Send Recurring Emails in PHP with SendGrid and Laravel 7 Task Scheduler
Time to read: 4 minutes
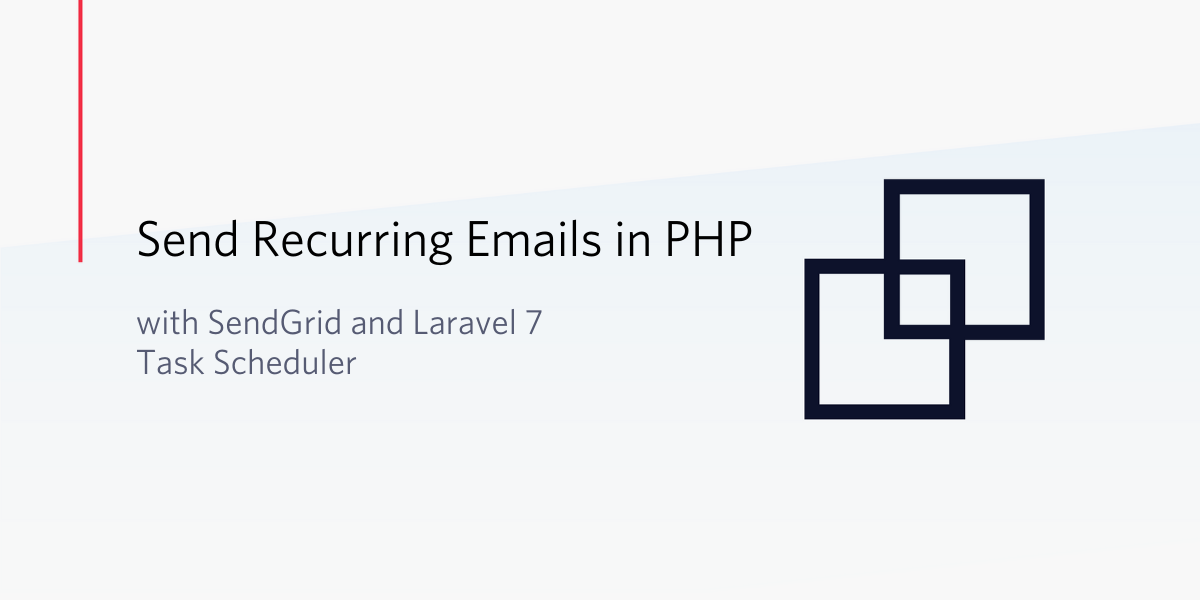
Occasionally, your application needs to perform some routine tasks in timed intervals such as sending out weekly reports to an administrator or sending monthly updates to your users.
Traditionally, such routine tasks are carried out via individual cron jobs that trigger a shell script or the part of the code to be executed. As the application grows and the number of tasks we need to run increases, cron jobs quickly become difficult to maintain, especially as they don’t come with error reporting/handling features and they can’t be placed in a Version Control System such as Git.
The Laravel Task Scheduler allows us to define our tasks as code while leveraging all of the logging and error handling features available in the framework. With the scheduler, we only need to define a single cron entry that executes the schedule at an interval.
What are we Building?
In this tutorial, we will be building an application that uses SendGrid and the Laravel Task Scheduler to send daily reports of the number of new signups each day.
Pre-requisites
To complete this tutorial you will need:
- Basic understanding of the Laravel framework
- Composer installed
- MySQL (or your preferred database engine) installed
- A SendGrid account (Create a new account here)
Setting up our Sample Application
Create a new Laravel application using Composer and enter into the directory.
Next, open your favorite IDE and update the database-related variables in your .env
file with your setup configuration.
This assumes that there is an existing database named daily_metrics
. If none exists, you can create it at this point.
By default, Laravel comes with an auto-generated users migration file at database/migrations/2014_10_12_00000_create_users_table.php
. This would be the only migration file we need as it contains all that we need for this application. Run php artisan migrate
in the project root to apply the schema defined in the migration file to your database. It will generate the users table with the columns defined in the up
method of the file.
Add the Laravel Authentication
Now that the users table has been created, we need a way to register users. Laravel 6+ has decoupled authentication by creating a package to scaffold all of the routes and views needed for registration. In the project directory, run the following commands:
After successful installation, launch the Laravel application by running php artisan serve
and navigate to http://127.0.0.1:8000/
to register a user. We will need to have a user added to the database in order to test the application at the end of the tutorial.
Installing and Setting Up the SendGrid Library
The SendGrid SDK helps us to easily use the SendGrid API in our PHP applications, thus, we would be using it in place of the built-in Laravel Mail. Install the library with the command below:
Next, retrieve your SendGrid API key from your SendGrid API Keys Settings and append it to your .env
file, alongside your preferred recipient email and name as shown:
Creating our Recurring Task
Tasks for the Scheduler can be defined in various forms including Closures, Queued Jobs, Artisan commands, and PHP invokable objects. In this case, we would be using an invokable object as it provides enough flexibility for us to achieve what we plan to do.
Create a new Tasks
directory in your app
folder i.e, in the same directory as Console
and Http
and add the SendDailyMetrics.php
file in the new folder by running the commands below in your terminal.
In your editor, open the SendDailyMetrics.php
file and add the code block below to it. Comments have been added to aid inclarity.
The __invoke
magic method above is what makes instances of the SendDailyMetrics
class invocable objects. They are executed when such instances are called as functions (which is how the Laravel Task Scheduler invokes them).
When the __invoke
method is called, we first retrieve the number of users that registered the previous day and the number that registered on the current day. Also, we calculate the percentage based on these two numbers which are then sent to the admin email as the day’s metric.
Next, we will update the schedule
method of our Console’s Kernel class to execute the task everyday at 11:59 PM, and send whatever output it has to a log file we have explicitly specified. That way, Laravel is also aware of our new Task. Open the Kernel.php
file in the app/Console/
directory and replace it’s schedule
method with the code below:
NOTE: Remember to import the SendDailyMetrics
class.
Testing our Application
Our application requires only the cron entry below to execute any number of tasks that are available. It will execute the scheduler every minute, which will in turn, go through the scheduled tasks and execute the ones that are due
NOTE: If you are unfamiliar with adding cron entries, the cron man page could give you a headstart or you can consider using tools like Laravel Forge.
While developing locally, you can lower the schedule frequency to a minute and directly execute php artisan schedule:run
to get the email immediately.
Conclusion
Recurring tasks are a frequently needed feature of modern applications and this tutorial provides a guide on implementing such features with Laravel, while also going through how to send emails with the Twilio SendGrid SDK.
Michael Okoko is a student currently pursuing a degree in Computer Science and Mathematics at Obafemi Awolowo University, Nigeria. He loves open source and is mostly interested in Linux, Golang, PHP, and fantasy novels! You can reach him via:
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.