First, we need to install the Twilio PHP SDK using Composer. In your terminal, run the command below:
Next, we need to set up our Laravel application to use SendGrid as the mail driver. Here is a link to a quick guide on how to set up SendGrid as your SMTP relay in Laravel.
After you have completed the setup of your SMTP relay, we will install Guzzle to execute the HTTP API requests. If you are unfamiliar, Guzzle is a great alternative to writing your server-side requests in cURL. Run the command below to install the latest version:
Once these steps are complete, update the .env
file with the credentials from your Twilio and SendGrid dashboards as shown below:
Create the Quote App
Let's flesh out the Quote model. Under normal circumstances, our model would primarily be responsible for reading and writing data into the table. We will extend its functionality with custom methods to fetch quotes from the forismatic and favqs APIs and send quotes. Finally, we will create the Quote artisan command to exploit our methods and provide CLI access to them.
Let's jump right in and get motivated :)
Create the Quote Model
Run the command below to create the model:
Create the Logic to Fetch and Send the Quotes
Because our goal is to generate diverse, random quotes, we will use a single function to fetch a quote from both the forismatic and favqs APIs. Open the app/Quote.php
file and add the following method.
In the code above, a GET
request is made to the two APIs. From the responses, the quote and author are extracted. The function then returns an array containing two quotes.
Next, we need to create a function to send the fetched quotes via SMS and email them using Twilio and SendGrid respectively.
Add the code below to the Quote model:
The sendSMS()
function accepts two parameters; the number of the recipient and the quotes in the form of the SMS body. Those parameters are then used to send an SMS using your Twilio enabled phone number.
Next, let's create the function to send the quotes via email. In app/Quote.php
add the following code:
The sendEmail()
function accepts two parameters; the email address of the recipient and the email body and then sends the email using a Mailable class. We’ll break down what a Mailable class is in the next section.
Creating a Mailable Class
In Laravel, each type of email sent by your application is represented as a "mailable" class. These classes are stored in the app/Mail
directory.
In your terminal, run the artisan command below:
The command generates a new file app/Mail/EmailQuote.php
.
The Mailable class has a build function where we can define the configurations for our email, indicate the template view file to be used, and also pass any needed parameters to our view template.
Update the file as shown below:
Next, create an emails
folder under resources/views
and add a file quote.blade.php
.
Update the file as shown below:
Consolidating the App
Our app is now taking shape. Since we have all of the components needed to build the app, let’s put everything together. Reopen the Quote
model in app/Quotes.php
and add this final method:
The Quote Artisan Command
Since the app needs to send quotes every day, we need to create a way to automate the process through task scheduling. In Laravel, we can achieve this through a number of ways notably through scheduling artisan commands, Queue jobs, shell commands e.t.c.
I have opted to create a custom artisan command due to its simplicity, but you can opt for any of the options available.
Laravel's command scheduler allows you to fluently and expressively define your command schedule within Laravel itself. When using the scheduler, only a single Cron entry is needed on your server. Your task schedule is defined in the app/Console/Kernel.php
file's schedule
method.
Run the artisan command below to generate the artisan command:
This command created a new command class in the app/Console/Commands
directory.
Open the file app/Console/Commands/sendQuote.php
and update the signature
variable and the handle
method as shown below:
Next, update app/Console/Kernel.php
file's schedule
method with this single line of code.
This command simply means, call php artisan send:quote
(the command we have just created) daily at 6.00 am in the morning.
Finally, add the following Cron entry to your server.
This Cron will call the Laravel command scheduler every minute. When the schedule:run
command is executed, Laravel will evaluate your scheduled tasks and runs the tasks that are due.
Testing
To test the final app, navigate to your project directory and run the artisan command below:
If all went well, you should get both an SMS and an email.
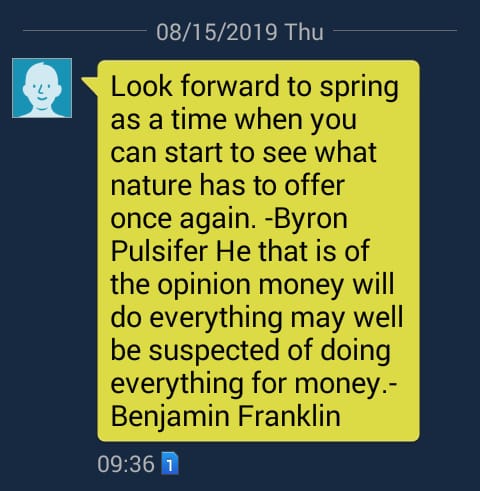
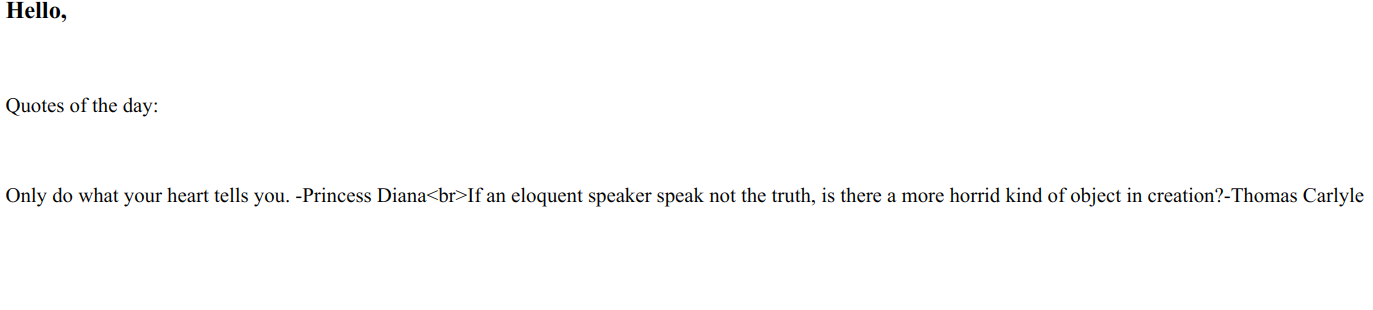
Conclusion
The aim of this tutorial is to keep things as simple as possible. To build upon this app, you can create an online portal where users can subscribe to get the quote of the day by providing either a phone number or email. The code is available on Github.
Have some ideas to better improve the app? Let me know. I can’t wait to see what you build.
Feel free to reach out to me:
- GitHub: mikelidbary
- Web: www.talwork.net
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.